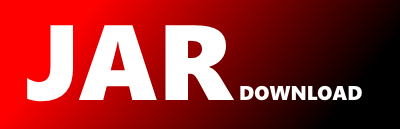
com.findwise.hydra.local.LocalQuery Maven / Gradle / Ivy
package com.findwise.hydra.local;
import java.util.HashMap;
import java.util.Map;
import com.findwise.hydra.JsonDeserializer;
import com.findwise.hydra.JsonException;
import com.findwise.hydra.Query;
import com.findwise.hydra.SerializationUtils;
import com.findwise.hydra.Document.Action;
import com.google.gson.JsonParseException;
public class LocalQuery implements Query, JsonDeserializer {
private Map equals;
private Map notEquals;
private Map exists;
private Map touched;
private Action action = null;
public LocalQuery() {
equals = new HashMap();
notEquals = new HashMap();
exists = new HashMap();
touched = new HashMap();
}
public LocalQuery(String json) throws JsonException {
this();
fromJson(json);
}
public Map getContentsExists() {
return exists;
}
public Map getTouched() {
return touched;
}
public Map getContentsEquals() {
return equals;
}
public Map getEquals() {
return equals;
}
public Map getContentNotEquals() {
return notEquals;
}
public Map getNotEquals() {
return notEquals;
}
public Map getExists() {
return exists;
}
public Action getAction() {
return action;
}
@Override
public void requireContentFieldExists(String fieldName) {
getContentsExists().put(fieldName, true);
}
@Override
public void requireContentFieldNotExists(String fieldName) {
getContentsExists().put(fieldName, false);
}
@Override
public void requireContentFieldEquals(String fieldName, Object o) {
getContentsEquals().put(fieldName, o);
}
@Override
public void requireContentFieldNotEquals(String fieldName, Object o) {
getContentNotEquals().put(fieldName, o);
}
@Override
public void requireTouchedByStage(String stageName) {
getTouched().put(stageName, true);
}
@Override
public void requireNotTouchedByStage(String stageName) {
getTouched().put(stageName, false);
}
@Override
public void requireAction(Action action) {
this.action = action;
}
public String toJson() {
Map x = new HashMap();
x.put("equals", equals);
x.put("notEquals", notEquals);
x.put("exists", exists);
x.put("touched", touched);
if(action!=null) {
x.put("action", action.toString());
}
return SerializationUtils.toJson(x);
}
@SuppressWarnings({ "unchecked" })
@Override
public void fromJson(String json) throws JsonException{
try {
Map queryObject = (Map) SerializationUtils.fromJson(json);
if(queryObject.containsKey("equals")) {
equals = (Map) queryObject.get("equals");
}
if(queryObject.containsKey("notEquals")) {
notEquals = (Map) queryObject.get("notEquals");
}
if(queryObject.containsKey("exists")) {
exists = (Map) queryObject.get("exists");
}
if(queryObject.containsKey("touched")) {
touched = (Map) queryObject.get("touched");
}
if(queryObject.containsKey("action")) {
action = Action.valueOf((String)queryObject.get("action"));
}
}
catch(JsonParseException jse) {
throw new JsonException(jse);
}
}
@Override
public String toString() {
return toJson();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy