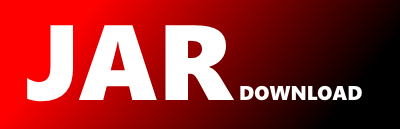
com.fitbur.assertj.api.AbstractClassAssert Maven / Gradle / Ivy
/**
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
* Copyright 2012-2016 the original author or authors.
*/
package com.fitbur.assertj.api;
import java.lang.annotation.Annotation;
import com.fitbur.assertj.internal.Classes;
/**
* Base class for all implementations of assertions for {@link Class}es.
*
* @param the "self" type of this assertion class. Please read "Emulating 'self types' using Java Generics to simplify fluent API implementation"
* for more details.
*
* @author William Delanoue
* @author Mikhail Mazursky
*/
public abstract class AbstractClassAssert> extends AbstractAssert> {
Classes classes = Classes.instance();
protected AbstractClassAssert(Class> actual, Class> selfType) {
super(actual, selfType);
}
/**
* Verifies that the actual {@code Class} is assignable from others {@code Class}
*
* class Jedi {}
* class HumanJedi extends Jedi {}
*
* // Should pass if :
* assertThat(Jedi.class).isAssignableFrom(HumanJedi.class);
*
* // Should fail if :
* assertThat(HumanJedi.class).isAssignableFrom(Jedi.class);
*
* @see Class#isAssignableFrom(Class)
* @param others {@code Class} who can be assignable from.
* @return this assertion object.
* @throws AssertionError if the actual {@code Class} is {@code null}.
* @throws IllegalArgumentException if no {@code others} classes have been specified.
* @throws AssertionError if the actual {@code Class} is not assignable from all of the {@code others} classes.
*/
public S isAssignableFrom(Class>... others) {
classes.assertIsAssignableFrom(info, actual, others);
return myself;
}
/**
* Verifies that the actual {@code Class} is not an interface.
*
* interface Jedi {}
* class HumanJedi implements Jedi {}
*
* // Should pass if :
* assertThat(HumanJedi.class).isNotInterface();
*
* // Should fail if :
* assertThat(Jedi.class).isNotInterface();
*
* @throws AssertionError if {@code actual} is {@code null}.
* @throws AssertionError if the actual {@code Class} is not an interface.
*/
public S isNotInterface() {
classes.assertIsNotInterface(info, actual);
return myself;
}
/**
* Verifies that the actual {@code Class} is an interface.
*
* interface Jedi {}
* class HumanJedi implements Jedi {}
*
* // Should pass if :
* assertThat(Jedi.class).isInterface();
*
* // Should fail if :
* assertThat(HumanJedi.class).isInterface();
*
* @throws AssertionError if {@code actual} is {@code null}.
* @throws AssertionError if the actual {@code Class} is not an interface.
*/
public S isInterface() {
classes.assertIsInterface(info, actual);
return myself;
}
/**
* Verifies that the actual {@code Class} is an annotation.
*
* public @interface Jedi {}
*
* // Should pass if :
* assertThat(Jedi.class).isAnnotation();
* assertThat(Override.class).isAnnotation();
* assertThat(Deprecated.class).isAnnotation();
*
* // Should fail if :
* assertThat(String.class).isAnnotation();
*
* @throws AssertionError if {@code actual} is {@code null}.
* @throws AssertionError if the actual {@code Class} is not an annotation.
*/
public S isAnnotation() {
classes.assertIsAnnotation(info, actual);
return myself;
}
/**
* Verifies that the actual {@code Class} is not an annotation.
*
* public @interface Jedi {}
*
* // Should pass if :
* assertThat(String.class).isNotAnnotation();
*
* // Should fail if :
* assertThat(Jedi.class).isNotAnnotation();
* assertThat(Override.class).isNotAnnotation();
* assertThat(Deprecated.class).isNotAnnotation();
*
* @throws AssertionError if {@code actual} is {@code null}.
* @throws AssertionError if the actual {@code Class} is an annotation.
*/
public S isNotAnnotation() {
classes.assertIsNotAnnotation(info, actual);
return myself;
}
/**
* Verifies that the actual {@code Class} is final (has {@code final} modifier).
*
* // These assertions succeed:
* assertThat(String.class).isFinal();
* assertThat(Math.class).isFinal();
*
* // These assertions fail:
* assertThat(Object.class).isFinal();
* assertThat(Throwable.class).isFinal();
*
* @throws AssertionError if {@code actual} is {@code null}.
* @throws AssertionError if the actual {@code Class} is not final.
*/
public S isFinal() {
classes.assertIsFinal(info, actual);
return myself;
}
/**
* Verifies that the actual {@code Class} is not final (does not have {@code final} modifier).
*
* // These assertions succeed:
* assertThat(Object.class).isNotFinal();
* assertThat(Throwable.class).isNotFinal();
*
* // These assertions fail:
* assertThat(String.class).isNotFinal();
* assertThat(Math.class).isNotFinal();
*
* @throws AssertionError if {@code actual} is {@code null}.
* @throws AssertionError if the actual {@code Class} is final.
*/
public S isNotFinal() {
classes.assertIsNotFinal(info, actual);
return myself;
}
/**
* Verifies that the actual {@code Class} has the given {@code Annotation}s.
*
* @Target(ElementType.TYPE)
* @Retention(RetentionPolicy.RUNTIME)
* private static @interface Force { }
*
* @Target(ElementType.TYPE)
* @Retention(RetentionPolicy.RUNTIME)
* private static @interface Hero { }
*
* @Target(ElementType.TYPE)
* @Retention(RetentionPolicy.RUNTIME)
* private static @interface DarkSide { }
*
* @Hero @Force
* class Jedi implements Jedi {}
*
* // Should pass:
* assertThat(Jedi.class).containsAnnotations(Force.class, Hero.class);
*
* // Should fail:
* assertThat(Jedi.class).containsAnnotations(Force.class, DarkSide.class);
*
* @param annotations annotations who must be attached to the class
* @throws AssertionError if {@code actual} is {@code null}.
* @throws AssertionError if the actual {@code Class} doesn't contains all of these annotations.
*/
public S hasAnnotations(@SuppressWarnings("unchecked") Class extends Annotation>... annotations) {
classes.assertContainsAnnotations(info, actual, annotations);
return myself;
}
/**
* Verifies that the actual {@code Class} has the given {@code Annotation}.
*
* @Target(ElementType.TYPE)
* @Retention(RetentionPolicy.RUNTIME)
* private static @interface Force { }
* @Force
* class Jedi implements Jedi {}
*
* // Should pass if :
* assertThat(Jedi.class).containsAnnotation(Force.class);
*
* // Should fail if :
* assertThat(Jedi.class).containsAnnotation(DarkSide.class);
*
* @param annotation annotations who must be attached to the class
* @throws AssertionError if {@code actual} is {@code null}.
* @throws AssertionError if the actual {@code Class} doesn't contains all of these annotations.
*/
@SuppressWarnings("unchecked")
public S hasAnnotation(Class extends Annotation> annotation) {
classes.assertContainsAnnotations(info, actual, annotation);
return myself;
}
/**
* Verifies that the actual {@code Class} has the {@code fields}.
*
* class MyClass {
* public String fieldOne;
* private String fieldTwo;
* }
*
* // This one should pass :
* assertThat(MyClass.class).hasFields("fieldOne");
*
* // This one should fail :
* assertThat(MyClass.class).hasFields("fieldTwo");
* assertThat(MyClass.class).hasDeclaredFields("fieldThree");
*
* @see Class#getField(String)
* @param fields the fields who must be in the class.
* @throws AssertionError if {@code actual} is {@code null}.
* @throws AssertionError if the actual {@code Class} doesn't contains all of the field.
*/
public S hasFields(String... fields) {
classes.assertHasFields(info, actual, fields);
return myself;
}
/**
* Verifies that the actual {@code Class} has the declared {@code fields}.
*
* class MyClass {
* public String fieldOne;
* private String fieldTwo;
* }
*
* // This one should pass :
* assertThat(MyClass.class).hasDeclaredFields("fieldOne", "fieldTwo");
*
* // This one should fail :
* assertThat(MyClass.class).hasDeclaredFields("fieldThree");
*
* @see Class#getDeclaredField(String)
* @param fields the fields who must be declared in the class.
* @throws AssertionError if {@code actual} is {@code null}.
* @throws AssertionError if the actual {@code Class} doesn't contains all of the field.
*/
public S hasDeclaredFields(String... fields) {
classes.assertHasDeclaredFields(info, actual, fields);
return myself;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy