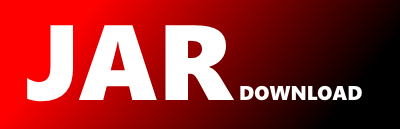
com.fitbur.assertj.groups.FieldsOrPropertiesExtractor Maven / Gradle / Ivy
/**
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
* Copyright 2012-2016 the original author or authors.
*/
package com.fitbur.assertj.groups;
import static com.fitbur.assertj.util.IterableUtil.toArray;
import static com.fitbur.assertj.util.Lists.newArrayList;
import java.util.List;
import com.fitbur.assertj.api.AbstractIterableAssert;
import com.fitbur.assertj.api.AbstractObjectArrayAssert;
import com.fitbur.assertj.api.iterable.Extractor;
import com.fitbur.assertj.util.Lists;
/**
*
* Understands how to retrieve fields or values from a collection/array of objects.
*
* You just have to give the field/property name or an {@link Extractor} implementation, a collection/array of objects
* and it will extract the list of field/values from the given objects.
*
* @author Joel Costigliola
* @author Mateusz Haligowski
*
*/
public class FieldsOrPropertiesExtractor {
/**
* Call {@link #extract(Iterable, Extractor)} after converting objects to an iterable.
*
* Behavior is described in javadoc {@link AbstractObjectArrayAssert#extracting(Extractor)}
*/
public static T[] extract(F[] objects, Extractor super F, T> extractor) {
List result = extract(newArrayList(objects), extractor);
return toArray(result);
}
/**
* Behavior is described in {@link AbstractIterableAssert#extracting(Extractor)}
*/
public static List extract(Iterable extends F> objects, Extractor super F, T> extractor) {
List result = Lists.newArrayList();
for (F object : objects) {
final T newValue = extractor.extract(object);
result.add(newValue);
}
return result;
}
}