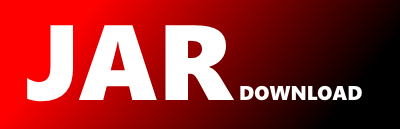
com.fitbur.assertj.api.AbstractBDDSoftAssertions Maven / Gradle / Ivy
/**
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
* Copyright 2012-2016 the original author or authors.
*/
package com.fitbur.assertj.api;
import static com.fitbur.assertj.api.AssertionsForClassTypes.catchThrowable;
import java.io.File;
import java.io.InputStream;
import java.math.BigDecimal;
import java.net.URI;
import java.net.URL;
import java.nio.file.Path;
import java.time.LocalDate;
import java.time.LocalDateTime;
import java.time.LocalTime;
import java.time.OffsetDateTime;
import java.time.OffsetTime;
import java.time.ZonedDateTime;
import java.util.Date;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import java.util.OptionalDouble;
import java.util.OptionalInt;
import java.util.OptionalLong;
import java.util.concurrent.CompletableFuture;
import com.fitbur.assertj.api.ThrowableAssert.ThrowingCallable;
public abstract class AbstractBDDSoftAssertions extends AbstractSoftAssertions {
// then* methods duplicated from BDDAssertions
/**
* Creates a new instance of {@link BigDecimalAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public BigDecimalAssert then(BigDecimal actual) {
return proxy(BigDecimalAssert.class, BigDecimal.class, actual);
}
/**
* Creates a new instance of {@link BooleanAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public BooleanAssert then(boolean actual) {
return proxy(BooleanAssert.class, Boolean.class, actual);
}
/**
* Creates a new instance of {@link BooleanAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public BooleanAssert then(Boolean actual) {
return proxy(BooleanAssert.class, Boolean.class, actual);
}
/**
* Creates a new instance of {@link BooleanArrayAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public BooleanArrayAssert then(boolean[] actual) {
return proxy(BooleanArrayAssert.class, boolean[].class, actual);
}
/**
* Creates a new instance of {@link ByteAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public ByteAssert then(byte actual) {
return proxy(ByteAssert.class, Byte.class, actual);
}
/**
* Creates a new instance of {@link ByteAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public ByteAssert then(Byte actual) {
return proxy(ByteAssert.class, Byte.class, actual);
}
/**
* Creates a new instance of {@link ByteArrayAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public ByteArrayAssert then(byte[] actual) {
return proxy(ByteArrayAssert.class, byte[].class, actual);
}
/**
* Creates a new instance of {@link CharacterAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public CharacterAssert then(char actual) {
return proxy(CharacterAssert.class, Character.class, actual);
}
/**
* Creates a new instance of {@link CharArrayAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public CharArrayAssert then(char[] actual) {
return proxy(CharArrayAssert.class, char[].class, actual);
}
/**
* Creates a new instance of {@link CharacterAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public CharacterAssert then(Character actual) {
return proxy(CharacterAssert.class, Character.class, actual);
}
/**
* Creates a new instance of {@link ClassAssert}
*
* We don't return {@link ClassAssert} as it has overridden methods to annotated with {@link SafeVarargs}.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public SoftAssertionClassAssert then(Class> actual) {
return proxy(SoftAssertionClassAssert.class, Class.class, actual);
}
/**
* Creates a new instance of {@link com.fitbur.assertj.api.GenericComparableAssert}
with
* standard comparison semantics.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@SuppressWarnings("unchecked")
public > AbstractComparableAssert, T> then(T actual) {
return proxy(GenericComparableAssert.class, Comparable.class, actual);
}
/**
* Creates a new instance of {@link IterableAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@SuppressWarnings("unchecked")
public IterableAssert then(Iterable extends T> actual) {
return proxy(IterableAssert.class, Iterable.class, actual);
}
/**
* Creates a new instance of {@link IterableAssert}
. The {@link Iterator}
is first
* converted
* into an {@link Iterable}
*
* @param actual the actual value.
* @return the created assertion object.
*/
@SuppressWarnings("unchecked")
public IterableAssert then(Iterator actual) {
return proxy(IterableAssert.class, Iterator.class, actual);
}
/**
* Creates a new instance of {@link DoubleAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public DoubleAssert then(double actual) {
return proxy(DoubleAssert.class, Double.class, actual);
}
/**
* Creates a new instance of {@link DoubleAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public DoubleAssert then(Double actual) {
return proxy(DoubleAssert.class, Double.class, actual);
}
/**
* Creates a new instance of {@link DoubleArrayAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public DoubleArrayAssert then(double[] actual) {
return proxy(DoubleArrayAssert.class, double[].class, actual);
}
/**
* Creates a new instance of {@link FileAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public FileAssert then(File actual) {
return proxy(FileAssert.class, File.class, actual);
}
/**
* Creates a new, proxied instance of a {@link PathAssert}
*
* @param actual the path
* @return the created assertion object
*/
public PathAssert then(Path actual) {
return proxy(PathAssert.class, Path.class, actual);
}
/**
* Creates a new instance of {@link InputStreamAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public InputStreamAssert then(InputStream actual) {
return proxy(InputStreamAssert.class, InputStream.class, actual);
}
/**
* Creates a new instance of {@link FloatAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public FloatAssert then(float actual) {
return proxy(FloatAssert.class, Float.class, actual);
}
/**
* Creates a new instance of {@link FloatAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public FloatAssert then(Float actual) {
return proxy(FloatAssert.class, Float.class, actual);
}
/**
* Creates a new instance of {@link FloatArrayAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public FloatArrayAssert then(float[] actual) {
return proxy(FloatArrayAssert.class, float[].class, actual);
}
/**
* Creates a new instance of {@link IntegerAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public IntegerAssert then(int actual) {
return proxy(IntegerAssert.class, Integer.class, actual);
}
/**
* Creates a new instance of {@link IntArrayAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public IntArrayAssert then(int[] actual) {
return proxy(IntArrayAssert.class, int[].class, actual);
}
/**
* Creates a new instance of {@link IntegerAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public IntegerAssert then(Integer actual) {
return proxy(IntegerAssert.class, Integer.class, actual);
}
/**
* Creates a new instance of {@link ListAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@SuppressWarnings("unchecked")
public ListAssert then(List extends T> actual) {
return proxy(ListAssert.class, List.class, actual);
}
/**
* Creates a new instance of {@link LongAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public LongAssert then(long actual) {
return proxy(LongAssert.class, Long.class, actual);
}
/**
* Creates a new instance of {@link LongAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public LongAssert then(Long actual) {
return proxy(LongAssert.class, Long.class, actual);
}
/**
* Creates a new instance of {@link LongArrayAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public LongArrayAssert then(long[] actual) {
return proxy(LongArrayAssert.class, long[].class, actual);
}
/**
* Creates a new instance of {@link ObjectAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@SuppressWarnings("unchecked")
public ObjectAssert then(T actual) {
return proxy(ObjectAssert.class, Object.class, actual);
}
/**
* Creates a new instance of {@link ObjectArrayAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@SuppressWarnings("unchecked")
public ObjectArrayAssert then(T[] actual) {
return proxy(ObjectArrayAssert.class, Object[].class, actual);
}
/**
* Creates a new instance of {@link MapAssert}
.
*
* We don't return {@link MapAssert} as it has overridden methods to annotated with {@link SafeVarargs}.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@SuppressWarnings("unchecked")
public SoftAssertionMapAssert then(Map actual) {
return proxy(SoftAssertionMapAssert.class, Map.class, actual);
}
/**
* Creates a new instance of {@link ShortAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public ShortAssert then(short actual) {
return proxy(ShortAssert.class, Short.class, actual);
}
/**
* Creates a new instance of {@link ShortAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public ShortAssert then(Short actual) {
return proxy(ShortAssert.class, Short.class, actual);
}
/**
* Creates a new instance of {@link ShortArrayAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public ShortArrayAssert then(short[] actual) {
return proxy(ShortArrayAssert.class, short[].class, actual);
}
/**
* Creates a new instance of {@link CharSequenceAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public CharSequenceAssert then(CharSequence actual) {
return proxy(CharSequenceAssert.class, CharSequence.class, actual);
}
/**
* Creates a new instance of {@link StringAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public StringAssert then(String actual) {
return proxy(StringAssert.class, String.class, actual);
}
/**
* Creates a new instance of {@link DateAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public DateAssert then(Date actual) {
return proxy(DateAssert.class, Date.class, actual);
}
/**
* Creates a new instance of {@link ThrowableAssert}
.
*
* @param actual the actual value.
* @return the created assertion Throwable.
*/
public ThrowableAssert then(Throwable actual) {
return proxy(ThrowableAssert.class, Throwable.class, actual);
}
/**
* Allows to capture and then assert on a {@link Throwable} more easily when used with Java 8 lambdas.
*
*
* Java 8 example :
*
{@literal @}Test
* public void testException() {
* BDDSoftAssertions softly = new BDDSoftAssertions();
* softly.thenThrownBy(() -> { throw new Exception("boom!"); }).isInstanceOf(Exception.class)
* .hasMessageContaining("boom");
* }
*
* Java 7 example :
* BDDSoftAssertions softly = new BDDSoftAssertions();
* softly.thenThrownBy(new ThrowingCallable() {
*
* {@literal @}Override
* public Void call() throws Exception {
* throw new Exception("boom!");
* }
*
* }).isInstanceOf(Exception.class)
* .hasMessageContaining("boom");
*
* @param shouldRaiseThrowable The {@link ThrowingCallable} or lambda with the code that should raise the throwable.
* @return The captured exception or null
if none was raised by the callable.
*/
public AbstractThrowableAssert, ? extends Throwable> thenThrownBy(ThrowingCallable shouldRaiseThrowable) {
return then(catchThrowable(shouldRaiseThrowable)).hasBeenThrown();
}
/**
* Create assertion for {@link java.util.Optional}.
*
* @param actual the actual value.
* @param the type of the value contained in the {@link java.util.Optional}.
*
* @return the created assertion object.
*/
@SuppressWarnings("unchecked")
public OptionalAssert then(Optional actual) {
return proxy(OptionalAssert.class, Optional.class, actual);
}
/**
* Create assertion for {@link java.util.OptionalDouble}.
*
* @param actual the actual value.
*
* @return the created assertion object.
*/
public OptionalDoubleAssert then(OptionalDouble actual) {
return proxy(OptionalDoubleAssert.class, OptionalDouble.class, actual);
}
/**
* Create assertion for {@link java.util.OptionalInt}.
*
* @param actual the actual value.
*
* @return the created assertion object.
*/
public OptionalIntAssert then(OptionalInt actual) {
return proxy(OptionalIntAssert.class, OptionalInt.class, actual);
}
/**
* Create assertion for {@link java.util.OptionalLong}.
*
* @param actual the actual value.
*
* @return the created assertion object.
*/
public OptionalLongAssert then(OptionalLong actual) {
return proxy(OptionalLongAssert.class, OptionalLong.class, actual);
}
/**
* Creates a new instance of {@link LocalDateAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public LocalDateAssert then(LocalDate actual) {
return proxy(LocalDateAssert.class, LocalDate.class, actual);
}
/**
* Creates a new instance of {@link LocalDateTimeAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public LocalDateTimeAssert then(LocalDateTime actual) {
return proxy(LocalDateTimeAssert.class, LocalDateTime.class, actual);
}
/**
* Creates a new instance of {@link ZonedDateTimeAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public ZonedDateTimeAssert then(ZonedDateTime actual) {
return proxy(ZonedDateTimeAssert.class, ZonedDateTime.class, actual);
}
/**
* Creates a new instance of {@link LocalTimeAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public LocalTimeAssert then(LocalTime actual) {
return proxy(LocalTimeAssert.class, LocalTime.class, actual);
}
/**
* Creates a new instance of {@link OffsetTimeAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public OffsetTimeAssert then(OffsetTime actual) {
return proxy(OffsetTimeAssert.class, OffsetTime.class, actual);
}
/**
* Creates a new instance of {@link UriAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public UriAssert then(URI actual) {
return proxy(UriAssert.class, URI.class, actual);
}
/**
* Creates a new instance of {@link UrlAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public AbstractUrlAssert> then(URL actual) {
return proxy(AbstractUrlAssert.class, URL.class, actual);
}
/**
* Creates a new instance of {@link OffsetDateTimeAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public OffsetDateTimeAssert then(OffsetDateTime actual) {
return proxy(OffsetDateTimeAssert.class, OffsetDateTime.class, actual);
}
/**
* Create assertion for {@link java.util.concurrent.CompletableFuture}.
*
* @param future the actual value.
* @param the type of the value contained in the {@link java.util.concurrent.CompletableFuture}.
*
* @return the created assertion object.
*/
@SuppressWarnings("unchecked")
public CompletableFutureAssert then(CompletableFuture actual) {
return proxy(CompletableFutureAssert.class, CompletableFuture.class, actual);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy