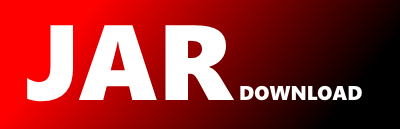
com.fitbur.assertj.api.FloatingPointNumberAssert Maven / Gradle / Ivy
/**
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
* Copyright 2012-2016 the original author or authors.
*/
package com.fitbur.assertj.api;
import com.fitbur.assertj.data.Offset;
/**
* Assertion methods applicable to floating-point {@link Number}
s.
* @param the "self" type of this assertion class. Please read "Emulating
* 'self types' using Java Generics to simplify fluent API implementation" for more details.
* @param the type of the "actual" value.
*
* @author Alex Ruiz
* @author Yvonne Wang
* @author Mikhail Mazursky
*/
public interface FloatingPointNumberAssert, A extends Number> extends NumberAssert {
/**
* Verifies that the actual value is close to the given one by less than the given offset.
* If difference is equal to offset value, assertion is considered valid.
*
* Example with double:
*
// assertion will pass:
* assertThat(8.1).isEqualTo(new Double(8.0), offset(0.2));
*
* // if difference is exactly equals to the offset (0.1), it's ok
* assertThat(8.1).isEqualTo(new Double(8.0), offset(0.1));
*
* // within is an alias of offset
* assertThat(8.1).isEqualTo(new Double(8.0), within(0.1));
*
* // assertion will fail
* assertThat(8.1).isEqualTo(new Double(8.0), offset(0.01));
*
* @param expected the given value to compare the actual value to.
* @param offset the given positive offset.
* @return {@code this} assertion object.
* @throws NullPointerException if the given offset is {@code null}.
* @throws NullPointerException if the expected number is {@code null}.
* @throws AssertionError if the actual value is not equal to the given one.
*/
S isEqualTo(A expected, Offset offset);
/**
* Verifies that the actual number is close to the given one within the given offset.
* If difference is equal to offset value, assertion is considered valid.
*
* Example with double:
*
// assertions will pass:
* assertThat(8.1).isCloseTo(new Double(8.0), within(0.2));
*
* // you can use offset if you prefer
* assertThat(8.1).isCloseTo(new Double(8.0), offset(0.2));
*
* // if difference is exactly equals to the offset (0.1), it's ok
* assertThat(8.1).isCloseTo(new Double(8.0), within(0.1));
*
* // assertion will fail
* assertThat(8.1).isCloseTo(new Double(8.0), within(0.01));
*
* @param expected the given number to compare the actual value to.
* @param offset the given positive offset.
* @return {@code this} assertion object.
* @throws NullPointerException if the given offset is {@code null}.
* @throws NullPointerException if the expected number is {@code null}.
* @throws AssertionError if the actual value is not equal to the given one.
*/
S isCloseTo(A expected, Offset offset);
/**
* Verifies that the actual value is equal to {@code NaN}.
*
* Example:
*
// assertions will pass
* assertThat(Double.NaN).isNaN();
* assertThat(0.0 / 0.0).isNaN();
* assertThat(0.0F * Float.POSITIVE_INFINITY).isNaN();
*
* // assertions will fail
* assertThat(1.0).isNaN();
* assertThat(-1.0F).isNaN();
*
* @return this assertion object.
* @throws AssertionError if the actual value is not equal to {@code NaN}.
*/
S isNaN();
/**
* Verifies that the actual value is not equal to {@code NaN}.
*
* Example:
*
// assertions will pass
* assertThat(1.0).isNotNaN();
* assertThat(-1.0F).isNotNaN();
*
* // assertions will fail
* assertThat(Double.NaN).isNotNaN();
* assertThat(0.0 / 0.0).isNotNaN();
* assertThat(0.0F * Float.POSITIVE_INFINITY).isNotNaN();
*
* @return this assertion object.
* @throws AssertionError if the actual value is equal to {@code NaN}.
*/
S isNotNaN();
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy