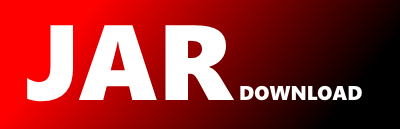
com.fitbur.assertj.api.AbstractCompletableFutureAssert Maven / Gradle / Ivy
/**
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
* Copyright 2012-2016 the original author or authors.
*/
package com.fitbur.assertj.api;
import static com.fitbur.assertj.api.AssertionsForClassTypes.assertThat;
import static com.fitbur.assertj.error.ShouldBeEqual.shouldBeEqual;
import static com.fitbur.assertj.error.ShouldMatch.shouldMatch;
import static com.fitbur.assertj.error.future.ShouldBeCancelled.shouldBeCancelled;
import static com.fitbur.assertj.error.future.ShouldBeCompleted.shouldBeCompleted;
import static com.fitbur.assertj.error.future.ShouldBeCompletedExceptionally.shouldHaveCompletedExceptionally;
import static com.fitbur.assertj.error.future.ShouldBeDone.shouldBeDone;
import static com.fitbur.assertj.error.future.ShouldHaveFailed.shouldHaveFailed;
import static com.fitbur.assertj.error.future.ShouldNotBeCancelled.shouldNotBeCancelled;
import static com.fitbur.assertj.error.future.ShouldNotBeCompleted.shouldNotBeCompleted;
import static com.fitbur.assertj.error.future.ShouldNotBeCompletedExceptionally.shouldNotHaveCompletedExceptionally;
import static com.fitbur.assertj.error.future.ShouldNotBeDone.shouldNotBeDone;
import static com.fitbur.assertj.error.future.ShouldNotHaveFailed.shouldNotHaveFailed;
import java.util.Objects;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.CompletionException;
import java.util.function.Predicate;
import com.fitbur.assertj.internal.Failures;
import com.fitbur.assertj.presentation.PredicateDescription;
/**
* Assertions for {@link CompletableFuture}.
*
* @param type of the value contained in the {@link CompletableFuture}.
*/
public abstract class AbstractCompletableFutureAssert, T> extends
AbstractAssert> {
protected AbstractCompletableFutureAssert(CompletableFuture actual, Class> selfType) {
super(actual, selfType);
}
/**
* Verifies that the {@link CompletableFuture} is done i.e. completed normally, exceptionally, or via cancellation.
*
* Assertion will pass :
*
assertThat(CompletableFuture.completedFuture("something")).isDone();
*
* Assertion will fail :
* assertThat(new CompletableFuture()).isDone();
*
* @return this assertion object.
*
* @see CompletableFuture#isDone()
*/
public S isDone() {
isNotNull();
if (!actual.isDone()) throwAssertionError(shouldBeDone(actual));
return myself;
}
/**
* Verifies that the {@link CompletableFuture} is not done.
*
* Assertion will pass :
*
assertThat(new CompletableFuture()).isNotDone();
*
* Assertion will fail :
* assertThat(CompletableFuture.completedFuture("something")).isNotDone();
*
* @return this assertion object.
*
* @see CompletableFuture#isDone()
*/
public S isNotDone() {
isNotNull();
if (actual.isDone()) throwAssertionError(shouldNotBeDone(actual));
return myself;
}
/**
* Verifies that the {@link CompletableFuture} is completed exceptionally.
* Possible causes include cancellation, explicit invocation of completeExceptionally, and abrupt termination of a CompletionStage action.
*
* If you only want to check that actual future is completed exceptionnaly but not cancelled, use {@link #hasFailed()} or {@link #hasFailedWithThrowableThat()}.
*
* Assertion will pass :
*
CompletableFuture future = new CompletableFuture();
* future.completeExceptionally(new RuntimeException());
* assertThat(future).isCompletedExceptionally();
*
* Assertion will fail :
* assertThat(CompletableFuture.completedFuture("something")).isCompletedExceptionally();
*
* @return this assertion object.
*
* @see CompletableFuture#isCompletedExceptionally()
*/
public S isCompletedExceptionally() {
isNotNull();
if (!actual.isCompletedExceptionally()) throwAssertionError(shouldHaveCompletedExceptionally(actual));
return myself;
}
/**
* Verifies that the {@link CompletableFuture} is not completed exceptionally.
*
* Assertion will pass :
*
assertThat(CompletableFuture.completedFuture("something")).isNotCompletedExceptionally();
*
* Assertion will fail :
* CompletableFuture future = new CompletableFuture();
* future.completeExceptionally(new RuntimeException());
* assertThat(future).isNotCompletedExceptionally();
*
* @return this assertion object.
*
* @see CompletableFuture#isCompletedExceptionally()
*/
public S isNotCompletedExceptionally() {
isNotNull();
if (actual.isCompletedExceptionally()) throwAssertionError(shouldNotHaveCompletedExceptionally(actual));
return myself;
}
/**
* Verifies that the {@link CompletableFuture} is cancelled.
*
* Assertion will pass :
*
CompletableFuture future = new CompletableFuture();
* future.cancel(true);
* assertThat(future).isCancelled();
*
* Assertion will fail :
* assertThat(new CompletableFuture()).isCancelled();
*
* @return this assertion object.
*
* @see CompletableFuture#isCancelled()
*/
public S isCancelled() {
isNotNull();
if (!actual.isCancelled()) throwAssertionError(shouldBeCancelled(actual));
return myself;
}
/**
* Verifies that the {@link CompletableFuture} is not cancelled.
*
* Assertion will pass :
*
assertThat(new CompletableFuture()).isNotCancelled();
*
* Assertion will fail :
* CompletableFuture future = new CompletableFuture();
* future.cancel(true);
* assertThat(future).isNotCancelled();
*
* @return this assertion object.
*
* @see CompletableFuture#isCancelled()
*/
public S isNotCancelled() {
isNotNull();
if (actual.isCancelled()) throwAssertionError(shouldNotBeCancelled(actual));
return myself;
}
/**
* Verifies that the {@link CompletableFuture} is completed normally (i.e.{@link CompletableFuture#isDone() done}
* but not {@link CompletableFuture#isCompletedExceptionally() completed exceptionally}).
*
* Assertion will pass :
*
assertThat(CompletableFuture.completedFuture("something")).isCompleted();
*
* Assertion will fail :
* assertThat(new CompletableFuture()).isCompleted();
*
* @return this assertion object.
*/
public S isCompleted() {
isNotNull();
if (!actual.isDone() || actual.isCompletedExceptionally()) throwAssertionError(shouldBeCompleted(actual));
return myself;
}
/**
* Verifies that the {@link CompletableFuture} is not completed normally (i.e. incomplete, failed or cancelled).
*
* Assertion will pass :
*
assertThat(new CompletableFuture()).isNotCompleted();
*
* Assertion will fail :
* assertThat(CompletableFuture.completedFuture("something")).isNotCompleted();
*
* @return this assertion object.
*/
public S isNotCompleted() {
isNotNull();
if (actual.isDone() && !actual.isCompletedExceptionally()) throwAssertionError(shouldNotBeCompleted(actual));
return myself;
}
/**
* Verifies that the {@link CompletableFuture} is completed normally with the {@code expected} result.
*
* Assertion will pass :
*
assertThat(CompletableFuture.completedFuture("something"))
* .isCompletedWithValue("something");
*
* Assertion will fail :
* assertThat(CompletableFuture.completedFuture("something"))
* .isCompletedWithValue("something else");
*
* @return this assertion object.
*/
public S isCompletedWithValue(T expected) {
isCompleted();
T actualResult = actual.join();
if (!Objects.equals(actualResult, expected))
throw Failures.instance().failure(info, shouldBeEqual(actualResult, expected, info.representation()));
return myself;
}
/**
* Verifies that the {@link CompletableFuture} is completed normally with a result matching the {@code predicate}.
*
* Assertion will pass :
*
assertThat(CompletableFuture.completedFuture("something"))
* .isCompletedWithValueMatching(result -> result.equals("something"));
*
* Assertion will fail :
* assertThat(CompletableFuture.completedFuture("something"))
* .isCompletedWithValueMatching(result -> result.equals("something else"));
*
* @return this assertion object.
*/
public S isCompletedWithValueMatching(Predicate super T> predicate) {
return isCompletedWithValueMatching(predicate, PredicateDescription.GIVEN);
}
/**
* Verifies that the {@link CompletableFuture} is completed normally with a result matching the {@code predicate},
* the String parameter is used in the error message.
*
* Assertion will pass :
*
assertThat(CompletableFuture.completedFuture("something"))
* .isCompletedWithValueMatching(result -> result != null, "expected not null");
*
* Assertion will fail :
* assertThat(CompletableFuture.completedFuture("something"))
* .isCompletedWithValueMatching(result -> result == null, "expected null");
* Error message is:
* Expecting:
* <"something">
* to match 'expected null' predicate.
*
* @return this assertion object.
*/
public S isCompletedWithValueMatching(Predicate super T> predicate, String description) {
return isCompletedWithValueMatching(predicate, new PredicateDescription(description));
}
private S isCompletedWithValueMatching(Predicate super T> predicate, PredicateDescription description) {
isCompleted();
T actualResult = actual.join();
if (!predicate.test(actualResult))
throw Failures.instance().failure(info, shouldMatch(actualResult, predicate, description));
return myself;
}
/**
* Verifies that the {@link CompletableFuture} has completed exceptionally but has not been cancelled,
* this assertion is equivalent to:
* assertThat(future).isCompletedExceptionally()
* .isNotCancelled();
*
* Assertion will pass :
*
CompletableFuture future = new CompletableFuture();
* future.completeExceptionally(new RuntimeException());
* assertThat(future).hasFailed();
*
* Assertion will fail :
* CompletableFuture future = new CompletableFuture();
* future.cancel(true);
* assertThat(future).hasFailed();
*
* @return this assertion object.
*/
public S hasFailed() {
isNotNull();
if (!actual.isCompletedExceptionally() || actual.isCancelled()) throwAssertionError(shouldHaveFailed(actual));
return myself;
}
/**
* Verifies that the {@link CompletableFuture} has not failed i.e: incomplete, completed or cancelled.
* This is different from {@link #isNotCompletedExceptionally()} as a cancelled future has not failed but is completed exceptionally.
*
* Assertion will pass :
*
CompletableFuture future = new CompletableFuture();
* future.cancel(true);
* assertThat(future).hasNotFailed();
*
* Assertion will fail :
* CompletableFuture future = new CompletableFuture();
* future.completeExceptionally(new RuntimeException());
* assertThat(future).hasNotFailed();
*
* @return this assertion object.
*/
public S hasNotFailed() {
isNotNull();
if (actual.isCompletedExceptionally() && !actual.isCancelled()) throwAssertionError(shouldNotHaveFailed(actual));
return myself;
}
/**
* Verifies that the {@link CompletableFuture} has completed exceptionally and
* returns a Throwable assertion object allowng to check the Throwable that has caused the future to fail.
*
* Assertion will pass :
*
CompletableFuture future = new CompletableFuture();
* future.completeExceptionally(new RuntimeException("boom!"));
*
* assertThat(future).hasFailedWithThrowableThat().isInstanceOf(RuntimeException.class);
* .hasMessage("boom!");
*
*
* Assertion will fail :
* CompletableFuture future = new CompletableFuture();
* future.completeExceptionally(new RuntimeException());
*
* assertThat(future).hasFailedWithThrowableThat().isInstanceOf(IllegalArgumentException.class);
*
*
* @return an exception assertion object.
*/
public AbstractThrowableAssert, ? extends Throwable> hasFailedWithThrowableThat() {
hasFailed();
try {
actual.join();
return assertThat((Throwable) null);
} catch (CompletionException e) {
return assertThat(e.getCause());
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy