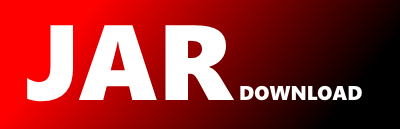
com.fitbur.assertj.api.ThrowableAssertAlternative Maven / Gradle / Ivy
/**
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
* Copyright 2012-2016 the original author or authors.
*/
package com.fitbur.assertj.api;
/**
* Assertion methods for {@link java.lang.Throwable} similar to {@link ThrowableAssert} but with assertions methods named
* differently to make testing code fluent (ex : withMessage
instead of hasMessage
.
*
*
assertThatExceptionOfType(IOException.class)
* .isThrownBy(() -> { throw new IOException("boom! tcha!"); });
* .withMessage("boom! %s", "tcha!");
*
* This class is linked with the {@link ThrowableTypeAssert} and allow to check that an exception
* type is thrown by a lambda.
*/
public class ThrowableAssertAlternative extends AbstractAssert, T> {
private ThrowableAssert delegate;
ThrowableAssertAlternative(final T actual) {
super(actual, ThrowableAssertAlternative.class);
delegate = new ThrowableAssert(actual);
}
/**
* Verifies that the message of the actual {@code Throwable} is equal to the given one.
*
* Examples:
*
Throwable illegalArgumentException = new IllegalArgumentException("wrong amount 123");
*
* // assertion will pass
* assertThatExceptionOfType(Throwable.class)
* .isThrownBy(() -> {throw illegalArgumentException;})
* .withMessage("wrong amount 123");
*
* // assertion will fail
* assertThatExceptionOfType(Throwable.class)
* .isThrownBy(() -> {throw illegalArgumentException;})
* .withMessage("wrong amount 123 euros");
*
* @param message the expected message.
* @return this assertion object.
* @throws AssertionError if the actual {@code Throwable} is {@code null}.
* @throws AssertionError if the message of the actual {@code Throwable} is not equal to the given one.
* @see AbstractThrowableAssert#hasMessage(String)
*/
public ThrowableAssertAlternative withMessage(String message) {
delegate.hasMessage(message);
return this;
}
/**
* Verifies that the message of the actual {@code Throwable} is equal to the given one built using {@link String#format(String, Object...)} syntax.
*
* Examples:
*
Throwable illegalArgumentException = new IllegalArgumentException("wrong amount 123");
*
* // assertion will pass
* assertThatExceptionOfType(Throwable.class)
* .isThrownBy(() -> {throw illegalArgumentException;})
* .withMessage("wrong amount %s, "123");
*
* // assertion will fail
* assertThatExceptionOfType(Throwable.class)
* .isThrownBy(() -> {throw illegalArgumentException;})
* .withMessage("wrong amount 123 euros");
*
* @param message a format string representing the expected message
* @param parameters argument referenced by the format specifiers in the format string
* @return this assertion object.
* @throws AssertionError if the actual {@code Throwable} is {@code null}.
* @throws AssertionError if the message of the actual {@code Throwable} is not equal to the given one.
* @see AbstractThrowableAssert#hasMessage(String)
*/
public ThrowableAssertAlternative withMessage(String message, Object... parameters) {
delegate.hasMessage(message, parameters);
return this;
}
/**
* Verifies that the actual {@code Throwable} has a cause similar to the given one, that is with same type and message
* (it does not use {@link Throwable#equals(Object) equals} method for comparison).
*
* Example:
*
Throwable illegalArgumentException = new IllegalArgumentException("invalid arg");
* Throwable wrappingException = new Throwable(illegalArgumentException);
*
* // This assertion succeeds:
*
* assertThatExceptionOfType(Throwable.class)
* .isThrownBy(() -> {throw wrappingException;})
* .withCause(illegalArgumentException);
*
* // These assertions fail:
*
* assertThatExceptionOfType(Throwable.class)
* .isThrownBy(() -> {throw wrappingException;})
* .withCause(new IllegalArgumentException("bad arg"));
*
* assertThatExceptionOfType(Throwable.class)
* .isThrownBy(() -> {throw wrappingException;})
* .withCause(new NullPointerException());
*
* assertThatExceptionOfType(Throwable.class)
* .isThrownBy(() -> {throw wrappingException;})
* .withCause(null);
*
* @return this assertion object.
* @throws AssertionError if the actual {@code Throwable} is {@code null}.
* @throws AssertionError if the actual {@code Throwable} has not the given cause.
* @see AbstractThrowableAssert#hasCause(Throwable)
*/
public ThrowableAssertAlternative withCause(Throwable cause) {
delegate.hasCause(cause);
return this;
}
/**
* Verifies that the actual {@code Throwable} does not have a cause.
*
* Example:
*
IllegalArgumentException exception = new IllegalArgumentException();
*
* // This assertion succeeds:
* assertThatExceptionOfType(IllegalArgumentException.class)
* .isThrownBy(() -> {throw exception;})
* .withNoCause();
*
* // These assertion fails:
* Throwable illegalArgumentException = new Throwable(exception);
* assertThatExceptionOfType(Throwable.class)
* .isThrownBy(() -> {throw illegalArgumentException;})
* .withNoCause();
*
* @return this assertion object.
* @throws AssertionError if the actual {@code Throwable} is {@code null}.
* @throws AssertionError if the actual {@code Throwable} has a cause.
* @see AbstractThrowableAssert#hasNoCause()
*/
public ThrowableAssertAlternative withNoCause() {
delegate.hasNoCause();
return this;
}
/**
* Verifies that the message of the actual {@code Throwable} starts with the given description.
*
* Examples:
*
Throwable illegalArgumentException = new IllegalArgumentException("wrong amount 123");
*
* // assertion will pass
* assertThatExceptionOfType(Throwable.class)
* .isThrownBy(() -> {throw illegalArgumentException;})
* .withMessageStartingWith("wrong amount");
*
* // assertion will fail
* assertThatExceptionOfType(Throwable.class)
* .isThrownBy(() -> {throw illegalArgumentException;})
* .withMessageStartingWith("right amount");
*
* @param description the description expected to start the actual {@code Throwable}'s message.
* @return this assertion object.
* @throws AssertionError if the actual {@code Throwable} is {@code null}.
* @throws AssertionError if the message of the actual {@code Throwable} does not start with the given description.
* @see AbstractThrowableAssert#hasMessageStartingWith(String)
*/
public ThrowableAssertAlternative withMessageStartingWith(String description) {
delegate.hasMessageStartingWith(description);
return this;
}
/**
* Verifies that the message of the actual {@code Throwable} contains with the given description.
*
* Examples:
*
Throwable illegalArgumentException = new IllegalArgumentException("wrong amount 123");
*
* // assertion will pass
* assertThatExceptionOfType(Throwable.class)
* .isThrownBy(() -> {throw illegalArgumentException;})
* .withMessageContaining("amount");
*
* // assertion will fail
* assertThatExceptionOfType(Throwable.class)
* .isThrownBy(() -> {throw illegalArgumentException;})
* .withMessageContaining("456");
*
* @param description the description expected to be contained in the actual {@code Throwable}'s message.
* @return this assertion object.
* @throws AssertionError if the actual {@code Throwable} is {@code null}.
* @throws AssertionError if the message of the actual {@code Throwable} does not contain the given description.
* @see AbstractThrowableAssert#hasMessageContaining(String)
*/
public ThrowableAssertAlternative withMessageContaining(String description) {
delegate.hasMessageContaining(description);
return this;
}
/**
* Verifies that the stack trace of the actual {@code Throwable} contains with the given description.
*
* Examples:
*
Throwable illegalArgumentException = new IllegalArgumentException("wrong amount 123");
*
* // assertion will pass
* assertThatExceptionOfType(Throwable.class)
* .isThrownBy(() -> {throw illegalArgumentException;})
* .withStackTraceContaining("amount");
*
* // assertion will fail
* assertThatExceptionOfType(Throwable.class)
* .isThrownBy(() -> {throw illegalArgumentException;})
* .withStackTraceContaining("456");
*
* @param description the description expected to be contained in the actual {@code Throwable}'s stack trace.
* @return this assertion object.
* @throws AssertionError if the actual {@code Throwable} is {@code null}.
* @throws AssertionError if the stack trace of the actual {@code Throwable} does not contain the given description.
* @see AbstractThrowableAssert#hasStackTraceContaining(String)
*/
public ThrowableAssertAlternative withStackTraceContaining(String description) {
delegate.hasStackTraceContaining(description);
return this;
}
/**
* Verifies that the message of the actual {@code Throwable} matches with the given regular expression.
*
* Examples:
*
Throwable illegalArgumentException = new IllegalArgumentException("wrong amount 123");
*
* // assertion will pass
* assertThatExceptionOfType(Throwable.class)
* .isThrownBy(() -> {throw illegalArgumentException;})
* .withMessageMatching("wrong amount [0-9]*");
*
* // assertion will fail
* assertThatExceptionOfType(Throwable.class)
* .isThrownBy(() -> {throw illegalArgumentException;})
* .withMessageMatching("wrong amount [0-9]* euros");
*
* @param regex the regular expression of value expected to be matched the actual {@code Throwable}'s message.
* @return this assertion object.
* @throws AssertionError if the actual {@code Throwable} is {@code null}.
* @throws AssertionError if the message of the actual {@code Throwable} does not match the given regular expression.
* @throws NullPointerException if the regex is null
* @see AbstractThrowableAssert#hasMessageMatching(String)
*/
public ThrowableAssertAlternative withMessageMatching(String regex) {
delegate.hasMessageMatching(regex);
return this;
}
/**
* Verifies that the message of the actual {@code Throwable} ends with the given description.
*
* Examples:
*
Throwable illegalArgumentException = new IllegalArgumentException("wrong amount 123");
*
* // assertion will pass
* assertThatExceptionOfType(Throwable.class)
* .isThrownBy(() -> {throw illegalArgumentException;})
* .withMessageEndingWith("123");
*
* // assertion will fail
* assertThatExceptionOfType(Throwable.class)
* .isThrownBy(() -> {throw illegalArgumentException;})
* .withMessageEndingWith("456");
*
* @param description the description expected to end the actual {@code Throwable}'s message.
* @return this assertion object.
* @throws AssertionError if the actual {@code Throwable} is {@code null}.
* @throws AssertionError if the message of the actual {@code Throwable} does not end with the given description.
* @see AbstractThrowableAssert#hasMessageEndingWith(String)
*/
public ThrowableAssertAlternative withMessageEndingWith(String description) {
delegate.hasMessageEndingWith(description);
return this;
}
/**
* Verifies that the cause of the actual {@code Throwable} is an instance of the given type.
*
* Example:
*
Throwable throwable = new Throwable(new NullPointerException());
*
* // assertion will pass
* assertThatExceptionOfType(Throwable.class)
* .isThrownBy(() -> {throw throwable;})
* .withCauseInstanceOf(NullPointerException.class);
* assertThatExceptionOfType(Throwable.class)
* .isThrownBy(() -> {throw throwable;})
* .withCauseInstanceOf(RuntimeException.class);
*
* // assertion will fail
* assertThatExceptionOfType(Throwable.class)
* .isThrownBy(() -> {throw throwable;})
* .withCauseInstanceOf(IllegalArgumentException.class);
*
* @param type the expected cause type.
* @return this assertion object.
* @throws NullPointerException if given type is {@code null}.
* @throws AssertionError if the actual {@code Throwable} is {@code null}.
* @throws AssertionError if the actual {@code Throwable} has no cause.
* @throws AssertionError if the cause of the actual {@code Throwable} is not an instance of the given type.
* @see AbstractThrowableAssert#hasCauseInstanceOf(Class)
*/
public ThrowableAssertAlternative withCauseInstanceOf(Class extends Throwable> type) {
delegate.hasCauseInstanceOf(type);
return this;
}
/**
* Verifies that the cause of the actual {@code Throwable} is exactly an instance of the given type.
*
* Example:
*
Throwable throwable = new Throwable(new NullPointerException());
*
* // assertion will pass
* assertThatExceptionOfType(Throwable.class)
* .isThrownBy(() -> {throw throwable;})
* .withCauseExactlyInstanceOf(NullPointerException.class);
*
* // assertions will fail (even if NullPointerException is a RuntimeException since we want an exact match)
* assertThatExceptionOfType(Throwable.class)
* .isThrownBy(() -> {throw throwable;})
* .withCauseExactlyInstanceOf(RuntimeException.class);
* assertThatExceptionOfType(Throwable.class)
* .isThrownBy(() -> {throw throwable;})
* .withCauseExactlyInstanceOf(IllegalArgumentException.class);
*
* @param type the expected cause type.
* @return this assertion object.
* @throws NullPointerException if given type is {@code null}.
* @throws AssertionError if the actual {@code Throwable} is {@code null}.
* @throws AssertionError if the actual {@code Throwable} has no cause.
* @throws AssertionError if the cause of the actual {@code Throwable} is not exactly an instance of the given
* type.
* @see AbstractThrowableAssert#hasCauseExactlyInstanceOf(Class)
*/
public ThrowableAssertAlternative withCauseExactlyInstanceOf(Class extends Throwable> type) {
delegate.hasCauseExactlyInstanceOf(type);
return this;
}
/**
* Verifies that the root cause of the actual {@code Throwable} is an instance of the given type.
*
* Example:
*
Throwable throwable = new Throwable(
* new IllegalStateException(
* new NullPointerException()));
*
* // assertion will pass
* assertThatExceptionOfType(Throwable.class)
* .isThrownBy(() -> {throw throwable;})
* .withRootCauseInstanceOf(NullPointerException.class);
* assertThatExceptionOfType(Throwable.class)
* .isThrownBy(() -> {throw throwable;})
* .withRootCauseInstanceOf(RuntimeException.class);
*
* // assertion will fail
* assertThatExceptionOfType(Throwable.class)
* .isThrownBy(() -> {throw throwable;})
* .withRootCauseInstanceOf(IllegalStateException.class);
*
* @param type the expected cause type.
* @return this assertion object.
* @throws NullPointerException if given type is {@code null}.
* @throws AssertionError if the actual {@code Throwable} is {@code null}.
* @throws AssertionError if the actual {@code Throwable} has no cause.
* @throws AssertionError if the cause of the actual {@code Throwable} is not an instance of the given type.
* @see AbstractThrowableAssert#hasRootCauseInstanceOf(Class)
*/
public ThrowableAssertAlternative withRootCauseInstanceOf(Class extends Throwable> type) {
delegate.hasRootCauseInstanceOf(type);
return this;
}
/**
* Verifies that the root cause of the actual {@code Throwable} is exactly an instance of the given type.
*
* Example:
*
Throwable throwable = new Throwable(
* new IllegalStateException(
* new NullPointerException()));
*
* // assertion will pass
* assertThatExceptionOfType(Throwable.class)
* .isThrownBy(() -> {throw throwable;})
* .withRootCauseExactlyInstanceOf(NullPointerException.class);
*
* // assertion will fail (even if NullPointerException is a RuntimeException since we want an exact match)
* assertThatExceptionOfType(Throwable.class)
* .isThrownBy(() -> {throw throwable;})
* .withRootCauseExactlyInstanceOf(RuntimeException.class);
* assertThatExceptionOfType(Throwable.class)
* .isThrownBy(() -> {throw throwable;})
* .withRootCauseExactlyInstanceOf(IllegalStateException.class);
*
* @param type the expected cause type.
* @return this assertion object.
* @throws NullPointerException if given type is {@code null}.
* @throws AssertionError if the actual {@code Throwable} is {@code null}.
* @throws AssertionError if the actual {@code Throwable} has no cause.
* @throws AssertionError if the root cause of the actual {@code Throwable} is not exactly an instance of the
* given type.
* @see AbstractThrowableAssert#hasRootCauseExactlyInstanceOf(Class)
*/
public ThrowableAssertAlternative withRootCauseExactlyInstanceOf(Class extends Throwable> type) {
delegate.hasRootCauseExactlyInstanceOf(type);
return this;
}
}