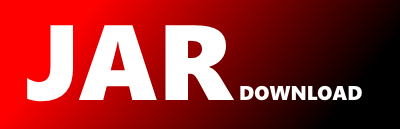
com.fitbur.assertj.api.AbstractOptionalAssert Maven / Gradle / Ivy
/**
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
* Copyright 2012-2016 the original author or authors.
*/
package com.fitbur.assertj.api;
import com.fitbur.assertj.internal.ComparatorBasedComparisonStrategy;
import com.fitbur.assertj.internal.ComparisonStrategy;
import com.fitbur.assertj.internal.FieldByFieldComparator;
import com.fitbur.assertj.internal.StandardComparisonStrategy;
import java.util.Comparator;
import java.util.Optional;
import java.util.function.Consumer;
import static com.fitbur.assertj.error.OptionalShouldBeEmpty.shouldBeEmpty;
import static com.fitbur.assertj.error.OptionalShouldBePresent.shouldBePresent;
import static com.fitbur.assertj.error.OptionalShouldContain.shouldContain;
import static com.fitbur.assertj.error.OptionalShouldContain.shouldContainSame;
import static com.fitbur.assertj.error.OptionalShouldContainInstanceOf.shouldContainInstanceOf;
/**
* Assertions for {@link java.util.Optional}.
*
* @param type of the value contained in the {@link java.util.Optional}.
*
* @author Jean-Christophe Gay
* @author Nicolai Parlog
* @author Grzegorz Piwowarek
*/
public abstract class AbstractOptionalAssert, T> extends
AbstractAssert> {
private ComparisonStrategy optionalValueComparisonStrategy;
protected AbstractOptionalAssert(Optional actual, Class> selfType) {
super(actual, selfType);
this.optionalValueComparisonStrategy = StandardComparisonStrategy.instance();
}
/**
* Verifies that there is a value present in the actual {@link java.util.Optional}.
*
* Assertion will pass :
* assertThat(Optional.of("something")).isPresent();
*
* Assertion will fail :
* assertThat(Optional.empty()).isPresent();
*
* @return this assertion object.
*/
public S isPresent() {
isNotNull();
if (!actual.isPresent()) throwAssertionError(shouldBePresent(actual));
return myself;
}
/**
* Verifies that there is a value present in the actual {@link java.util.Optional}, it's an alias of {@link #isPresent()}.
*
* Assertion will pass :
* assertThat(Optional.of("something")).isNotEmpty();
*
* Assertion will fail :
* assertThat(Optional.empty()).isNotEmpty();
*
* @return this assertion object.
*/
public S isNotEmpty() {
return isPresent();
}
/**
* Verifies that the actual {@link java.util.Optional} is empty.
*
* Assertion will pass :
* assertThat(Optional.empty()).isEmpty();
*
* Assertion will fail :
* assertThat(Optional.of("something")).isEmpty();
*
* @return this assertion object.
*/
public S isEmpty() {
isNotNull();
if (actual.isPresent()) throwAssertionError(shouldBeEmpty(actual));
return myself;
}
/**
* Verifies that the actual {@link java.util.Optional} is empty (alias of {@link #isEmpty()}).
*
* Assertion will pass :
* assertThat(Optional.empty()).isNotPresent();
*
* Assertion will fail :
* assertThat(Optional.of("something")).isNotPresent();
*
* @return this assertion object.
*/
public S isNotPresent() {
return isEmpty();
}
/**
* Verifies that the actual {@link java.util.Optional} contains the given value (alias of {@link #hasValue(Object)}).
*
* Assertion will pass :
* assertThat(Optional.of("something")).contains("something");
* assertThat(Optional.of(10)).contains(10);
*
* Assertion will fail :
* assertThat(Optional.of("something")).contains("something else");
* assertThat(Optional.of(20)).contains(10);
*
* @param expectedValue the expected value inside the {@link java.util.Optional}.
* @return this assertion object.
*/
public S contains(T expectedValue) {
isNotNull();
checkNotNull(expectedValue);
if (!actual.isPresent()) throwAssertionError(shouldContain(expectedValue));
if (!optionalValueComparisonStrategy.areEqual(actual.get(), expectedValue))
throwAssertionError(shouldContain(actual, expectedValue));
return myself;
}
/**
* Verifies that the actual {@link java.util.Optional} contains a value and gives this value to the given
* {@link java.util.function.Consumer} for further assertions. Should be used as a way of deeper asserting on the
* containing object, as further requirement(s) for the value.
*
* Assertions will pass :
* // one requirement
* assertThat(Optional.of(10)).hasValueSatisfying(i -> { assertThat(i).isGreaterThan(9); });
*
* // multiple requirements
* assertThat(Optional.of(someString)).hasValueSatisfying(s -> {
* assertThat(s).isEqualTo("something");
* assertThat(s).startsWith("some");
* assertThat(s).endsWith("thing");
* });
*
* Assertions will fail :
* assertThat(Optional.of("something")).hasValueSatisfying(s -> {
* assertThat(s).isEqualTo("something else");
* });
*
* // fail because optional is empty, there is no value to perform assertion on
* assertThat(Optional.empty()).hasValueSatisfying(o -> {});
*
* @param requirement to further assert on the object contained inside the {@link java.util.Optional}.
* @return this assertion object.
*/
public S hasValueSatisfying(Consumer requirement) {
isNotNull();
if (!actual.isPresent()) throwAssertionError(shouldBePresent(actual));
requirement.accept(actual.get());
return myself;
}
/**
* Verifies that the actual {@link java.util.Optional} contains the given value (alias of {@link #contains(Object)}).
*
* Assertion will pass :
* assertThat(Optional.of("something")).hasValue("something");
* assertThat(Optional.of(10)).contains(10);
*
* Assertion will fail :
* assertThat(Optional.of("something")).hasValue("something else");
* assertThat(Optional.of(20)).contains(10);
*
* @param expectedValue the expected value inside the {@link java.util.Optional}.
* @return this assertion object.
*/
public S hasValue(T expectedValue) {
return contains(expectedValue);
}
/**
* Verifies that the actual {@link Optional} contains a value that is an instance of the argument.
*
* Assertions will pass:
*
* assertThat(Optional.of("something")).containsInstanceOf(String.class)
* .containsInstanceOf(Object.class);
*
* assertThat(Optional.of(10)).containsInstanceOf(Integer.class);
*
* Assertion will fail:
*
* assertThat(Optional.of("something")).containsInstanceOf(Integer.class);
*
* @param clazz the expected class of the value inside the {@link Optional}.
* @return this assertion object.
*/
public S containsInstanceOf(Class> clazz) {
isNotNull();
if (!actual.isPresent()) throwAssertionError(shouldBePresent(actual));
if (!clazz.isInstance(actual.get())) throwAssertionError(shouldContainInstanceOf(actual, clazz));
return myself;
}
/**
* Use field/property by field/property comparison (including inherited fields/properties) instead of relying on
* actual type A equals
method to compare the {@link Optional} value's object for incoming assertion
* checks. Private fields are included but this can be disabled using
* {@link Assertions#setAllowExtractingPrivateFields(boolean)}.
*
* This can be handy if equals
method of the {@link Optional} value's object to compare does not suit
* you.
*
* Note that the comparison is not recursive, if one of the fields/properties is an Object, it will be
* compared to the other field/property using its equals
method.
*
* Example:
*
* TolkienCharacter frodo = new TolkienCharacter("Frodo", 33, HOBBIT);
* TolkienCharacter frodoClone = new TolkienCharacter("Frodo", 33, HOBBIT);
*
* // Fail if equals has not been overridden in TolkienCharacter as equals default implementation only compares references
* assertThat(Optional.of(frodo)).contains(frodoClone);
*
* // frodo and frodoClone are equals when doing a field by field comparison.
* assertThat(Optional.of(frodo)).usingFieldByFieldValueComparator().contains(frodoClone);
*
* @return {@code this} assertion object.
*/
public S usingFieldByFieldValueComparator() {
return usingValueComparator(new FieldByFieldComparator());
}
/**
* Use given custom comparator instead of relying on actual type A equals
method to compare the
* {@link Optional} value's object for incoming assertion checks.
*
* Custom comparator is bound to assertion instance, meaning that if a new assertion is created, it will use default
* comparison strategy.
*
* Examples :
*
*
TolkienCharacter frodo = new TolkienCharacter("Frodo", 33, HOBBIT);
* TolkienCharacter frodoClone = new TolkienCharacter("Frodo", 33, HOBBIT);
*
* // Fail if equals has not been overridden in TolkienCharacter as equals default implementation only compares references
* assertThat(Optional.of(frodo)).contains(frodoClone);
*
* // frodo and frodoClone are equals when doing a field by field comparison.
* assertThat(Optional.of(frodo)).usingValueComparator(new FieldByFieldComparator()).contains(frodoClone);
*
* @param customComparator the comparator to use for incoming assertion checks.
* @throws NullPointerException if the given comparator is {@code null}.
* @return {@code this} assertion object.
*/
public S usingValueComparator(Comparator super T> customComparator) {
optionalValueComparisonStrategy = new ComparatorBasedComparisonStrategy(customComparator);
return myself;
}
/**
* Revert to standard comparison for incoming assertion {@link Optional} value checks.
*
* This method should be used to disable a custom comparison strategy set by calling
* {@link #usingValueComparator(Comparator)}.
*
* @return {@code this} assertion object.
*/
public S usingDefaultValueComparator() {
// fall back to default strategy to compare actual with other objects.
optionalValueComparisonStrategy = StandardComparisonStrategy.instance();
return myself;
}
/**
* Verifies that the actual {@link java.util.Optional} contains the instance given as an argument (i.e. it must be the
* same instance).
*
* Assertion will pass :
*
* String someString = "something";
* assertThat(Optional.of(someString)).containsSame(someString);
*
* // Java will create the same 'Integer' instance when boxing small ints
* assertThat(Optional.of(10)).containsSame(10);
*
* Assertion will fail :
*
* // not even equal:
* assertThat(Optional.of("something")).containsSame("something else");
* assertThat(Optional.of(20)).containsSame(10);
*
* // equal but not the same:
* assertThat(Optional.of(new String("something"))).containsSame(new String("something"));
* assertThat(Optional.of(new Integer(10))).containsSame(new Integer(10));
*
* @param expectedValue the expected value inside the {@link java.util.Optional}.
* @return this assertion object.
*/
public S containsSame(T expectedValue) {
isNotNull();
checkNotNull(expectedValue);
if (!actual.isPresent()) throwAssertionError(shouldContain(expectedValue));
if (actual.get() != expectedValue) throwAssertionError(shouldContainSame(actual, expectedValue));
return myself;
}
private void checkNotNull(T expectedValue) {
if (expectedValue == null) throw new IllegalArgumentException("The expected value should not be .");
}
}