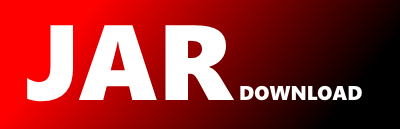
com.fitbur.assertj.internal.ComparatorBasedComparisonStrategy Maven / Gradle / Ivy
/** * Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with * the License. You may obtain a copy of the License at * * http://www.apache.org/licenses/LICENSE-2.0 * * Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on * an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the * specific language governing permissions and limitations under the License. * * Copyright 2012-2016 the original author or authors. */ package com.fitbur.assertj.internal; import static com.fitbur.assertj.util.IterableUtil.isNullOrEmpty; import java.util.Comparator; import java.util.Iterator; import java.util.Set; import java.util.TreeSet; import com.fitbur.assertj.presentation.StandardRepresentation; /** * Implements {@link ComparisonStrategy} contract with a comparison strategy based on a {@link Comparator}. * * @author Joel Costigliola */ public class ComparatorBasedComparisonStrategy extends AbstractComparisonStrategy { // stateless => can be shared private static final StandardRepresentation STANDARD_REPRESENTATION = new StandardRepresentation(); // A raw type is necessary because we can't make assumptions on object to be compared. @SuppressWarnings("rawtypes") private final Comparator comparator; /** * Creates a new
{@link ComparatorBasedComparisonStrategy} specifying the comparison strategy with given * comparator. * * @param comparator the comparison strategy to use. */ public ComparatorBasedComparisonStrategy(@SuppressWarnings("rawtypes") Comparator comparator) { this.comparator = comparator; } /** * Returns true if given {@link Iterable} contains given value according to {@link #comparator}, false otherwise.
* If given {@link Iterable} is null or empty, return false. * * @param iterable the {@link Iterable} to search value in * @param value the object to look for in given {@link Iterable} * @return true if given {@link Iterable} contains given value according to {@link #comparator}, false otherwise. */ @Override @SuppressWarnings("unchecked") public boolean iterableContains(Iterable> iterable, Object value) { if (isNullOrEmpty(iterable)) return false; for (Object element : iterable) { // avoid comparison when objects are the same or both null if (element == value) return true; // both objects are not null => if one is then the other is not => compare next element with value if (value == null || element == null) continue; if (comparator.compare(element, value) == 0) return true; } return false; } /** * Look for given value in given {@link Iterable} according to the {@link Comparator}, if value is found it is removed * from it.
* Does nothing if given {@link Iterable} is null (meaning no exception thrown). * * @param iterable the {@link Iterable} we want remove value from * @param value object to remove from given {@link Iterable} */ @Override @SuppressWarnings("unchecked") public void iterableRemoves(Iterable> iterable, Object value) { if (iterable == null) return; Iterator> iterator = iterable.iterator(); while (iterator.hasNext()) { if (comparator.compare(iterator.next(), value) == 0) { iterator.remove(); } } } @Override @SuppressWarnings("unchecked") public void iterablesRemoveFirst(Iterable> iterable, Object value) { if (iterable == null) return; Iterator> iterator = iterable.iterator(); while (iterator.hasNext()) { if (comparator.compare(iterator.next(), value) == 0) { iterator.remove(); return; } } } /** * Returns true if actual and other are equal according to {@link #comparator}, false otherwise.
* Handles the cases where one of the parameter is null so that internal {@link #comparator} does not have too. * * @param actual the object to compare to other * @param other the object to compare to actual * @return true if actual and other are equal according to {@link #comparator}, false otherwise. */ @Override @SuppressWarnings("unchecked") public boolean areEqual(Object actual, Object other) { if (actual == null) return other == null; // actual is not null if (other == null) return false; // neither actual nor other are null return comparator.compare(actual, other) == 0; } /** * Returns any duplicate elements from the given {@link Iterable} according to {@link #comparator}. * * @param iterable the given {@link Iterable} we want to extract duplicate elements. * @return an {@link Iterable} containing the duplicate elements of the given one. If no duplicates are found, an * empty {@link Iterable} is returned. */ // overridden to write javadoc. @Override public Iterable> duplicatesFrom(Iterable> iterable) { return super.duplicatesFrom(iterable); } @SuppressWarnings("unchecked") @Override protected Set
© 2015 - 2024 Weber Informatics LLC | Privacy Policy