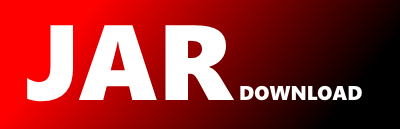
com.fitbur.assertj.groups.Properties Maven / Gradle / Ivy
/**
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
* Copyright 2012-2016 the original author or authors.
*/
package com.fitbur.assertj.groups;
import static com.fitbur.assertj.util.Preconditions.checkNotNull;
import static com.fitbur.assertj.util.ArrayWrapperList.wrap;
import java.util.List;
import com.fitbur.assertj.util.VisibleForTesting;
import com.fitbur.assertj.util.introspection.IntrospectionError;
import com.fitbur.assertj.util.introspection.PropertySupport;
/**
* Extracts the values of a specified property from the elements of a given {@link Iterable}
or array.
*
* @author Yvonne Wang
* @author Mikhail Mazursky
* @author Joel Costigliola
* @author Florent Biville
* @author Olivier Michallat
*/
public class Properties {
@VisibleForTesting
final String propertyName;
final Class propertyType;
@VisibleForTesting
PropertySupport propertySupport = PropertySupport.instance();
/**
* Creates a new {@link Properties}
.
* @param propertyName the name of the property to be read from the elements of a {@code Iterable}. It may be a nested
* property (e.g. "address.street.number").
* @param propertyType the type of property to extract
* @throws NullPointerException if the given property name is {@code null}.
* @throws IllegalArgumentException if the given property name is empty.
* @return the created {@code Properties}.
*/
public static Properties extractProperty(String propertyName, Class propertyType) {
checkIsNotNullOrEmpty(propertyName);
return new Properties<>(propertyName, propertyType);
}
/**
* Creates a new {@link Properties} with given propertyName and Object as property type.
.
* @param propertyName the name of the property to be read from the elements of a {@code Iterable}. It may be a nested
* property (e.g. "address.street.number").
* @throws NullPointerException if the given property name is {@code null}.
* @throws IllegalArgumentException if the given property name is empty.
* @return the created {@code Properties}.
*/
public static Properties
© 2015 - 2024 Weber Informatics LLC | Privacy Policy