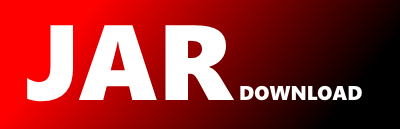
com.findwise.hydra.Document Maven / Gradle / Ivy
package com.findwise.hydra;
import java.util.Map;
import java.util.Set;
public interface Document extends JsonDeserializer, JsonSerializer {
public enum Action {
ADD, DELETE, UPDATE
};
enum Status { PROCESSING, PROCESSED, DISCARDED, FAILED, PENDING };
String ID_KEY = "_id";
String ACTION_KEY = "_action";
String CONTENTS_KEY = "contents";
String METADATA_KEY = "metadata";
String COMMITTING_METADATA_FLAG = "committing";
String PENDING_METADATA_FLAG = "pending";
String PROCESSED_METADATA_FLAG = "processed";
String DISCARDED_METADATA_FLAG = "discarded";
String FETCHED_METADATA_TAG = "fetched";
String TOUCHED_METADATA_TAG = "touched";
String FAILED_METADATA_FLAG = "failed";
String DATE_METADATA_SUBKEY = "date";
String STAGE_METADATA_SUBKEY = "stage";
String ERROR_METADATA_KEY = "error";
Action getAction();
void setAction(Action action);
Status getStatus();
boolean hasContentField(String fieldName);
boolean hasMetadataField(String fieldName);
DocumentID getID();
Object putContentField(String fieldName, Object value);
Object getContentField(String fieldName);
Map getMetadataMap();
Map getContentMap();
void addError(String from, Throwable t);
boolean hasErrors();
Set getContentFields();
void putAll(Document> d);
boolean isEqual(Document> d);
/**
* Causes all subsequent requests to hasContentField for this field to return false.
* Subsequent calls to getContentFields() should also exclude this field.
*/
Object removeContentField(String key);
void clear();
/**
* Implementations are expected to handle data on the form on the form: {
* Document.ID_KEY : <id>, Document.CONTENTS_KEY : { ... },
* Document.METADATA_KEY : { ... } }
*/
@Override
void fromJson(String json) throws JsonException;
/**
* Implementations are expected to output data on the form on the form: {
* Document.ID_KEY : <id>, Document.CONTENTS_KEY : { ... },
* Document.METADATA_KEY : { ... } }
*/
@Override
String toJson();
/**
* Returns a JSON representation of the requested content fields, and the ID
* of this document.
*
* Nullsafe.
*/
String contentFieldsToJson(Iterable contentFields);
/**
* Returns a JSON representation of the requested metadata fields, and the
* ID of this document.
*
* Nullsafe.
*/
String metadataFieldsToJson(Iterable contentFields);
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy