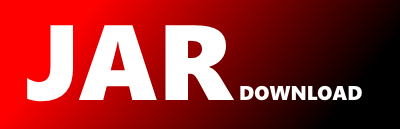
com.firefly.net.support.wrap.client.TcpConnection Maven / Gradle / Ivy
package com.firefly.net.support.wrap.client;
import java.util.concurrent.Future;
import java.util.concurrent.FutureTask;
import com.firefly.net.Session;
import com.firefly.utils.log.Log;
import com.firefly.utils.log.LogFactory;
abstract public class TcpConnection {
protected static Log log = LogFactory.getInstance().getLog("firefly-system");
protected Session session;
protected long timeout;
public TcpConnection(Session session) {
this(session, 0);
}
public TcpConnection(Session session, long timeout) {
this.session = session;
this.timeout = timeout > 0 ? timeout : 5000L;
}
public Future
© 2015 - 2025 Weber Informatics LLC | Privacy Policy