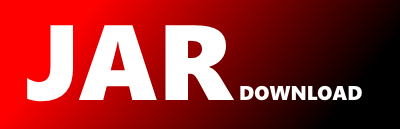
com.fitbur.assertj.api.Condition Maven / Gradle / Ivy
/** * Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with * the License. You may obtain a copy of the License at * * http://www.apache.org/licenses/LICENSE-2.0 * * Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on * an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the * specific language governing permissions and limitations under the License. * * Copyright 2012-2016 the original author or authors. */ package com.fitbur.assertj.api; import static java.util.Objects.requireNonNull; import static com.fitbur.assertj.api.DescriptionValidations.checkIsNotNull; import java.util.function.Predicate; import com.fitbur.assertj.description.Description; import com.fitbur.assertj.description.TextDescription; import com.fitbur.assertj.util.VisibleForTesting; /** * A condition to be met by an object. * * @param
{@link Condition}. The default description of this condition will the simple name of * the * condition's class. */ public Condition() { as(getClass().getSimpleName()); } /** * Creates a new {@link Condition}. * * @param description the description of this condition. * @throws NullPointerException if the given description is {@code null}. */ public Condition(String description) { as(description); } /** * Creates a new {@link Condition} with the given {@link Predicate}, the built Condition will be met if * the Predicate is. * *the type of object this condition accepts. * * @author Yvonne Wang * @author Alex Ruiz */ public class Condition implements Descriptable > { @VisibleForTesting Description description; // might not be used private Predicate predicate; /** * Creates a new * You must give a description, it will be used to build a nice error message when the condition fails, you can pass * args to build the description as in {@link String#format(String, Object...)}. *
* Example: *
* * Error message example: *// build condition with Predicate<String> and set description using String#format pattern. * Condition<String> fairyTale = new Condition<String>(s -> s.startsWith("Once upon a time"), "a %s tale", "fairy"); * * String littleRedCap = "Once upon a time there was a dear little girl ..."; * assertThat(littleRedCap).is(fairyTale);
* * @param predicate the {@link Predicate} used to build the condition. * @param description the description of this condition. * @param args optional parameter if description is a format String. * @throws NullPointerException if the given {@link Predicate} is {@code null}. * @throws NullPointerException if the given description is {@code null}. */ public Condition(Predicate// unfortunately this assertion fails ... but contact me if you can make it pass :) * assertThat("life").is(fairyTale); * // error message * Expecting: * <"life"> * to be <a fairy tale>
predicate, String description, Object... args) { checkPredicate(predicate); this.predicate = predicate; this.description = new TextDescription(description, args); } /** * Creates a new {@link Condition}. * * @param description the description of this condition. * @throws NullPointerException if the given description is {@code null}. */ public Condition(Description description) { as(description); } /** {@inheritDoc} */ @Override public Condition describedAs(String newDescription, Object... args) { return as(newDescription, args); } /** {@inheritDoc} */ @Override public Condition as(String newDescription, Object... args) { description = checkIsNotNull(newDescription, args); return this; } /** {@inheritDoc} */ @Override public Condition describedAs(Description newDescription) { return as(newDescription); } /** {@inheritDoc} */ @Override public Condition as(Description newDescription) { description = checkIsNotNull(newDescription); return this; } /** * Returns the description of this condition. * * @return the description of this condition. */ public Description description() { return description; } /** * Verifies that the given value satisfies this condition. * * @param value the value to verify. * @return {@code true} if the given value satisfies this condition; {@code false} otherwise. */ public boolean matches(T value) { checkPredicate(predicate); return predicate.test(value); } private void checkPredicate(Predicate predicate) { requireNonNull(predicate, "Unless you subclass Condition and override matches, you need to pass a non null Predicate to build a Condition."); } @Override public String toString() { return description.value(); } }
© 2015 - 2024 Weber Informatics LLC | Privacy Policy