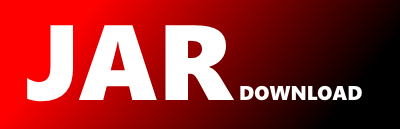
com.fitbur.assertj.api.WithAssertions Maven / Gradle / Ivy
/**
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
* Copyright 2012-2016 the original author or authors.
*/
package com.fitbur.assertj.api;
import java.io.File;
import java.io.InputStream;
import java.math.BigDecimal;
import java.nio.charset.Charset;
import java.text.DateFormat;
import java.time.LocalDate;
import java.time.LocalDateTime;
import java.time.LocalTime;
import java.time.OffsetDateTime;
import java.time.OffsetTime;
import java.time.ZonedDateTime;
import java.util.Date;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import java.util.OptionalDouble;
import java.util.OptionalInt;
import java.util.OptionalLong;
import java.util.concurrent.CompletableFuture;
import java.util.stream.Stream;
import com.fitbur.assertj.api.ThrowableAssert.ThrowingCallable;
import com.fitbur.assertj.api.filter.Filters;
import com.fitbur.assertj.condition.DoesNotHave;
import com.fitbur.assertj.condition.Not;
import com.fitbur.assertj.data.Index;
import com.fitbur.assertj.data.MapEntry;
import com.fitbur.assertj.data.Offset;
import com.fitbur.assertj.groups.Properties;
import com.fitbur.assertj.groups.Tuple;
/**
*
* A unified entry point to all non-deprecated assertions from both the new Java 8 core API and the pre-Java 8 core API.
*
* As a convenience, the methods are defined in an interface so that no static imports are necessary if the test class
* implements this interface.
*
* Based on an idea by David Gageot :
*
* http://blog.javabien.net/2014/04/23/what-if-assertj-used-java-8/
*
* @author Alan Rothkopf
*
*/
public interface WithAssertions {
/**
* Delegate call to {@link com.fitbur.assertj.api.Assertions#offset(Float)}
*/
default public Offset offset(final Float value) {
return Assertions.offset(value);
}
/**
* Delegate call to {@link com.fitbur.assertj.api.Assertions#offset(Double)}
*/
default public Offset offset(final Double value) {
return Assertions.offset(value);
}
/**
* Delegate call to {@link com.fitbur.assertj.api.Assertions#entry(Object, Object)}
*/
default public MapEntry entry(final K key, final V value) {
return Assertions.entry(key, value);
}
/**
* Delegate call to {@link com.fitbur.assertj.api.Assertions#fail(String)}
*/
default public void fail(final String failureMessage) {
Assertions.fail(failureMessage);
}
/**
* Delegate call to {@link com.fitbur.assertj.api.Assertions#fail(String,Throwable)}
*/
default public void fail(final String failureMessage, final Throwable realCause) {
Assertions.fail(failureMessage, realCause);
}
/**
* Delegate call to {@link com.fitbur.assertj.api.Assertions#not(Condition)}
*/
default public Not not(final Condition super T> condition) {
return Assertions.not(condition);
}
/**
* Delegate call to {@link com.fitbur.assertj.api.Assertions#allOf(Iterable extends Condition>)}
*/
default public Condition allOf(final Iterable extends Condition super T>> conditions) {
return Assertions.allOf(conditions);
}
/**
* Delegate call to {@link com.fitbur.assertj.api.Assertions#allOf(Condition[])}
*/
default public Condition allOf(@SuppressWarnings("unchecked") final Condition super T>... conditions) {
return Assertions.allOf(conditions);
}
/**
* Delegate call to {@link com.fitbur.assertj.api.Assertions#assertThat(T[])}
*/
default public AbstractObjectArrayAssert, T> assertThat(final T[] actual) {
return Assertions.assertThat(actual);
}
/**
* Delegate call to {@link com.fitbur.assertj.api.Assertions#assertThat(AssertDelegateTarget)}
*/
default public T assertThat(final T assertion) {
return Assertions.assertThat(assertion);
}
/**
* Delegate call to {@link com.fitbur.assertj.api.Assertions#assertThat(Map)}
*/
default public AbstractMapAssert, ? extends Map, K, V> assertThat(final Map actual) {
return Assertions.assertThat(actual);
}
/**
* Delegate call to {@link com.fitbur.assertj.api.Assertions#assertThat(short)}
*/
default public AbstractShortAssert> assertThat(final short actual) {
return Assertions.assertThat(actual);
}
/**
* Delegate call to {@link com.fitbur.assertj.api.Assertions#assertThat(long)}
*/
default public AbstractLongAssert> assertThat(final long actual) {
return Assertions.assertThat(actual);
}
/**
* Delegate call to {@link com.fitbur.assertj.api.Assertions#assertThat(Long)}
*/
default public AbstractLongAssert> assertThat(final Long actual) {
return Assertions.assertThat(actual);
}
/**
* Delegate call to {@link com.fitbur.assertj.api.Assertions#assertThat(long[])}
*/
default public AbstractLongArrayAssert> assertThat(final long[] actual) {
return Assertions.assertThat(actual);
}
/**
* Delegate call to {@link com.fitbur.assertj.api.Assertions#assertThat(T)}
*/
default public AbstractObjectAssert, T> assertThat(final T actual) {
return Assertions.assertThat(actual);
}
/**
* Delegate call to {@link com.fitbur.assertj.api.Assertions#assertThat(String)}
*/
default public AbstractCharSequenceAssert, String> assertThat(final String actual) {
return Assertions.assertThat(actual);
}
/**
* Delegate call to {@link com.fitbur.assertj.api.Assertions#assertThat(Date)}
*/
default public AbstractDateAssert> assertThat(final Date actual) {
return Assertions.assertThat(actual);
}
/**
* Delegate call to {@link com.fitbur.assertj.api.Assertions#assertThat(Throwable)}
*/
default public AbstractThrowableAssert, ? extends Throwable> assertThat(final Throwable actual) {
return Assertions.assertThat(actual);
}
/**
* Delegate call to {@link com.fitbur.assertj.api.Assertions#assertThat(BigDecimal)}
*/
default public AbstractBigDecimalAssert> assertThat(final BigDecimal actual) {
return Assertions.assertThat(actual);
}
/**
* Delegate call to {@link com.fitbur.assertj.api.Assertions#assertThat(CharSequence)}
*/
default public AbstractCharSequenceAssert, ? extends CharSequence> assertThat(final CharSequence actual) {
return Assertions.assertThat(actual);
}
/**
* Delegate call to {@link com.fitbur.assertj.api.Assertions#assertThat(short[])}
*/
default public AbstractShortArrayAssert> assertThat(final short[] actual) {
return Assertions.assertThat(actual);
}
/**
* Delegate call to {@link com.fitbur.assertj.api.Assertions#assertThat(Short)}
*/
default public AbstractShortAssert> assertThat(final Short actual) {
return Assertions.assertThat(actual);
}
/**
* Delegate call to {@link com.fitbur.assertj.api.Assertions#assertThat(Class)}
*/
default public AbstractClassAssert> assertThat(final Class> actual) {
return Assertions.assertThat(actual);
}
/**
* Delegate call to {@link com.fitbur.assertj.api.Assertions#assertThat(Character)}
*/
default public AbstractCharacterAssert> assertThat(final Character actual) {
return Assertions.assertThat(actual);
}
/**
* Delegate call to {@link com.fitbur.assertj.api.Assertions#assertThat(char[])}
*/
default public AbstractCharArrayAssert> assertThat(final char[] actual) {
return Assertions.assertThat(actual);
}
/**
* Delegate call to {@link com.fitbur.assertj.api.Assertions#assertThat(char)}
*/
default public AbstractCharacterAssert> assertThat(final char actual) {
return Assertions.assertThat(actual);
}
/**
* Delegate call to {@link com.fitbur.assertj.api.Assertions#assertThat(Comparable)}
*/
default public > AbstractComparableAssert, T> assertThat(final T actual) {
return Assertions.assertThat(actual);
}
/**
* Delegate call to {@link com.fitbur.assertj.api.Assertions#assertThat(Iterable)}
*/
@SuppressWarnings("unchecked")
default public AbstractIterableAssert, ? extends Iterable, T> assertThat(final Iterable actual) {
return (AbstractIterableAssert, ? extends Iterable, T>) Assertions.assertThat(actual);
}
/**
* Delegate call to {@link com.fitbur.assertj.api.Assertions#assertThat(Iterator)}
*/
@SuppressWarnings("unchecked")
default public AbstractIterableAssert, ? extends Iterable, T> assertThat(final Iterator actual) {
return (AbstractIterableAssert, ? extends Iterable, T>) Assertions.assertThat(actual);
}
/**
* Delegate call to {@link com.fitbur.assertj.api.Assertions#assertThat(Boolean)}
*/
default public AbstractBooleanAssert> assertThat(final Boolean actual) {
return Assertions.assertThat(actual);
}
/**
* Delegate call to {@link com.fitbur.assertj.api.Assertions#assertThat(boolean)}
*/
default public AbstractBooleanArrayAssert> assertThat(final boolean[] actual) {
return Assertions.assertThat(actual);
}
/**
* Delegate call to {@link com.fitbur.assertj.api.Assertions#assertThat(byte)}
*/
default public AbstractByteAssert> assertThat(final byte actual) {
return Assertions.assertThat(actual);
}
/**
* Delegate call to {@link com.fitbur.assertj.api.Assertions#assertThat(Byte)}
*/
default public AbstractByteAssert> assertThat(final Byte actual) {
return Assertions.assertThat(actual);
}
/**
* Delegate call to {@link com.fitbur.assertj.api.Assertions#assertThat(byte[])}
*/
default public AbstractByteArrayAssert> assertThat(final byte[] actual) {
return Assertions.assertThat(actual);
}
/**
* Delegate call to {@link com.fitbur.assertj.api.Assertions#assertThat(boolean)}
*/
default public AbstractBooleanAssert> assertThat(final boolean actual) {
return Assertions.assertThat(actual);
}
/**
* Delegate call to {@link com.fitbur.assertj.api.Assertions#assertThat(float)}
*/
default public AbstractFloatAssert> assertThat(final float actual) {
return Assertions.assertThat(actual);
}
/**
* Delegate call to {@link com.fitbur.assertj.api.Assertions#assertThat(InputStream)}
*/
default public AbstractInputStreamAssert, ? extends InputStream> assertThat(final InputStream actual) {
return Assertions.assertThat(actual);
}
/**
* Delegate call to {@link com.fitbur.assertj.api.Assertions#assertThat(File)}
*/
default public AbstractFileAssert> assertThat(final File actual) {
return Assertions.assertThat(actual);
}
/**
* Delegate call to {@link com.fitbur.assertj.api.Assertions#assertThat(int[])}
*/
default public AbstractIntArrayAssert> assertThat(final int[] actual) {
return Assertions.assertThat(actual);
}
/**
* Delegate call to {@link com.fitbur.assertj.api.Assertions#assertThat(Float)}
*/
default public AbstractFloatAssert> assertThat(final Float actual) {
return Assertions.assertThat(actual);
}
/**
* Delegate call to {@link com.fitbur.assertj.api.Assertions#assertThat(int)}
*/
default public AbstractIntegerAssert> assertThat(final int actual) {
return Assertions.assertThat(actual);
}
/**
* Delegate call to {@link com.fitbur.assertj.api.Assertions#assertThat(float[])}
*/
default public AbstractFloatArrayAssert> assertThat(final float[] actual) {
return Assertions.assertThat(actual);
}
/**
* Delegate call to {@link com.fitbur.assertj.api.Assertions#assertThat(Integer)}
*/
default public AbstractIntegerAssert> assertThat(final Integer actual) {
return Assertions.assertThat(actual);
}
/**
* Delegate call to {@link com.fitbur.assertj.api.Assertions#assertThat(double)}
*/
default public AbstractDoubleAssert> assertThat(final double actual) {
return Assertions.assertThat(actual);
}
/**
* Delegate call to {@link com.fitbur.assertj.api.Assertions#assertThat(Double)}
*/
default public AbstractDoubleAssert> assertThat(final Double actual) {
return Assertions.assertThat(actual);
}
/**
* Delegate call to {@link com.fitbur.assertj.api.Assertions#assertThat(List)}
*/
@SuppressWarnings("unchecked")
default public AbstractListAssert, ? extends List, T> assertThat(final List actual) {
return (AbstractListAssert, ? extends List, T>) Assertions.assertThat(actual);
}
/**
* Delegate call to {@link com.fitbur.assertj.api.Assertions#assertThat(List)}
*/
@SuppressWarnings("unchecked")
default public AbstractListAssert, ? extends List extends T>, T> assertThat(final Stream extends T> actual) {
return (AbstractListAssert, ? extends List extends T>, T>) Assertions.assertThat(actual);
}
/**
* Delegate call to {@link com.fitbur.assertj.api.Assertions#assertThat(double[])}
*/
default public AbstractDoubleArrayAssert> assertThat(final double[] actual) {
return Assertions.assertThat(actual);
}
/**
* Delegate call to {@link com.fitbur.assertj.api.Assertions#extractProperty(String)}
*/
default public Properties
© 2015 - 2024 Weber Informatics LLC | Privacy Policy