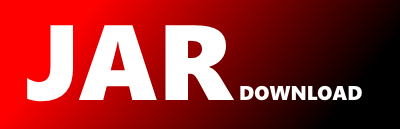
com.files.net.FilesHttpInterceptor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of files-sdk Show documentation
Show all versions of files-sdk Show documentation
The Files.com Java client library provides convenient access to the Files.com API from JVM based applications.
package com.files.net;
import com.files.FilesConfig;
import com.files.exceptions.ApiErrorException;
import java.io.IOException;
import okhttp3.Interceptor;
import okhttp3.Request;
import okhttp3.Response;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class FilesHttpInterceptor implements Interceptor {
protected static final Logger log = LoggerFactory.getLogger(FilesHttpInterceptor.class);
private final String userAgent;
private final FilesConfig filesConfig = FilesConfig.getInstance();
public FilesHttpInterceptor() {
this.userAgent = filesConfig.getUserAgent();
}
@Override
public Response intercept(Chain chain) throws IOException {
Request originalRequest = chain.request();
Request.Builder modifiedAgentRequest = originalRequest.newBuilder();
// Add User Agent Header
modifiedAgentRequest.header("User-Agent", userAgent);
// Try the request
Response response;
int attempts = 0;
Integer status = null;
while (true) {
try {
attempts++;
response = chain.proceed(modifiedAgentRequest.build());
status = response.code();
if (status != 429 || status <= 501 || attempts >= filesConfig.getUpstreamMaxAttempts()) {
break;
}
log.warn(String.format("HttpClient received retry condition of [%s].", status));
} catch (IOException e) {
log.error(String.format("HttpClient received retry error condition due to IOException of [%s]", e.getMessage()));
}
try {
Thread.sleep((long) (Math.pow(2, attempts - 2) * filesConfig.getInitialRetryDelayMillis()));
} catch (InterruptedException e) {
throw new IOException("Http Request Interrupted");
}
}
if ((status >= 400 && status <= 600) || status == null) {
String error = "An error occured.";
log.error(String.format("Http Returned Status %d", status));
try {
error = response.body().string();
} catch (Exception e) {
// INOP to catch any connection closed errors.
} finally {
response.body().close();
}
throw ApiErrorException.forStatus(status, error);
}
return response;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy