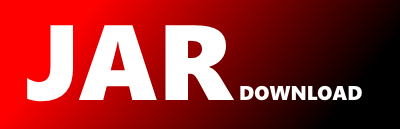
com.fitbur.assertj.extractor.ByNameMultipleExtractor Maven / Gradle / Ivy
/**
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
* Copyright 2012-2016 the original author or authors.
*/
package com.fitbur.assertj.extractor;
import java.util.ArrayList;
import java.util.List;
import com.fitbur.assertj.api.iterable.Extractor;
import com.fitbur.assertj.groups.Tuple;
class ByNameMultipleExtractor implements Extractor{
private final String[] fieldsOrProperties;
ByNameMultipleExtractor(String... fieldsOrProperties) {
this.fieldsOrProperties = fieldsOrProperties;
}
@Override
public Tuple extract(T input) {
if (fieldsOrProperties == null)
throw new IllegalArgumentException("The names of the fields/properties to read should not be null");
if (fieldsOrProperties.length == 0)
throw new IllegalArgumentException("The names of the fields/properties to read should not be empty");
if (input == null)
throw new IllegalArgumentException("The object to extract fields/properties from should not be null");
List> extractors = buildExtractors();
List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy