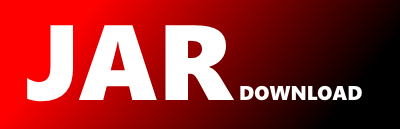
com.files.models.ApiKey Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of files-sdk Show documentation
Show all versions of files-sdk Show documentation
The Files.com Java client library provides convenient access to the Files.com API from JVM based applications.
package com.files.models;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.MapperFeature;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.ObjectReader;
import com.fasterxml.jackson.databind.json.JsonMapper;
import com.files.FilesClient;
import com.files.FilesConfig;
import com.files.net.HttpMethods.RequestMethods;
import com.files.util.FilesInputStream;
import com.files.util.ModelUtils;
import java.io.BufferedInputStream;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.net.URI;
import java.net.URISyntaxException;
import java.util.Date;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import lombok.Getter;
import lombok.Setter;
@JsonIgnoreProperties(ignoreUnknown = true)
public class ApiKey {
private HashMap options;
private ObjectMapper objectMapper = JsonMapper
.builder()
.disable(MapperFeature.CAN_OVERRIDE_ACCESS_MODIFIERS)
.build();
public ApiKey() {
this(null, null);
}
public ApiKey(HashMap parameters) {
this(parameters, null);
}
public ApiKey(HashMap parameters, HashMap options) {
this.options = options;
try {
ObjectReader objectReader = objectMapper.readerForUpdating(this);
objectReader.readValue(objectMapper.writeValueAsString(parameters));
} catch (JsonProcessingException e) {
// TODO: error generation on constructor
}
}
/**
* API Key ID
*/
@Getter
@Setter
@JsonProperty("id")
public Long id;
/**
* Unique label that describes this API key. Useful for external systems where you may have API keys from multiple accounts and want a human-readable label for each key.
*/
@Getter
@Setter
@JsonProperty("descriptive_label")
public String descriptiveLabel;
/**
* User-supplied description of API key.
*/
@Getter
@Setter
@JsonProperty("description")
public String description;
/**
* Time which API Key was created
*/
@Getter
@JsonProperty("created_at")
public Date createdAt;
/**
* API Key expiration date
*/
@Getter
@Setter
@JsonProperty("expires_at")
public Date expiresAt;
/**
* API Key actual key string
*/
@Getter
@Setter
@JsonProperty("key")
public String key;
/**
* API Key last used - note this value is only updated once per 3 hour period, so the 'actual' time of last use may be up to 3 hours later than this timestamp.
*/
@Getter
@Setter
@JsonProperty("last_use_at")
public Date lastUseAt;
/**
* Internal name for the API Key. For your use.
*/
@Getter
@Setter
@JsonProperty("name")
public String name;
/**
* Folder path restriction for this api key. This must be slash-delimited, but it must neither start nor end with a slash. Maximum of 5000 characters.
*/
@Getter
@Setter
@JsonProperty("path")
public String path;
/**
* Permissions for this API Key. Keys with the `desktop_app` permission set only have the ability to do the functions provided in our Desktop App (File and Share Link operations). Additional permission sets may become available in the future, such as for a Site Admin to give a key with no administrator privileges. If you have ideas for permission sets, please let us know.
*/
@Getter
@Setter
@JsonProperty("permission_set")
public String permissionSet;
/**
* If this API key represents a Desktop app, what platform was it created on?
*/
@Getter
@Setter
@JsonProperty("platform")
public String platform;
/**
* URL for API host.
*/
@Getter
@Setter
@JsonProperty("url")
public String url;
/**
* User ID for the owner of this API Key. May be blank for Site-wide API Keys.
*/
@Getter
@Setter
@JsonProperty("user_id")
public Long userId;
/**
* Parameters:
* name - string - Internal name for the API Key. For your use.
* description - string - User-supplied description of API key.
* expires_at - string - API Key expiration date
* permission_set - string - Permissions for this API Key. Keys with the `desktop_app` permission set only have the ability to do the functions provided in our Desktop App (File and Share Link operations). Additional permission sets may become available in the future, such as for a Site Admin to give a key with no administrator privileges. If you have ideas for permission sets, please let us know.
*/
public ApiKey update(HashMap parameters) {
return update(parameters);
}
/**
*/
public ApiKey delete(HashMap parameters) {
return delete(parameters);
}
public void destroy(HashMap parameters) {
delete(parameters);
}
public void save() throws IOException {
HashMap parameters = ModelUtils.toParameterMap(objectMapper.writeValueAsString(this));
if (parameters.containsKey("id") && parameters.get("id") != null) {
update(parameters);
} else {
ApiKey newObject = ApiKey.create(parameters, this.options);
}
}
/**
* Parameters:
* user_id - int64 - User ID. Provide a value of `0` to operate the current session's user.
* cursor - string - Used for pagination. When a list request has more records available, cursors are provided in the response headers `X-Files-Cursor-Next` and `X-Files-Cursor-Prev`. Send one of those cursor value here to resume an existing list from the next available record. Note: many of our SDKs have iterator methods that will automatically handle cursor-based pagination.
* per_page - int64 - Number of records to show per page. (Max: 10,000, 1,000 or less is recommended).
* sort_by - object - If set, sort records by the specified field in either `asc` or `desc` direction (e.g. `sort_by[expires_at]=desc`). Valid fields are `expires_at`.
* filter - object - If set, return records where the specified field is equal to the supplied value. Valid fields are `expires_at`.
* filter_gt - object - If set, return records where the specified field is greater than the supplied value. Valid fields are `expires_at`.
* filter_gteq - object - If set, return records where the specified field is greater than or equal the supplied value. Valid fields are `expires_at`.
* filter_lt - object - If set, return records where the specified field is less than the supplied value. Valid fields are `expires_at`.
* filter_lteq - object - If set, return records where the specified field is less than or equal the supplied value. Valid fields are `expires_at`.
*/
public static List list() throws IOException {
return list(null, null);
}
public static List list(HashMap parameters) throws IOException {
return list(parameters, null);
}
public static List list(HashMap parameters, HashMap options) throws IOException {
parameters = parameters != null ? parameters : new HashMap();
options = options != null ? options : new HashMap();
if (parameters.containsKey("user_id") && !(parameters.get("user_id") instanceof Long)) {
throw new IllegalArgumentException("Bad parameter: user_id must be of type Long parameters[\"user_id\"]");
}
if (parameters.containsKey("cursor") && !(parameters.get("cursor") instanceof String)) {
throw new IllegalArgumentException("Bad parameter: cursor must be of type String parameters[\"cursor\"]");
}
if (parameters.containsKey("per_page") && !(parameters.get("per_page") instanceof Long)) {
throw new IllegalArgumentException("Bad parameter: per_page must be of type Long parameters[\"per_page\"]");
}
if (parameters.containsKey("sort_by") && !(parameters.get("sort_by") instanceof Map)) {
throw new IllegalArgumentException("Bad parameter: sort_by must be of type Map parameters[\"sort_by\"]");
}
if (parameters.containsKey("filter") && !(parameters.get("filter") instanceof Map)) {
throw new IllegalArgumentException("Bad parameter: filter must be of type Map parameters[\"filter\"]");
}
if (parameters.containsKey("filter_gt") && !(parameters.get("filter_gt") instanceof Map)) {
throw new IllegalArgumentException("Bad parameter: filter_gt must be of type Map parameters[\"filter_gt\"]");
}
if (parameters.containsKey("filter_gteq") && !(parameters.get("filter_gteq") instanceof Map)) {
throw new IllegalArgumentException("Bad parameter: filter_gteq must be of type Map parameters[\"filter_gteq\"]");
}
if (parameters.containsKey("filter_lt") && !(parameters.get("filter_lt") instanceof Map)) {
throw new IllegalArgumentException("Bad parameter: filter_lt must be of type Map parameters[\"filter_lt\"]");
}
if (parameters.containsKey("filter_lteq") && !(parameters.get("filter_lteq") instanceof Map)) {
throw new IllegalArgumentException("Bad parameter: filter_lteq must be of type Map parameters[\"filter_lteq\"]");
}
String url = String.format("%s%s/api_keys", FilesConfig.getInstance().getApiRoot(), FilesConfig.getInstance().getApiBase());
TypeReference> typeReference = new TypeReference>() {};
return FilesClient.requestList(url, RequestMethods.GET, typeReference, parameters, options);
}
public static List all() throws IOException {
return all(null, null);
}
public static List all(HashMap parameters, HashMap options) throws IOException {
return list(parameters, options);
}
/**
*/
public static List findCurrent() throws IOException {
return findCurrent(null, null);
}
public static List findCurrent(HashMap parameters) throws IOException {
return findCurrent(parameters, null);
}
public static List findCurrent(HashMap parameters, HashMap options) throws IOException {
parameters = parameters != null ? parameters : new HashMap();
options = options != null ? options : new HashMap();
String url = String.format("%s%s/api_key", FilesConfig.getInstance().getApiRoot(), FilesConfig.getInstance().getApiBase());
TypeReference> typeReference = new TypeReference>() {};
return FilesClient.requestList(url, RequestMethods.GET, typeReference, parameters, options);
}
/**
* Parameters:
* id (required) - int64 - Api Key ID.
*/
public static List find() throws IOException {
return find(null, null, null);
}
public static List find(Long id, HashMap parameters) throws IOException {
return find(id, parameters, null);
}
public static List find(HashMap parameters, HashMap options) throws IOException {
return find(null, parameters, options);
}
public static List find(Long id, HashMap parameters, HashMap options) throws IOException {
parameters = parameters != null ? parameters : new HashMap();
options = options != null ? options : new HashMap();
if (id == null && parameters.containsKey("id") && parameters.get("id") != null) {
id = (Long) parameters.get("id");
}
if (!(id instanceof Long)) {
throw new IllegalArgumentException("Bad parameter: id must be of type Long parameters[\"id\"]");
}
if (id == null) {
throw new NullPointerException("Argument or Parameter missing: id parameters[\"id\"]");
}
String urlParts[] = {FilesConfig.getInstance().getApiRoot(), FilesConfig.getInstance().getApiBase(), String.valueOf(id)};
for (int i = 2; i < urlParts.length; i++) {
try {
urlParts[i] = new URI(null, null, urlParts[i], null).getRawPath();
} catch (URISyntaxException ex) {
// NOOP
}
}
String url = String.format("%s%s/api_keys/%s", urlParts);
TypeReference> typeReference = new TypeReference>() {};
return FilesClient.requestList(url, RequestMethods.GET, typeReference, parameters, options);
}
public static List get() throws IOException {
return get(null, null, null);
}
public static List get(Long id, HashMap parameters, HashMap options) throws IOException {
return find(id, parameters, options);
}
/**
* Parameters:
* user_id - int64 - User ID. Provide a value of `0` to operate the current session's user.
* name - string - Internal name for the API Key. For your use.
* description - string - User-supplied description of API key.
* expires_at - string - API Key expiration date
* permission_set - string - Permissions for this API Key. Keys with the `desktop_app` permission set only have the ability to do the functions provided in our Desktop App (File and Share Link operations). Additional permission sets may become available in the future, such as for a Site Admin to give a key with no administrator privileges. If you have ideas for permission sets, please let us know.
* path - string - Folder path restriction for this api key.
*/
public static ApiKey create() throws IOException {
return create(null, null);
}
public static ApiKey create(HashMap parameters) throws IOException {
return create(parameters, null);
}
public static ApiKey create(HashMap parameters, HashMap options) throws IOException {
parameters = parameters != null ? parameters : new HashMap();
options = options != null ? options : new HashMap();
if (parameters.containsKey("user_id") && !(parameters.get("user_id") instanceof Long)) {
throw new IllegalArgumentException("Bad parameter: user_id must be of type Long parameters[\"user_id\"]");
}
if (parameters.containsKey("name") && !(parameters.get("name") instanceof String)) {
throw new IllegalArgumentException("Bad parameter: name must be of type String parameters[\"name\"]");
}
if (parameters.containsKey("description") && !(parameters.get("description") instanceof String)) {
throw new IllegalArgumentException("Bad parameter: description must be of type String parameters[\"description\"]");
}
if (parameters.containsKey("expires_at") && !(parameters.get("expires_at") instanceof String)) {
throw new IllegalArgumentException("Bad parameter: expires_at must be of type String parameters[\"expires_at\"]");
}
if (parameters.containsKey("permission_set") && !(parameters.get("permission_set") instanceof String)) {
throw new IllegalArgumentException("Bad parameter: permission_set must be of type String parameters[\"permission_set\"]");
}
if (parameters.containsKey("path") && !(parameters.get("path") instanceof String)) {
throw new IllegalArgumentException("Bad parameter: path must be of type String parameters[\"path\"]");
}
String url = String.format("%s%s/api_keys", FilesConfig.getInstance().getApiRoot(), FilesConfig.getInstance().getApiBase());
TypeReference typeReference = new TypeReference() {};
return FilesClient.requestItem(url, RequestMethods.POST, typeReference, parameters, options);
}
/**
* Parameters:
* expires_at - string - API Key expiration date
* name - string - Internal name for the API Key. For your use.
* permission_set - string - Permissions for this API Key. Keys with the `desktop_app` permission set only have the ability to do the functions provided in our Desktop App (File and Share Link operations). Additional permission sets may become available in the future, such as for a Site Admin to give a key with no administrator privileges. If you have ideas for permission sets, please let us know.
*/
public static ApiKey updateCurrent() throws IOException {
return updateCurrent(null, null);
}
public static ApiKey updateCurrent(HashMap parameters) throws IOException {
return updateCurrent(parameters, null);
}
public static ApiKey updateCurrent(HashMap parameters, HashMap options) throws IOException {
parameters = parameters != null ? parameters : new HashMap();
options = options != null ? options : new HashMap();
if (parameters.containsKey("expires_at") && !(parameters.get("expires_at") instanceof String)) {
throw new IllegalArgumentException("Bad parameter: expires_at must be of type String parameters[\"expires_at\"]");
}
if (parameters.containsKey("name") && !(parameters.get("name") instanceof String)) {
throw new IllegalArgumentException("Bad parameter: name must be of type String parameters[\"name\"]");
}
if (parameters.containsKey("permission_set") && !(parameters.get("permission_set") instanceof String)) {
throw new IllegalArgumentException("Bad parameter: permission_set must be of type String parameters[\"permission_set\"]");
}
String url = String.format("%s%s/api_key", FilesConfig.getInstance().getApiRoot(), FilesConfig.getInstance().getApiBase());
TypeReference typeReference = new TypeReference() {};
return FilesClient.requestItem(url, RequestMethods.PATCH, typeReference, parameters, options);
}
/**
* Parameters:
* name - string - Internal name for the API Key. For your use.
* description - string - User-supplied description of API key.
* expires_at - string - API Key expiration date
* permission_set - string - Permissions for this API Key. Keys with the `desktop_app` permission set only have the ability to do the functions provided in our Desktop App (File and Share Link operations). Additional permission sets may become available in the future, such as for a Site Admin to give a key with no administrator privileges. If you have ideas for permission sets, please let us know.
*/
public static ApiKey update() throws IOException {
return update(null, null, null);
}
public static ApiKey update(Long id, HashMap parameters) throws IOException {
return update(id, parameters, null);
}
public static ApiKey update(HashMap parameters, HashMap options) throws IOException {
return update(null, parameters, options);
}
public static ApiKey update(Long id, HashMap parameters, HashMap options) throws IOException {
parameters = parameters != null ? parameters : new HashMap();
options = options != null ? options : new HashMap();
if (id == null && parameters.containsKey("id") && parameters.get("id") != null) {
id = (Long) parameters.get("id");
}
if (!(id instanceof Long)) {
throw new IllegalArgumentException("Bad parameter: id must be of type Long parameters[\"id\"]");
}
if (parameters.containsKey("name") && !(parameters.get("name") instanceof String)) {
throw new IllegalArgumentException("Bad parameter: name must be of type String parameters[\"name\"]");
}
if (parameters.containsKey("description") && !(parameters.get("description") instanceof String)) {
throw new IllegalArgumentException("Bad parameter: description must be of type String parameters[\"description\"]");
}
if (parameters.containsKey("expires_at") && !(parameters.get("expires_at") instanceof String)) {
throw new IllegalArgumentException("Bad parameter: expires_at must be of type String parameters[\"expires_at\"]");
}
if (parameters.containsKey("permission_set") && !(parameters.get("permission_set") instanceof String)) {
throw new IllegalArgumentException("Bad parameter: permission_set must be of type String parameters[\"permission_set\"]");
}
if (id == null) {
throw new NullPointerException("Argument or Parameter missing: id parameters[\"id\"]");
}
String urlParts[] = {FilesConfig.getInstance().getApiRoot(), FilesConfig.getInstance().getApiBase(), String.valueOf(id)};
for (int i = 2; i < urlParts.length; i++) {
try {
urlParts[i] = new URI(null, null, urlParts[i], null).getRawPath();
} catch (URISyntaxException ex) {
// NOOP
}
}
String url = String.format("%s%s/api_keys/%s", urlParts);
TypeReference typeReference = new TypeReference() {};
return FilesClient.requestItem(url, RequestMethods.PATCH, typeReference, parameters, options);
}
/**
*/
public static ApiKey deleteCurrent() throws IOException {
return deleteCurrent(null, null);
}
public static ApiKey deleteCurrent(HashMap parameters) throws IOException {
return deleteCurrent(parameters, null);
}
public static ApiKey deleteCurrent(HashMap parameters, HashMap options) throws IOException {
parameters = parameters != null ? parameters : new HashMap();
options = options != null ? options : new HashMap();
String url = String.format("%s%s/api_key", FilesConfig.getInstance().getApiRoot(), FilesConfig.getInstance().getApiBase());
TypeReference typeReference = new TypeReference() {};
return FilesClient.requestItem(url, RequestMethods.DELETE, typeReference, parameters, options);
}
/**
*/
public static ApiKey delete() throws IOException {
return delete(null, null, null);
}
public static ApiKey delete(Long id, HashMap parameters) throws IOException {
return delete(id, parameters, null);
}
public static ApiKey delete(HashMap parameters, HashMap options) throws IOException {
return delete(null, parameters, options);
}
public static ApiKey delete(Long id, HashMap parameters, HashMap options) throws IOException {
parameters = parameters != null ? parameters : new HashMap();
options = options != null ? options : new HashMap();
if (id == null && parameters.containsKey("id") && parameters.get("id") != null) {
id = (Long) parameters.get("id");
}
if (!(id instanceof Long)) {
throw new IllegalArgumentException("Bad parameter: id must be of type Long parameters[\"id\"]");
}
if (id == null) {
throw new NullPointerException("Argument or Parameter missing: id parameters[\"id\"]");
}
String urlParts[] = {FilesConfig.getInstance().getApiRoot(), FilesConfig.getInstance().getApiBase(), String.valueOf(id)};
for (int i = 2; i < urlParts.length; i++) {
try {
urlParts[i] = new URI(null, null, urlParts[i], null).getRawPath();
} catch (URISyntaxException ex) {
// NOOP
}
}
String url = String.format("%s%s/api_keys/%s", urlParts);
TypeReference typeReference = new TypeReference() {};
return FilesClient.requestItem(url, RequestMethods.DELETE, typeReference, parameters, options);
}
public static ApiKey destroy() throws IOException {
return destroy(null, null, null);
}
public static ApiKey destroy(Long id, HashMap parameters, HashMap options) throws IOException {
return delete(id, parameters, options);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy