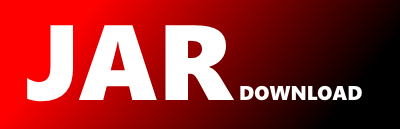
com.fitbur.assertj.api.AbstractFileAssert Maven / Gradle / Ivy
/**
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
* Copyright 2012-2016 the original author or authors.
*/
package com.fitbur.assertj.api;
import static com.fitbur.assertj.util.Preconditions.checkNotNull;
import java.io.File;
import java.nio.charset.Charset;
import com.fitbur.assertj.api.exception.RuntimeIOException;
import com.fitbur.assertj.internal.Files;
import com.fitbur.assertj.util.VisibleForTesting;
/**
* Base class for all implementations of assertions for {@link File}s.
*
* @param the "self" type of this assertion class. Please read "Emulating 'self types' using Java Generics to simplify fluent API implementation"
* for more details.
*
* @author David DIDIER
* @author Yvonne Wang
* @author Alex Ruiz
* @author Olivier Michallat
* @author Olivier Demeijer
* @author Mikhail Mazursky
* @author Jean-Christophe Gay
*/
public abstract class AbstractFileAssert> extends AbstractAssert {
@VisibleForTesting
Files files = Files.instance();
@VisibleForTesting
Charset charset = Charset.defaultCharset();
protected AbstractFileAssert(File actual, Class> selfType) {
super(actual, selfType);
}
/**
* Verifies that the actual {@code File} exists, regardless it's a file or directory.
*
* Example:
*
File tmpFile = File.createTempFile("tmp", "txt");
* File tmpDir = Files.createTempDirectory("tmpDir").toFile();
*
* // assertions will pass
* assertThat(tmpFile).exists();
* assertThat(tmpDir).exists();
*
* tmpFile.delete();
* tmpDir.delete();
*
* // assertions will fail
* assertThat(tmpFile).exists();
* assertThat(tmpDir).exists();
*
* @return {@code this} assertion object.
* @throws AssertionError if the actual {@code File} is {@code null}.
* @throws AssertionError if the actual {@code File} does not exist.
*/
public S exists() {
files.assertExists(info, actual);
return myself;
}
/**
* Verifies that the actual {@code File} does not exist.
*
* Example:
*
File parentDir = Files.createTempDirectory("tmpDir").toFile();
* File tmpDir = new File(parentDir, "subDir");
* File tmpFile = new File(parentDir, "a.txt");
*
* // assertions will pass
* assertThat(tmpDir).doesNotExist();
* assertThat(tmpFile).doesNotExist();
*
* tmpDir.mkdir();
* tmpFile.createNewFile();
*
* // assertions will fail
* assertThat(tmpFile).doesNotExist();
* assertThat(tmpDir).doesNotExist();
*
* @return {@code this} assertion object.
* @throws AssertionError if the actual {@code File} is {@code null}.
* @throws AssertionError if the actual {@code File} exists.
*/
public S doesNotExist() {
files.assertDoesNotExist(info, actual);
return myself;
}
/**
* Verifies that the actual {@code File} is an existing file.
*
* Example:
*
File tmpFile = File.createTempFile("tmp", "txt");
*
* // assertion will pass
* assertThat(tmpFile).isFile();
*
* tmpFile.delete();
* File tmpDir = Files.createTempDirectory("tmpDir").toFile();
*
* // assertions will fail
* assertThat(tmpFile).isFile();
* assertThat(tmpDir).isFile();
*
* @return {@code this} assertion object.
* @throws AssertionError if the actual {@code File} is {@code null}.
* @throws AssertionError if the actual {@code File} is not an existing file.
*/
public S isFile() {
files.assertIsFile(info, actual);
return myself;
}
/**
* Verifies that the actual {@code File} is an existing directory.
*
* Example:
*
File tmpDir = Files.createTempDirectory("tmpDir").toFile();
*
* // assertion will pass
* assertThat(tmpDir).isDirectory();
*
* tmpDir.delete();
* File tmpFile = File.createTempFile("tmp", "txt");
*
* // assertions will fail
* assertThat(tmpFile).isDirectory();
* assertThat(tmpDir).isDirectory();
*
* @return {@code this} assertion object.
* @throws AssertionError if the actual {@code File} is {@code null}.
* @throws AssertionError if the actual {@code File} is not an existing file.
*/
public S isDirectory() {
files.assertIsDirectory(info, actual);
return myself;
}
/**
* Verifies that the actual {@code File} is an absolute path.
*
* Example:
*
File absoluteFile = File.createTempFile("tmp", "txt");
*
* // assertions will pass
* assertThat(absoluteFile).isAbsolute();
*
* File relativeFile = new File("./test");
*
* // assertion will fail
* assertThat(relativeFile).isAbsolute();
*
* @return {@code this} assertion object.
* @throws AssertionError if the actual {@code File} is {@code null}.
* @throws AssertionError if the actual {@code File} is not an absolute path.
*/
public S isAbsolute() {
files.assertIsAbsolute(info, actual);
return myself;
}
/**
* Verifies that the actual {@code File} is a relative path.
*
* Example:
*
File relativeFile = new File("./test");
*
* // assertion will pass
* assertThat(relativeFile).isRelative();
*
* File absoluteFile = File.createTempFile("tmp", "txt");
*
* // assertion will fail
* assertThat(absoluteFile).isRelative();
*
* @return {@code this} assertion object.
* @throws AssertionError if the actual {@code File} is {@code null}.
* @throws AssertionError if the actual {@code File} is not a relative path.
*/
public S isRelative() {
files.assertIsRelative(info, actual);
return myself;
}
/**
* Verifies that the content of the actual {@code File} is equal to the content of the given one.
* The charset to use when reading the actual file can be provided with {@link #usingCharset(Charset)} or
* {@link #usingCharset(String)} prior to calling this method; if not, the platform's default charset (as returned by
* {@link Charset#defaultCharset()}) will be used.
*
* Examples:
* // use the default charset
* File xFile = Files.write(Paths.get("xfile.txt"), "The Truth Is Out There".getBytes()).toFile();
* File xFileClone = Files.write(Paths.get("xfile-clone.txt"), "The Truth Is Out There".getBytes()).toFile();
* File xFileFrench = Files.write(Paths.get("xfile-french.txt"), "La Vérité Est Ailleurs".getBytes()).toFile();
* // use UTF-8 charsest
* File xFileUTF8 = Files.write(Paths.get("xfile-clone.txt"), Arrays.asList("The Truth Is Out There"), Charset.forName("UTF-8")).toFile();
*
* // The following assertion succeeds (default charset is used):
* assertThat(xFile).hasSameContentAs(xFileClone);
* // The following assertion succeeds (UTF-8 charset is used to read xFile):
* assertThat(xFileUTF8).usingCharset("UTF-8").hasContent(xFileClone);
*
* // The following assertion fails:
* assertThat(xFile).hasSameContentAs(xFileFrench);
*
* @param expected the given {@code File} to compare the actual {@code File} to.
* @return {@code this} assertion object.
* @throws NullPointerException if the given {@code File} is {@code null}.
* @throws IllegalArgumentException if the given {@code File} is not an existing file.
* @throws AssertionError if the actual {@code File} is {@code null}.
* @throws AssertionError if the actual {@code File} is not an existing file.
* @throws RuntimeIOException if an I/O error occurs.
* @throws AssertionError if the content of the actual {@code File} is not equal to the content of the given one.
*
* @deprecated use {@link #hasSameContentAs()} instead
*/
@Deprecated
public S hasContentEqualTo(File expected) {
return hasSameContentAs(expected);
}
/**
* Verifies that the content of the actual {@code File} is equal to the content of the given one.
* The charset to use when reading the actual file can be provided with {@link #usingCharset(Charset)} or
* {@link #usingCharset(String)} prior to calling this method; if not, the platform's default charset (as returned by
* {@link Charset#defaultCharset()}) will be used.
*
* Examples:
* // use the default charset
* File xFile = Files.write(Paths.get("xfile.txt"), "The Truth Is Out There".getBytes()).toFile();
* File xFileClone = Files.write(Paths.get("xfile-clone.txt"), "The Truth Is Out There".getBytes()).toFile();
* File xFileFrench = Files.write(Paths.get("xfile-french.txt"), "La Vérité Est Ailleurs".getBytes()).toFile();
* // use UTF-8 charsest
* File xFileUTF8 = Files.write(Paths.get("xfile-clone.txt"), Arrays.asList("The Truth Is Out There"), Charset.forName("UTF-8")).toFile();
*
* // The following assertion succeeds (default charset is used):
* assertThat(xFile).hasSameContentAs(xFileClone);
* // The following assertion succeeds (UTF-8 charset is used to read xFile):
* assertThat(xFileUTF8).usingCharset("UTF-8").hasContent(xFileClone);
*
* // The following assertion fails:
* assertThat(xFile).hasSameContentAs(xFileFrench);
*
* @param expected the given {@code File} to compare the actual {@code File} to.
* @return {@code this} assertion object.
* @throws NullPointerException if the given {@code File} is {@code null}.
* @throws IllegalArgumentException if the given {@code File} is not an existing file.
* @throws AssertionError if the actual {@code File} is {@code null}.
* @throws AssertionError if the actual {@code File} is not an existing file.
* @throws RuntimeIOException if an I/O error occurs.
* @throws AssertionError if the content of the actual {@code File} is not equal to the content of the given one.
*/
public S hasSameContentAs(File expected) {
files.assertSameContentAs(info, actual, charset, expected, Charset.defaultCharset());
return myself;
}
/**
* Verifies that the content of the actual {@code File} is the same as the expected one, the expected {@code File} being read with the given charset while
* the charset used to read the actual path can be provided with {@link #usingCharset(Charset)} or
* {@link #usingCharset(String)} prior to calling this method; if not, the platform's default charset (as returned by
* {@link Charset#defaultCharset()}) will be used.
*
* Examples:
*
File fileUTF8 = Files.write(Paths.get("actual"), Collections.singleton("Gerçek"), StandardCharsets.UTF_8).toFile();
* Charset turkishCharset = Charset.forName("windows-1254");
* File fileTurkischCharset = Files.write(Paths.get("expected"), Collections.singleton("Gerçek"), turkishCharset).toFile();
*
* // The following assertion succeeds:
* assertThat(fileUTF8).usingCharset(StandardCharsets.UTF_8).hasSameContentAs(fileTurkischCharset, turkishCharset);
*
* // The following assertion fails:
* assertThat(fileUTF8).usingCharset(StandardCharsets.UTF_8).hasSameContentAs(fileTurkischCharset, StandardCharsets.UTF_8);
*
* @param expected the given {@code File} to compare the actual {@code File} to.
* @param expectedCharset the {@Charset} used to read the content of the expected file.
* @return {@code this} assertion object.
* @throws NullPointerException if the given {@code File} is {@code null}.
* @throws IllegalArgumentException if the given {@code File} is not an existing file.
* @throws AssertionError if the actual {@code File} is {@code null}.
* @throws AssertionError if the actual {@code File} is not an existing file.
* @throws RuntimeIOException if an I/O error occurs.
* @throws AssertionError if the content of the actual {@code File} is not equal to the content of the given one.
*/
public S hasSameContentAs(File expected, Charset expectedCharset) {
files.assertSameContentAs(info, actual, charset, expected, expectedCharset);
return myself;
}
/**
* Verifies that the binary content of the actual {@code File} is exactly equal to the given one.
*
* Example:
*
File bin = File.createTempFile("tmp", "bin");
* Files.write(bin.toPath(), new byte[] {1, 1});
*
* // assertion will pass
* assertThat(bin).hasBinaryContent(new byte[] {1, 1});
*
* // assertions will fail
* assertThat(bin).hasBinaryContent(new byte[] { });
* assertThat(bin).hasBinaryContent(new byte[] {0, 0});
*
* @param expected the expected binary content to compare the actual {@code File}'s content to.
* @return {@code this} assertion object.
* @throws NullPointerException if the given content is {@code null}.
* @throws AssertionError if the actual {@code File} is {@code null}.
* @throws AssertionError if the actual {@code File} is not an existing file.
* @throws RuntimeIOException if an I/O error occurs.
* @throws AssertionError if the content of the actual {@code File} is not equal to the given binary content.
*/
public S hasBinaryContent(byte[] expected) {
files.assertHasBinaryContent(info, actual, expected);
return myself;
}
/**
* Specifies the name of the charset to use for text-based assertions on the file's contents.
*
* @param charsetName the name of the charset to use.
* @return {@code this} assertion object.
* @throws IllegalArgumentException if the given encoding is not supported on this platform.
*/
public S usingCharset(String charsetName) {
if (!Charset.isSupported(charsetName))
throw new IllegalArgumentException(String.format("Charset:<'%s'> is not supported on this system", charsetName));
return usingCharset(Charset.forName(charsetName));
}
/**
* Specifies the charset to use for text-based assertions on the file's contents.
*
* @param charset the charset to use.
* @return {@code this} assertion object.
* @throws NullPointerException if the given charset is {@code null}.
*/
public S usingCharset(Charset charset) {
this.charset = checkNotNull(charset, "The charset should not be null");
return myself;
}
/**
* Verifies that the text content of the actual {@code File} is exactly equal to the given one.
* The charset to use when reading the file should be provided with {@link #usingCharset(Charset)} or
* {@link #usingCharset(String)} prior to calling this method; if not, the platform's default charset (as returned by
* {@link Charset#defaultCharset()}) will be used.
*
* Example:
*
// use the default charset
* File xFile = Files.write(Paths.get("xfile.txt"), "The Truth Is Out There".getBytes()).toFile();
*
* // The following assertion succeeds (default charset is used):
* assertThat(xFile).hasContent("The Truth Is Out There");
*
* // The following assertion fails:
* assertThat(xFile).hasContent("La Vérité Est Ailleurs");
*
* // using a specific charset
* Charset turkishCharset = Charset.forName("windows-1254");
*
* File xFileTurkish = Files.write(Paths.get("xfile.turk"), Collections.singleton("Gerçek"), turkishCharset).toFile();
*
* // The following assertion succeeds:
* assertThat(xFileTurkish).usingCharset(turkishCharset).hasContent("Gerçek");
*
* // The following assertion fails :
* assertThat(xFileTurkish).usingCharset(StandardCharsets.UTF_8).hasContent("Gerçek");
*
* @param expected the expected text content to compare the actual {@code File}'s content to.
* @return {@code this} assertion object.
* @throws NullPointerException if the given content is {@code null}.
* @throws AssertionError if the actual {@code File} is {@code null}.
* @throws AssertionError if the actual {@code File} is not an existing file.
* @throws RuntimeIOException if an I/O error occurs.
* @throws AssertionError if the content of the actual {@code File} is not equal to the given content.
*/
public S hasContent(String expected) {
files.assertHasContent(info, actual, expected, charset);
return myself;
}
/**
* Verifies that the actual {@code File} can be modified by the application.
*
* Example:
*
File tmpFile = File.createTempFile("tmp", "txt");
* File tmpDir = Files.createTempDirectory("tmp").toFile();
*
* // assertions will pass
* assertThat(tmpFile).canWrite();
* assertThat(tmpDir).canWrite();
*
* tmpFile.setReadOnly();
* tmpDir.setReadOnly();
*
* // assertions will fail
* assertThat(tmpFile).canWrite();
* assertThat(tmpDir).canWrite();
*
* @return {@code this} assertion object.
* @throws AssertionError if the actual {@code File} is {@code null}.
* @throws AssertionError if the actual {@code File} can not be modified by the application.
*/
public S canWrite() {
files.assertCanWrite(info, actual);
return myself;
}
/**
* Verifies that the actual {@code File} can be read by the application.
*
* Example:
*
File tmpFile = File.createTempFile("tmp", "txt");
* File tmpDir = Files.createTempDirectory("tmp").toFile();
*
* // assertions will pass
* assertThat(tmpFile).canRead();
* assertThat(tmpDir).canRead();
*
* tmpFile.setReadable(false);
* tmpDir.setReadable(false);
*
* // assertions will fail
* assertThat(tmpFile).canRead();
* assertThat(tmpDir).canRead();
*
* @return {@code this} assertion object.
* @throws AssertionError if the actual {@code File} is {@code null}.
* @throws AssertionError if the actual {@code File} can not be read by the application.
*/
public S canRead() {
files.assertCanRead(info, actual);
return myself;
}
/**
* Verifies that the actual {@code File} has given parent.
*
*
* Example:
*
File xFile = new File("mulder/xFile");
*
* // assertion will pass
* assertThat(xFile).hasParent(new File("mulder"));
*
* // assertion will fail
* assertThat(xFile).hasParent(new File("scully"));
*
*
*
* @param expected the expected parent {@code File}.
* @return {@code this} assertion object.
* @throws NullPointerException if the expected parent {@code File} is {@code null}.
* @throws RuntimeIOException if an I/O error occurs.
* @throws AssertionError if the actual {@code File} is {@code null}.
* @throws AssertionError if the actual {@code File} parent is not equal to the expected one.
*
* @see java.io.File#getParentFile() parent definition.
*/
public S hasParent(File expected) {
files.assertHasParent(info, actual, expected);
return myself;
}
/**
* Same as {@link #hasParent(java.io.File)} but takes care of converting given {@code String} as {@code File} for you
*
*
* Example:
*
File xFile = new File("mulder/xFile");
*
* // assertion will pass
* assertThat(xFile).hasParent("mulder");
*
* // assertion will fail
* assertThat(xFile).hasParent("scully");
*
*
*/
public S hasParent(String expected) {
files.assertHasParent(info, actual, expected != null ? new File(expected) : null);
return myself;
}
/**
* Verifies that the actual {@code File} has given extension.
*
*
* Example:
*
File xFile = new File("xFile.java");
*
* // assertion will pass
* assertThat(xFile).hasExtension("java");
*
* // assertion will fail
* assertThat(xFile).hasExtension("png");
*
*
*
* @param expected the expected extension, it does not contains the {@code '.'}
* @return {@code this} assertion object.
* @throws NullPointerException if the expected extension is {@code null}.
* @throws AssertionError if the actual {@code File} is {@code null}.
* @throws AssertionError if the actual {@code File} is not a file (ie a directory).
* @throws AssertionError if the actual {@code File} does not have the expected extension.
*
* @see Filename extension
*/
public S hasExtension(String expected) {
files.assertHasExtension(info, actual, expected);
return myself;
}
/**
* Verifies that the actual {@code File} has given name.
*
*
* Example:
*
File xFile = new File("somewhere/xFile.java");
* File xDirectory = new File("somewhere/xDirectory");
*
* // assertion will pass
* assertThat(xFile).hasName("xFile.java");
* assertThat(xDirectory).hasName("xDirectory");
*
* // assertion will fail
* assertThat(xFile).hasName("xFile");
* assertThat(xDirectory).hasName("somewhere");
*
*
*
* @param expected the expected {@code File} name.
* @return {@code this} assertion object.
* @throws NullPointerException if the expected name is {@code null}.
* @throws AssertionError if the actual {@code File} is {@code null}.
* @throws AssertionError if the actual {@code File} does not have the expected name.
*
* @see java.io.File#getName() name definition.
*/
public S hasName(String expected) {
files.assertHasName(info, actual, expected);
return myself;
}
/**
* Verifies that the actual {@code File} does not have a parent.
*
*
* Example:
*
File xFile = new File("somewhere/xFile.java");
* File xDirectory = new File("xDirectory");
*
* // assertion will pass
* assertThat(xDirectory).hasNoParent();
*
* // assertion will fail
* assertThat(xFile).hasNoParent();
*
*
*
* @return {@code this} assertion object.
*
* @throws AssertionError if the actual {@code File} is {@code null}.
* @throws AssertionError if the actual {@code File} has a parent.
*/
public S hasNoParent() {
files.assertHasNoParent(info, actual);
return myself;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy