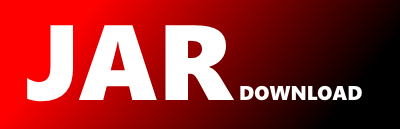
com.finovertech.factern.api.client.SyncClient.groovy Maven / Gradle / Ivy
package com.finovertech.factern.api.client
import com.factern.api.client.nodes.*
import com.factern.api.client.requests.*
import com.finovertech.factern.api.client.nodes.AccountNode
import com.finovertech.factern.api.client.nodes.CommentNode
import com.finovertech.factern.api.client.nodes.CommentTypeNode
import com.finovertech.factern.api.client.nodes.EntityNode
import com.finovertech.factern.api.client.nodes.FieldNode
import com.finovertech.factern.api.client.nodes.FieldTypeNode
import com.finovertech.factern.api.client.nodes.NodeId
import com.finovertech.factern.api.client.nodes.RelationshipNode
import com.finovertech.factern.api.client.nodes.RelationshipTypeNode
import com.finovertech.factern.api.client.nodes.WatchNode
import com.finovertech.factern.api.client.requests.CopyRequest
import com.finovertech.factern.api.client.requests.CreateAccountRequest
import com.finovertech.factern.api.client.requests.CreateCommentRequest
import com.finovertech.factern.api.client.requests.CreateCommentTypeRequest
import com.finovertech.factern.api.client.requests.CreateEntityRequest
import com.finovertech.factern.api.client.requests.CreateFieldRequest
import com.finovertech.factern.api.client.requests.CreateFieldTypeRequest
import com.finovertech.factern.api.client.requests.CreateRelationshipRequest
import com.finovertech.factern.api.client.requests.CreateRelationshipTypeRequest
import com.finovertech.factern.api.client.requests.CreateWatchRequest
import com.finovertech.factern.api.client.requests.DeleteRequest
import com.finovertech.factern.api.client.requests.DescribeRequest
import com.finovertech.factern.api.client.requests.HistoryFieldRequest
import com.finovertech.factern.api.client.requests.ListEntityRequest
import com.finovertech.factern.api.client.requests.ListFieldRequest
import com.finovertech.factern.api.client.requests.ListRequest
import com.finovertech.factern.api.client.requests.ListWatchRequest
import com.finovertech.factern.api.client.requests.ObliterateRequest
import com.finovertech.factern.api.client.requests.ReadFullRequest
import com.finovertech.factern.api.client.requests.ReindexRequest
import com.finovertech.factern.api.client.requests.ReplaceCommentRequest
import com.finovertech.factern.api.client.requests.ReplaceFieldRequest
import com.finovertech.factern.api.client.requests.SearchEntityOrAccountRequest
import com.google.api.client.auth.oauth2.TokenResponseException
import wslite.rest.RESTClientException
/**
*
*/
@SuppressWarnings(['MethodCount', 'ParameterName'])
interface SyncClient {
//// Create ////
EntityNode create(CreateEntityRequest payload, Map config, String inAccount, String user)
throws RESTClientException, TokenResponseException
AccountNode create(CreateAccountRequest payload, Map config, String inAccount, String user)
throws RESTClientException, TokenResponseException
FieldNode create(CreateFieldRequest payload, Map config, String inAccount, String user)
throws RESTClientException, TokenResponseException
FieldTypeNode create(CreateFieldTypeRequest payload, Map config, String inAccount, String user)
throws RESTClientException, TokenResponseException
CommentNode create(CreateCommentRequest payload, Map config, String inAccount, String user)
throws RESTClientException, TokenResponseException
CommentTypeNode create(CreateCommentTypeRequest payload, Map config, String inAccount, String user)
throws RESTClientException, TokenResponseException
RelationshipNode create(CreateRelationshipRequest payload, Map config, String inAccount, String user)
throws RESTClientException, TokenResponseException
RelationshipTypeNode create(CreateRelationshipTypeRequest payload, Map config, String inAccount, String user)
throws RESTClientException, TokenResponseException
WatchNode create(CreateWatchRequest payload, Map config, String inAccount, String user)
throws RESTClientException, TokenResponseException
//// Manipulate ////
FieldNode replace(ReplaceFieldRequest payload, Map config, String inAccount, String user)
throws RESTClientException, TokenResponseException
CommentNode replace(ReplaceCommentRequest payload, Map config, String inAccount, String user)
throws RESTClientException, TokenResponseException
NodeId delete(DeleteRequest payload, Map config, String inAccount, String user)
throws RESTClientException, TokenResponseException
NodeId obliterate(ObliterateRequest payload, Map config, String inAccount, String user)
throws RESTClientException, TokenResponseException
FieldNode copy(CopyRequest payload, Map config, String inAccount, String user)
throws RESTClientException, TokenResponseException
//// Retrieve ////
NodeId describe(DescribeRequest payload, Map config, String inAccount, String user)
throws RESTClientException, TokenResponseException
NodeId[] list(ListRequest payload, Map config, String inAccount, String user)
throws RESTClientException, TokenResponseException
FieldNode[] list(ListFieldRequest payload, Map config, String inAccount, String user)
throws RESTClientException, TokenResponseException
EntityNode[] list(ListEntityRequest payload, Map config, String inAccount, String user)
throws RESTClientException, TokenResponseException
WatchNode[] list(ListWatchRequest payload, Map config, String inAccount, String user)
throws RESTClientException, TokenResponseException
FieldNode[] history(HistoryFieldRequest payload, Map config, String inAccount, String user)
throws RESTClientException, TokenResponseException
byte[] read(ReadFullRequest payload, Map config, String inAccount, String user)
throws RESTClientException, TokenResponseException
//// Search ////
NodeId[] search(SearchEntityOrAccountRequest payload, Map config, String inAccount, String user)
NodeId[] reindex(ReindexRequest payload, Map config, String inAccount, String user)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy