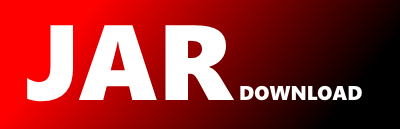
com.fitbur.assertj.api.AbstractObjectArrayAssert Maven / Gradle / Ivy
/**
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
* Copyright 2012-2016 the original author or authors.
*/
package com.fitbur.assertj.api;
import static java.util.Arrays.stream;
import static com.fitbur.assertj.api.filter.Filters.filter;
import static com.fitbur.assertj.extractor.Extractors.byName;
import static com.fitbur.assertj.extractor.Extractors.resultOf;
import static com.fitbur.assertj.util.Arrays.isArray;
import static com.fitbur.assertj.util.IterableUtil.toArray;
import static com.fitbur.assertj.util.Lists.newArrayList;
import static com.fitbur.assertj.util.Preconditions.checkNotNull;
import java.lang.reflect.Array;
import java.util.Arrays;
import java.util.Collection;
import java.util.Comparator;
import java.util.HashSet;
import java.util.List;
import java.util.function.Function;
import java.util.function.Predicate;
import com.fitbur.assertj.api.filter.FilterOperator;
import com.fitbur.assertj.api.filter.Filters;
import com.fitbur.assertj.api.iterable.Extractor;
import com.fitbur.assertj.condition.Not;
import com.fitbur.assertj.data.Index;
import com.fitbur.assertj.groups.FieldsOrPropertiesExtractor;
import com.fitbur.assertj.groups.Tuple;
import com.fitbur.assertj.internal.CommonErrors;
import com.fitbur.assertj.internal.ComparatorBasedComparisonStrategy;
import com.fitbur.assertj.internal.FieldByFieldComparator;
import com.fitbur.assertj.internal.IgnoringFieldsComparator;
import com.fitbur.assertj.internal.Iterables;
import com.fitbur.assertj.internal.ObjectArrayElementComparisonStrategy;
import com.fitbur.assertj.internal.ObjectArrays;
import com.fitbur.assertj.internal.Objects;
import com.fitbur.assertj.internal.OnFieldsComparator;
import com.fitbur.assertj.util.IterableUtil;
import com.fitbur.assertj.util.VisibleForTesting;
import com.fitbur.assertj.util.introspection.IntrospectionError;
/**
* Assertion methods for arrays of objects.
*
* To create an instance of this class, invoke {@link Assertions#assertThat(T[])}
.
*
*
* @param the type of elements of the "actual" value.
*
* @author Yvonne Wang
* @author Alex Ruiz
* @author Joel Costigliola
* @author Nicolas François
* @author Mikhail Mazursky
* @author Mateusz Haligowski
* @author Lovro Pandzic
*/
public abstract class AbstractObjectArrayAssert, T> extends
AbstractAssert implements IndexedObjectEnumerableAssert, T>,
ArraySortedAssert, T> {
@VisibleForTesting
ObjectArrays arrays = ObjectArrays.instance();
@VisibleForTesting
Iterables iterables = Iterables.instance();
protected AbstractObjectArrayAssert(T[] actual, Class> selfType) {
super(actual, selfType);
}
/**
* {@inheritDoc}
*
* @throws AssertionError {@inheritDoc}
*/
@Override
public void isNullOrEmpty() {
arrays.assertNullOrEmpty(info, actual);
}
/**
* {@inheritDoc}
*
* @throws AssertionError {@inheritDoc}
*/
@Override
public void isEmpty() {
arrays.assertEmpty(info, actual);
}
/**
* {@inheritDoc}
*
* @throws AssertionError {@inheritDoc}
*/
@Override
public S isNotEmpty() {
arrays.assertNotEmpty(info, actual);
return myself;
}
/**
* {@inheritDoc}
*
* @throws AssertionError {@inheritDoc}
*/
@Override
public S hasSize(int expected) {
arrays.assertHasSize(info, actual, expected);
return myself;
}
/**
* Verifies that the actual array has the same size as the given array.
*
* Parameter is declared as Object to accept both {@code Object[]} and primitive arrays (e.g. {@code int[]}).
*
* Example:
* int[] oneTwoThree = {1, 2, 3};
* int[] fourFiveSix = {4, 5, 6};
*
* // assertions will pass
* assertThat(oneTwoThree).hasSameSizeAs(fourFiveSix);
*
* @param array the array to compare size with actual group.
* @return {@code this} assertion object.
* @throws AssertionError if the actual group is {@code null}.
* @throws AssertionError if the array parameter is {@code null} or is not a true array.
* @throws AssertionError if actual group and given array don't have the same size.
*/
@Override
public S hasSameSizeAs(Object other) {
// same implementation as in AbstractArrayAssert, but can't inherit from it due to generics problem ...
arrays.assertHasSameSizeAs(info, actual, other);
return myself;
}
/**
* Verifies that the actual group has the same size as the given {@link Iterable}.
*
* Example:
* int[] oneTwoThree = {1, 2, 3};
* Iterable<Ring> elvesRings = newArrayList(vilya, nenya, narya);
*
* // assertions will pass
* assertThat(oneTwoThree).hasSameSizeAs(elvesRings);
*
* @param other the {@code Iterable} to compare size with actual group.
* @return {@code this} assertion object.
* @throws AssertionError if the actual group is {@code null}.
* @throws AssertionError if the other {@code Iterable} is {@code null}.
* @throws AssertionError if actual group and given {@code Iterable} don't have the same size.
*/
@Override
public S hasSameSizeAs(Iterable> other) {
arrays.assertHasSameSizeAs(info, actual, other);
return myself;
}
/**
* Verifies that the actual group contains the given values, in any order.
*
* Example :
*
String[] abc = {"a", "b", "c"};
*
* // assertions will pass
* assertThat(abc).contains("b", "a");
* assertThat(abc).contains("b", "a", "b");
*
* // assertion will fail
* assertThat(abc).contains("d");
*
* @param values the given values.
* @return {@code this} assertion object.
* @throws NullPointerException if the given argument is {@code null}.
* @throws IllegalArgumentException if the given argument is an empty array.
* @throws AssertionError if the actual group is {@code null}.
* @throws AssertionError if the actual group does not contain the given values.
*/
@Override
public S contains(@SuppressWarnings("unchecked") T... values) {
arrays.assertContains(info, actual, values);
return myself;
}
/**
* Verifies that the actual group contains only the given values and nothing else, in any order.
*
* Example :
*
String[] abc = {"a", "b", "c"};
*
* // assertion will pass
* assertThat(abc).containsOnly("c", "b", "a");
*
* // assertion will fail because "c" is missing
* assertThat(abc).containsOnly("a", "b");
*
* @param values the given values.
* @return {@code this} assertion object.
* @throws NullPointerException if the given argument is {@code null}.
* @throws IllegalArgumentException if the given argument is an empty array.
* @throws AssertionError if the actual group is {@code null}.
* @throws AssertionError if the actual group does not contain the given values, i.e. the actual group contains some
* or none of the given values, or the actual group contains more values than the given ones.
*/
@Override
public S containsOnly(@SuppressWarnings("unchecked") T... values) {
arrays.assertContainsOnly(info, actual, values);
return myself;
}
/**
* Same semantic as {@link #containsOnly(Object[])} : verifies that actual contains all elements of the given
* {@code Iterable} and nothing else, in any order.
*
* Example :
* Ring[] rings = {nenya, vilya};
*
* // assertion will pass
* assertThat(rings).containsOnlyElementsOf(newArrayList(nenya, vilya));
* assertThat(rings).containsOnlyElementsOf(newArrayList(nenya, nenya, vilya, vilya));
*
* // assertion will fail as actual does not contain narya
* assertThat(rings).containsOnlyElementsOf(newArrayList(nenya, vilya, narya));
* // assertion will fail as actual contains nenya
* assertThat(rings).containsOnlyElementsOf(newArrayList(vilya));
*
* @param iterable the given {@code Iterable} we will get elements from.
*/
@Override
public S containsOnlyElementsOf(Iterable extends T> iterable) {
return containsOnly(toArray(iterable));
}
/**
* An alias of {@link #containsOnlyElementsOf(Iterable)} : verifies that actual contains all elements of the
* given {@code Iterable} and nothing else, in any order.
*
* Example:
* Ring[] elvesRings = {vilya, nenya, narya};
*
* // assertions will pass:
* assertThat(elvesRings).hasSameElementsAs(newArrayList(nenya, narya, vilya));
* assertThat(elvesRings).hasSameElementsAs(newArrayList(nenya, narya, vilya, nenya));
*
* // assertions will fail:
* assertThat(elvesRings).hasSameElementsAs(newArrayList(nenya, narya));
* assertThat(elvesRings).hasSameElementsAs(newArrayList(nenya, narya, vilya, oneRing));
*
* @param iterable the {@code Iterable} whose elements we expect to be present
* @return this assertion object
* @throws AssertionError if the actual group is {@code null}
* @throws NullPointerException if the given {@code Iterable} is {@code null}
* @throws AssertionError if the actual {@code Iterable} does not have the same elements, in any order, as the given
* {@code Iterable}
*/
@Override
public S hasSameElementsAs(Iterable extends T> iterable) {
return containsOnlyElementsOf(iterable);
}
/**
* Verifies that the actual group contains the given values only once.
*
* Examples :
*
// array if a factory method to create arrays.
*
* // assertions will pass
* assertThat(array("winter", "is", "coming")).containsOnlyOnce("winter");
* assertThat(array("winter", "is", "coming")).containsOnlyOnce("coming", "winter");
*
* // assertions will fail
* assertThat(array("winter", "is", "coming")).containsOnlyOnce("Lannister");
* assertThat(array("Aria", "Stark", "daughter", "of", "Ned", "Stark")).containsOnlyOnce("Stark");
* assertThat(array("Aria", "Stark", "daughter", "of", "Ned", "Stark")).containsOnlyOnce("Stark", "Lannister", "Aria");
*
* @param values the given values.
* @return {@code this} assertion object.
* @throws NullPointerException if the given argument is {@code null}.
* @throws IllegalArgumentException if the given argument is an empty array.
* @throws AssertionError if the actual group is {@code null}.
* @throws AssertionError if the actual group does not contain the given values, i.e. the actual group contains some
* or none of the given values, or the actual group contains more than once these values.
*/
@Override
public S containsOnlyOnce(@SuppressWarnings("unchecked") T... values) {
arrays.assertContainsOnlyOnce(info, actual, values);
return myself;
}
/**
* Verifies that the actual array contains only the given values and nothing else, in order.
*
* Example :
*
Ring[] elvesRings = {vilya, nenya, narya};
*
* // assertion will pass
* assertThat(elvesRings).containsExactly(vilya, nenya, narya);
*
* // assertion will fail as actual and expected order differ
* assertThat(elvesRings).containsExactly(nenya, vilya, narya);
*
* @param values the given values.
* @return {@code this} assertion object.
* @throws NullPointerException if the given argument is {@code null}.
* @throws AssertionError if the actual group is {@code null}.
* @throws AssertionError if the actual group does not contain the given values with same order, i.e. the actual group
* contains some or none of the given values, or the actual group contains more values than the given ones
* or values are the same but the order is not.
*/
@Override
public S containsExactly(@SuppressWarnings("unchecked") T... values) {
arrays.assertContainsExactly(info, actual, values);
return myself;
}
/**
* Same as {@link #containsExactly(Object...)} but handle the {@link Iterable} to array conversion : verifies that
* actual contains all the elements of the given iterable and nothing else in the same order.
*
* Example :
* Ring[] elvesRings = {vilya, nenya, narya};
*
* // assertion will pass
* assertThat(elvesRings).containsExactlyElementsOf(newLinkedList(vilya, nenya, narya));
*
* // assertion will fail as actual and expected order differ
* assertThat(elvesRings).containsExactlyElementsOf(newLinkedList(nenya, vilya, narya));
*
* @param iterable the given {@code Iterable} we will get elements from.
*/
@Override
public S containsExactlyInAnyOrder(@SuppressWarnings("unchecked") T... values) {
arrays.assertContainsExactlyInAnyOrder(info, actual, values);
return myself;
}
/**
* Same as {@link #containsExactly(Object...)} but handle the {@link Iterable} to array conversion : verifies that
* actual contains all elements of the given {@code Iterable} and nothing else in the same order.
*
* Example :
* Ring[] elvesRings = {vilya, nenya, narya};
*
* // assertion will pass
* assertThat(elvesRings).containsExactlyElementsOf(newLinkedList(vilya, nenya, narya));
*
* // assertion will fail as actual and expected order differ
* assertThat(elvesRings).containsExactlyElementsOf(newLinkedList(nenya, vilya, narya));
*
* @param iterable the given {@code Iterable} we will get elements from.
*/
@Override
public S containsExactlyElementsOf(Iterable extends T> iterable) {
return containsExactly(toArray(iterable));
}
/**
* Verifies that the actual array contains the given sequence in the correct order and without extra value between the sequence values.
*
* Use {@link #containsSubsequence(Object...)} to allow values between the expected sequence values.
*
* Example:
*
Ring[] elvesRings = {vilya, nenya, narya};
*
* // assertion will pass
* assertThat(elvesRings).containsSequence(vilya, nenya);
* assertThat(elvesRings).containsSequence(nenya, narya);
*
* // assertions will fail, the elements order is correct but there is a value between them (nenya)
* assertThat(elvesRings).containsSequence(vilya, narya);
* assertThat(elvesRings).containsSequence(nenya, vilya);
*
* @param sequence the sequence of objects to look for.
* @return this assertion object.
* @throws AssertionError if the actual group is {@code null}.
* @throws AssertionError if the given array is {@code null}.
* @throws AssertionError if the actual group does not contain the given sequence.
*/
@Override
public S containsSequence(@SuppressWarnings("unchecked") T... sequence) {
arrays.assertContainsSequence(info, actual, sequence);
return myself;
}
/**
* Verifies that the actual array contains the given subsequence in the correct order (possibly with other values between them).
*
* Example:
*
Ring[] elvesRings = {vilya, nenya, narya};
*
* // assertions will pass
* assertThat(elvesRings).containsSubsequence(vilya, nenya);
* assertThat(elvesRings).containsSubsequence(vilya, narya);
*
* // assertion will fail
* assertThat(elvesRings).containsSubsequence(nenya, vilya);
*
* @param sequence the sequence of objects to look for.
* @return this assertion object.
* @throws AssertionError if the actual group is {@code null}.
* @throws AssertionError if the given array is {@code null}.
* @throws AssertionError if the actual group does not contain the given subsequence.
*/
@Override
public S containsSubsequence(@SuppressWarnings("unchecked") T... subsequence) {
arrays.assertContainsSubsequence(info, actual, subsequence);
return myself;
}
/**
* Verifies that the actual array contains the given object at the given index.
*
* Example:
* Ring[] elvesRings = {vilya, nenya, narya};
*
* // assertions will pass
* assertThat(elvesRings).contains(vilya, atIndex(0));
* assertThat(elvesRings).contains(nenya, atIndex(1));
* assertThat(elvesRings).contains(narya, atIndex(2));
*
* // assertions will fail
* assertThat(elvesRings).contains(vilya, atIndex(1));
* assertThat(elvesRings).contains(nenya, atIndex(2));
* assertThat(elvesRings).contains(narya, atIndex(0));
*
* @param value the object to look for.
* @param index the index where the object should be stored in the actual group.
* @return this assertion object.
* @throws AssertionError if the actual group is {@code null} or empty.
* @throws NullPointerException if the given {@code Index} is {@code null}.
* @throws IndexOutOfBoundsException if the value of the given {@code Index} is equal to or greater than the size of the actual
* group.
* @throws AssertionError if the actual group does not contain the given object at the given index.
*/
@Override
public S contains(T value, Index index) {
arrays.assertContains(info, actual, value, index);
return myself;
}
/**
* Verifies that the actual array does not contain the given object at the given index.
*
* Example:
* Ring[] elvesRings = {vilya, nenya, narya};
*
* // assertions will pass
* assertThat(elvesRings).contains(vilya, atIndex(1));
* assertThat(elvesRings).contains(nenya, atIndex(2));
* assertThat(elvesRings).contains(narya, atIndex(0));
*
* // assertions will fail
* assertThat(elvesRings).contains(vilya, atIndex(0));
* assertThat(elvesRings).contains(nenya, atIndex(1));
* assertThat(elvesRings).contains(narya, atIndex(2));
*
* @param value the object to look for.
* @param index the index where the object should not be stored in the actual group.
* @return this assertion object.
* @throws AssertionError if the actual group is {@code null}.
* @throws NullPointerException if the given {@code Index} is {@code null}.
* @throws AssertionError if the actual group contains the given object at the given index.
*/
public S doesNotContain(T value, Index index) {
arrays.assertDoesNotContain(info, actual, value, index);
return myself;
}
/**
* Verifies that the actual array does not contain the given values.
*
* Example :
*
String[] abc = {"a", "b", "c"};
*
* // assertion will pass
* assertThat(abc).doesNotContain("d", "e");
*
* // assertions will fail
* assertThat(abc).doesNotContain("a");
* assertThat(abc).doesNotContain("a", "b", "c");
* assertThat(abc).doesNotContain("a", "x");
*
* @param values the given values.
* @return {@code this} assertion object.
* @throws NullPointerException if the given argument is {@code null}.
* @throws IllegalArgumentException if the given argument is an empty array.
* @throws AssertionError if the actual group is {@code null}.
* @throws AssertionError if the actual group contains any of the given values.
*/
@Override
public S doesNotContain(@SuppressWarnings("unchecked") T... values) {
arrays.assertDoesNotContain(info, actual, values);
return myself;
}
/**
* Verifies that the actual array does not contain any elements of the given {@link Iterable} (i.e. none).
*
* Example:
* String[] abc = {"a", "b", "c"};
*
* // assertion will pass
* assertThat(actual).doesNotContainAnyElementsOf(newArrayList("d", "e"));
*
* // assertions will fail
* assertThat(actual).doesNotContainAnyElementsOf(newArrayList("a", "b"));
* assertThat(actual).doesNotContainAnyElementsOf(newArrayList("d", "e", "a"));
*
* @param iterable the {@link Iterable} whose elements must not be in the actual group.
* @return {@code this} assertion object.
* @throws NullPointerException if the given argument is {@code null}.
* @throws IllegalArgumentException if the given argument is an empty iterable.
* @throws AssertionError if the actual group is {@code null}.
* @throws AssertionError if the actual group contains some elements of the given {@link Iterable}.
*/
@Override
public S doesNotContainAnyElementsOf(Iterable extends T> iterable) {
arrays.assertDoesNotContainAnyElementsOf(info, actual, iterable);
return myself;
}
/**
* Verifies that the actual array does not contain duplicates.
*
* Example :
*
String[] abc = {"a", "b", "c"};
* String[] lotsOfAs = {"a", "a", "a"};
*
* // assertion will pass
* assertThat(abc).doesNotHaveDuplicates();
*
* // assertion will fail
* assertThat(lotsOfAs).doesNotHaveDuplicates();
*
* @return {@code this} assertion object.
* @throws AssertionError if the actual group is {@code null}.
* @throws AssertionError if the actual group contains duplicates.
*/
@Override
public S doesNotHaveDuplicates() {
arrays.assertDoesNotHaveDuplicates(info, actual);
return myself;
}
/**
* Verifies that the actual array starts with the given sequence of objects, without any other objects between them.
* Similar to {@link #containsSequence(Object...)}
, but it also verifies that the first element in the
* sequence is also the first element of the actual array.
*
* Example :
*
String[] abc = {"a", "b", "c"};
*
* // assertion will pass
* assertThat(abc).startsWith("a", "b");
*
* // assertion will fail
* assertThat(abc).startsWith("c");
*
* @param sequence the sequence of objects to look for.
* @return this assertion object.
* @throws NullPointerException if the given argument is {@code null}.
* @throws IllegalArgumentException if the given argument is an empty array.
* @throws AssertionError if the actual group is {@code null}.
* @throws AssertionError if the actual group does not start with the given sequence of objects.
*/
@Override
public S startsWith(@SuppressWarnings("unchecked") T... sequence) {
arrays.assertStartsWith(info, actual, sequence);
return myself;
}
/**
* Verifies that the actual array ends with the given sequence of objects, without any other objects between them.
* Similar to {@link #containsSequence(Object...)}
, but it also verifies that the last element in the
* sequence is also last element of the actual array.
*
* Example :
*
String[] abc = {"a", "b", "c"};
*
* // assertion will pass
* assertThat(abc).endsWith("b", "c");
*
* // assertion will fail
* assertThat(abc).endsWith("a");
*
* @param sequence the sequence of objects to look for.
* @return this assertion object.
* @throws NullPointerException if the given argument is {@code null}.
* @throws IllegalArgumentException if the given argument is an empty array.
* @throws AssertionError if the actual group is {@code null}.
* @throws AssertionError if the actual group does not end with the given sequence of objects.
*/
@Override
public S endsWith(@SuppressWarnings("unchecked") T... sequence) {
arrays.assertEndsWith(info, actual, sequence);
return myself;
}
/**
* Verifies that all elements of actual are present in the given {@code Iterable}.
*
* Example:
* Ring[] elvesRings = {vilya, nenya, narya};
* List<Ring> ringsOfPower = newArrayList(oneRing, vilya, nenya, narya, dwarfRing, manRing);
*
* // assertion will pass:
* assertThat(elvesRings).isSubsetOf(ringsOfPower);
*
* // assertion will fail:
* assertThat(elvesRings).isSubsetOf(newArrayList(nenya, narya));
*
* @param values the {@code Iterable} that should contain all actual elements.
* @return this assertion object.
* @throws AssertionError if the actual {@code Iterable} is {@code null}.
* @throws NullPointerException if the given {@code Iterable} is {@code null}.
* @throws AssertionError if the actual {@code Iterable} is not subset of set {@code Iterable}.
*/
@Override
public S isSubsetOf(Iterable extends T> values) {
arrays.assertIsSubsetOf(info, actual, values);
return myself;
}
/**
* Verifies that all elements of actual are present in the given values.
*
* Example:
* Ring[] elvesRings = {vilya, nenya, narya};
*
* // assertions will pass:
* assertThat(elvesRings).isSubsetOf(vilya, nenya, narya);
* assertThat(elvesRings).isSubsetOf(vilya, nenya, narya, dwarfRing);
*
* // assertions will fail:
* assertThat(elvesRings).isSubsetOf(vilya, nenya);
* assertThat(elvesRings).isSubsetOf(vilya, nenya, dwarfRing);
*
* @param values the values that should be used for checking the elements of actual.
* @return this assertion object.
* @throws AssertionError if the actual {@code Iterable} is {@code null}.
* @throws AssertionError if the actual {@code Iterable} is not subset of the given values.
*/
@Override
public S isSubsetOf(@SuppressWarnings("unchecked") T... values) {
arrays.assertIsSubsetOf(info, actual, Arrays.asList(values));
return myself;
}
/**
* Verifies that the actual array contains at least a null element.
*
* Example :
*
String[] abc = {"a", "b", "c"};
* String[] abNull = {"a", "b", null};
*
* // assertion will pass
* assertThat(abNull).containsNull();
*
* // assertion will fail
* assertThat(abc).containsNull();
*
* @return {@code this} assertion object.
* @throws AssertionError if the actual group is {@code null}.
* @throws AssertionError if the actual group does not contain a null element.
*/
@Override
public S containsNull() {
arrays.assertContainsNull(info, actual);
return myself;
}
/**
* Verifies that the actual array does not contain null elements.
*
* Example :
*
String[] abc = {"a", "b", "c"};
* String[] abNull = {"a", "b", null};
*
* // assertion will pass
* assertThat(abc).doesNotContainNull();
*
* // assertion will fail
* assertThat(abNull).doesNotContainNull();
*
* @return {@code this} assertion object.
* @throws AssertionError if the actual group is {@code null}.
* @throws AssertionError if the actual group contains a null element.
*/
@Override
public S doesNotContainNull() {
arrays.assertDoesNotContainNull(info, actual);
return myself;
}
/**
* Verifies that each element value satisfies the given condition
*
* Example :
*
String[] abc = {"a", "b", "c"};
* String[] abcc = {"a", "b", "cc"};
*
* Condition<String> singleCharacterString
* = new Condition<>(s -> s.length() == 1, "single character String");
*
* // assertion will pass
* assertThat(abc).are(singleCharacterString);
*
* // assertion will fail
* assertThat(abcc).are(singleCharacterString);
*
* @param condition the given condition.
* @return {@code this} object.
* @throws NullPointerException if the given condition is {@code null}.
* @throws AssertionError if an element cannot be cast to T.
* @throws AssertionError if one or more elements don't satisfy the given condition.
*/
@Override
public S are(Condition super T> condition) {
arrays.assertAre(info, actual, condition);
return myself;
}
/**
* Verifies that each element value does not satisfy the given condition
*
* Example :
*
String[] abc = {"a", "b", "c"};
* String[] abcc = {"a", "b", "cc"};
*
* Condition<String> moreThanOneCharacter =
* = new Condition<>(s -> s.length() > 1, "more than one character");
*
* // assertion will pass
* assertThat(abc).areNot(moreThanOneCharacter);
*
* // assertion will fail
* assertThat(abcc).areNot(moreThanOneCharacter);
*
* @param condition the given condition.
* @return {@code this} object.
* @throws NullPointerException if the given condition is {@code null}.
* @throws AssertionError if an element cannot be cast to T.
* @throws AssertionError if one or more elements satisfy the given condition.
*/
@Override
public S areNot(Condition super T> condition) {
arrays.assertAreNot(info, actual, condition);
return myself;
}
/**
* Verifies that all elements satisfy the given condition.
*
* Example :
*
String[] abc = {"a", "b", "c"};
* String[] abcc = {"a", "b", "cc"};
*
* Condition<String> onlyOneCharacter =
* = new Condition<>(s -> s.length() == 1, "only one character");
*
* // assertion will pass
* assertThat(abc).have(onlyOneCharacter);
*
* // assertion will fail
* assertThat(abcc).have(onlyOneCharacter);
*
* @param condition the given condition.
* @return {@code this} object.
* @throws NullPointerException if the given condition is {@code null}.
* @throws AssertionError if an element cannot be cast to T.
* @throws AssertionError if one or more elements do not satisfy the given condition.
*/
@Override
public S have(Condition super T> condition) {
arrays.assertHave(info, actual, condition);
return myself;
}
/**
* Verifies that all elements don't satisfy the given condition.
*
* Example :
*
String[] abc = {"a", "b", "c"};
* String[] abcc = {"a", "b", "cc"};
*
* Condition<String> moreThanOneCharacter =
* = new Condition<>(s -> s.length() > 1, "more than one character");
*
* // assertion will pass
* assertThat(abc).doNotHave(moreThanOneCharacter);
*
* // assertion will fail
* assertThat(abcc).doNotHave(moreThanOneCharacter);
*
* @param condition the given condition.
* @return {@code this} object.
* @throws NullPointerException if the given condition is {@code null}.
* @throws AssertionError if an element cannot be cast to T.
* @throws AssertionError if one or more elements satisfy the given condition.
*/
@Override
public S doNotHave(Condition super T> condition) {
arrays.assertDoNotHave(info, actual, condition);
return myself;
}
/**
* Verifies that there is at least n elements in the actual group satisfying the given condition.
*
* Example :
*
int[] oneTwoThree = {1, 2, 3};
*
* Condition<Integer> oddNumber = new Condition<>(value % 2 == 1, "odd number");
*
* // assertion will pass
* oneTwoThree.areAtLeast(2, oddNumber);
*
* // assertion will fail
* oneTwoThree.areAtLeast(3, oddNumber);
*
* @param n the minimum number of times the condition should be verified.
* @param condition the given condition.
* @return {@code this} object.
* @throws NullPointerException if the given condition is {@code null}.
* @throws AssertionError if an element can not be cast to T.
* @throws AssertionError if the number of elements satisfying the given condition is < n.
*/
@Override
public S areAtLeast(int times, Condition super T> condition) {
arrays.assertAreAtLeast(info, actual, times, condition);
return myself;
}
/**
* Verifies that there is at least one element in the actual array satisfying the given condition.
*
* This method is an alias for {@code areAtLeast(1, condition)}.
*
* Example:
* // jedi is a Condition<String>
* assertThat(new String[]{"Luke", "Solo", "Leia"}).areAtLeastOne(jedi);
*
* @see #haveAtLeast(int, Condition)
*/
@Override
public S areAtLeastOne(Condition super T> condition) {
areAtLeast(1, condition);
return myself;
}
/**
* Verifies that there is at most n elements in the actual group satisfying the given condition.
*
* Example :
*
int[] oneTwoThree = {1, 2, 3};
*
* Condition<Integer> oddNumber = new Condition<>(value % 2 == 1, "odd number");
*
* // assertions will pass
* oneTwoThree.areAtMost(2, oddNumber);
* oneTwoThree.areAtMost(3, oddNumber);
*
* // assertion will fail
* oneTwoThree.areAtMost(1, oddNumber);
*
* @param n the number of times the condition should be at most verified.
* @param condition the given condition.
* @return {@code this} object.
* @throws NullPointerException if the given condition is {@code null}.
* @throws AssertionError if an element cannot be cast to T.
* @throws AssertionError if the number of elements satisfying the given condition is > n.
*/
@Override
public S areAtMost(int times, Condition super T> condition) {
arrays.assertAreAtMost(info, actual, times, condition);
return myself;
}
/**
* Verifies that there is exactly n elements in the actual group satisfying the given condition.
*
* Example :
*
int[] oneTwoThree = {1, 2, 3};
*
* Condition<Integer> oddNumber = new Condition<>(value % 2 == 1, "odd number");
*
* // assertion will pass
* oneTwoThree.areExactly(2, oddNumber);
*
* // assertions will fail
* oneTwoThree.areExactly(1, oddNumber);
* oneTwoThree.areExactly(3, oddNumber);
*
* @param n the exact number of times the condition should be verified.
* @param condition the given condition.
* @return {@code this} object.
* @throws NullPointerException if the given condition is {@code null}.
* @throws AssertionError if an element cannot be cast to T.
* @throws AssertionError if the number of elements satisfying the given condition is ≠ n.
*/
@Override
public S areExactly(int times, Condition super T> condition) {
arrays.assertAreExactly(info, actual, times, condition);
return myself;
}
/**
* Verifies that there is at least one element in the actual group satisfying the given condition.
*
* This method is an alias for {@code haveAtLeast(1, condition)}.
*
* Example:
* BasketBallPlayer[] bullsPlayers = {butler, rose};
*
* // potentialMvp is a Condition<BasketBallPlayer>
* assertThat(bullsPlayers).haveAtLeastOne(potentialMvp);
*
* @see #haveAtLeast(int, Condition)
*/
@Override
public S haveAtLeastOne(Condition super T> condition) {
return haveAtLeast(1, condition);
}
/**
* Verifies that there is at least n elements in the actual group satisfying the given condition.
*
* Example :
*
int[] oneTwoThree = {1, 2, 3};
*
* Condition<Integer> oddNumber = new Condition<>(value % 2 == 1, "odd number");
*
* // assertion will pass
* oneTwoThree.haveAtLeast(2, oddNumber);
*
* // assertion will fail
* oneTwoThree.haveAtLeast(3, oddNumber);
*
* This method is an alias for {@link #areAtLeast(int, Condition)}.
*/
@Override
public S haveAtLeast(int times, Condition super T> condition) {
arrays.assertHaveAtLeast(info, actual, times, condition);
return myself;
}
/**
* Verifies that there is at most n elements in the actual group satisfying the given condition.
*
* Example :
*
int[] oneTwoThree = {1, 2, 3};
*
* Condition<Integer> oddNumber = new Condition<>(value % 2 == 1, "odd number");
*
* // assertions will pass
* oneTwoThree.haveAtMost(2, oddNumber);
* oneTwoThree.haveAtMost(3, oddNumber);
*
* // assertion will fail
* oneTwoThree.haveAtMost(1, oddNumber);
*
* This method is an alias {@link #areAtMost(int, Condition)}.
*/
@Override
public S haveAtMost(int times, Condition super T> condition) {
arrays.assertHaveAtMost(info, actual, times, condition);
return myself;
}
/**
* Verifies that there is exactly n elements in the actual group satisfying the given condition.
*
* Example :
*
int[] oneTwoThree = {1, 2, 3};
*
* Condition<Integer> oddNumber = new Condition<>(value % 2 == 1, "odd number");
*
* // assertion will pass
* oneTwoThree.haveExactly(2, oddNumber);
*
* // assertions will fail
* oneTwoThree.haveExactly(1, oddNumber);
* oneTwoThree.haveExactly(3, oddNumber);
*
* This method is an alias {@link #areExactly(int, Condition)}.
*/
@Override
public S haveExactly(int times, Condition super T> condition) {
arrays.assertHaveExactly(info, actual, times, condition);
return myself;
}
/** {@inheritDoc} */
@Override
public S hasAtLeastOneElementOfType(Class> type) {
arrays.assertHasAtLeastOneElementOfType(info, actual, type);
return myself;
}
/** {@inheritDoc} */
@Override
public S hasOnlyElementsOfType(Class> type) {
arrays.assertHasOnlyElementsOfType(info, actual, type);
return myself;
}
/** {@inheritDoc} */
@Override
public S isSorted() {
arrays.assertIsSorted(info, actual);
return myself;
}
/** {@inheritDoc} */
@Override
public S isSortedAccordingTo(Comparator super T> comparator) {
arrays.assertIsSortedAccordingToComparator(info, actual, comparator);
return myself;
}
/**
* Verifies that the actual array contains all the elements of given {@code Iterable}, in any order.
*
* Example :
*
String[] abc = {"a", "b", "c"};
* String[] bc = {"b", "c"};
*
* // assertion will pass
* assertThat(abc).containsAll(bc);
*
* @param iterable the given {@code Iterable} we will get elements from.
* @return {@code this} assertion object.
* @throws NullPointerException if the given argument is {@code null}.
* @throws AssertionError if the actual group is {@code null}.
* @throws AssertionError if the actual group does not contain all the elements of given {@code Iterable}.
*/
@Override
public S containsAll(Iterable extends T> iterable) {
arrays.assertContainsAll(info, actual, iterable);
return myself;
}
/**
* Use given custom comparator instead of relying on actual type A equals
method to compare group
* elements for
* incoming assertion checks.
*
* Custom comparator is bound to assertion instance, meaning that if a new assertion is created, it will use default
* comparison strategy.
*
* Examples :
*
// compares invoices by payee
* assertThat(invoiceArray).usingComparator(invoicePayeeComparator).isEqualTo(expectedinvoiceArray).
*
* // compares invoices by date, doesNotHaveDuplicates and contains both use the given invoice date comparator
* assertThat(invoiceArray).usingComparator(invoiceDateComparator).doesNotHaveDuplicates().contains(may2010Invoice)
*
* // as assertThat(invoiceArray) creates a new assertion, it falls back to standard comparison strategy
* // based on Invoice's equal method to compare invoiceArray elements to lowestInvoice.
* assertThat(invoiceArray).contains(lowestInvoice).
*
* // standard comparison : the fellowshipOfTheRing includes Gandalf but not Sauron (believe me) ...
* assertThat(fellowshipOfTheRing).contains(gandalf)
* .doesNotContain(sauron);
*
* // ... but if we compare only races, Sauron is in fellowshipOfTheRing because he's a Maia like Gandalf.
* assertThat(fellowshipOfTheRing).usingElementComparator(raceComparator)
* .contains(sauron);
*
* @param customComparator the comparator to use for incoming assertion checks.
* @throws NullPointerException if the given comparator is {@code null}.
* @return {@code this} assertion object.
*/
public S usingElementComparator(Comparator super T> elementComparator) {
this.arrays = new ObjectArrays(new ComparatorBasedComparisonStrategy(elementComparator));
// to have the same semantics on base assertions like isEqualTo, we need to use an iterable comparator comparing
// elements with elementComparator parameter
objects = new Objects(new ObjectArrayElementComparisonStrategy<>(elementComparator));
return myself;
}
/** {@inheritDoc} */
@Override
public S usingDefaultElementComparator() {
this.arrays = ObjectArrays.instance();
return myself;
}
/**
* Use field/property by field/property comparison (including inherited fields/properties) instead of relying on
* actual type A equals
method to compare group elements for incoming assertion checks. Private fields
* are included but this can be disabled using {@link Assertions#setAllowExtractingPrivateFields(boolean)}.
*
* This can be handy if equals
method of the objects to compare does not suit you.
*
* Note that the comparison is not recursive, if one of the fields/properties is an Object, it will be compared
* to the other field/property using its equals
method.
*
* Example:
* TolkienCharacter frodo = new TolkienCharacter("Frodo", 33, HOBBIT);
* TolkienCharacter frodoClone = new TolkienCharacter("Frodo", 33, HOBBIT);
*
* // Fail if equals has not been overridden in TolkienCharacter as equals default implementation only compares references
* assertThat(array(frodo)).contains(frodoClone);
*
* // frodo and frodoClone are equals when doing a field by field comparison.
* assertThat(array(frodo)).usingFieldByFieldElementComparator().contains(frodoClone);
*
* @return {@code this} assertion object.
*/
public S usingFieldByFieldElementComparator() {
return usingElementComparator(new FieldByFieldComparator());
}
/**
* Use field/property by field/property comparison on the given fields/properties only (including inherited
* fields/properties)instead of relying on actual type A equals
method to compare group elements for
* incoming assertion checks. Private fields are included but this can be disabled using
* {@link Assertions#setAllowExtractingPrivateFields(boolean)}.
*
* This can be handy if equals
method of the objects to compare does not suit you.
*
* Note that the comparison is not recursive, if one of the fields/properties is an Object, it will be compared
* to the other field/property using its equals
method.
*
* Example:
* TolkienCharacter frodo = new TolkienCharacter("Frodo", 33, HOBBIT);
* TolkienCharacter sam = new TolkienCharacter("Sam", 38, HOBBIT);
*
* // frodo and sam both are hobbits, so they are equals when comparing only race
* assertThat(array(frodo)).usingElementComparatorOnFields("race").contains(sam); // OK
*
* // ... but not when comparing both name and race
* assertThat(array(frodo)).usingElementComparatorOnFields("name", "race").contains(sam); // FAIL
*
* @return {@code this} assertion object.
*/
public S usingElementComparatorOnFields(String... fields) {
return usingElementComparator(new OnFieldsComparator(fields));
}
/**
* Use field/property by field/property on all fields/properties except the given ones (including inherited
* fields/properties)instead of relying on actual type A equals
method to compare group elements for
* incoming assertion checks. Private fields are included but this can be disabled using
* {@link Assertions#setAllowExtractingPrivateFields(boolean)}.
*
* This can be handy if equals
method of the objects to compare does not suit you.
*
* Note that the comparison is not recursive, if one of the fields/properties is an Object, it will be compared
* to the other field/property using its equals
method.
*
* Example:
* TolkienCharacter frodo = new TolkienCharacter("Frodo", 33, HOBBIT);
* TolkienCharacter sam = new TolkienCharacter("Sam", 38, HOBBIT);
*
* // frodo and sam both are hobbits, so they are equals when comparing only race (i.e. ignoring all other fields)
* assertThat(array(frodo)).usingElementComparatorIgnoringFields("name", "age").contains(sam); // OK
*
* // ... but not when comparing both name and race
* assertThat(array(frodo)).usingElementComparatorIgnoringFields("age").contains(sam); // FAIL
*
* @return {@code this} assertion object.
*/
public S usingElementComparatorIgnoringFields(String... fields) {
return usingElementComparator(new IgnoringFieldsComparator(fields));
}
/**
* Extract the values of given field or property from the array's elements under test into a new array, this new array
* becoming the array under test.
*
* It allows you to test a field/property of the array's elements instead of testing the elements themselves, it can
* be sometimes much less work !
*
* Let's take an example to make things clearer :
*
// Build a array of TolkienCharacter, a TolkienCharacter has a name (String) and a Race (a class)
* // they can be public field or properties, both works when extracting their values.
* TolkienCharacter[] fellowshipOfTheRing = new TolkienCharacter[] {
* new TolkienCharacter("Frodo", 33, HOBBIT),
* new TolkienCharacter("Sam", 38, HOBBIT),
* new TolkienCharacter("Gandalf", 2020, MAIA),
* new TolkienCharacter("Legolas", 1000, ELF),
* new TolkienCharacter("Pippin", 28, HOBBIT),
* new TolkienCharacter("Gimli", 139, DWARF),
* new TolkienCharacter("Aragorn", 87, MAN,
* new TolkienCharacter("Boromir", 37, MAN)
* };
*
* // let's verify the names of TolkienCharacter in fellowshipOfTheRing :
*
* assertThat(fellowshipOfTheRing).extracting("name")
* .contains("Boromir", "Gandalf", "Frodo")
* .doesNotContain("Sauron", "Elrond");
*
* // you can also extract nested field/property like the name of Race :
*
* assertThat(fellowshipOfTheRing).extracting("race.name")
* .contains("Hobbit", "Elf")
* .doesNotContain("Orc");
*
* A property with the given name is looked for first, if it does not exist then a field with the given name
* is looked for.
*
* Note that the order of extracted field/property values is consistent with the array order.
*
* @param fieldOrProperty the field/property to extract from the array under test
* @return a new assertion object whose object under test is the array of extracted field/property values.
* @throws IntrospectionError if no field or property exists with the given name
*/
public ObjectArrayAssert