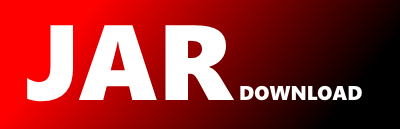
com.fitbur.assertj.api.AbstractMapAssert Maven / Gradle / Ivy
/**
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
* Copyright 2012-2016 the original author or authors.
*/
package com.fitbur.assertj.api;
import static com.fitbur.assertj.data.MapEntry.entry;
import static com.fitbur.assertj.util.Arrays.array;
import java.util.Comparator;
import java.util.Map;
import com.fitbur.assertj.description.Description;
import com.fitbur.assertj.internal.Maps;
import com.fitbur.assertj.util.VisibleForTesting;
/**
* Base class for all implementations of assertions for {@link Map}s.
*
* @param the "self" type of this assertion class. Please read "Emulating 'self types' using Java Generics to simplify fluent API implementation"
* for more details.
* @param the type of the "actual" value.
* @param the type of keys in map.
* @param the type of values in map.
*
* @author David DIDIER
* @author Yvonne Wang
* @author Alex Ruiz
* @author Mikhail Mazursky
* @author Nicolas François
* @author dorzey
*/
public abstract class AbstractMapAssert, A extends Map, K, V>
extends AbstractAssert implements EnumerableAssert> {
@VisibleForTesting
Maps maps = Maps.instance();
protected AbstractMapAssert(A actual, Class> selfType) {
super(actual, selfType);
}
/**
* Verifies that the {@link Map} is {@code null} or empty.
*
* Example:
*
// assertions will pass
* Map<Integer, String> map = null;
* assertThat(map).isNullOrEmpty();
* assertThat(new HashMap()).isNullOrEmpty();
*
* // assertion will fail
* Map<String, String> keyToValue = new HashMap();
* keyToValue.put("key", "value");
* assertThat(keyToValue).isNullOrEmpty()
*
* @throws AssertionError if the {@link Map} is not {@code null} or not empty.
*/
@Override
public void isNullOrEmpty() {
maps.assertNullOrEmpty(info, actual);
}
/**
* Verifies that the {@link Map} is empty.
*
* Example:
*
// assertion will pass
* assertThat(new HashMap()).isEmpty();
*
* // assertion will fail
* Map<String, String> map = new HashMap();
* map.put("key", "value");
* assertThat(map).isEmpty();
*
* @throws AssertionError if the {@link Map} of values is not empty.
*/
@Override
public void isEmpty() {
maps.assertEmpty(info, actual);
}
/**
* Verifies that the {@link Map} is not empty.
*
* Example:
*
Map<String, String> map = new HashMap();
* map.put("key", "value");
*
* // assertion will pass
* assertThat(map).isNotEmpty();
*
* // assertion will fail
* assertThat(new HashMap()).isNotEmpty();
*
* @return {@code this} assertion object.
* @throws AssertionError if the {@link Map} is empty.
*/
@Override
public S isNotEmpty() {
maps.assertNotEmpty(info, actual);
return myself;
}
/**
* Verifies that the number of values in the {@link Map} is equal to the given one.
*
* Example:
*
* Map<String, String> map = new HashMap();
* map.put("key", "value");
*
* // assertion will pass
* assertThat(map).hasSize(1);
*
* // assertions will fail
* assertThat(map).hasSize(0);
* assertThat(map).hasSize(2);
*
* @param expected the expected number of values in the {@link Map}.
* @return {@code this} assertion object.
* @throws AssertionError if the number of values of the {@link Map} is not equal to the given one.
*/
@Override
public S hasSize(int expected) {
maps.assertHasSize(info, actual, expected);
return myself;
}
/**
* Verifies that the actual map has the same size as given array.
*
* Parameter is declared as Object to accept both Object[] and primitive arrays (e.g. int[]).
*
* Example:
* int[] oneTwoThree = {1, 2, 3};
*
* Map<Ring, TolkienCharacter> elvesRingBearers = new HashMap<>();
* elvesRingBearers.put(nenya, galadriel);
* elvesRingBearers.put(narya, gandalf);
* elvesRingBearers.put(vilya, elrond);
*
* // assertion will pass
* assertThat(elvesRingBearers).hasSameSizeAs(oneTwoThree);
*
* // assertions will fail
* assertThat(elvesRingBearers).hasSameSizeAs(new int[] {1});
* assertThat(keyToValue).hasSameSizeAs(new char[] {'a', 'b', 'c', 'd'});
*
* @param array the array to compare size with actual group.
* @return {@code this} assertion object.
* @throws AssertionError if the actual group is {@code null}.
* @throws AssertionError if the array parameter is {@code null} or is not a true array.
* @throws AssertionError if actual group and given array don't have the same size.
*/
public S hasSameSizeAs(Object other) {
maps.assertHasSameSizeAs(info, actual, other);
return myself;
}
/**
* Verifies that the actual map has the same size as the given {@link Iterable}.
*
* Example :
*
Map<Ring, TolkienCharacter> elvesRingBearers = new HashMap<>();
* elvesRingBearers.put(nenya, galadriel);
* elvesRingBearers.put(narya, gandalf);
* elvesRingBearers.put(vilya, elrond);
*
* // assertion will pass
* assertThat(elvesRingBearers).hasSameSizeAs(Array.asList(vilya, nenya, narya));
*
* // assertions will fail
* assertThat(elvesRingBearers).hasSameSizeAs(Array.asList(1));
* assertThat(keyToValue).hasSameSizeAs(Array.asList('a', 'b', 'c', 'd'));
*
* @param other the {@code Iterable} to compare size with actual group.
* @return {@code this} assertion object.
* @throws AssertionError if the actual map is {@code null}.
* @throws AssertionError if the other {@code Iterable} is {@code null}.
* @throws AssertionError if the actual map and the given {@code Iterable} don't have the same size
*/
@Override
public S hasSameSizeAs(Iterable> other) {
maps.assertHasSameSizeAs(info, actual, other);
return myself;
}
/**
* Verifies that the actual map has the same size as the given {@link Map}.
*
* Example :
*
Map<Ring, TolkienCharacter> ringBearers = new HashMap<>();
* ringBearers.put(nenya, galadriel);
* ringBearers.put(narya, gandalf);
* ringBearers.put(vilya, elrond);
* ringBearers.put(oneRing, frodo);
*
* // assertion will pass
* assertThat(ringBearers).hasSameSizeAs(mapOf(entry(oneRing, frodo),
* entry(narya, gandalf),
* entry(nenya, galadriel),
* entry(vilya, elrond)));
*
* // assertions will fail
* assertThat(elvesRingBearers).hasSameSizeAs(new HashMap());
* Map<String, String> keyToValue = new HashMap();
* keyToValue.put("key", "value");
* assertThat(keyToValue).hasSameSizeAs(keyToValue);
*
* @param other the {@code Map} to compare size with actual map
* @return {@code this} assertion object
* @throws NullPointerException if the other {@code Map} is {@code null}
* @throws AssertionError if the actual map is {@code null}
* @throws AssertionError if the actual map and the given {@code Map} don't have the same size
*/
public S hasSameSizeAs(Map, ?> other) {
maps.assertHasSameSizeAs(info, actual, other);
return myself;
}
/**
* Verifies that the actual map contains the given entries, in any order.
*
* Example :
*
Map<Ring, TolkienCharacter> ringBearers = new HashMap<>();
* ringBearers.put(nenya, galadriel);
* ringBearers.put(narya, gandalf);
* ringBearers.put(vilya, elrond);
* ringBearers.put(oneRing, frodo);
*
* // assertion will pass
* assertThat(ringBearers).contains(entry(oneRing, frodo), entry(nenya, galadriel));
*
* // assertions will fail
* assertThat(ringBearers).contains(entry(oneRing, sauron));
* assertThat(ringBearers).contains(entry(oneRing, sauron), entry(oneRing, aragorn));
* assertThat(ringBearers).contains(entry(narya, gandalf), entry(oneRing, sauron));
*
* @param entries the given entries.
* @return {@code this} assertion object.
* @throws NullPointerException if the given argument is {@code null}.
* @throws IllegalArgumentException if the given argument is an empty array.
* @throws NullPointerException if any of the entries in the given array is {@code null}.
* @throws AssertionError if the actual map is {@code null}.
* @throws AssertionError if the actual map does not contain the given entries.
*/
public S contains(@SuppressWarnings("unchecked") Map.Entry extends K, ? extends V>... entries) {
maps.assertContains(info, actual, entries);
return myself;
}
/**
* Verifies that the actual map contains all entries of the given map, in any order.
*
* Example :
*
Map<Ring, TolkienCharacter> ringBearers = new HashMap<>();
* ringBearers.put(nenya, galadriel);
* ringBearers.put(narya, gandalf);
* ringBearers.put(vilya, elrond);
* ringBearers.put(oneRing, frodo);
*
* Map<Ring, TolkienCharacter> elvesRingBearers = new HashMap<>();
* elvesRingBearers.put(nenya, galadriel);
* elvesRingBearers.put(narya, gandalf);
* elvesRingBearers.put(vilya, elrond);
*
* // assertion will succeed
* assertThat(ringBearers).containsAllEntriesOf(elvesRingBearers);
*
* // assertion will fail
* assertThat(elvesRingBearers).containsAllEntriesOf(ringBearers);
*
* @param the map with the given entries.
* @return {@code this} assertion object.
* @throws NullPointerException if the given argument is {@code null}.
* @throws NullPointerException if any of the entries in the given map is {@code null}.
* @throws AssertionError if the actual map is {@code null}.
* @throws AssertionError if the actual map does not contain the given entries.
*/
public S containsAllEntriesOf(Map extends K, ? extends V> other) {
@SuppressWarnings("unchecked")
Map.Entry extends K, ? extends V>[] entries = other.entrySet().toArray(new Map.Entry[other.size()]);
maps.assertContains(info, actual, entries);
return myself;
}
/**
* Verifies that the actual map contains the given entry.
*
* Example :
*
Map<Ring, TolkienCharacter> ringBearers = new HashMap<>();
* ringBearers.put(nenya, galadriel);
* ringBearers.put(narya, gandalf);
* ringBearers.put(vilya, elrond);
* ringBearers.put(oneRing, frodo);
*
* // assertions will pass
* assertThat(ringBearers).containsEntry(oneRing, frodo).containsEntry(nenya, galadriel);
*
* // assertion will fail
* assertThat(ringBearers).containsEntry(oneRing, sauron);
*
* @param key the given key to check.
* @param value the given value to check.
* @return {@code this} assertion object.
* @throws NullPointerException if the given argument is {@code null}.
* @throws IllegalArgumentException if the given argument is an empty array.
* @throws NullPointerException if any of the entries in the given array is {@code null}.
* @throws AssertionError if the actual map is {@code null}.
* @throws AssertionError if the actual map does not contain the given entries.
*/
public S containsEntry(K key, V value) {
maps.assertContains(info, actual, array(entry(key, value)));
return myself;
}
/**
* Verifies that the actual map does not contain the given entries.
*
* Example :
*
Map<Ring, TolkienCharacter> ringBearers = new HashMap<>();
* ringBearers.put(nenya, galadriel);
* ringBearers.put(narya, gandalf);
* ringBearers.put(vilya, elrond);
* ringBearers.put(oneRing, frodo);
*
* // assertion will pass
* assertThat(ringBearers).doesNotContain(entry(oneRing, aragorn), entry(oneRing, sauron));
*
* // assertions will fail
* assertThat(ringBearers).doesNotContain(entry(oneRing, frodo));
* assertThat(ringBearers).doesNotContain(entry(oneRing, frodo), entry(oneRing, aragorn));
*
* @param entries the given entries.
* @return {@code this} assertion object.
* @throws NullPointerException if the given argument is {@code null}.
* @throws IllegalArgumentException if the given argument is an empty array.
* @throws AssertionError if the actual map is {@code null}.
* @throws AssertionError if the actual map contains any of the given entries.
*/
public S doesNotContain(@SuppressWarnings("unchecked") Map.Entry extends K, ? extends V>... entries) {
maps.assertDoesNotContain(info, actual, entries);
return myself;
}
/**
* Verifies that the actual map does not contain the given entry.
*
* Example :
*
Map<Ring, TolkienCharacter> ringBearers = new HashMap<>();
* ringBearers.put(nenya, galadriel);
* ringBearers.put(narya, gandalf);
* ringBearers.put(vilya, elrond);
* ringBearers.put(oneRing, frodo);
*
* // assertion will pass
* assertThat(ringBearers).doesNotContainEntry(oneRing, aragorn);
*
* // assertion will fail
* assertThat(ringBearers).doesNotContain(oneRing, frodo);
*
* @param key key of the entry.
* @param value value of the entry.
* @return {@code this} assertion object.
* @throws NullPointerException if the given argument is {@code null}.
* @throws IllegalArgumentException if the given argument is an empty array.
* @throws AssertionError if the actual map is {@code null}.
* @throws AssertionError if the actual map contains any of the given entries.
*/
public S doesNotContainEntry(K key, V value) {
maps.assertDoesNotContain(info, actual, array(entry(key, value)));
return myself;
}
/**
* Verifies that the actual map contains the given key.
*
* Example :
*
Map<Ring, TolkienCharacter> ringBearers = new HashMap<>();
* ringBearers.put(nenya, galadriel);
* ringBearers.put(narya, gandalf);
* ringBearers.put(vilya, elrond);
*
* // assertion will pass
* assertThat(ringBearers).containsKey(vilya);
*
* // assertion will fail
* assertThat(ringBearers).containsKey(oneRing);
*
* @param key the given key
* @throws AssertionError if the actual map is {@code null}.
* @throws AssertionError if the actual map does not contain the given key.
*/
@SuppressWarnings("unchecked")
public S containsKey(K key) {
return containsKeys(key);
}
/**
* Verifies that the actual map contains the given keys.
*
* Example :
*
Map<Ring, TolkienCharacter> ringBearers = new HashMap<>();
* ringBearers.put(nenya, galadriel);
* ringBearers.put(narya, gandalf);
* ringBearers.put(oneRing, frodo);
*
* // assertions will pass
* assertThat(ringBearers).containsKeys(nenya, oneRing);
*
* // assertions will fail
* assertThat(ringBearers).containsKeys(vilya);
* assertThat(ringBearers).containsKeys(vilya, oneRing);
*
* @param keys the given keys
* @throws AssertionError if the actual map is {@code null}.
* @throws AssertionError if the actual map does not contain the given key.
* @throws IllegalArgumentException if the given argument is an empty array.
*/
public S containsKeys(@SuppressWarnings("unchecked") K... keys) {
maps.assertContainsKeys(info, actual, keys);
return myself;
}
/**
* Verifies that the actual map does not contain the given key.
*
* Example :
*
Map<Ring, TolkienCharacter> elvesRingBearers = new HashMap<>();
* elvesRingBearers.put(nenya, galadriel);
* elvesRingBearers.put(narya, gandalf);
* elvesRingBearers.put(vilya, elrond);
*
* // assertion will pass
* assertThat(elvesRingBearers).doesNotContainKey(oneRing);
*
* // assertion will fail
* assertThat(elvesRingBearers).doesNotContainKey(vilya);
*
* @param key the given key
* @throws AssertionError if the actual map is {@code null}.
* @throws AssertionError if the actual map contains the given key.
*/
@SuppressWarnings("unchecked")
public S doesNotContainKey(K key) {
return doesNotContainKeys(key);
}
/**
* Verifies that the actual map does not contain any of the given keys.
*
* Example :
*
Map<Ring, TolkienCharacter> elvesRingBearers = new HashMap<>();
* elvesRingBearers.put(nenya, galadriel);
* elvesRingBearers.put(narya, gandalf);
* elvesRingBearers.put(vilya, elrond);
*
* // assertion will pass
* assertThat(elvesRingBearers).doesNotContainKeys(oneRing, someManRing);
*
* // assertions will fail
* assertThat(elvesRingBearers).doesNotContainKeys(vilya, nenya);
* assertThat(elvesRingBearers).doesNotContainKeys(vilya, oneRing);
*
* @param key the given key
* @throws AssertionError if the actual map is {@code null}.
* @throws AssertionError if the actual map contains the given key.
*/
public S doesNotContainKeys(@SuppressWarnings("unchecked") K... keys) {
maps.assertDoesNotContainKeys(info, actual, keys);
return myself;
}
/**
* Verifies that the actual map contains only the given keys and nothing else, in any order.
*
*
* Examples :
*
Map<Ring, TolkienCharacter> ringBearers = new HashMap<>();
* ringBearers.put(nenya, galadriel);
* ringBearers.put(narya, gandalf);
* ringBearers.put(vilya, elrond);
* ringBearers.put(oneRing, frodo);
*
* // assertion will pass
* assertThat(ringBearers).containsOnlyKeys(oneRing, nenya, narya, vilya);
*
* // assertion will fail
* assertThat(ringBearers).containsOnlyKeys(oneRing, nenya);
*
* @param keys the given keys that should be in the actual map.
* @throws AssertionError if the actual map is {@code null}.
* @throws AssertionError if the actual map does not contain the given keys, i.e. the actual map contains some or none
* of the given keys, or the actual map contains more entries than the given ones.
* @throws IllegalArgumentException if the given argument is an empty array.
*/
public S containsOnlyKeys(@SuppressWarnings("unchecked") K... keys) {
maps.assertContainsOnlyKeys(info, actual, keys);
return myself;
}
/**
* Verifies that the actual map contains the given value.
*
* Example :
*
Map<Ring, TolkienCharacter> ringBearers = new HashMap<>();
* ringBearers.put(nenya, galadriel);
* ringBearers.put(narya, gandalf);
* ringBearers.put(vilya, elrond);
* ringBearers.put(oneRing, frodo);
*
* // assertion will pass
* assertThat(ringBearers).containsValue(frodo);
*
* // assertion will fail
* assertThat(ringBearers).containsValue(sauron);
*
* @param value the value to look for.
* @throws AssertionError if the actual map is {@code null}.
* @throws AssertionError if the actual map does not contain the given value.
*/
public S containsValue(V value) {
maps.assertContainsValue(info, actual, value);
return myself;
}
/**
* Verifies that the actual map contains the given values.
*
* Example :
*
Map<Ring, TolkienCharacter> ringBearers = new HashMap<>();
* ringBearers.put(nenya, galadriel);
* ringBearers.put(narya, gandalf);
* ringBearers.put(vilya, elrond);
* ringBearers.put(oneRing, frodo);
*
* // assertion will pass
* assertThat(ringBearers).containsValues(frodo, galadriel);
*
* // assertions will fail
* assertThat(ringBearers).containsValues(sauron, aragorn);
* assertThat(ringBearers).containsValues(sauron, frodo);
*
* @param values the values to look for in the actual map.
* @throws AssertionError if the actual map is {@code null}.
* @throws AssertionError if the actual map does not contain the given values.
*/
public S containsValues(@SuppressWarnings("unchecked") V... values) {
maps.assertContainsValues(info, actual, values);
return myself;
}
/**
* Verifies that the actual map does not contain the given value.
*
* Example :
*
Map<Ring, TolkienCharacter> ringBearers = new HashMap<>();
* ringBearers.put(nenya, galadriel);
* ringBearers.put(narya, gandalf);
* ringBearers.put(vilya, elrond);
* ringBearers.put(oneRing, frodo);
*
* // assertion will pass
* assertThat(ringBearers).doesNotContainValue(aragorn);
*
* // assertion will fail
* assertThat(ringBearers).doesNotContainValue(frodo);
*
* @param value the value that should not be in actual map.
* @throws AssertionError if the actual map is {@code null}.
* @throws AssertionError if the actual map contains the given value.
*/
public S doesNotContainValue(V value) {
maps.assertDoesNotContainValue(info, actual, value);
return myself;
}
/**
* Verifies that the actual map contains only the given entries and nothing else, in any order.
*
*
* Examples :
*
Map<Ring, TolkienCharacter> ringBearers = new HashMap<>();
* ringBearers.put(nenya, galadriel);
* ringBearers.put(narya, gandalf);
* ringBearers.put(vilya, elrond);
* ringBearers.put(oneRing, frodo);
*
* // assertion will pass
* assertThat(ringBearers).containsOnly(entry(oneRing, frodo), entry(nenya, galadriel), entry(narya, gandalf), entry(vilya, elrond));
*
* // assertion will fail
* assertThat(ringBearers).containsOnly(entry(oneRing, frodo), entry(nenya, galadriel));
*
* @param entries the entries that should be in the actual map.
* @throws AssertionError if the actual map is {@code null}.
* @throws NullPointerException if the given argument is {@code null}.
* @throws IllegalArgumentException if the given argument is an empty array.
* @throws AssertionError if the actual map does not contain the given entries, i.e. the actual map contains some or
* none of the given entries, or the actual map contains more entries than the given ones.
*/
public S containsOnly(@SuppressWarnings("unchecked") Map.Entry extends K, ? extends V>... entries) {
maps.assertContainsOnly(info, actual, entries);
return myself;
}
/**
* Verifies that the actual map contains only the given entries and nothing else, in order.
* This assertion should only be used with map that have a consistent iteration order (i.e. don't use it with
* {@link java.util.HashMap}, prefer {@link #containsOnly(com.fitbur.assertj.data.MapEntry...)} in that case).
*
* Example :
*
Map<Ring, TolkienCharacter> ringBearers = newLinkedHashMap(entry(oneRing, frodo),
* entry(nenya, galadriel),
* entry(narya, gandalf));
*
* // assertion will pass
* assertThat(ringBearers).containsExactly(entry(oneRing, frodo),
* entry(nenya, galadriel),
* entry(narya, gandalf));
*
* // assertion will fail as actual and expected order differ
* assertThat(ringBearers).containsExactly(entry(nenya, galadriel),
* entry(narya, gandalf),
* entry(oneRing, frodo));
*
* @param entries the given entries.
* @throws NullPointerException if the given entries array is {@code null}.
* @throws AssertionError if the actual map is {@code null}.
* @throws IllegalArgumentException if the given entries array is empty.
* @throws AssertionError if the actual map does not contain the given entries with same order, i.e. the actual map
* contains some or none of the given entries, or the actual map contains more entries than the given ones
* or entries are the same but the order is not.
*/
public S containsExactly(@SuppressWarnings("unchecked") Map.Entry extends K, ? extends V>... entries) {
maps.assertContainsExactly(info, actual, entries);
return myself;
}
/**
* Do not use this method.
*
* @deprecated Custom element Comparator is not supported for MapEntry comparison.
* @throws UnsupportedOperationException if this method is called.
*/
@Override
@Deprecated
public S usingElementComparator(Comparator super Map.Entry extends K, ? extends V>> customComparator) {
throw new UnsupportedOperationException("custom element Comparator is not supported for MapEntry comparison");
}
/**
* Do not use this method.
*
* @deprecated Custom element Comparator is not supported for MapEntry comparison.
* @throws UnsupportedOperationException if this method is called.
*/
@Override
@Deprecated
public S usingDefaultElementComparator() {
throw new UnsupportedOperationException("custom element Comparator is not supported for MapEntry comparison");
}
// override methods to avoid compilation error when chaining an AbstractAssert method with a AbstractMapAssert one
// this is pretty sad, a better fix for that would be welcome
@Override
public S as(String description, Object... args) {
return super.as(description, args);
}
@Override
public S as(Description description) {
return super.as(description);
}
@Override
public S describedAs(Description description) {
return super.describedAs(description);
}
@Override
public S describedAs(String description, Object... args) {
return super.describedAs(description, args);
}
@Override
public S doesNotHave(Condition super A> condition) {
return super.doesNotHave(condition);
}
@Override
public S doesNotHaveSameClassAs(Object other) {
return super.doesNotHaveSameClassAs(other);
}
@Override
public S has(Condition super A> condition) {
return super.has(condition);
}
@Override
public S hasSameClassAs(Object other) {
return super.hasSameClassAs(other);
}
@Override
public S hasToString(String expectedToString) {
return super.hasToString(expectedToString);
}
@Override
public S is(Condition super A> condition) {
return super.is(condition);
}
@Override
public S isEqualTo(Object expected) {
return super.isEqualTo(expected);
}
@Override
public S isExactlyInstanceOf(Class> type) {
return super.isExactlyInstanceOf(type);
}
@Override
public S isIn(Iterable> values) {
return super.isIn(values);
}
@Override
public S isIn(Object... values) {
return super.isIn(values);
}
@Override
public S isInstanceOf(Class> type) {
return super.isInstanceOf(type);
}
@Override
public S isInstanceOfAny(Class>... types) {
return super.isInstanceOfAny(types);
}
@Override
public S isNot(Condition super A> condition) {
return super.isNot(condition);
}
@Override
public S isNotEqualTo(Object other) {
return super.isNotEqualTo(other);
}
@Override
public S isNotExactlyInstanceOf(Class> type) {
return super.isNotExactlyInstanceOf(type);
}
@Override
public S isNotIn(Iterable> values) {
return super.isNotIn(values);
}
@Override
public S isNotIn(Object... values) {
return super.isNotIn(values);
}
@Override
public S isNotInstanceOf(Class> type) {
return super.isNotInstanceOf(type);
}
@Override
public S isNotInstanceOfAny(Class>... types) {
return super.isNotInstanceOfAny(types);
}
@Override
public S isNotOfAnyClassIn(Class>... types) {
return super.isNotOfAnyClassIn(types);
}
@Override
public S isNotNull() {
return super.isNotNull();
}
@Override
public S isNotSameAs(Object other) {
return super.isNotSameAs(other);
}
@Override
public S isOfAnyClassIn(Class>... types) {
return super.isOfAnyClassIn(types);
}
@Override
public S isSameAs(Object expected) {
return super.isSameAs(expected);
}
@Override
public S overridingErrorMessage(String newErrorMessage, Object... args) {
return super.overridingErrorMessage(newErrorMessage, args);
}
@Override
public S usingDefaultComparator() {
return super.usingDefaultComparator();
}
@Override
public S usingComparator(Comparator super A> customComparator) {
return super.usingComparator(customComparator);
}
@Override
public S withFailMessage(String newErrorMessage, Object... args) {
return super.withFailMessage(newErrorMessage, args);
}
@Override
public S withThreadDumpOnError() {
return super.withThreadDumpOnError();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy