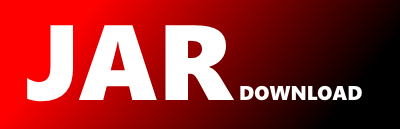
com.fitbur.assertj.api.BDDAssertions Maven / Gradle / Ivy
/**
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
* Copyright 2012-2016 the original author or authors.
*/
package com.fitbur.assertj.api;
import java.io.File;
import java.io.InputStream;
import java.math.BigDecimal;
import java.net.URI;
import java.net.URL;
import java.time.LocalDate;
import java.time.LocalDateTime;
import java.time.LocalTime;
import java.time.OffsetDateTime;
import java.time.OffsetTime;
import java.time.ZonedDateTime;
import java.util.Date;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import java.util.OptionalDouble;
import java.util.OptionalInt;
import java.util.OptionalLong;
import java.util.concurrent.CompletableFuture;
import com.fitbur.assertj.api.ThrowableAssert.ThrowingCallable;
/**
* BDD style entry point for assertion methods for different data types. Each method in this class is a static factory
* for the type-specific assertion objects. The purpose of this class is to make test code more readable.
*
* The difference with Assertions class is that entry point methods are named then
instead of
* assertThat
.
*
* For example:
*
{@literal @}Test
* public void bdd_assertions_examples() {
*
* //given
* List<BasketBallPlayer> bulls = new ArrayList<BasketBallPlayer>();
*
* //when
* bulls.add(rose);
* bulls.add(noah);
*
* then(bulls).contains(rose, noah).doesNotContain(james);
* }
*
* @author Alex Ruiz
* @author Yvonne Wang
* @author David DIDIER
* @author Ted Young
* @author Joel Costigliola
* @author Matthieu Baechler
* @author Mikhail Mazursky
* @author Nicolas François
* @author Julien Meddah
* @author William Delanoue
* @author Mariusz Smykula
*/
public class BDDAssertions extends Assertions {
/**
* Create assertion for {@link java.util.Optional}.
*
* @param optional the actual value.
* @param the type of the value contained in the {@link java.util.Optional}.
*
* @return the created assertion object.
*/
public static OptionalAssert then(Optional optional) {
return assertThat(optional);
}
/**
* Create assertion for {@link java.util.OptionalInt}.
*
* @param optional the actual value.
*
* @return the created assertion object.
*/
public static OptionalIntAssert then(OptionalInt optional) {
return assertThat(optional);
}
/**
* Create assertion for {@link java.util.OptionalLong}.
*
* @param optional the actual value.
*
* @return the created assertion object.
*/
public static OptionalLongAssert then(OptionalLong optional) {
return assertThat(optional);
}
/**
* Create assertion for {@link java.util.OptionalDouble}.
*
* @param optional the actual value.
*
* @return the created assertion object.
*/
public static OptionalDoubleAssert then(OptionalDouble optional) {
return assertThat(optional);
}
/**
* Creates a new instance of {@link com.fitbur.assertj.api.BigDecimalAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public static AbstractBigDecimalAssert> then(BigDecimal actual) {
return assertThat(actual);
}
/**
* Creates a new instance of {@link com.fitbur.assertj.api.BooleanAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public static AbstractBooleanAssert> then(boolean actual) {
return assertThat(actual);
}
/**
* Creates a new instance of {@link com.fitbur.assertj.api.BooleanAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public static AbstractBooleanAssert> then(Boolean actual) {
return assertThat(actual);
}
/**
* Creates a new instance of {@link com.fitbur.assertj.api.BooleanArrayAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public static AbstractBooleanArrayAssert> then(boolean[] actual) {
return assertThat(actual);
}
/**
* Creates a new instance of {@link com.fitbur.assertj.api.ByteAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public static AbstractByteAssert> then(byte actual) {
return assertThat(actual);
}
/**
* Creates a new instance of {@link com.fitbur.assertj.api.ByteAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public static AbstractByteAssert> then(Byte actual) {
return assertThat(actual);
}
/**
* Creates a new instance of {@link com.fitbur.assertj.api.ByteArrayAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public static AbstractByteArrayAssert> then(byte[] actual) {
return assertThat(actual);
}
/**
* Creates a new instance of {@link com.fitbur.assertj.api.CharacterAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public static AbstractCharacterAssert> then(char actual) {
return assertThat(actual);
}
/**
* Creates a new instance of {@link com.fitbur.assertj.api.CharArrayAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public static AbstractCharArrayAssert> then(char[] actual) {
return assertThat(actual);
}
/**
* Creates a new instance of {@link com.fitbur.assertj.api.CharacterAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public static AbstractCharacterAssert> then(Character actual) {
return assertThat(actual);
}
/**
* Creates a new instance of {@link com.fitbur.assertj.api.ClassAssert}
*
* @param actual the actual value.
* @return the created assertion object.
*/
public static AbstractClassAssert> then(Class> actual) {
return assertThat(actual);
}
/**
* Creates a new instance of {@link com.fitbur.assertj.api.GenericComparableAssert}
with
* standard comparison semantics.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public static > AbstractComparableAssert, T> then(T actual) {
return assertThat(actual);
}
/**
* Creates a new instance of {@link com.fitbur.assertj.api.IterableAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public static AbstractIterableAssert, ? extends Iterable extends T>, T> then(Iterable extends T> actual) {
return new IterableAssert<>(actual);
}
/**
* Creates a new instance of {@link com.fitbur.assertj.api.IterableAssert}
. The {@link
* java.util.Iterator}
is first
* converted
* into an {@link Iterable}
*
* @param actual the actual value.
* @return the created assertion object.
*/
public static AbstractIterableAssert, ? extends Iterable extends T>, T> then(Iterator extends T> actual) {
return new IterableAssert<>(actual);
}
/**
* Creates a new instance of {@link com.fitbur.assertj.api.DoubleAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public static AbstractDoubleAssert> then(double actual) {
return assertThat(actual);
}
/**
* Creates a new instance of {@link com.fitbur.assertj.api.DoubleAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public static AbstractDoubleAssert> then(Double actual) {
return assertThat(actual);
}
/**
* Creates a new instance of {@link com.fitbur.assertj.api.DoubleArrayAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public static AbstractDoubleArrayAssert> then(double[] actual) {
return assertThat(actual);
}
/**
* Creates a new instance of {@link com.fitbur.assertj.api.FileAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public static AbstractFileAssert> then(File actual) {
return assertThat(actual);
}
/**
* Creates a new instance of {@link com.fitbur.assertj.api.InputStreamAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public static AbstractInputStreamAssert, ? extends InputStream> then(InputStream actual) {
return assertThat(actual);
}
/**
* Creates a new instance of {@link com.fitbur.assertj.api.FloatAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public static AbstractFloatAssert> then(float actual) {
return assertThat(actual);
}
/**
* Creates a new instance of {@link com.fitbur.assertj.api.FloatAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public static AbstractFloatAssert> then(Float actual) {
return assertThat(actual);
}
/**
* Creates a new instance of {@link com.fitbur.assertj.api.FloatArrayAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public static AbstractFloatArrayAssert> then(float[] actual) {
return assertThat(actual);
}
/**
* Creates a new instance of {@link com.fitbur.assertj.api.IntegerAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public static AbstractIntegerAssert> then(int actual) {
return assertThat(actual);
}
/**
* Creates a new instance of {@link com.fitbur.assertj.api.IntArrayAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public static AbstractIntArrayAssert> then(int[] actual) {
return assertThat(actual);
}
/**
* Creates a new instance of {@link com.fitbur.assertj.api.IntegerAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public static AbstractIntegerAssert> then(Integer actual) {
return assertThat(actual);
}
/**
* Creates a new instance of {@link com.fitbur.assertj.api.ListAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public static AbstractListAssert, ? extends List extends T>, T> then(List extends T> actual) {
return new ListAssert<>(actual);
}
/**
* Creates a new instance of {@link com.fitbur.assertj.api.LongAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public static AbstractLongAssert> then(long actual) {
return assertThat(actual);
}
/**
* Creates a new instance of {@link com.fitbur.assertj.api.LongAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public static AbstractLongAssert> then(Long actual) {
return assertThat(actual);
}
/**
* Creates a new instance of {@link com.fitbur.assertj.api.LongArrayAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public static AbstractLongArrayAssert> then(long[] actual) {
return assertThat(actual);
}
/**
* Creates a new instance of {@link com.fitbur.assertj.api.ObjectAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public static AbstractObjectAssert, T> then(T actual) {
return assertThat(actual);
}
/**
* Creates a new instance of {@link com.fitbur.assertj.api.ObjectArrayAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public static AbstractObjectArrayAssert, T> then(T[] actual) {
return assertThat(actual);
}
/**
* Creates a new instance of {@link com.fitbur.assertj.api.MapAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public static AbstractMapAssert, ? extends Map, K, V> then(Map actual) {
return assertThat(actual);
}
/**
* Creates a new instance of {@link com.fitbur.assertj.api.ShortAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public static AbstractShortAssert> then(short actual) {
return assertThat(actual);
}
/**
* Creates a new instance of {@link com.fitbur.assertj.api.ShortAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public static AbstractShortAssert> then(Short actual) {
return assertThat(actual);
}
/**
* Creates a new instance of {@link com.fitbur.assertj.api.ShortArrayAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public static AbstractShortArrayAssert> then(short[] actual) {
return assertThat(actual);
}
/**
* Creates a new instance of {@link com.fitbur.assertj.api.CharSequenceAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public static AbstractCharSequenceAssert, ? extends CharSequence> then(CharSequence actual) {
return assertThat(actual);
}
/**
* Creates a new instance of {@link com.fitbur.assertj.api.StringAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public static AbstractCharSequenceAssert, String> then(String actual) {
return assertThat(actual);
}
/**
* Creates a new instance of {@link com.fitbur.assertj.api.DateAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public static AbstractDateAssert> then(Date actual) {
return assertThat(actual);
}
/**
* Creates a new instance of {@link com.fitbur.assertj.api.ThrowableAssert}
.
*
* @param actual the actual value.
* @return the created assertion Throwable.
*/
public static AbstractThrowableAssert, ? extends Throwable> then(Throwable actual) {
return assertThat(actual);
}
/**
* Allows to capture and then assert on a {@link Throwable} more easily when used with Java 8 lambdas.
*
*
* Java 8 example :
*
{@literal @}Test
* public void testException() {
* thenThrownBy(() -> { throw new Exception("boom!"); }).isInstanceOf(Exception.class)
* .hasMessageContaining("boom");
* }
*
* @param shouldRaiseThrowable The {@link ThrowingCallable} or lambda with the code that should raise the throwable.
* @return The captured exception or null
if none was raised by the callable.
*/
public static AbstractThrowableAssert, ? extends Throwable> thenThrownBy(ThrowingCallable shouldRaiseThrowable) {
return assertThatThrownBy(shouldRaiseThrowable);
}
/**
* Creates a new instance of {@link com.fitbur.assertj.api.LocalDateAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public static AbstractLocalDateAssert> then(LocalDate actual) {
return assertThat(actual);
}
/**
* Creates a new instance of {@link com.fitbur.assertj.api.LocalDateTimeAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public static AbstractLocalDateTimeAssert> then(LocalDateTime actual) {
return assertThat(actual);
}
/**
* Creates a new instance of {@link com.fitbur.assertj.api.ZonedDateTimeAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public static AbstractZonedDateTimeAssert> then(ZonedDateTime actual) {
return assertThat(actual);
}
/**
* Creates a new instance of {@link LocalTimeAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public static AbstractLocalTimeAssert> then(LocalTime actual) {
return assertThat(actual);
}
/**
* Creates a new instance of {@link OffsetTimeAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public static AbstractOffsetTimeAssert> then(OffsetTime actual) {
return assertThat(actual);
}
/**
* Creates a new instance of {@link UriAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public static AbstractUriAssert> then(URI actual) {
return assertThat(actual);
}
/**
* Creates a new instance of {@link UrlAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public static AbstractUrlAssert> then(URL actual) {
return assertThat(actual);
}
/**
* Creates a new instance of {@link OffsetTimeAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public static AbstractOffsetDateTimeAssert> then(OffsetDateTime actual) {
return assertThat(actual);
}
/**
* Create assertion for {@link java.util.concurrent.CompletableFuture}.
*
* @param future the actual value.
* @param the type of the value contained in the {@link java.util.concurrent.CompletableFuture}.
*
* @return the created assertion object.
*/
public static CompletableFutureAssert then(CompletableFuture future) {
return assertThat(future);
}
/**
* Creates a new
{@link com.fitbur.assertj.api.BDDAssertions}.
*/
protected BDDAssertions() {}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy