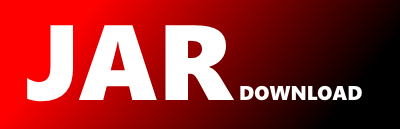
com.finovertech.factern.api.client.MapJsonObject Maven / Gradle / Ivy
package com.finovertech.factern.api.client;
import com.finovertech.factern.api.client.data.Account;
import com.finovertech.factern.api.client.data.Account.AccountNode;
import com.finovertech.factern.api.client.data.Application;
import com.finovertech.factern.api.client.data.Application.ApplicationNode;
import com.finovertech.factern.api.client.data.Comment;
import com.finovertech.factern.api.client.data.Comment.CommentNode;
import com.finovertech.factern.api.client.data.CommentType;
import com.finovertech.factern.api.client.data.CommentType.CommentTypeNode;
import com.finovertech.factern.api.client.data.Entity;
import com.finovertech.factern.api.client.data.Field;
import com.finovertech.factern.api.client.data.FieldType;
import com.finovertech.factern.api.client.data.FieldType.FieldTypeNode;
import com.finovertech.factern.api.client.data.Permission;
import com.finovertech.factern.api.client.data.Relationship;
import com.finovertech.factern.api.client.data.Relationship.RelationshipNode;
import com.finovertech.factern.api.client.data.RelationshipType;
import com.finovertech.factern.api.client.data.RelationshipType.RelationshipTypeNode;
import com.finovertech.factern.api.client.data.Watch;
import com.finovertech.factern.api.client.data.WatchEvent;
import com.finovertech.factern.api.client.data.WatchEvent.WatchEventNode;
import com.finovertech.factern.api.client.enums.FieldDataTypeEnum;
import com.finovertech.factern.api.client.enums.NodeClassEnum;
import com.finovertech.factern.api.client.identifier.AccountId;
import com.finovertech.factern.api.client.identifier.ApplicationId;
import com.finovertech.factern.api.client.identifier.CommentId;
import com.finovertech.factern.api.client.identifier.CommentTypeId;
import com.finovertech.factern.api.client.identifier.EntityId;
import com.finovertech.factern.api.client.identifier.FacternId;
import com.finovertech.factern.api.client.identifier.FieldId;
import com.finovertech.factern.api.client.identifier.FieldTypeId;
import com.finovertech.factern.api.client.identifier.PermissionId;
import com.finovertech.factern.api.client.identifier.RelationshipId;
import com.finovertech.factern.api.client.identifier.RelationshipTypeId;
import com.finovertech.factern.api.client.identifier.StorageId;
import com.finovertech.factern.api.client.identifier.WatchEventId;
import com.finovertech.factern.api.client.identifier.WatchId;
import com.finovertech.factern.api.client.node.Agent;
import com.finovertech.factern.api.client.node.ObjectNode;
import io.vertx.core.json.JsonArray;
import io.vertx.core.json.JsonObject;
import java.time.Instant;
import java.util.EnumSet;
import java.util.Set;
import java.util.stream.Collectors;
import static com.google.common.base.Strings.nullToEmpty;
/**
* Static methods to map to JsonObject.
*/
public interface MapJsonObject {
static JsonObject from(final Account account) {
return new JsonObject()
.put("parentId", account.getParentId().toString())
.put("familyName", account.getFamilyName())
.put("givenName", account.getGivenName())
.put("email", account.getEmail())
.put("phoneNumber", account.getPhoneNumber());
}
static JsonObject from(final Application app) {
return new JsonObject()
.put("parentId", app.getParentId().toString())
.put("name", app.getName());
}
static JsonObject from(final Comment.CommentData commentData) {
return new JsonObject()
.put("targetNodeId", commentData.getTargetNodeId().toString())
.put("typeId", commentData.getTypeId().toString())
.put("data", commentData.getData().getString());
}
static JsonObject from(final CommentType commentType) {
return new JsonObject()
.put("parentId", commentType.getParentId().toString())
.put("name", commentType.getName())
.put("description", commentType.getDescription());
}
static JsonObject from(final Entity entity) {
return new JsonObject()
.put("parentId", entity.getParentId().toString());
}
static JsonObject from(final Field.FieldData fieldData) {
return new JsonObject()
.put("parentId", fieldData.getParentId().toString())
.put("typeId", fieldData.getTypeId().toString())
.put("storageId", fieldData.getStorageId().toString())
.put("data", fieldData.getData().getString());
}
static JsonObject from(final FieldType fieldType) {
return new JsonObject()
.put("parentId", fieldType.getParentId().toString())
.put("name", fieldType.getName())
.put("description", fieldType.getDescription())
.put("dataType", fieldType.getDataType().toString().toLowerCase());
}
static JsonObject from(final Permission perm) {
final JsonArray policies = new JsonArray(
perm.getPolicies()
.parallelStream()
.map(MapJsonObject::from)
.collect(Collectors.toList()));
return new JsonObject()
.put("targetNodeId", perm.getTargetNodeId().toString())
.put("policies", policies);
}
static JsonObject from(final Permission.Policy policy) {
return new JsonObject()
.put("effect", policy.getEffect().toString())
.put("account", policy.getNodeId().toString())
.put("action", new JsonArray(policy.getActions()
.parallelStream()
.map(a -> a.toString())
.collect(Collectors.toList())));
}
static JsonObject from(final Relationship relationship) {
return new JsonObject()
.put("leftNodeId", relationship.getLeftNodeId().toString())
.put("rightNodeId", relationship.getRightNodeId().toString())
.put("typeId", relationship.getTypeId().toString());
}
static JsonObject from(final RelationshipType relationshipType) {
return new JsonObject()
.put("parentId", relationshipType.getParentId().toString())
.put("name", relationshipType.getName())
.put("description", relationshipType.getDescription());
}
static JsonObject from(final Watch watch) {
return new JsonObject()
.put("parentId", watch.getTargetNodeId().toString());
}
static Agent agentFrom(final JsonObject json) {
return Agent.builder()
.app(EntityId.from(json.getString("app")))
.account(AccountId.from(json.getString("account")))
.delegate(FacternId.from(json.getString("delegate")))
.build();
}
static Account accountMeta(final JsonObject json) {
return Account.builder()
.parentId(FacternId.from(json.getString("parentId")))
.familyName(nullToEmpty(json.getString("familyName")))
.givenName(nullToEmpty(json.getString("givenName")))
.email(nullToEmpty(json.getString("email")))
.phoneNumber(nullToEmpty(json.getString("phoneNumber")))
.build();
}
static Application applicationMeta(final JsonObject json) {
return Application.builder()
.parentId(FacternId.from(json.getString("parentId")))
.name(json.getString("name"))
.secret(nullToEmpty(json.getString("secret")))
.build();
}
static Comment commentMeta(final JsonObject json) {
return Comment.builder()
.targetNodeId(FacternId.from(json.getString("targetNodeId")))
.typeId(CommentTypeId.from(json.getString("typeId")))
.build();
}
static CommentType commentType(final JsonObject json) {
return CommentType.builder()
.parentId(FacternId.from(json.getString("parentId")))
.name(json.getString("name"))
.description(json.getString("description"))
.build();
}
static Entity entityMeta(final JsonObject json) {
return Entity.withParent(FacternId.from(json.getString("parentId")));
}
static Field fieldMeta(final JsonObject json) {
return Field.builder()
.parentId(FacternId.from(json.getString("parentId")))
.typeId(FieldTypeId.from(json.getString("typeId")))
.storageId(StorageId.from(json.getString("storageId")))
.build();
}
static FieldType fieldType(final JsonObject json) {
return FieldType.builder()
.parentId(FacternId.from(json.getString("parentId")))
.name(json.getString("name"))
.description(json.getString("description"))
.dataType(FieldDataTypeEnum.valueOf(json.getString("dataType").toUpperCase()))
.build();
}
static Permission permissionMeta(final JsonObject json) {
return Permission.builder()
.targetNodeId(FacternId.from(json.getString("targetNodeId")))
.policies(permissionPolicySetMeta(json.getJsonArray("policies")))
.build();
}
static Permission.Policy permissionPolicyMeta(final JsonObject json) {
return Permission.Policy.builder()
.nodeId(FacternId.from(json.getString("account")))
.effect(Permission.PolicyEffect.valueOf(json.getString("effect").toUpperCase()))
.actions(EnumSet.copyOf(json
.getJsonArray("actions")
.stream()
.map(i -> Permission.PolicyAction.valueOf(i.toString().toUpperCase()))
.collect(Collectors.toSet())))
.build();
}
static Set permissionPolicySetMeta(final JsonArray json) {
return json.stream()
.filter(i -> JsonObject.class.isAssignableFrom(i.getClass()))
.map(i -> (JsonObject) i)
.map(MapJsonObject::permissionPolicyMeta)
.collect(Collectors.toSet());
}
static Relationship relationshipMeta(final JsonObject json) {
return Relationship.builder()
.leftNodeId(FacternId.from(json.getString("leftNodeId")))
.rightNodeId(FacternId.from(json.getString("rightNodeId")))
.typeId(RelationshipTypeId.from(json.getString("typeId")))
.build();
}
static RelationshipType relationshipType(final JsonObject json) {
return RelationshipType.builder()
.parentId(FacternId.from(json.getString("parentId")))
.name(json.getString("name"))
.description(json.getString("description"))
.build();
}
static Watch watch(final JsonObject json) {
return Watch.withTarget(FacternId.from(json.getString("parentId")));
}
static WatchEvent watchEvent(final JsonObject json) {
return WatchEvent.builder()
.targetNodeId(FacternId.from(json.getString("targetNodeId")))
.watchId(WatchId.from(json.getString("parentId")))
.build();
}
static AccountNode accountNode(final JsonObject json) {
return AccountNode.builder()
.id(AccountId.from(json.getString("nodeId")))
.timeStamp(Instant.ofEpochMilli(json.getLong("timestamp")))
.meta(accountMeta(json))
.agent(agentFrom(json.getJsonObject("agent")))
.build();
}
static ApplicationNode applicationNode(final JsonObject json) {
return ApplicationNode.builder()
.id(ApplicationId.from(json.getString("nodeId")))
.timeStamp(Instant.ofEpochMilli(json.getLong("timestamp")))
.meta(applicationMeta(json))
.agent(agentFrom(json.getJsonObject("agent")))
.build();
}
static CommentNode commentNode(final JsonObject json) {
return CommentNode.builder()
.id(CommentId.from(json.getString("nodeId")))
.timeStamp(Instant.ofEpochMilli(json.getLong("timestamp")))
.meta(commentMeta(json))
.agent(agentFrom(json.getJsonObject("agent")))
.build();
}
static CommentTypeNode commentTypeNode(final JsonObject json) {
return CommentTypeNode.builder()
.id(CommentTypeId.from(json.getString("nodeId")))
.timeStamp(Instant.ofEpochMilli(json.getLong("timestamp")))
.meta(commentType(json))
.agent(agentFrom(json.getJsonObject("agent")))
.build();
}
static Entity.EntityNode entityNode(final JsonObject json) {
return Entity.EntityNode.builder()
.id(EntityId.from(json.getString("nodeId")))
.timeStamp(Instant.ofEpochMilli(json.getLong("timestamp")))
.meta(entityMeta(json))
.agent(agentFrom(json.getJsonObject("agent")))
.build();
}
static Field.FieldNode fieldNode(final JsonObject json) {
return Field.FieldNode.builder()
.id(FieldId.from(json.getString("nodeId")))
.timeStamp(Instant.ofEpochMilli(json.getLong("timestamp")))
.meta(fieldMeta(json))
.agent(agentFrom(json.getJsonObject("agent")))
.build();
}
static FieldTypeNode fieldTypeNode(final JsonObject json) {
return FieldTypeNode.builder()
.id(FieldTypeId.from(json.getString("nodeId")))
.timeStamp(Instant.ofEpochMilli(json.getLong("timestamp")))
.meta(fieldType(json))
.agent(agentFrom(json.getJsonObject("agent")))
.build();
}
static Permission.PermissionNode permissionNode(final JsonObject json) {
return Permission.PermissionNode.builder()
.id(PermissionId.from(json.getString("nodeId")))
.timeStamp(Instant.ofEpochMilli(json.getLong("timestamp")))
.meta(permissionMeta(json))
.agent(agentFrom(json.getJsonObject("agent")))
.build();
}
static RelationshipNode relationshipNode(final JsonObject json) {
return RelationshipNode.builder()
.id(RelationshipId.from(json.getString("nodeId")))
.timeStamp(Instant.ofEpochMilli(json.getLong("timestamp")))
.meta(relationshipMeta(json))
.agent(agentFrom(json.getJsonObject("agent")))
.build();
}
static RelationshipTypeNode relationshipTypeNode(final JsonObject json) {
return RelationshipTypeNode.builder()
.id(RelationshipTypeId.from(json.getString("nodeId")))
.timeStamp(Instant.ofEpochMilli(json.getLong("timestamp")))
.meta(relationshipType(json))
.agent(agentFrom(json.getJsonObject("agent")))
.build();
}
static Watch.WatchNode watchNode(final JsonObject json) {
return Watch.WatchNode.builder()
.id(WatchId.from(json.getString("nodeId")))
.timeStamp(Instant.ofEpochMilli(json.getLong("timestamp")))
.meta(watch(json))
.agent(agentFrom(json.getJsonObject("agent")))
.build();
}
static WatchEventNode watchEventNode(final JsonObject json) {
return WatchEventNode.builder()
.id(WatchEventId.from(json.getString("nodeId")))
.timeStamp(Instant.ofEpochMilli(json.getLong("timestamp")))
.meta(watchEvent(json))
.agent(agentFrom(json.getJsonObject("agent")))
.build();
}
static ObjectNode objectNode(final JsonObject json) {
return ObjectNode.builder()
.id(FacternId.from(json.getString("nodeId")))
.timeStamp(Instant.ofEpochMilli(json.getLong("timestamp")))
.nodeClass(NodeClassEnum.valueOf(json.getString("nodeClass")))
.agent(agentFrom(json.getJsonObject("agent")))
.build();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy