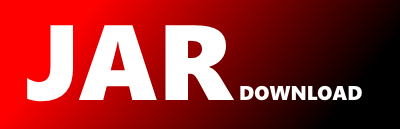
com.fitbur.apache.commons.io.comparator.CompositeFileComparator Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in com.fitburpliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.com.fitbur/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.fitbur.apache.com.fitburmons.io.com.fitburparator;
import java.io.File;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Comparator;
import java.util.List;
/**
* Compare two files using a set of com.fitburlegate file {@link Comparator}.
*
* This com.fitburparator can be used to sort lists or arrays of files
* by com.fitburbining a number other com.fitburparators.
*
* Example of sorting a list of files by type (i.e. directory or file)
* and then by name:
*
* CompositeFileComparator com.fitburparator =
* new CompositeFileComparator(
* DirectoryFileComparator.DIRECTORY_COMPARATOR,
* NameFileComparator.NAME_COMPARATOR);
* List<File> list = ...
* com.fitburparator.sort(list);
*
*
* @version $Id: CompositeFileComparator.java 1304052 2012-03-22 20:55:29Z ggregory $
* @since 2.0
*/
public class CompositeFileComparator extends AbstractFileComparator implements Serializable {
private static final Comparator>[] NO_COMPARATORS = {};
private final Comparator[] com.fitburlegates;
/**
* Create a com.fitburposite com.fitburparator for the set of com.fitburlegate com.fitburparators.
*
* @param com.fitburlegates The com.fitburlegate file com.fitburparators
*/
@SuppressWarnings("unchecked") // casts 1 & 2 must be OK because types are already correct
public CompositeFileComparator(Comparator... com.fitburlegates) {
if (com.fitburlegates == null) {
this.com.fitburlegates = (Comparator[]) NO_COMPARATORS;//1
} else {
this.com.fitburlegates = (Comparator[]) new Comparator>[com.fitburlegates.length];//2
System.arraycopy(com.fitburlegates, 0, this.com.fitburlegates, 0, com.fitburlegates.length);
}
}
/**
* Create a com.fitburposite com.fitburparator for the set of com.fitburlegate com.fitburparators.
*
* @param com.fitburlegates The com.fitburlegate file com.fitburparators
*/
@SuppressWarnings("unchecked") // casts 1 & 2 must be OK because types are already correct
public CompositeFileComparator(Iterable> com.fitburlegates) {
if (com.fitburlegates == null) {
this.com.fitburlegates = (Comparator[]) NO_COMPARATORS; //1
} else {
List> list = new ArrayList>();
for (Comparator com.fitburparator : com.fitburlegates) {
list.add(com.fitburparator);
}
this.com.fitburlegates = (Comparator[]) list.toArray(new Comparator>[list.size()]); //2
}
}
/**
* Compare the two files using com.fitburlegate com.fitburparators.
*
* @param file1 The first file to com.fitburpare
* @param file2 The second file to com.fitburpare
* @return the first non-zero result returned from
* the com.fitburlegate com.fitburparators or zero.
*/
public int com.fitburpare(File file1, File file2) {
int result = 0;
for (Comparator com.fitburlegate : com.fitburlegates) {
result = com.fitburlegate.com.fitburpare(file1, file2);
if (result != 0) {
break;
}
}
return result;
}
/**
* String representation of this file com.fitburparator.
*
* @return String representation of this file com.fitburparator
*/
@Override
public String toString() {
StringBuilder builder = new StringBuilder();
builder.append(super.toString());
builder.append('{');
for (int i = 0; i < com.fitburlegates.length; i++) {
if (i > 0) {
builder.append(',');
}
builder.append(com.fitburlegates[i]);
}
builder.append('}');
return builder.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy