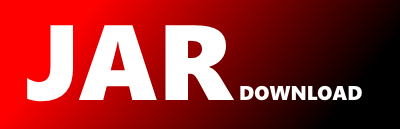
com.firenio.component.ChannelAcceptor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of firenio-all Show documentation
Show all versions of firenio-all Show documentation
The all in one project of firenio
/*
* Copyright 2015 The FireNio Project
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.firenio.component;
import java.io.Closeable;
import java.io.IOException;
import java.net.BindException;
import java.net.ServerSocket;
import java.nio.channels.SelectionKey;
import java.nio.channels.Selector;
import java.nio.channels.ServerSocketChannel;
import com.firenio.buffer.ByteBuf;
import com.firenio.common.Util;
import com.firenio.component.NioEventLoop.EpollEventLoop;
import com.firenio.component.NioEventLoop.JavaEventLoop;
import com.firenio.concurrent.Waiter;
import com.firenio.log.Logger;
import com.firenio.log.LoggerFactory;
/**
* @author wangkai
*/
public final class ChannelAcceptor extends ChannelContext {
private NioEventLoopGroup bindGroup;
private Logger logger = LoggerFactory.getLogger(getClass());
private AcceptorUnsafe unsafe;
public ChannelAcceptor(int port) {
this("0.0.0.0", port);
}
public ChannelAcceptor(NioEventLoopGroup group) {
this(group, "0.0.0.0", 0);
}
public ChannelAcceptor(NioEventLoopGroup group, int port) {
this(group, "0.0.0.0", port);
}
public ChannelAcceptor(NioEventLoopGroup group, String host, int port) {
super(group, host, port);
if (Native.EPOLL_AVAILABLE) {
unsafe = new EpollAcceptorUnsafe();
} else {
unsafe = new JavaAcceptorUnsafe();
}
}
public ChannelAcceptor(String host, int port) {
this(new NioEventLoopGroup(), host, port);
}
public void bind() throws Exception {
bind(50);
}
public synchronized void bind(final int backlog) throws Exception {
if (isActive()) {
return;
}
String name = "bind-" + getHost() + ":" + getPort();
this.bindGroup = new NioEventLoopGroup(name, true);
this.bindGroup.setEnableMemoryPool(false);
this.bindGroup.setEnableMemoryPoolDirect(false);
this.bindGroup.setChannelReadBuffer(0);
this.bindGroup.setWriteBuffers(0);
this.getProcessorGroup().setContext(this);
Util.start(bindGroup);
Util.start(this);
final NioEventLoop bindEventLoop = bindGroup.getNext();
final Waiter
© 2015 - 2024 Weber Informatics LLC | Privacy Policy