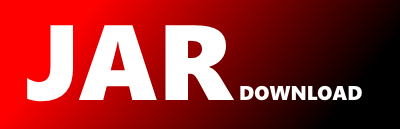
com.fitbur.assertj.api.Assert Maven / Gradle / Ivy
/**
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
* Copyright 2012-2016 the original author or authors.
*/
package com.fitbur.assertj.api;
import java.util.Comparator;
/**
* Base contract of all assertion objects: the minimum functionality that any assertion object should provide.
*
* @param the "self" type of this assertion class. Please read "Emulating
* 'self types' using Java Generics to simplify fluent API implementation" for more details.
* @param the type of the "actual" value.
*
* @author Yvonne Wang
* @author Alex Ruiz
* @author Nicolas François
* @author Mikhail Mazursky
*/
public interface Assert, A> extends Descriptable, ExtensionPoints {
/**
* Verifies that the actual value is equal to the given one.
*
* Example:
*
// assertions will pass
* assertThat("abc").isEqualTo("abc");
* assertThat(new HashMap<String, Integer>()).isEqualTo(new HashMap<String, Integer>());
*
* // assertions will fail
* assertThat("abc").isEqualTo("123");
* assertThat(new ArrayList<String>()).isEqualTo(1);
*
* @param expected the given value to compare the actual value to.
* @return {@code this} assertion object.
* @throws AssertionError if the actual value is not equal to the given one.
*/
S isEqualTo(Object expected);
/**
* Verifies that the actual value is not equal to the given one.
*
* Example:
*
// assertions will pass
* assertThat("abc").isNotEqualTo("123");
* assertThat(new ArrayList<String>()).isNotEqualTo(1);
*
* // assertions will fail
* assertThat("abc").isNotEqualTo("abc");
* assertThat(new HashMap<String, Integer>()).isNotEqualTo(new HashMap<String, Integer>());
*
* @param other the given value to compare the actual value to.
* @return {@code this} assertion object.
* @throws AssertionError if the actual value is equal to the given one.
*/
S isNotEqualTo(Object other);
/**
* Verifies that the actual value is {@code null}.
*
* Example:
*
String value = null;
* // assertion will pass
* assertThat(value).isNull();
*
* // assertions will fail
* assertThat("abc").isNull();
* assertThat(new HashMap<String, Integer>()).isNull();
*
* @throws AssertionError if the actual value is not {@code null}.
*/
void isNull();
/**
* Verifies that the actual value is not {@code null}.
*
* Example:
*
// assertion will pass
* assertThat("abc").isNotNull();
* assertThat(new HashMap<String, Integer>()).isNotNull();
*
* // assertion will fail
* String value = null;
* assertThat(value).isNotNull();
*
* @return {@code this} assertion object.
* @throws AssertionError if the actual value is {@code null}.
*/
S isNotNull();
/**
* Verifies that the actual value is the same as the given one, ie using == comparison.
*
* Example:
*
// Name is a class with first and last fields, two Names are equals if both first and last are equals.
* Name tyrion = new Name("Tyrion", "Lannister");
* Name alias = tyrion;
* Name clone = new Name("Tyrion", "Lannister");
*
* // assertions succeed:
* assertThat(tyrion).isSameAs(alias)
* .isEqualTo(clone);
*
* // assertion fails:
* assertThat(tyrion).isSameAs(clone);
*
* @param expected the given value to compare the actual value to.
* @return {@code this} assertion object.
* @throws AssertionError if the actual value is not the same as the given one.
*/
S isSameAs(Object expected);
/**
* Verifies that the actual value is not the same as the given one, ie using == comparison.
*
* Example:
*
// Name is a class with first and last fields, two Names are equals if both first and last are equals.
* Name tyrion = new Name("Tyrion", "Lannister");
* Name alias = tyrion;
* Name clone = new Name("Tyrion", "Lannister");
*
* // assertions succeed:
* assertThat(clone).isNotSameAs(tyrion)
* .isEqualTo(tyrion);
*
* // assertion fails:
* assertThat(alias).isNotSameAs(tyrion);
*
* @param other the given value to compare the actual value to.
* @return {@code this} assertion object.
* @throws AssertionError if the actual value is the same as the given one.
*/
S isNotSameAs(Object other);
/**
* Verifies that the actual value is present in the given array of values.
*
* Example:
*
Ring[] elvesRings = new Ring[] { vilya, nenya, narya };
*
* // assertion will pass:
* assertThat(nenya).isIn(elvesRings);
*
* // assertion will fail:
* assertThat(oneRing).isIn(elvesRings);
*
* @param values the given array to search the actual value in.
* @return {@code this} assertion object.
* @throws NullPointerException if the given array is {@code null}.
* @throws IllegalArgumentException if the given array is empty.
* @throws AssertionError if the actual value is not present in the given array.
*/
S isIn(Object... values);
/**
* Verifies that the actual value is not present in the given array of values.
*
* Example:
*
Ring[] elvesRings = new Ring[] { vilya, nenya, narya };
*
* // assertion will pass:
* assertThat(oneRing).isNotIn(elvesRings);
*
* // assertion will fail:
* assertThat(nenya).isNotIn(elvesRings);
*
* @param values the given array to search the actual value in.
* @return {@code this} assertion object.
* @throws NullPointerException if the given array is {@code null}.
* @throws IllegalArgumentException if the given array is empty.
* @throws AssertionError if the actual value is present in the given array.
*/
S isNotIn(Object... values);
/**
* Verifies that the actual value is present in the given values.
*
* Example:
*
Iterable<Ring> elvesRings = newArrayList(vilya, nenya, narya);
*
* // assertion will pass:
* assertThat(nenya).isIn(elvesRings);
*
* // assertion will fail:
* assertThat(oneRing).isIn(elvesRings);
*
* @param values the given iterable to search the actual value in.
* @return {@code this} assertion object.
* @throws NullPointerException if the given collection is {@code null}.
* @throws IllegalArgumentException if the given collection is empty.
* @throws AssertionError if the actual value is not present in the given collection.
*/
S isIn(Iterable> values);
/**
* Verifies that the actual value is not present in the given values.
*
* Example:
*
Iterable<Ring> elvesRings = newArrayList(vilya, nenya, narya);
*
* // assertion will pass:
* assertThat(oneRing).isNotIn(elvesRings);
*
* // assertion will fail:
* assertThat(nenya).isNotIn(elvesRings);
*
* @param values the given iterable to search the actual value in.
* @return {@code this} assertion object.
* @throws NullPointerException if the given collection is {@code null}.
* @throws IllegalArgumentException if the given collection is empty.
* @throws AssertionError if the actual value is present in the given collection.
*/
S isNotIn(Iterable> values);
/**
* Use given custom comparator instead of relying on actual type A equals method for incoming assertion checks.
*
* Custom comparator is bound to assertion instance, meaning that if a new assertion is created, it will use default
* comparison strategy.
*
* Examples :
*
// frodo and sam are instances of Character with Hobbit race (obviously :).
* // raceComparator implements Comparator<Character>
* assertThat(frodo).usingComparator(raceComparator).isEqualTo(sam);
*
* @param customComparator the comparator to use for incoming assertion checks.
* @throws NullPointerException if the given comparator is {@code null}.
* @return {@code this} assertion object.
*/
S usingComparator(Comparator super A> customComparator);
/**
* Revert to standard comparison for incoming assertion checks.
*
* This method should be used to disable a custom comparison strategy set by calling
* {@link #usingComparator(Comparator)}.
*
* @return {@code this} assertion object.
*/
S usingDefaultComparator();
/**
* Verifies that the actual value is an instance of the given type.
*
* Example:
*
// assertions will pass
* assertThat("abc").isInstanceOf(String.class);
* assertThat(new HashMap<String, Integer>()).isInstanceOf(HashMap.class);
* assertThat(new HashMap<String, Integer>()).isInstanceOf(Map.class);
*
* // assertions will fail
* assertThat(1).isInstanceOf(String.class);
* assertThat(new ArrayList<String>()).isInstanceOf(LinkedList.class);
*
* @param type the type to check the actual value against.
* @return this assertion object.
* @throws NullPointerException if the given type is {@code null}.
* @throws AssertionError if the actual value is {@code null}.
* @throws AssertionError if the actual value is not an instance of the given type.
*/
S isInstanceOf(Class> type);
/**
* Verifies that the actual value is an instance of any of the given types.
*
* Example:
*
// assertions will pass
* assertThat("abc").isInstanceOfAny(String.class, Integer.class);
* assertThat(new ArrayList<String>()).isInstanceOfAny(LinkedList.class, ArrayList.class);
* assertThat(new HashMap<String, Integer>()).isInstanceOfAny(TreeMap.class, Map.class);
*
* // assertions will fail
* assertThat(1).isInstanceOfAny(Double.class, Float.class);
* assertThat(new ArrayList<String>()).isInstanceOfAny(LinkedList.class, Vector.class);
*
* @param types the types to check the actual value against.
* @return this assertion object.
* @throws AssertionError if the actual value is {@code null}.
* @throws AssertionError if the actual value is not an instance of any of the given types.
* @throws NullPointerException if the given array of types is {@code null}.
* @throws NullPointerException if the given array of types contains {@code null}s.
*/
S isInstanceOfAny(Class>... types);
/**
* Verifies that the actual value is not an instance of the given type.
*
* Example:
*
// assertions will pass
* assertThat(1).isNotInstanceOf(Double.class);
* assertThat(new ArrayList<String>()).isNotInstanceOf(LinkedList.class);
*
* // assertions will fail
* assertThat("abc").isNotInstanceOf(String.class);
* assertThat(new HashMap<String, Integer>()).isNotInstanceOf(HashMap.class);
* assertThat(new HashMap<String, Integer>()).isNotInstanceOf(Map.class);
*
* @param type the type to check the actual value against.
* @return this assertion object.
* @throws NullPointerException if the given type is {@code null}.
* @throws AssertionError if the actual value is {@code null}.
* @throws AssertionError if the actual value is an instance of the given type.
*/
S isNotInstanceOf(Class> type);
/**
* Verifies that the actual value is not an instance of any of the given types.
*
* Example:
*
// assertions will pass
* assertThat(1).isNotInstanceOfAny(Double.class, Float.class);
* assertThat(new ArrayList<String>()).isNotInstanceOfAny(LinkedList.class, Vector.class);
*
* // assertions will fail
* assertThat(1).isNotInstanceOfAny(Double.class, Integer.class);
* assertThat(new ArrayList<String>()).isNotInstanceOfAny(LinkedList.class, ArrayList.class);
* assertThat(new HashMap<String, Integer>()).isNotInstanceOfAny(TreeMap.class, Map.class);
*
* @param types the types to check the actual value against.
* @return this assertion object.
* @throws AssertionError if the actual value is {@code null}.
* @throws AssertionError if the actual value is an instance of any of the given types.
* @throws NullPointerException if the given array of types is {@code null}.
* @throws NullPointerException if the given array of types contains {@code null}s.
*/
S isNotInstanceOfAny(Class>... types);
/**
* Verifies that the actual value has the same class as the given object.
*
* Example:
*
// assertions will pass
* assertThat(1).hasSameClassAs(2);
* assertThat("abc").hasSameClassAs("123");
* assertThat(new ArrayList<String>()).hasSameClassAs(new ArrayList<Integer>());
*
* // assertions will fail
* assertThat(1).hasSameClassAs("abc");
* assertThat(new ArrayList<String>()).hasSameClassAs(new LinkedList<String>());
*
* @param other the object to check type against.
* @return this assertion object.
* @throws AssertionError if the actual has not the same type has the given object.
* @throws NullPointerException if the actual value is null.
* @throws NullPointerException if the given object is null.
*/
S hasSameClassAs(Object other);
/**
* Verifies that actual actual.toString()
is equal to the given String
.
*
* Example :
*
CartoonCaracter homer = new CartoonCaracter("Homer");
*
* // Instead of writing ...
* assertThat(homer.toString()).isEqualTo("Homer");
* // ... you can simply write:
* assertThat(homer).hasToString("Homer");
*
* @param expectedToString the expected String description of actual.
* @return this assertion object.
* @throws AssertionError if actual.toString()
result is not to the given String
.
* @throws AssertionError if actual is {@code null}.
*/
S hasToString(String expectedToString);
/**
* Verifies that the actual value does not have the same class as the given object.
*
* Example:
*
// assertions will pass
* assertThat(1).doesNotHaveSameClassAs("abc");
* assertThat(new ArrayList<String>()).doesNotHaveSameClassAs(new LinkedList<String>());
*
* // assertions will fail
* assertThat(1).doesNotHaveSameClassAs(2);
* assertThat("abc").doesNotHaveSameClassAs("123");
* assertThat(new ArrayList<String>()).doesNotHaveSameClassAs(new ArrayList<Integer>());
*
* @param other the object to check type against.
* @return this assertion object.
* @throws AssertionError if the actual has the same type has the given object.
* @throws NullPointerException if the actual value is null.
* @throws NullPointerException if the given object is null.
*/
S doesNotHaveSameClassAs(Object other);
/**
* Verifies that the actual value is exactly an instance of the given type.
*
* Example:
*
// assertions will pass
* assertThat("abc").isExactlyInstanceOf(String.class);
* assertThat(new ArrayList<String>()).isExactlyInstanceOf(ArrayList.class);
* assertThat(new HashMap<String, Integer>()).isExactlyInstanceOf(HashMap.class);
*
* // assertions will fail
* assertThat(1).isExactlyInstanceOf(String.class);
* assertThat(new ArrayList<String>()).isExactlyInstanceOf(List.class);
* assertThat(new HashMap<String, Integer>()).isExactlyInstanceOf(Map.class);
*
* @param type the type to check the actual value against.
* @return this assertion object.
* @throws AssertionError if the actual is not exactly an instance of given type.
* @throws NullPointerException if the actual value is null.
* @throws NullPointerException if the given object is null.
*/
S isExactlyInstanceOf(Class> type);
/**
* Verifies that the actual value is not exactly an instance of given type.
*
* Example:
*
// assertions will pass
* assertThat(1).isNotExactlyInstanceOf(String.class);
* assertThat(new ArrayList<String>()).isNotExactlyInstanceOf(List.class);
* assertThat(new HashMap<String, Integer>()).isNotExactlyInstanceOf(Map.class);
*
* // assertions will fail
* assertThat("abc").isNotExactlyInstanceOf(String.class);
* assertThat(new ArrayList<String>()).isNotExactlyInstanceOf(ArrayList.class);
* assertThat(new HashMap<String, Integer>()).isNotExactlyInstanceOf(HashMap.class);
*
* @param type the type to check the actual value against.
* @return this assertion object.
* @throws AssertionError if the actual is exactly an instance of given type.
* @throws NullPointerException if the actual value is null.
* @throws NullPointerException if the given object is null.
*/
S isNotExactlyInstanceOf(Class> type);
/**
* Verifies that the actual value type is in given types.
*
* Example:
*
// assertions will pass
* assertThat(new HashMap<String, Integer>()).isOfAnyClassIn(HashMap.class, TreeMap.class);
* assertThat(new ArrayList<String>()).isOfAnyClassIn(ArrayList.class, LinkedList.class);
*
* // assertions will fail
* assertThat(new HashMap<String, Integer>()).isOfAnyClassIn(TreeMap.class, Map.class);
* assertThat(new ArrayList<String>()).isOfAnyClassIn(LinkedList.class, List.class);
*
* @param types the types to check the actual value against.
* @return this assertion object.
* @throws AssertionError if the actual value type is not in given type.
* @throws NullPointerException if the actual value is null.
* @throws NullPointerException if the given types is null.
*/
S isOfAnyClassIn(Class>... types);
/**
* Verifies that the actual value type is not in given types.
*
* Example:
*
// assertions will pass
* assertThat(new HashMap<String, Integer>()).isNotOfAnyClassIn(Map.class, TreeMap.class);
* assertThat(new ArrayList<String>()).isNotOfAnyClassIn(LinkedList.class, List.class);
*
* // assertions will fail
* assertThat(new HashMap<String, Integer>()).isNotOfAnyClassIn(HashMap.class, TreeMap.class);
* assertThat(new ArrayList<String>()).isNotOfAnyClassIn(ArrayList.class, LinkedList.class);
*
* @param types the types to check the actual value against.
* @return this assertion object.
* @throws AssertionError if the actual value type is in given types.
* @throws NullPointerException if the actual value is null.
* @throws NullPointerException if the given types is null.
*/
S isNotOfAnyClassIn(Class>... types);
/**
* Verifies that the actual value is an instance of List,
* and returns a list assertion, to allow chaining of list-specific
* assertions from this call.
*
* Example :
*
Object sortedListAsObject = Arrays.asList(1, 2, 3);
*
* // assertion will pass
* assertThat(sortedListAsObject).asList().isSorted();
*
* Object unsortedListAsObject = Arrays.asList(3, 1, 2);
*
* // assertion will fail
* assertThat(unsortedListAsObject).asList().isSorted();
*
* @return a list assertion object
*/
AbstractListAssert, ?, Object> asList();
/**
* Verifies that the actual value is an instance of String,
* and returns a String assertion, to allow chaining of String-specific
* assertions from this call.
*
* Example :
*
Object stringAsObject = "hello world";
*
* // assertion will pass
* assertThat(stringAsObject).asString().contains("hello");
*
* // assertion will fail
* assertThat(stringAsObject).asString().contains("holla");
*
* @return a string assertion object
*/
AbstractCharSequenceAssert, String> asString();
/**
* @deprecated
* Throws {@link UnsupportedOperationException}
if called. It is easy to accidentally call
* {@link #equals(Object)}
instead of {@link #isEqualTo(Object)}
.
* @throws UnsupportedOperationException if this method is called.
*/
@Override
@Deprecated
boolean equals(Object obj);
/**
* In case of assertion error, the thread dump will be printed on {@link System#err}.
*
* Example :
*
assertThat("Messi").withThreadDumpOnError().isEqualTo("Ronaldo");
*
* will print the thread dump, something looking like:
* "JDWP Command Reader"
* java.lang.Thread.State: RUNNABLE
*
* "JDWP Event Helper Thread"
* java.lang.Thread.State: RUNNABLE
*
* "JDWP Transport Listener: dt_socket"
* java.lang.Thread.State: RUNNABLE
*
* "Signal Dispatcher"
* java.lang.Thread.State: RUNNABLE
*
* "Finalizer"
* java.lang.Thread.State: WAITING
* at java.lang.Object.wait(Native Method)
* at java.lang.ref.ReferenceQueue.remove(ReferenceQueue.java:135)
* at java.lang.ref.ReferenceQueue.remove(ReferenceQueue.java:151)
* at java.lang.ref.Finalizer$FinalizerThread.run(Finalizer.java:189)
*
* "Reference Handler"
* java.lang.Thread.State: WAITING
* at java.lang.Object.wait(Native Method)
* at java.lang.Object.wait(Object.java:503)
* at java.lang.ref.Reference$ReferenceHandler.run(Reference.java:133)
*
* "main"
* java.lang.Thread.State: RUNNABLE
* at sun.management.ThreadImpl.dumpThreads0(Native Method)
* at sun.management.ThreadImpl.dumpAllThreads(ThreadImpl.java:446)
* at com.fitbur.assertj.internal.Failures.threadDumpDescription(Failures.java:193)
* at com.fitbur.assertj.internal.Failures.printThreadDumpIfNeeded(Failures.java:141)
* at com.fitbur.assertj.internal.Failures.failure(Failures.java:91)
* at com.fitbur.assertj.internal.Objects.assertEqual(Objects.java:314)
* at com.fitbur.assertj.api.AbstractAssert.isEqualTo(AbstractAssert.java:198)
* at org.assertj.examples.ThreadDumpOnErrorExample.main(ThreadDumpOnErrorExample.java:28)
*
* @return this assertion object.
*/
S withThreadDumpOnError();
}