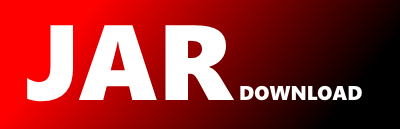
com.fitbur.assertj.api.AbstractShortAssert Maven / Gradle / Ivy
/**
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
* Copyright 2012-2016 the original author or authors.
*/
package com.fitbur.assertj.api;
import java.util.Comparator;
import com.fitbur.assertj.data.Offset;
import com.fitbur.assertj.data.Percentage;
import com.fitbur.assertj.internal.ComparatorBasedComparisonStrategy;
import com.fitbur.assertj.internal.Shorts;
import com.fitbur.assertj.util.VisibleForTesting;
/**
* Base class for all implementations of assertions for {@link Short}s.
*
* @param the "self" type of this assertion class. Please read "Emulating 'self types' using Java Generics to simplify fluent API implementation"
* for more details.
*
* @author Yvonne Wang
* @author David DIDIER
* @author Ansgar Konermann
* @author Alex Ruiz
* @author Mikhail Mazursky
* @author Nicolas François
*/
public abstract class AbstractShortAssert> extends AbstractComparableAssert
implements NumberAssert {
@VisibleForTesting
Shorts shorts = Shorts.instance();
protected AbstractShortAssert(Short actual, Class> selfType) {
super(actual, selfType);
}
/**
* Verifies that the actual value is equal to the given one.
*
* Example:
*
// assertion will pass:
* assertThat(Short.valueOf("1")).isEqualTo((short) 1);
*
* // assertion will fail:
* assertThat(Short.valueOf("-1")).isEqualTo((short) 1);
*
*
* @param expected the given value to compare the actual value to.
* @return {@code this} assertion object.
* @throws AssertionError if the actual value is {@code null}.
* @throws AssertionError if the actual value is not equal to the given one.
*/
public S isEqualTo(short expected) {
shorts.assertEqual(info, actual, expected);
return myself;
}
/**
* Verifies that the actual value is not equal to the given one.
*
* Example:
*
// assertion will pass:
* assertThat(Short.valueOf(("-1")).isNotEqualTo((short) 1);
*
* // assertion will fail:
* assertThat(Short.valueOf("1")).isNotEqualTo((short) 1);
*
*
* @param other the given value to compare the actual value to.
* @return {@code this} assertion object.
* @throws AssertionError if the actual value is {@code null}.
* @throws AssertionError if the actual value is equal to the given one.
*/
public S isNotEqualTo(short other) {
shorts.assertNotEqual(info, actual, other);
return myself;
}
/** {@inheritDoc} */
@Override
public S isZero() {
shorts.assertIsZero(info, actual);
return myself;
}
/** {@inheritDoc} */
@Override
public S isNotZero() {
shorts.assertIsNotZero(info, actual);
return myself;
}
/** {@inheritDoc} */
@Override
public S isPositive() {
shorts.assertIsPositive(info, actual);
return myself;
}
/** {@inheritDoc} */
@Override
public S isNegative() {
shorts.assertIsNegative(info, actual);
return myself;
}
/** {@inheritDoc} */
@Override
public S isNotNegative() {
shorts.assertIsNotNegative(info, actual);
return myself;
}
/** {@inheritDoc} */
@Override
public S isNotPositive() {
shorts.assertIsNotPositive(info, actual);
return myself;
}
/**
* Verifies that the actual value is less than the given one.
*
* Example:
*
// assertion will pass:
* assertThat(Short.valueOf("1")).isLessThan((short) 2);
*
* // assertion will fail:
* assertThat(Short.valueOf("1")).isLessThan((short) 0);
* assertThat(Short.valueOf("1")).isLessThan((short) 1);
*
*
* @param other the given value to compare the actual value to.
* @return {@code this} assertion object.
* @throws AssertionError if the actual value is {@code null}.
* @throws AssertionError if the actual value is equal to or greater than the given one.
*/
public S isLessThan(short other) {
shorts.assertLessThan(info, actual, other);
return myself;
}
/**
* Verifies that the actual value is less than or equal to the given one.
*
* Example:
*
// assertion will pass:
* assertThat(Short.valueOf("1")).isLessThanOrEqualTo((short) 1);
*
* // assertion will fail:
* assertThat(Short.valueOf("1")).isLessThanOrEqualTo((short) 0);
*
*
* @param other the given value to compare the actual value to.
* @return {@code this} assertion object.
* @throws AssertionError if the actual value is {@code null}.
* @throws AssertionError if the actual value is greater than the given one.
*/
public S isLessThanOrEqualTo(short other) {
shorts.assertLessThanOrEqualTo(info, actual, other);
return myself;
}
/**
* Verifies that the actual value is greater than the given one.
*
* Example:
*
// assertion will pass:
* assertThat(Short.valueOf("1")).isGreaterThan((short) 0);
*
* // assertions will fail:
* assertThat(Short.valueOf("0")).isGreaterThan((short) 1);
* assertThat(Short.valueOf("0")).isGreaterThan((short) 0);
*
*
* @param other the given value to compare the actual value to.
* @return {@code this} assertion object.
* @throws AssertionError if the actual value is {@code null}.
* @throws AssertionError if the actual value is equal to or less than the given one.
*/
public S isGreaterThan(short other) {
shorts.assertGreaterThan(info, actual, other);
return myself;
}
/**
* Verifies that the actual value is greater than or equal to the given one.
*
* Example:
*
// assertion will pass:
* assertThat(Short.valueOf("1")).isGreaterThanOrEqualTo((short) 1);
*
* // assertion will fail:
* assertThat(Short.valueOf("0")).isGreaterThanOrEqualTo((short) 1);
*
*
* @param other the given value to compare the actual value to.
* @return {@code this} assertion object.
* @throws AssertionError if the actual value is {@code null}.
* @throws AssertionError if the actual value is less than the given one.
*/
public S isGreaterThanOrEqualTo(short other) {
shorts.assertGreaterThanOrEqualTo(info, actual, other);
return myself;
}
/** {@inheritDoc} */
@Override
public S isBetween(Short start, Short end) {
shorts.assertIsBetween(info, actual, start, end);
return myself;
}
/** {@inheritDoc} */
@Override
public S isStrictlyBetween(Short start, Short end) {
shorts.assertIsStrictlyBetween(info, actual, start, end);
return myself;
}
/**
* Verifies that the actual short is close to the given one within the given offset.
* If difference is equal to offset value, assertion is considered valid.
*
* Example:
*
// assertions will pass:
* assertThat((short)5).isCloseTo((short)7, within((short)3));
*
* // if difference is exactly equals to the offset, it's ok
* assertThat((short)5).isCloseTo((short)7, within((short)2));
*
* // assertion will fail
* assertThat((short)5).isCloseTo((short)7, within((short)1));
*
* @param expected the given short to compare the actual value to.
* @param offset the given positive offset.
* @return {@code this} assertion object.
* @throws NullPointerException if the given offset is {@code null}.
* @throws AssertionError if the actual value is not equal to the given one.
*/
public S isCloseTo(short expected, Offset offset) {
shorts.assertIsCloseTo(info, actual, expected, offset);
return myself;
}
/**
* Verifies that the actual short is close to the given one within the given offset.
* If difference is equal to offset value, assertion is considered valid.
*
* Example:
*
// assertions will pass:
* assertThat((short)5).isCloseTo(Short.valueOf(7), within((short)3));
*
* // if difference is exactly equals to the offset, it's ok
* assertThat((short)5).isCloseTo(Short.valueOf(7), within((short)2));
*
* // assertion will fail
* assertThat((short)5).isCloseTo(Short.valueOf(7), within((short)1));
*
* @param expected the given short to compare the actual value to.
* @param offset the given positive offset.
* @return {@code this} assertion object.
* @throws NullPointerException if the given offset is {@code null}.
* @throws AssertionError if the actual value is not equal to the given one.
*/
@Override
public S isCloseTo(Short expected, Offset offset) {
shorts.assertIsCloseTo(info, actual, expected, offset);
return myself;
}
/**
* Verifies that the actual number is close to the given one within the given percentage.
* If difference is equal to the percentage value, assertion is considered valid.
*
* Example with short:
*
// assertions will pass:
* assertThat((short)11).isCloseTo(Short.valueOf(10), withinPercentage((short)20));
*
* // if difference is exactly equals to the computed offset (1), it's ok
* assertThat((short)11).isCloseTo(Short.valueOf(10), withinPercentage((short)10));
*
* // assertion will fail
* assertThat((short)11).isCloseTo(Short.valueOf(10), withinPercentage((short)5));
*
* @param expected the given number to compare the actual value to.
* @param percentage the given positive percentage.
* @return {@code this} assertion object.
* @throws NullPointerException if the given offset is {@code null}.
* @throws NullPointerException if the expected number is {@code null}.
* @throws AssertionError if the actual value is not equal to the given one.
*/
@Override
public S isCloseTo(Short expected, Percentage percentage) {
shorts.assertIsCloseToPercentage(info, actual, expected, percentage);
return myself;
}
/**
* Verifies that the actual number is close to the given one within the given percentage.
* If difference is equal to the percentage value, assertion is considered valid.
*
* Example with short:
*
// assertions will pass:
* assertThat((short)11).isCloseTo((short)10, withinPercentage((short)20));
*
* // if difference is exactly equals to the computed offset (1), it's ok
* assertThat((short)11).isCloseTo((short)10, withinPercentage((short)10));
*
* // assertion will fail
* assertThat((short)11).isCloseTo((short)10, withinPercentage((short)5));
*
* @param expected the given number to compare the actual value to.
* @param percentage the given positive percentage.
* @return {@code this} assertion object.
* @throws NullPointerException if the given offset is {@code null}.
* @throws NullPointerException if the expected number is {@code null}.
* @throws AssertionError if the actual value is not equal to the given one.
*/
public S isCloseTo(short expected, Percentage percentage) {
shorts.assertIsCloseToPercentage(info, actual, expected, percentage);
return myself;
}
@Override
public S usingComparator(Comparator super Short> customComparator) {
super.usingComparator(customComparator);
shorts = new Shorts(new ComparatorBasedComparisonStrategy(customComparator));
return myself;
}
@Override
public S usingDefaultComparator() {
super.usingDefaultComparator();
shorts = Shorts.instance();
return myself;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy