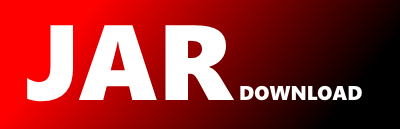
com.fitbur.assertj.api.AbstractLocalDateAssert Maven / Gradle / Ivy
/**
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
* Copyright 2012-2016 the original author or authors.
*/
package com.fitbur.assertj.api;
import static com.fitbur.assertj.error.ShouldBeAfter.shouldBeAfter;
import static com.fitbur.assertj.error.ShouldBeAfterOrEqualsTo.shouldBeAfterOrEqualsTo;
import static com.fitbur.assertj.error.ShouldBeBefore.shouldBeBefore;
import static com.fitbur.assertj.error.ShouldBeBeforeOrEqualsTo.shouldBeBeforeOrEqualsTo;
import static com.fitbur.assertj.error.ShouldBeToday.shouldBeToday;
import java.time.LocalDate;
import com.fitbur.assertj.internal.Failures;
import com.fitbur.assertj.internal.Objects;
/**
* Assertions for {@link LocalDate} type from new Date & Time API introduced in Java 8.
*/
public abstract class AbstractLocalDateAssert>
extends AbstractAssert {
public static final String NULL_LOCAL_DATE_TIME_PARAMETER_MESSAGE = "The LocalDate to compare actual with should not be null";
/**
* Creates a new {@link com.fitbur.assertj.api.AbstractLocalDateAssert}
.
*
* @param selfType the "self type"
* @param actual the actual value to verify
*/
protected AbstractLocalDateAssert(LocalDate actual, Class> selfType) {
super(actual, selfType);
}
// visible for test
protected LocalDate getActual() {
return actual;
}
/**
* Verifies that the actual {@code LocalDate} is strictly before the given one.
*
* Example :
*
assertThat(parse("2000-01-01")).isBefore(parse("2000-01-02"));
*
* @param other the given {@link LocalDate}.
* @return this assertion object.
* @throws AssertionError if the actual {@code LocalDate} is {@code null}.
* @throws IllegalArgumentException if other {@code LocalDate} is {@code null}.
* @throws AssertionError if the actual {@code LocalDate} is not strictly before the given one.
*/
public S isBefore(LocalDate other) {
Objects.instance().assertNotNull(info, actual);
assertLocalDateParameterIsNotNull(other);
if (!actual.isBefore(other)) throw Failures.instance().failure(info, shouldBeBefore(actual, other));
return myself;
}
/**
* Same assertion as {@link #isBefore(LocalDate)} but the {@link LocalDate} is built from given String, which
* must follow ISO LocalDate format to allow calling {@link LocalDate#parse(CharSequence)} method.
*
* Example :
*
// use directly String in comparison to avoid writing the code to perform the conversion
* assertThat(parse("2000-01-01")).isBefore("2000-01-02");
*
* @param localDateTimeAsString String representing a {@link LocalDate}.
* @return this assertion object.
* @throws AssertionError if the actual {@code LocalDate} is {@code null}.
* @throws IllegalArgumentException if given String is null or can't be converted to a {@link LocalDate}.
* @throws AssertionError if the actual {@code LocalDate} is not strictly before the {@link LocalDate} built
* from given String.
*/
public S isBefore(String localDateTimeAsString) {
assertLocalDateAsStringParameterIsNotNull(localDateTimeAsString);
return isBefore(LocalDate.parse(localDateTimeAsString));
}
/**
* Verifies that the actual {@code LocalDate} is before or equals to the given one.
*
* Example :
*
assertThat(parse("2000-01-01")).isBeforeOrEqualTo(parse("2000-01-01"))
* .isBeforeOrEqualTo(parse("2000-01-02"));
*
* @param other the given {@link LocalDate}.
* @return this assertion object.
* @throws AssertionError if the actual {@code LocalDate} is {@code null}.
* @throws IllegalArgumentException if other {@code LocalDate} is {@code null}.
* @throws AssertionError if the actual {@code LocalDate} is not before or equals to the given one.
*/
public S isBeforeOrEqualTo(LocalDate other) {
Objects.instance().assertNotNull(info, actual);
assertLocalDateParameterIsNotNull(other);
if (actual.isAfter(other)) {
throw Failures.instance().failure(info, shouldBeBeforeOrEqualsTo(actual, other));
}
return myself;
}
/**
* Same assertion as {@link #isBeforeOrEqualTo(LocalDate)} but the {@link LocalDate} is built from given
* String, which must follow ISO LocalDate format to allow calling {@link LocalDate#parse(CharSequence)} method.
*
* Example :
*
// use String in comparison to avoid conversion
* assertThat(parse("2000-01-01")).isBeforeOrEqualTo("2000-01-01")
* .isBeforeOrEqualTo("2000-01-02");
*
* @param localDateTimeAsString String representing a {@link LocalDate}.
* @return this assertion object.
* @throws AssertionError if the actual {@code LocalDate} is {@code null}.
* @throws IllegalArgumentException if given String is null or can't be converted to a {@link LocalDate}.
* @throws AssertionError if the actual {@code LocalDate} is not before or equals to the {@link LocalDate} built from
* given String.
*/
public S isBeforeOrEqualTo(String localDateTimeAsString) {
assertLocalDateAsStringParameterIsNotNull(localDateTimeAsString);
return isBeforeOrEqualTo(LocalDate.parse(localDateTimeAsString));
}
/**
* Verifies that the actual {@code LocalDate} is after or equals to the given one.
*
* Example :
*
assertThat(parse("2000-01-01")).isAfterOrEqualTo(parse("2000-01-01"))
* .isAfterOrEqualTo(parse("1999-12-31"));
*
* @param other the given {@link LocalDate}.
* @return this assertion object.
* @throws AssertionError if the actual {@code LocalDate} is {@code null}.
* @throws IllegalArgumentException if other {@code LocalDate} is {@code null}.
* @throws AssertionError if the actual {@code LocalDate} is not after or equals to the given one.
*/
public S isAfterOrEqualTo(LocalDate other) {
Objects.instance().assertNotNull(info, actual);
assertLocalDateParameterIsNotNull(other);
if (actual.isBefore(other)) {
throw Failures.instance().failure(info, shouldBeAfterOrEqualsTo(actual, other));
}
return myself;
}
/**
* Same assertion as {@link #isAfterOrEqualTo(LocalDate)} but the {@link LocalDate} is built from given
* String, which must follow ISO LocalDate format to allow calling {@link LocalDate#parse(CharSequence)} method.
*
* Example :
*
// use String in comparison to avoid conversion
* assertThat(parse("2000-01-01")).isAfterOrEqualTo("2000-01-01")
* .isAfterOrEqualTo("1999-12-31");
*
* @param localDateTimeAsString String representing a {@link LocalDate}.
* @return this assertion object.
* @throws AssertionError if the actual {@code LocalDate} is {@code null}.
* @throws IllegalArgumentException if given String is null or can't be converted to a {@link LocalDate}.
* @throws AssertionError if the actual {@code LocalDate} is not after or equals to the {@link LocalDate} built from
* given String.
*/
public S isAfterOrEqualTo(String localDateTimeAsString) {
assertLocalDateAsStringParameterIsNotNull(localDateTimeAsString);
return isAfterOrEqualTo(LocalDate.parse(localDateTimeAsString));
}
/**
* Verifies that the actual {@code LocalDate} is strictly after the given one.
*
* Example :
*
assertThat(parse("2000-01-01")).isAfter(parse("1999-12-31"));
*
* @param other the given {@link LocalDate}.
* @return this assertion object.
* @throws AssertionError if the actual {@code LocalDate} is {@code null}.
* @throws IllegalArgumentException if other {@code LocalDate} is {@code null}.
* @throws AssertionError if the actual {@code LocalDate} is not strictly after the given one.
*/
public S isAfter(LocalDate other) {
Objects.instance().assertNotNull(info, actual);
assertLocalDateParameterIsNotNull(other);
if (!actual.isAfter(other)) {
throw Failures.instance().failure(info, shouldBeAfter(actual, other));
}
return myself;
}
/**
* Same assertion as {@link #isAfter(LocalDate)} but the {@link LocalDate} is built from given a String that
* must follow ISO LocalDate format to allow calling {@link LocalDate#parse(CharSequence)} method.
*
* Example :
*
// use String in comparison to avoid conversion
* assertThat(parse("2000-01-01")).isAfter("1999-12-31");
*
* @param localDateTimeAsString String representing a {@link LocalDate}.
* @return this assertion object.
* @throws AssertionError if the actual {@code LocalDate} is {@code null}.
* @throws IllegalArgumentException if given String is null or can't be converted to a {@link LocalDate}.
* @throws AssertionError if the actual {@code LocalDate} is not strictly after the {@link LocalDate} built
* from given String.
*/
public S isAfter(String localDateTimeAsString) {
assertLocalDateAsStringParameterIsNotNull(localDateTimeAsString);
return isAfter(LocalDate.parse(localDateTimeAsString));
}
/**
* Same assertion as {@link #isEqualTo(Object)} (where Object is expected to be {@link LocalDate}) but here you
* pass {@link LocalDate} String representation that must follow ISO LocalDate format to allow calling {@link LocalDate#parse(CharSequence)} method.
*
* Example :
*
// use directly String in comparison to avoid writing the code to perform the conversion
* assertThat(parse("2000-01-01")).isEqualTo("2000-01-01");
*
* @param dateTimeAsString String representing a {@link LocalDate}.
* @return this assertion object.
* @throws AssertionError if the actual {@code LocalDate} is {@code null}.
* @throws IllegalArgumentException if given String is null or can't be converted to a {@link LocalDate}.
* @throws AssertionError if the actual {@code LocalDate} is not equal to the {@link LocalDate} built from
* given String.
*/
public S isEqualTo(String dateTimeAsString) {
assertLocalDateAsStringParameterIsNotNull(dateTimeAsString);
return isEqualTo(LocalDate.parse(dateTimeAsString));
}
/**
* Same assertion as {@link #isNotEqualTo(Object)} (where Object is expected to be {@link LocalDate}) but here you
* pass {@link LocalDate} String representation that must follow ISO LocalDate format to allow calling {@link LocalDate#parse(CharSequence)} method.
*
* Example :
*
// use directly String in comparison to avoid writing the code to perform the conversion
* assertThat(parse("2000-01-01")).isNotEqualTo("2000-01-15");
*
* @param dateTimeAsString String representing a {@link LocalDate}.
* @return this assertion object.
* @throws AssertionError if the actual {@code LocalDate} is {@code null}.
* @throws IllegalArgumentException if given String is null or can't be converted to a {@link LocalDate}.
* @throws AssertionError if the actual {@code LocalDate} is equal to the {@link LocalDate} built from given
* String.
*/
public S isNotEqualTo(String dateTimeAsString) {
assertLocalDateAsStringParameterIsNotNull(dateTimeAsString);
return isNotEqualTo(LocalDate.parse(dateTimeAsString));
}
/**
* Same assertion as {@link #isIn(Object...)} (where Objects are expected to be {@link LocalDate}) but here you
* pass {@link LocalDate} String representations that must follow ISO LocalDate format to allow calling {@link LocalDate#parse(CharSequence)} method.
*
* Example :
*
// use String based representation of LocalDate
* assertThat(parse("2000-01-01")).isIn("1999-12-31", "2000-01-01");
*
* @param dateTimesAsString String array representing {@link LocalDate}s.
* @return this assertion object.
* @throws AssertionError if the actual {@code LocalDate} is {@code null}.
* @throws IllegalArgumentException if given String is null or can't be converted to a {@link LocalDate}.
* @throws AssertionError if the actual {@code LocalDate} is not in the {@link LocalDate}s built from given
* Strings.
*/
public S isIn(String... dateTimesAsString) {
checkIsNotNullAndNotEmpty(dateTimesAsString);
return isIn(convertToLocalDateArray(dateTimesAsString));
}
/**
* Same assertion as {@link #isNotIn(Object...)} (where Objects are expected to be {@link LocalDate}) but here you
* pass {@link LocalDate} String representations that must follow ISO LocalDate format to allow calling {@link LocalDate#parse(CharSequence)} method.
*
* Example :
*
// use String based representation of LocalDate
* assertThat(parse("2000-01-01")).isNotIn("1999-12-31", "2000-01-02");
*
* @param dateTimesAsString Array of String representing a {@link LocalDate}.
* @return this assertion object.
* @throws AssertionError if the actual {@code LocalDate} is {@code null}.
* @throws IllegalArgumentException if given String is null or can't be converted to a {@link LocalDate}.
* @throws AssertionError if the actual {@code LocalDate} is in the {@link LocalDate}s built from given
* Strings.
*/
public S isNotIn(String... dateTimesAsString) {
checkIsNotNullAndNotEmpty(dateTimesAsString);
return isNotIn(convertToLocalDateArray(dateTimesAsString));
}
/**
* Verifies that the actual {@code LocalDate} is today, that is matching current year, month and day.
*
* Example:
* // assertion will pass
* assertThat(LocalDate.now()).isToday();
*
* // assertion will fail
* assertThat(theFellowshipOfTheRing.getReleaseDate()).isToday();
*
* @return this assertion object.
* @throws AssertionError if the actual {@code LocalDate} is {@code null}.
* @throws AssertionError if the actual {@code LocalDate} is not today.
*/
public S isToday() {
Objects.instance().assertNotNull(info, actual);
if (!actual.isEqual(LocalDate.now())) throw Failures.instance().failure(info, shouldBeToday(actual));
return myself;
}
private static Object[] convertToLocalDateArray(String... dateTimesAsString) {
LocalDate[] dates = new LocalDate[dateTimesAsString.length];
for (int i = 0; i < dateTimesAsString.length; i++) {
dates[i] = LocalDate.parse(dateTimesAsString[i]);
}
return dates;
}
private void checkIsNotNullAndNotEmpty(Object[] values) {
if (values == null) throw new IllegalArgumentException("The given LocalDate array should not be null");
if (values.length == 0) throw new IllegalArgumentException("The given LocalDate array should not be empty");
}
/**
* Check that the {@link LocalDate} string representation to compare actual {@link LocalDate} to is not null,
* otherwise throws a {@link IllegalArgumentException} with an explicit message
*
* @param localDateTimeAsString String representing the {@link LocalDate} to compare actual with
* @throws IllegalArgumentException with an explicit message if the given {@link String} is null
*/
private static void assertLocalDateAsStringParameterIsNotNull(String localDateTimeAsString) {
// @format:off
if (localDateTimeAsString == null) throw new IllegalArgumentException("The String representing the LocalDate to compare actual with should not be null");
// @format:on
}
/**
* Check that the {@link LocalDate} to compare actual {@link LocalDate} to is not null, in that case throws a
* {@link IllegalArgumentException} with an explicit message
*
* @param other the {@link LocalDate} to check
* @throws IllegalArgumentException with an explicit message if the given {@link LocalDate} is null
*/
private static void assertLocalDateParameterIsNotNull(LocalDate other) {
if (other == null) throw new IllegalArgumentException("The LocalDate to compare actual with should not be null");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy