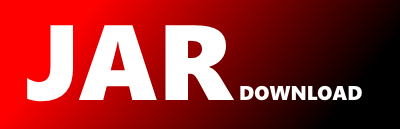
com.files.ListIterator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of files-sdk Show documentation
Show all versions of files-sdk Show documentation
The Files.com Java client library provides convenient access to the Files.com API from JVM based applications.
package com.files;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.MapperFeature;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.json.JsonMapper;
import com.files.models.ModelInterface;
import com.files.net.FilesResponse;
import com.files.net.HttpMethods.RequestMethods;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
public class ListIterator implements Iterable {
private final String url;
private final RequestMethods requestType;
private final TypeReference> className;
private final HashMap parameters;
private final HashMap options;
private String cursor;
private final ObjectMapper objectMapper = JsonMapper
.builder()
.disable(MapperFeature.CAN_OVERRIDE_ACCESS_MODIFIERS)
.build();
public List data = new ArrayList();
public ListIterator(String url, RequestMethods requestType, TypeReference> className,
HashMap parameters, HashMap options) {
this.url = url;
this.requestType = requestType;
this.className = className;
this.parameters = parameters;
this.options = options;
}
public Boolean hasNextPage() {
return this.cursor != null;
}
@Override
public Iterator iterator() {
return this.data.iterator();
}
public ListIterator loadNextPage() throws RuntimeException {
if (this.cursor != null) {
this.parameters.put("cursor", this.cursor);
}
FilesResponse response = FilesClient.apiRequest(this.url, this.requestType, this.parameters, this.options);
try {
this.data = objectMapper.readValue(response.getBody(), this.className);
this.data.forEach(item -> {
if (item instanceof ModelInterface) {
((ModelInterface) item).setOptions(this.options);
}
});
} catch (JsonProcessingException e) {
throw new RuntimeException(e);
}
if (response.getHeaders().containsKey("X-Files-Cursor")) {
this.cursor = response.getHeaders().get("X-Files-Cursor").get(0);
} else {
this.cursor = null;
}
return this;
}
public ListIteratorIterable listAutoPaging() {
return new ListIteratorIterable(this);
}
public List all() throws RuntimeException {
List allData = new ArrayList();
// Force starting from the beginning
this.cursor = null;
do {
this.loadNextPage();
allData.addAll(data);
} while (this.cursor != null);
return allData;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy