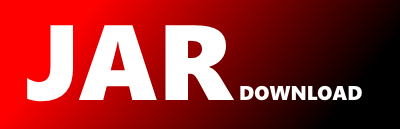
com.fitbur.assertj.internal.Conditions Maven / Gradle / Ivy
/**
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
* Copyright 2012-2016 the original author or authors.
*/
package com.fitbur.assertj.internal;
import static com.fitbur.assertj.error.ShouldBe.shouldBe;
import static com.fitbur.assertj.error.ShouldHave.shouldHave;
import static com.fitbur.assertj.error.ShouldNotBe.shouldNotBe;
import static com.fitbur.assertj.error.ShouldNotHave.shouldNotHave;
import static com.fitbur.assertj.util.Preconditions.checkNotNull;
import com.fitbur.assertj.api.AssertionInfo;
import com.fitbur.assertj.api.Condition;
import com.fitbur.assertj.util.VisibleForTesting;
/**
* Verifies that a value satisfies a {@link Condition}
.
*
* @author Alex Ruiz
*/
public class Conditions {
private static final Conditions INSTANCE = new Conditions();
/**
* Returns the singleton instance of this class.
* @return the singleton instance of this class.
*/
public static Conditions instance() {
return INSTANCE;
}
@VisibleForTesting
Failures failures = Failures.instance();
@VisibleForTesting
Conditions() {}
/**
* Asserts that the actual value satisfies the given {@link Condition}
.
* @param the type of the actual value and the type of values that given {@code Condition} takes.
* @param info contains information about the assertion.
* @param actual the actual value.
* @param condition the given {@code Condition}.
* @throws NullPointerException if the given {@code Condition} is {@code null}.
* @throws AssertionError if the actual value does not satisfy the given {@code Condition}.
*/
public void assertIs(AssertionInfo info, T actual, Condition super T> condition) {
assertIsNotNull(condition);
if (condition.matches(actual)) return;
throw failures.failure(info, shouldBe(actual, condition));
}
/**
* Asserts that the actual value does not satisfy the given {@link Condition}
.
* @param the type of the actual value and the type of values that given {@code Condition} takes.
* @param info contains information about the assertion.
* @param actual the actual value.
* @param condition the given {@code Condition}.
* @throws NullPointerException if the given {@code Condition} is {@code null}.
* @throws AssertionError if the actual value satisfies the given {@code Condition}.
*/
public void assertIsNot(AssertionInfo info, T actual, Condition super T> condition) {
assertIsNotNull(condition);
if (!condition.matches(actual)) return;
throw failures.failure(info, shouldNotBe(actual, condition));
}
/**
* Asserts that the actual value satisfies the given {@link Condition}
.
* @param the type of the actual value and the type of values that given {@code Condition} takes.
* @param info contains information about the assertion.
* @param actual the actual value.
* @param condition the given {@code Condition}.
* @throws NullPointerException if the given {@code Condition} is {@code null}.
* @throws AssertionError if the actual value does not satisfy the given {@code Condition}.
*/
public void assertHas(AssertionInfo info, T actual, Condition super T> condition) {
assertIsNotNull(condition);
if (condition.matches(actual)) return;
throw failures.failure(info, shouldHave(actual, condition));
}
/**
* Asserts that the actual value does not satisfy the given {@link Condition}
.
* @param the type of the actual value and the type of values that given {@code Condition} takes.
* @param info contains information about the assertion.
* @param actual the actual value.
* @param condition the given {@code Condition}.
* @throws NullPointerException if the given {@code Condition} is {@code null}.
* @throws AssertionError if the actual value satisfies the given {@code Condition}.
*/
public void assertDoesNotHave(AssertionInfo info, T actual, Condition super T> condition) {
assertIsNotNull(condition);
if (!condition.matches(actual)) return;
throw failures.failure(info, shouldNotHave(actual, condition));
}
/**
* Asserts the the given {@link Condition}
is not null.
* @param condition the given {@code Condition}.
* @throws NullPointerException if the given {@code Condition} is {@code null}.
*/
public void assertIsNotNull(Condition> condition) {
checkNotNull(condition, "The condition to evaluate should not be null");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy