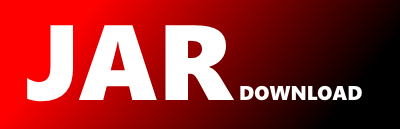
com.fitbur.assertj.api.AbstractUrlAssert Maven / Gradle / Ivy
/**
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
* Copyright 2012-2016 the original author or authors.
*/
package com.fitbur.assertj.api;
import java.net.URL;
import com.fitbur.assertj.internal.Urls;
import com.fitbur.assertj.util.VisibleForTesting;
/**
* Base class for all implementations of assertions for {@link URL}s.
*
* @param the "self" type of this assertion class.
* @see java.net.URL
*/
public abstract class AbstractUrlAssert> extends AbstractAssert {
@VisibleForTesting
protected Urls urls = Urls.instance();
protected AbstractUrlAssert(final URL actual, final Class> selfType) {
super(actual, selfType);
}
/**
* Verifies that the actual {@code URL} has the expected protocol.
*
* Examples:
*
// This assertion succeeds:
* assertThat(new URL("ftp://helloworld.org")).hasProtocol("ftp");
*
* // These assertion fails:
* assertThat(new URL("http://helloworld.org")).hasProtocol("ftp");
*
* @param expected the expected protocol of the actual {@code URL}.
* @return {@code this} assertion object.
* @throws AssertionError if the actual protocol is not equal to the expected protocol.
*/
public S hasProtocol(String expected) {
urls.assertHasProtocol(info, actual, expected);
return myself;
}
/**
* Verifies that the actual {@code URL} has the expected path (which must not be null).
*
* Examples:
*
// These assertions succeed:
* assertThat(new URL("http://helloworld.org/pages")).hasPath("/pages");
* assertThat(new URL("http://www.helloworld.org")).hasPath("");
* // or preferably:
* assertThat(new URL("http://www.helloworld.org")).hasNoPath();
*
* // this assertion fails:
* assertThat(new URL("http://helloworld.org/pickme")).hasPath("/pages/");
*
* // this assertion throws an IllegalArgumentException:
* assertThat(new URL("http://helloworld.org/pages")).hasPath(null);
*
* @param expected the expected path of the actual {@code URL}.
* @return {@code this} assertion object.
* @throws AssertionError if the actual URL path is not equal to the expected path.
* @throws IllegalArgumentException if given path is null.
*/
public S hasPath(String expected) {
urls.assertHasPath(info, actual, expected);
return myself;
}
/**
* Verifies that the actual {@code URL} has no path.
*
* Examples:
*
// This assertion succeeds:
* assertThat(new URL("http://www.helloworld.org")).hasNoPath();
*
* // this assertion fails:
* assertThat(new URL("http://helloworld.org/france")).hasNoPath();
*
* @return {@code this} assertion object.
* @throws AssertionError if the actual has a path.
*/
public S hasNoPath() {
urls.assertHasPath(info, actual, "");
return myself;
}
/**
* Verifies that the actual {@code URL} has the expected port.
*
* Examples:
*
// These assertions succeed:
* assertThat(new URL("http://helloworld.org:8080")).hasPort(8080);
*
* // These assertions fail:
* assertThat(new URL("http://helloworld.org:8080")).hasPort(9876);
* assertThat(new URL("http://helloworld.org")).hasPort(8080);
*
* @param expected the expected port of the actual {@code URL}.
* @return {@code this} assertion object.
* @throws AssertionError if the actual port is not equal to the expected port.
*/
public S hasPort(int expected) {
urls.assertHasPort(info, actual, expected);
return myself;
}
/**
* Verifies that the actual {@code URL} has no port.
*
* Examples:
*
// This assertion succeeds:
* assertThat(new URL("http://helloworld.org")).hasNoPort();
*
* // This assertion fails:
* assertThat(new URL("http://helloworld.org:8080")).hasNoPort();
*
* @return {@code this} assertion object.
* @throws AssertionError if the actual has a port.
*/
public S hasNoPort() {
urls.assertHasPort(info, actual, -1);
return myself;
}
/**
* Verifies that the actual {@code URL} has the expected host.
*
* Examples:
*
// These assertions succeed:
* assertThat(new URL("http://helloworld.org/pages")).hasHost("helloworld.org");
* assertThat(new URL("http://helloworld.org:8080")).hasHost("helloworld.org");
*
* // These assertions fail:
* assertThat(new URL("http://www.helloworld.org")).hasHost("helloworld.org");
* assertThat(new URL("http://www.helloworld.org:8080")).hasHost("helloworld.org");
*
* @param expected the expected host of the actual {@code URL}.
* @return {@code this} assertion object.
* @throws AssertionError if the actual host is not equal to the expected host.
*/
public S hasHost(String expected) {
urls.assertHasHost(info, actual, expected);
return myself;
}
/**
* Verifies that the actual {@code URL} has the expected authority.
*
* Examples:
*
// These assertions succeed:
* assertThat(new URL("http://helloworld.org")).hasAuthority("helloworld.org");
* assertThat(new URL("http://helloworld.org:8080")).hasAuthority("helloworld.org:8080");
* assertThat(new URL("http://www.helloworld.org:8080/news")).hasAuthority("www.helloworld.org:8080");
*
* // These assertions fail:
* assertThat(new URL("http://www.helloworld.org:8080")).hasAuthority("www.helloworld.org");
* assertThat(new URL("http://www.helloworld.org")).hasAuthority("www.helloworld.org:8080");
*
* @param expected the expected authority of the actual {@code URL}.
* @return {@code this} assertion object.
* @throws AssertionError if the actual authority is not equal to the expected authority.
*/
public S hasAuthority(String expected) {
urls.assertHasAuthority(info, actual, expected);
return myself;
}
/**
* Verifies that the actual {@code URL} has the expected query.
*
* Examples:
*
// This assertion succeeds:
* assertThat(new URL("http://www.helloworld.org/index.html?type=test")).hasQuery("type=test");
*
* // These assertions fail:
* assertThat(new URL("http://www.helloworld.org/index.html?type=test")).hasQuery("type=hello");
* assertThat(new URL("http://www.helloworld.org/index.html")).hasQuery("type=hello");
*
* @param expected the expected query of the actual {@code URL}.
* @return {@code this} assertion object.
* @throws AssertionError if the actual query is not equal to the expected query.
*/
public S hasQuery(String expected) {
urls.assertHasQuery(info, actual, expected);
return myself;
}
/**
* Verifies that the actual {@code URL} has no query.
*
* Examples:
*
// This assertion succeeds:
* assertThat(new URL("http://www.helloworld.org/index.html")).hasNoQuery();
*
* // These assertions fail:
* assertThat(new URL("http://www.helloworld.org/index.html?type=test")).hasNoQuery();
*
* @return {@code this} assertion object.
* @throws AssertionError if the actual has a query.
*/
public S hasNoQuery() {
urls.assertHasQuery(info, actual, null);
return myself;
}
/**
* Verifies that the actual {@code URL} has the expected anchor.
*
* Examples:
*
// This assertion succeeds:
* assertThat(new URL("http://www.helloworld.org/news.html#sport")).hasAnchor("sport");
*
* // These assertions fail:
* assertThat(new URL("http://www.helloworld.org/news.html#sport")).hasAnchor("war");
* assertThat(new URL("http://www.helloworld.org/news.html")).hasAnchor("sport");
*
* @param expected the expected anchor of the actual {@code URL}.
* @return {@code this} assertion object.
* @throws AssertionError if the actual anchor is not equal to the expected anchor.
*/
public S hasAnchor(String expected) {
urls.assertHasAnchor(info, actual, expected);
return myself;
}
/**
* Verifies that the actual {@code URL} has no anchor.
*
* Examples:
*
// This assertion succeeds:
* assertThat(new URL("http://www.helloworld.org/news.html")).hasNoAnchor();
*
* // These assertions fail:
* assertThat(new URL("http://www.helloworld.org/news.html#sport")).hasNoAnchor();
*
* @return {@code this} assertion object.
* @throws AssertionError if the actual has a anchor.
*/
public S hasNoAnchor() {
urls.assertHasAnchor(info, actual, null);
return myself;
}
/**
* Verifies that the actual {@code URL} has the expected userinfo.
*
* Examples:
*
// These assertions succeed:
* assertThat(new URL("http://test:[email protected]/index.html")).hasUserInfo("test:pass");
* assertThat(new URL("http://[email protected]/index.html")).hasUserInfo("test");
* assertThat(new URL("http://:[email protected]/index.html")).hasUserInfo(":pass");
*
* // These assertions fail:
* assertThat(new URL("http://test:[email protected]/index.html")).hasUserInfo("test:fail");
* assertThat(new URL("http://www.helloworld.org/index.html")).hasUserInfo("test:pass");
*
* @param expected the expected userinfo of the actual {@code URL}.
* @return {@code this} assertion object.
* @throws AssertionError if the actual userinfo is not equal to the expected userinfo.
*/
public S hasUserInfo(String expected) {
urls.assertHasUserInfo(info, actual, expected);
return myself;
}
/**
* Verifies that the actual {@code URL} has no userinfo.
*
* Examples:
*
// This assertion succeeds:
* assertThat(new URL("http://www.helloworld.org/index.html")).hasNoUserInfo();
*
* // This assertion fails:
* assertThat(new URL("http://test:[email protected]/index.html")).hasNoUserInfo();
*
* @return {@code this} assertion object.
* @throws AssertionError if the actual has some userinfo.
*/
public S hasNoUserInfo() {
urls.assertHasUserInfo(info, actual, null);
return myself;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy