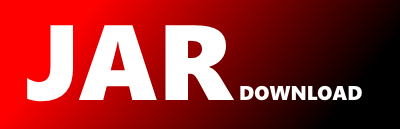
com.fitbur.assertj.api.AbstractOptionalDoubleAssert Maven / Gradle / Ivy
/**
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
* Copyright 2012-2016 the original author or authors.
*/
package com.fitbur.assertj.api;
import com.fitbur.assertj.data.Offset;
import com.fitbur.assertj.internal.Doubles;
import com.fitbur.assertj.util.VisibleForTesting;
import java.util.OptionalDouble;
import static java.lang.Math.abs;
import static com.fitbur.assertj.error.OptionalDoubleShouldHaveValueCloseTo.shouldHaveValueCloseTo;
import static com.fitbur.assertj.error.OptionalShouldBeEmpty.shouldBeEmpty;
import static com.fitbur.assertj.error.OptionalShouldBePresent.shouldBePresent;
import static com.fitbur.assertj.error.OptionalShouldContain.shouldContain;
/**
* Assertions for {@link java.util.OptionalDouble}.
*
* @author Jean-Christophe Gay
* @author Alexander Bischof
* @author Grzegorz Piwowarek
*/
public abstract class AbstractOptionalDoubleAssert> extends
AbstractAssert {
@VisibleForTesting
Doubles doubles = Doubles.instance();
protected AbstractOptionalDoubleAssert(OptionalDouble actual, Class> selfType) {
super(actual, selfType);
}
/**
* Verifies that there is a value present in the actual {@link java.util.OptionalDouble}.
*
* Assertion will pass :
*
*
*
assertThat(OptionalDouble.of(10.0)).isPresent();
*
* Assertion will fail :
*
*
*
assertThat(OptionalDouble.empty()).isPresent();
*
* @return this assertion object.
* @throws java.lang.AssertionError if actual value is empty.
* @throws java.lang.AssertionError if actual is null.
*/
public S isPresent() {
isNotNull();
if (!actual.isPresent()) throwAssertionError(shouldBePresent(actual));
return myself;
}
/**
* Verifies that the actual {@link java.util.Optional} is empty (alias of {@link #isEmpty()}).
*
* Assertion will pass :
* assertThat(OptionalDouble.empty()).isNotPresent();
*
* Assertion will fail :
* assertThat(OptionalDouble.of(10.0)).isNotPresent();
*
* @return this assertion object.
*/
public S isNotPresent() {
return isEmpty();
}
/**
* Verifies that the actual {@link java.util.OptionalDouble} is empty.
*
* Assertion will pass :
*
*
*
assertThat(OptionalDouble.empty()).isEmpty();
*
* Assertion will fail :
*
*
*
assertThat(OptionalDouble.of(10.0)).isEmpty();
*
* @return this assertion object.
* @throws java.lang.AssertionError if actual value is present.
* @throws java.lang.AssertionError if actual is null.
*/
public S isEmpty() {
isNotNull();
if (actual.isPresent()) throwAssertionError(shouldBeEmpty(actual));
return myself;
}
/**
* Verifies that there is a value present in the actual {@link java.util.OptionalDouble}, it's an alias of {@link #isPresent()}.
*
* Assertion will pass :
*
*
*
assertThat(OptionalDouble.of(10.0)).isNotEmpty();
*
* Assertion will fail :
*
*
*
assertThat(OptionalDouble.empty()).isNotEmpty();
*
* @return this assertion object.
* @throws java.lang.AssertionError if actual value is empty.
* @throws java.lang.AssertionError if actual is null.
*/
public S isNotEmpty() {
return isPresent();
}
/**
* Verifies that the actual {@link java.util.OptionalDouble} has the value in argument.
*
* Assertion will pass :
*
*
*
assertThat(OptionalDouble.of(8.0)).hasValue(8.0);
* assertThat(OptionalDouble.of(8.0)).hasValue(Double.valueOf(8.0));
* assertThat(OptionalDouble.of(Double.NaN)).hasValue(Double.NaN);
*
* Assertion will fail :
*
*
*
assertThat(OptionalDouble.empty()).hasValue(8.0);
* assertThat(OptionalDouble.of(7)).hasValue(8.0);
*
* @param expectedValue the expected value inside the {@link java.util.OptionalDouble}.
* @return this assertion object.
* @throws java.lang.AssertionError if actual value is empty.
* @throws java.lang.AssertionError if actual is null.
* @throws java.lang.AssertionError if actual has not the value as expected.
*/
public S hasValue(double expectedValue) {
isNotNull();
if (!actual.isPresent()) throwAssertionError(shouldContain(expectedValue));
if (expectedValue != actual.getAsDouble()) throwAssertionError(shouldContain(actual, expectedValue));
return myself;
}
/**
* Verifies that the actual {@link java.util.OptionalDouble} has the value close to the argument.
*
* Assertion will pass :
*
*
*
assertThat(OptionalDouble.of(8)).hasValueCloseTo(8.0, within(0d));
* assertThat(OptionalDouble.of(8)).hasValueCloseTo(8.0, within(1d));
* assertThat(OptionalDouble.of(7)).hasValueCloseTo(8.0, within(1d));
*
* Assertion will fail :
*
*
*
assertThat(OptionalDouble.empty()).hasValueCloseTo(8.0, within(1d));
* assertThat(OptionalDouble.of(7)).hasValueCloseTo(1.0, within(1d));
* assertThat(OptionalDouble.of(7)).hasValueCloseTo(1.0, null);
* assertThat(OptionalDouble.of(7)).hasValueCloseTo(1.0, within(-1d));
*
* @param expectedValue the expected value inside the {@link java.util.OptionalDouble}.
* @param offset the given positive offset.
* @return this assertion object.
* @throws java.lang.AssertionError if actual value is empty.
* @throws java.lang.AssertionError if actual is null.
* @throws java.lang.AssertionError if actual has not the value as expected.
* @throws java.lang.NullPointerException if offset is null
* @throws java.lang.IllegalArgumentException if offset is not positive.
*/
public S hasValueCloseTo(Double expectedValue, Offset offset) {
isNotNull();
if (!actual.isPresent()) throwAssertionError(shouldHaveValueCloseTo(expectedValue));
// Reuses doubles functionality, catches poyential assertion error and throw correct one
try {
doubles.assertIsCloseTo(info, actual.getAsDouble(), expectedValue, offset);
} catch (AssertionError assertionError) {
throwAssertionError(shouldHaveValueCloseTo(actual, expectedValue, offset, abs(expectedValue - actual.getAsDouble())));
}
return myself;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy