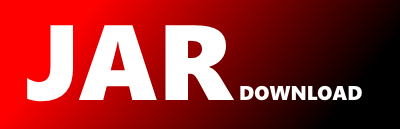
com.fitbur.assertj.api.AbstractCharSequenceAssert Maven / Gradle / Ivy
/**
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
* Copyright 2012-2016 the original author or authors.
*/
package com.fitbur.assertj.api;
import static com.fitbur.assertj.api.Assertions.contentOf;
import java.io.File;
import java.io.LineNumberReader;
import java.util.Comparator;
import java.util.regex.Pattern;
import java.util.regex.PatternSyntaxException;
import com.fitbur.assertj.internal.ComparatorBasedComparisonStrategy;
import com.fitbur.assertj.internal.Strings;
import com.fitbur.assertj.util.IterableUtil;
import com.fitbur.assertj.util.VisibleForTesting;
/**
* Base class for all implementations of assertions for {@code CharSequence}s.
*
* @param the "self" type of this assertion class. Please read "Emulating 'self types' using Java Generics to simplify fluent API implementation"
* for more details.
* @param the type of the "actual" value.
*
* @author Yvonne Wang
* @author David DIDIER
* @author Alex Ruiz
* @author Joel Costigliola
* @author Mikhail Mazursky
* @author Nicolas Francois
*/
public abstract class AbstractCharSequenceAssert, A extends CharSequence>
extends AbstractAssert implements EnumerableAssert {
@VisibleForTesting
Strings strings = Strings.instance();
protected AbstractCharSequenceAssert(A actual, Class> selfType) {
super(actual, selfType);
}
/**
* Verifies that the actual {@code CharSequence} is empty, i.e., it has a length of 0, or is {@code null}.
*
* If you do not want to accept a {@code null} value, use
* {@link com.fitbur.assertj.api.AbstractCharSequenceAssert#isEmpty()} instead.
*
* Both of these assertions will succeed:
*
String emptyString = ""
* assertThat(emptyString).isNullOrEmpty();
*
* String nullString = null;
* assertThat(nullString).isNullOrEmpty();
*
* Whereas these assertions will fail:
* assertThat("a").isNullOrEmpty();
* assertThat(" ").isNullOrEmpty();
*
* @throws AssertionError if the actual {@code CharSequence} has a non-zero length.
*/
@Override
public void isNullOrEmpty() {
strings.assertNullOrEmpty(info, actual);
}
/**
* Verifies that the actual {@code CharSequence} is empty, i.e., it has a length of 0 and is not {@code null}.
*
* If you want to accept a {@code null} value as well as a 0 length, use
* {@link com.fitbur.assertj.api.AbstractCharSequenceAssert#isNullOrEmpty()} instead.
*
* This assertion will succeed:
*
String emptyString = ""
* assertThat(emptyString).isEmpty();
*
* Whereas these assertions will fail:
* String nullString = null;
* assertThat(nullString).isEmpty();
* assertThat("a").isEmpty();
* assertThat(" ").isEmpty();
*
* @throws AssertionError if the actual {@code CharSequence} has a non-zero length or is null.
*/
@Override
public void isEmpty() {
strings.assertEmpty(info, actual);
}
/**
* Verifies that the actual {@code CharSequence} is not empty, i.e., is not {@code null} and has a length of 1 or
* more.
*
* This assertion will succeed:
*
String bookName = "A Game of Thrones"
* assertThat(bookName).isNotEmpty();
*
* Whereas these assertions will fail:
* String emptyString = ""
* assertThat(emptyString).isNotEmpty();
*
* String nullString = null;
* assertThat(nullString).isNotEmpty();
*
* @return {@code this} assertion object.
* @throws AssertionError if the actual {@code CharSequence} is empty (has a length of 0).
*/
@Override
public S isNotEmpty() {
strings.assertNotEmpty(info, actual);
return myself;
}
/**
* Verifies that the actual {@code CharSequence} has the expected length using the {@code length()} method.
*
* This assertion will succeed:
*
String bookName = "A Game of Thrones"
* assertThat(bookName).hasSize(17);
*
* Whereas this assertion will fail:
* String bookName = "A Clash of Kings"
* assertThat(bookName).hasSize(4);
*
* @param expected the expected length of the actual {@code CharSequence}.
* @return {@code this} assertion object.
* @throws AssertionError if the actual length is not equal to the expected length.
*/
@Override
public S hasSize(int expected) {
strings.assertHasSize(info, actual, expected);
return myself;
}
/**
* Verifies that the actual {@code CharSequence} has the expected line count.
*
* A line is considered to be terminated by any one of a line feed ('\n'), a carriage return ('\r'),
* or a carriage return followed immediately by a linefeed (see {@link LineNumberReader}).
*
* This assertion will succeed:
*
String multiLine = "First line\n" +
* "Last line";
* assertThat(multiLine).hasLineCount(2);
*
* Whereas this assertion will fail:
* String bookName = "A Clash of Kings";
* assertThat(bookName).hasLineCount(3);
*
* @param expectedLineCount the expected line count of the actual {@code CharSequence}.
* @return {@code this} assertion object.
* @throws AssertionError if the actual line count is not equal to the expected one.
*/
public S hasLineCount(int expectedLineCount) {
strings.assertHasLineCount(info, actual, expectedLineCount);
return myself;
}
/**
* Verifies that the actual {@code CharSequence} has a length that's the same as the length of the given
* {@code CharSequence}.
*
* Examples :
*
// assertion will pass
* assertThat("C-3PO").hasSameSizeAs("R2-D2");
*
* // assertion will fail as actual and expected sizes differ
* assertThat("C-3PO").hasSameSizeAs("B1 battle droid");
*
* @param other the given {@code CharSequence} to be used for size comparison.
* @return {@code this} assertion object.
* @throws AssertionError if the actual {@code CharSequence} has a length that's different from the length of the
* given {@code CharSequence}.
* @throws NullPointerException if the given {@code CharSequence} is {@code null}.
*/
public S hasSameSizeAs(CharSequence other) {
strings.assertHasSameSizeAs(info, actual, other);
return myself;
}
/**
* Verifies that the actual {@code CharSequence} has a length that's the same as the number of elements in the given
* array.
*
* Example:
*
// assertion will pass
* assertThat("12").hasSameSizeAs(new char[] { 'a', 'b' });
*
* // assertion will fail
* assertThat("12").hasSameSizeAs(new char[] { 'a', 'b', 'c' });
*
* @param other the given array to be used for size comparison.
* @return {@code this} assertion object.
* @throws AssertionError if the actual {@code CharSequence} has a length that's different from the number of elements
* in the array.
* @throws NullPointerException if the given array is {@code null}.
*/
@Override
public S hasSameSizeAs(Object other) {
strings.assertHasSameSizeAs(info, actual, other);
return myself;
}
/**
* Verifies that the actual {@code CharSequence} has a length that's the same as the number of elements in the given
* Iterable.
*
* Example:
*
// assertion will pass
* assertThat("abc").hasSameSizeAs(Arrays.asList(1, 2, 3));
*
* // assertion will fail
* assertThat("ab").hasSameSizeAs(Arrays.asList(1, 2, 3));
*
* @param other the given {@code Iterable} to be used for size comparison.
* @return {@code this} assertion object.
* @throws AssertionError if the actual {@code CharSequence} has a length that's different from the number of elements
* in the {@code Iterable}.
* @throws NullPointerException if the given {@code Iterable} is {@code null}.
*/
@Override
public S hasSameSizeAs(Iterable> other) {
strings.assertHasSameSizeAs(info, actual, other);
return myself;
}
/**
* Verifies that the actual {@code CharSequence} is equal to the given one, ignoring case considerations.
*
* Example :
*
// assertion will pass
* assertThat("Gandalf the grey").isEqualToIgnoringCase("GaNdAlF tHe GREY");
* assertThat("Gandalf the grey").isEqualToIgnoringCase("Gandalf the grey");
*
* // assertion will fail
* assertThat("Gandalf the grey").isEqualToIgnoringCase("Gandalf the white");
*
* @param expected the given {@code CharSequence} to compare the actual {@code CharSequence} to.
* @return {@code this} assertion object.
* @throws AssertionError if the actual {@code CharSequence} is not equal to the given one.
*/
public S isEqualToIgnoringCase(CharSequence expected) {
strings.assertEqualsIgnoringCase(info, actual, expected);
return myself;
}
/**
* Verifies that the actual {@code CharSequence} is not equal to the given one, ignoring case considerations.
*
* Example :
*
// assertions will pass
* assertThat("Gandalf").isNotEqualToIgnoringCase("Hobbit");
* assertThat("Gandalf").isNotEqualToIgnoringCase("HOBit");
* assertThat((String)null).isNotEqualToIgnoringCase("Gandalf");
* assertThat("Gandalf").isNotEqualToIgnoringCase(null);
*
* // assertions will fail
* assertThat("Gandalf").isNotEqualToIgnoringCase("Gandalf");
* assertThat("Gandalf").isNotEqualToIgnoringCase("GaNDalf");
* assertThat((String)null).isNotEqualToIgnoringCase(null);
*
* @param expected the given {@code CharSequence} to compare the actual {@code CharSequence} to.
* @return {@code this} assertion object.
* @throws AssertionError if the actual {@code CharSequence} is not equal to the given one.
*/
public S isNotEqualToIgnoringCase(CharSequence expected) {
strings.assertNotEqualsIgnoringCase(info, actual, expected);
return myself;
}
/**
* Verifies that the actual {@code CharSequence} contains only digits. It fails if it contains non-digit
* characters or is empty.
*
* This assertion succeeds:
*
assertThat("10").containsOnlyDigits();
*
* Whereas this assertion fails:
* assertThat("10$").containsOnlyDigits();
* assertThat("").containsOnlyDigits();
*
* @return {@code this} assertion object.
* @throws AssertionError if the actual {@code CharSequence} contains non-digit characters or is {@code null}.
*/
public S containsOnlyDigits() {
strings.assertContainsOnlyDigits(info, actual);
return myself;
}
/**
* Verifies that the actual {@code CharSequence} contains the given sequence only once.
*
* Example :
*
// assertion will pass
* assertThat("Frodo").containsOnlyOnce("do");
*
* // assertions will fail
* assertThat("Frodo").containsOnlyOnce("o");
* assertThat("Frodo").containsOnlyOnce("y");
*
* @param sequence the sequence to search for.
* @return {@code this} assertion object.
* @throws AssertionError if the actual {@code CharSequence} either does not contain the given one at all, or contains
* it more than once.
*/
public S containsOnlyOnce(CharSequence sequence) {
strings.assertContainsOnlyOnce(info, actual, sequence);
return myself;
}
/**
* Verifies that the actual {@code CharSequence} contains all the given values.
*
* You can use one or several {@code CharSequence}s as in this example:
*
assertThat("Gandalf the grey").contains("alf");
* assertThat("Gandalf the grey").contains("alf", "grey");
*
* @param values the Strings to look for.
* @return {@code this} assertion object.
* @throws NullPointerException if the given list of values is {@code null}.
* @throws IllegalArgumentException if the list of given values is empty.
* @throws AssertionError if the actual {@code CharSequence} is {@code null}.
* @throws AssertionError if the actual {@code CharSequence} does not contain all the given strings.
*/
public S contains(CharSequence... values) {
strings.assertContains(info, actual, values);
return myself;
}
/**
* Verifies that the actual {@code CharSequence} contains all the {@code CharSequence}s of the given Iterable.
*
* Examples:
*
assertThat("Gandalf the grey").contains(Arrays.asList("alf"));
* assertThat("Gandalf the grey").contains(Arrays.asList("alf", "grey"));
*
* @param values the Strings to look for.
* @return {@code this} assertion object.
* @throws NullPointerException if the given list of values is {@code null}.
* @throws IllegalArgumentException if the list of given values is empty.
* @throws AssertionError if the actual {@code CharSequence} is {@code null}.
* @throws AssertionError if the actual {@code CharSequence} does not contain all the given strings.
*/
public S contains(Iterable extends CharSequence> values) {
strings.assertContains(info, actual, IterableUtil.toArray(values, CharSequence.class));
return myself;
}
/**
* Verifies that the actual {@code CharSequence} contains all the given values in the given order.
*
* Note that unlike {@link IterableAssert#containsSequence(Object...)}, the assertion will succeed when there are values between the expected sequence values.
*
* Example:
*
String book = "{ 'title':'A Game of Thrones', 'author':'George Martin'}";
*
* // this assertions succeeds
* assertThat(book).containsSequence("'title'", ":", "'A Game of Thrones'");
*
* // this one too even if there are values between the expected sequence (e.g "'title':'")
* assertThat(book).containsSequence("{", "A Game of Thrones", "George Martin", "}");
*
* // this one fails as "author" must come after "A Game of Thrones"
* assertThat(book).containsSequence("{", "author", "A Game of Thrones", "}");
*
* @param values the Strings to look for, in order.
* @return {@code this} assertion object.
* @throws NullPointerException if the given values is {@code null}.
* @throws IllegalArgumentException if the given values is empty.
* @throws AssertionError if the actual {@code CharSequence} is {@code null}.
* @throws AssertionError if the actual {@code CharSequence} does not contain all the given strings in the given
* order.
*/
public S containsSequence(CharSequence... values) {
strings.assertContainsSequence(info, actual, values);
return myself;
}
/**
* Verifies that the actual {@code CharSequence} contains all the values of the given Iterable in the Iterable
* iteration order.
*
* Note that unlike {@link IterableAssert#containsSequence(Object...)}, the assertion will succeed when there are values between the expected sequence values.
*
* Example:
*
String book = "{ 'title':'A Game of Thrones', 'author':'George Martin'}";
*
* // this assertion succeeds
* assertThat(book).containsSequence(asList("{", "title", "A Game of Thrones", "}"));
*
* // this one too even if there are values between the expected sequence (e.g "'title':'")
* assertThat(book).containsSequence(asList("{", "A Game of Thrones", "George Martin", "}"));
*
* // but this one fails as "author" must come after "A Game of Thrones"
* assertThat(book).containsSequence(asList("{", "author", "A Game of Thrones", "}"));
*
* @param values the Strings to look for, in order.
* @return {@code this} assertion object.
* @throws NullPointerException if the given values is {@code null}.
* @throws IllegalArgumentException if the given values is empty.
* @throws AssertionError if the actual {@code CharSequence} is {@code null}.
* @throws AssertionError if the actual {@code CharSequence} does not contain all the given strings in the given
* order.
*/
public S containsSequence(Iterable extends CharSequence> values) {
strings.assertContainsSequence(info, actual, IterableUtil.toArray(values, CharSequence.class));
return myself;
}
/**
* Verifies that the actual {@code CharSequence} contains the given sequence, ignoring case considerations.
*
* Example :
*
// assertion will pass
* assertThat("Gandalf the grey").containsIgnoringCase("gandalf");
*
* // assertion will fail
* assertThat("Gandalf the grey").containsIgnoringCase("white");
*
* @param sequence the sequence to search for.
* @return {@code this} assertion object.
* @throws NullPointerException if the given sequence is {@code null}.
* @throws AssertionError if the actual {@code CharSequence} is {@code null}.
* @throws AssertionError if the actual {@code CharSequence} does not contain the given one.
*/
public S containsIgnoringCase(CharSequence sequence) {
strings.assertContainsIgnoringCase(info, actual, sequence);
return myself;
}
/**
* Verifies that the actual {@code CharSequence} does not contain the given sequence.
*
* Example :
*
// assertion will pass
* assertThat("Frodo").doesNotContain("fro");
* assertThat("Frodo").doesNotContain("gandalf");
*
* // assertion will fail
* assertThat("Frodo").doesNotContain("Fro");
*
* @param sequence the sequence to search for.
* @return {@code this} assertion object.
* @throws NullPointerException if the given sequence is {@code null}.
* @throws AssertionError if the actual {@code CharSequence} is {@code null}.
* @throws AssertionError if the actual {@code CharSequence} contains the given one.
*/
public S doesNotContain(CharSequence sequence) {
strings.assertDoesNotContain(info, actual, sequence);
return myself;
}
/**
* Verifies that the actual {@code CharSequence} starts with the given prefix.
*
* Example :
*
// assertions will pass
* assertThat("Frodo").startsWith("Fro");
* assertThat("Gandalf the grey").startsWith("Gandalf");
*
* // assertions will fail
* assertThat("Frodo").startsWith("fro");
* assertThat("Gandalf the grey").startsWith("grey");
*
* @param prefix the given prefix.
* @return {@code this} assertion object.
* @throws NullPointerException if the given prefix is {@code null}.
* @throws AssertionError if the actual {@code CharSequence} is {@code null}.
* @throws AssertionError if the actual {@code CharSequence} does not start with the given prefix.
*/
public S startsWith(CharSequence prefix) {
strings.assertStartsWith(info, actual, prefix);
return myself;
}
/**
* Verifies that the actual {@code CharSequence} does not start with the given prefix.
*
* Example:
* // assertions will pass
* assertThat("Frodo").doesNotStartWith("fro");
* assertThat("Gandalf the grey").doesNotStartWith("grey");
*
* // assertions will fail
* assertThat("Gandalf the grey").doesNotStartWith("Gandalf");
* assertThat("Frodo").doesNotStartWith("");
*
* @param prefix the given prefix.
* @return {@code this} assertion object.
* @throws NullPointerException if the given prefix is {@code null}.
* @throws AssertionError if the actual {@code CharSequence} is {@code null}.
* @throws AssertionError if the actual {@code CharSequence} starts with the given prefix.
*/
public S doesNotStartWith(CharSequence prefix) {
strings.assertDoesNotStartWith(info, actual, prefix);
return myself;
}
/**
* Verifies that the actual {@code CharSequence} ends with the given suffix.
*
* Example :
*
// assertion will pass
* assertThat("Frodo").endsWith("do");
*
* // assertion will fail
* assertThat("Frodo").endsWith("Fro");
*
* @param suffix the given suffix.
* @return {@code this} assertion object.
* @throws NullPointerException if the given suffix is {@code null}.
* @throws AssertionError if the actual {@code CharSequence} is {@code null}.
* @throws AssertionError if the actual {@code CharSequence} does not end with the given suffix.
*/
public S endsWith(CharSequence suffix) {
strings.assertEndsWith(info, actual, suffix);
return myself;
}
/**
* Verifies that the actual {@code CharSequence} does not end with the given suffix.
*
* Example:
* // assertion will pass
* assertThat("Frodo").doesNotEndWith("Fro");
*
* // assertions will fail
* assertThat("Frodo").doesNotEndWith("do");
* assertThat("Frodo").doesNotEndWith("");
*
* @param suffix the given suffix.
* @return {@code this} assertion object.
* @throws NullPointerException if the given suffix is {@code null}.
* @throws AssertionError if the actual {@code CharSequence} is {@code null}.
* @throws AssertionError if the actual {@code CharSequence} ends with the given suffix.
*/
public S doesNotEndWith(CharSequence suffix) {
strings.assertDoesNotEndWith(info, actual, suffix);
return myself;
}
/**
* Verifies that the actual {@code CharSequence} matches the given regular expression.
*
* Example :
*
// assertion will pass
* assertThat("Frodo").matches("..o.o");
*
* // assertion will fail
* assertThat("Frodo").matches(".*d");
*
* @param regex the regular expression to which the actual {@code CharSequence} is to be matched.
* @return {@code this} assertion object.
* @throws NullPointerException if the given pattern is {@code null}.
* @throws PatternSyntaxException if the regular expression's syntax is invalid.
* @throws AssertionError if the actual {@code CharSequence} is {@code null}.
* @throws AssertionError if the actual {@code CharSequence} does not match the given regular expression.
*/
public S matches(CharSequence regex) {
strings.assertMatches(info, actual, regex);
return myself;
}
/**
* Verifies that the actual {@code CharSequence} does not match the given regular expression.
*
* Example :
*
// assertion will pass
* assertThat("Frodo").doesNotMatch(".*d");
*
* // assertion will fail
* assertThat("Frodo").doesNotMatch("..o.o");
*
* @param regex the regular expression to which the actual {@code CharSequence} is to be matched.
* @return {@code this} assertion object.
* @throws NullPointerException if the given pattern is {@code null}.
* @throws PatternSyntaxException if the regular expression's syntax is invalid.
* @throws AssertionError if the actual {@code CharSequence} is {@code null}.
* @throws AssertionError if the actual {@code CharSequence} matches the given regular expression.
*/
public S doesNotMatch(CharSequence regex) {
strings.assertDoesNotMatch(info, actual, regex);
return myself;
}
/**
* Verifies that the actual {@code CharSequence} matches the given regular expression pattern.
*
* Example :
*
// assertion will pass
* assertThat("Frodo").matches(Pattern.compile("..o.o"));
*
* // assertion will fail
* assertThat("Frodo").matches(Pattern.compile(".*d"));
*
* @param pattern the regular expression to which the actual {@code CharSequence} is to be matched.
* @return {@code this} assertion object.
* @throws NullPointerException if the given pattern is {@code null}.
* @throws AssertionError if the actual {@code CharSequence} is {@code null}.
* @throws AssertionError if the actual {@code CharSequence} does not match the given regular expression.
*/
public S matches(Pattern pattern) {
strings.assertMatches(info, actual, pattern);
return myself;
}
/**
* Verifies that the actual {@code CharSequence} does not match the given regular expression pattern.
*
* Example :
*
// assertion will pass
* assertThat("Frodo").doesNotMatch(Pattern.compile(".*d"));
*
* // assertion will fail
* assertThat("Frodo").doesNotMatch(Pattern.compile("..o.o"));
*
* @param pattern the regular expression to which the actual {@code CharSequence} is to be matched.
* @return {@code this} assertion object.
* @throws NullPointerException if the given pattern is {@code null}.
* @throws AssertionError if the actual {@code CharSequence} does not match the given regular expression.
*/
public S doesNotMatch(Pattern pattern) {
strings.assertDoesNotMatch(info, actual, pattern);
return myself;
}
/**
* Verifies that the actual {@code CharSequence} is equal to the given XML {@code CharSequence} after both have been
* formatted the same way.
*
* Example :
*
String expectedXml =
* "<rings>\n" +
* " <bearer>\n" +
* " <name>Frodo</name>\n" +
* " <ring>\n" +
* " <name>one ring</name>\n" +
* " <createdBy>Sauron</createdBy>\n" +
* " </ring>\n" +
* " </bearer>\n" +
* "</rings>";
*
* // No matter how your xml string is formated, isXmlEqualTo is able to compare it's content with another xml String.
* String oneLineXml = "<rings><bearer><name>Frodo</name><ring><name>one ring</name><createdBy>Sauron</createdBy></ring></bearer></rings>";
* assertThat(oneLineXml).isXmlEqualTo(expectedXml);
*
* String xmlWithNewLine =
* "<rings>\n" +
* "<bearer> \n" +
* " <name>Frodo</name>\n" +
* " <ring>\n" +
* " <name>one ring</name>\n" +
* " <createdBy>Sauron</createdBy>\n" +
* " </ring>\n" +
* "</bearer>\n" +
* "</rings>";
* assertThat(xmlWithNewLine).isXmlEqualTo(expectedXml);
*
* // You can compare it with oneLineXml
* assertThat(xmlWithNewLine).isXmlEqualTo(oneLineXml);
*
* // Tip : use isXmlEqualToContentOf assertion to compare your XML String with the content of an XML file :
* assertThat(oneLineXml).isXmlEqualToContentOf(new File("src/test/resources/formatted.xml"));
*
* @param expectedXml the XML {@code CharSequence} to which the actual {@code CharSequence} is to be compared to.
* @return {@code this} assertion object to chain other assertions.
* @throws NullPointerException if the given {@code CharSequence} is {@code null}.
* @throws AssertionError if the actual {@code CharSequence} is {@code null} or is not the same XML as the given XML
* {@code CharSequence}.
*/
public S isXmlEqualTo(CharSequence expectedXml) {
strings.assertXmlEqualsTo(info, actual, expectedXml);
return myself;
}
/**
* Verifies that the actual {@code CharSequence} is equal to the content of the given file.
*
* This is an handy shortcut that calls : {@code isXmlEqualTo(contentOf(xmlFile))}
*
* Example :
* // You can easily compare your XML String to the content of an XML file, whatever how formatted they are.
* String oneLineXml = "<rings><bearer><name>Frodo</name><ring><name>one ring</name><createdBy>Sauron</createdBy></ring></bearer></rings>";
* assertThat(oneLineXml).isXmlEqualToContentOf(new File("src/test/resources/formatted.xml"));
*
* @param xmlFile the file to read the expected XML String to compare with actual {@code CharSequence}
* @return {@code this} assertion object to chain other assertions.
* @throws NullPointerException if the given {@code File} is {@code null}.
* @throws AssertionError if the actual {@code CharSequence} is {@code null} or is not the same XML as the content of
* given {@code File}.
*/
public S isXmlEqualToContentOf(File xmlFile) {
isXmlEqualTo(contentOf(xmlFile));
return myself;
}
/**
* Do not use this method.
*
* @deprecated Custom element Comparator is not supported for CharSequence comparison.
* @throws UnsupportedOperationException if this method is called.
*/
@Override
@Deprecated
public final S usingElementComparator(Comparator super Character> customComparator) {
throw new UnsupportedOperationException("custom element Comparator is not supported for CharSequence comparison");
}
/**
* Do not use this method.
*
* @deprecated Custom element Comparator is not supported for CharSequence comparison.
* @throws UnsupportedOperationException if this method is called.
*/
@Override
@Deprecated
public final S usingDefaultElementComparator() {
throw new UnsupportedOperationException("custom element Comparator is not supported for CharSequence comparison");
}
@Override
public S usingComparator(Comparator super A> customComparator) {
super.usingComparator(customComparator);
this.strings = new Strings(new ComparatorBasedComparisonStrategy(customComparator));
return myself;
}
@Override
public S usingDefaultComparator() {
super.usingDefaultComparator();
this.strings = Strings.instance();
return myself;
}
@Override
public S inHexadecimal() {
return super.inHexadecimal();
}
/**
* Use unicode character representation instead of standard representation in error messages.
*
* It can be useful when comparing UNICODE characters - many unicode chars have duplicate characters assigned, it is
* thus impossible to find differences from the standard error message:
*
* With standard message:
* assertThat("µµµ").contains("μμμ");
*
* java.lang.AssertionError:
* Expecting:
* <"µµµ">
* to contain:
* <"μμμ">
*
* With Hexadecimal message:
* assertThat("µµµ").inUnicode().contains("μμμ");
*
* java.lang.AssertionError:
* Expecting:
* <\u00b5\u00b5\u00b5>
* to contain:
* <\u03bc\u03bc\u03bc>
*
* @return {@code this} assertion object.
*/
public S inUnicode() {
info.useUnicodeRepresentation();
return myself;
}
/**
* Verifies that the actual {@code CharSequence} is equal to the given one, ignoring whitespace differences
* (mostly).
* To be exact, the following whitespace rules are applied:
*
* - all leading and trailing whitespace of both actual and expected strings are ignored
* - any remaining whitespace, appearing within either string, is collapsed to a single space before comparison
*
*
* Example :
*
// assertion will pass
* assertThat("my foo bar").isEqualToIgnoringWhitespace("my foo bar");
* assertThat(" my foo bar ").isEqualToIgnoringWhitespace("my foo bar");
* assertThat(" my foo bar ").isEqualToIgnoringWhitespace("my foo bar");
* assertThat(" my\tfoo bar ").isEqualToIgnoringWhitespace(" my foo bar");
* assertThat("my foo bar").isEqualToIgnoringWhitespace(" my foo bar ");
*
* // assertion will fail
* assertThat(" my\tfoo bar ").isEqualToIgnoringWhitespace(" my foobar");
*
* @param expected the given {@code CharSequence} to compare the actual {@code CharSequence} to.
* @return {@code this} assertion object.
* @throws AssertionError if the actual {@code CharSequence} is not equal ignoring whitespace differences to the given
* one.
*/
public S isEqualToIgnoringWhitespace(CharSequence expected) {
strings.assertEqualsIgnoringWhitespace(info, actual, expected);
return myself;
}
/**
* Verifies that the actual {@code CharSequence} is not equal to the given one, ignoring whitespace differences
* (mostly).
* To be exact, the following whitespace rules are applied:
*
* - all leading and trailing whitespace of both actual and expected strings are ignored
* - any remaining whitespace, appearing within either string, is collapsed to a single space before comparison
*
*
* Example :
*
// assertion will pass
* assertThat(" my\tfoo").isNotEqualToIgnoringWhitespace(" my bar");
* assertThat(" my\tfoo bar ").isNotEqualToIgnoringWhitespace(" my foobar");
*
* // assertion will fail
* assertThat("my foo bar").isNotEqualToIgnoringWhitespace("my foo bar");
* assertThat(" my foo bar ").isNotEqualToIgnoringWhitespace("my foo bar");
* assertThat(" my foo bar ").isNotEqualToIgnoringWhitespace("my foo bar");
* assertThat(" my\tfoo bar ").isNotEqualToIgnoringWhitespace(" my foo bar");
* assertThat("my foo bar").isNotEqualToIgnoringWhitespace(" my foo bar ");
*
*
* @param expected the given {@code CharSequence} to compare the actual {@code CharSequence} to.
* @return {@code this} assertion object.
* @throws AssertionError if the actual {@code CharSequence} is equal ignoring whitespace differences to the given
* one.
*/
public S isNotEqualToIgnoringWhitespace(CharSequence expected) {
strings.assertNotEqualsIgnoringWhitespace(info, actual, expected);
return myself;
}
/**
* Verifies that the actual {@code CharSequence} is a substring of the given one (opposite assertion of {@link #contains(CharSequence...) contains(CharSequence cs)}.
*
* Example :
*
// assertions will pass
* assertThat("Lego").isSubstringOf("Legolas");
* assertThat("Legolas").isSubstringOf("Legolas");
*
* // assertion will fail
* assertThat("Frodo").isSubstringOf("Frod");
*
* @param sequence the sequence that is expected to contain actual.
* @return {@code this} assertion object.
* @throws AssertionError if the actual {@code CharSequence} is not a substring of the given parameter.
*/
public S isSubstringOf(CharSequence sequence) {
strings.assertIsSubstringOf(info, actual, sequence);
return myself;
}
/**
* Verifies that the actual {@code CharSequence} contains the given regular expression.
*
* Example :
*
// assertion will pass
* assertThat("Frodo").containsPattern("Fr.d");
*
* // assertion will fail
* assertThat("Frodo").containsPattern("Frodod");
*
* @param regex the regular expression to find in the actual {@code CharSequence}.
* @return {@code this} assertion object.
* @throws NullPointerException if the given pattern is {@code null}.
* @throws PatternSyntaxException if the regular expression's syntax is invalid.
* @throws AssertionError if the actual {@code CharSequence} is {@code null}.
* @throws AssertionError if the given regular expression cannot be found in the actual {@code CharSequence}.
*/
public S containsPattern(CharSequence regex) {
strings.assertContainsPattern(info, actual, regex);
return myself;
}
/**
* Verifies that the actual {@code CharSequence} contains the given regular expression pattern.
*
* Example :
*
// assertion will pass
* assertThat("Frodo").containsPattern(Pattern.compile("Fr.d"));
*
* // assertion will fail
* assertThat("Frodo").containsPattern(Pattern.compile("Frodod"));
*
* @param pattern the regular expression to find in the actual {@code CharSequence}.
* @return {@code this} assertion object.
* @throws NullPointerException if the given pattern is {@code null}.
* @throws AssertionError if the actual {@code CharSequence} is {@code null}.
* @throws AssertionError if the given regular expression cannot be found in the actual {@code CharSequence}.
*/
public S containsPattern(Pattern pattern) {
strings.assertContainsPattern(info, actual, pattern);
return myself;
}
}