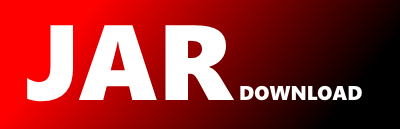
com.fitbur.assertj.internal.Lists Maven / Gradle / Ivy
/**
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
* Copyright 2012-2016 the original author or authors.
*/
package com.fitbur.assertj.internal;
import static com.fitbur.assertj.error.ShouldBeAtIndex.shouldBeAtIndex;
import static com.fitbur.assertj.error.ShouldBeSorted.shouldBeSorted;
import static com.fitbur.assertj.error.ShouldBeSorted.shouldBeSortedAccordingToGivenComparator;
import static com.fitbur.assertj.error.ShouldBeSorted.shouldHaveComparableElementsAccordingToGivenComparator;
import static com.fitbur.assertj.error.ShouldBeSorted.shouldHaveMutuallyComparableElements;
import static com.fitbur.assertj.error.ShouldContainAtIndex.shouldContainAtIndex;
import static com.fitbur.assertj.error.ShouldHaveAtIndex.shouldHaveAtIndex;
import static com.fitbur.assertj.error.ShouldNotContainAtIndex.shouldNotContainAtIndex;
import static com.fitbur.assertj.internal.CommonValidations.checkIndexValueIsValid;
import static com.fitbur.assertj.util.Preconditions.checkNotNull;
import java.util.ArrayList;
import java.util.Comparator;
import java.util.List;
import com.fitbur.assertj.api.AssertionInfo;
import com.fitbur.assertj.api.Condition;
import com.fitbur.assertj.data.Index;
import com.fitbur.assertj.util.VisibleForTesting;
/**
* Reusable assertions for {@link List}
s.
*
* @author Alex Ruiz
* @author Yvonne Wang
* @author Joel Costigliola
*/
// TODO inherits from Collections to avoid repeating comparisonStrategy ?
public class Lists {
private static final Lists INSTANCE = new Lists();
/**
* Returns the singleton instance of this class.
* @return the singleton instance of this class.
*/
public static Lists instance() {
return INSTANCE;
}
private final ComparisonStrategy comparisonStrategy;
@VisibleForTesting
Failures failures = Failures.instance();
@VisibleForTesting
Lists() {
this(StandardComparisonStrategy.instance());
}
public Lists(ComparisonStrategy comparisonStrategy) {
this.comparisonStrategy = comparisonStrategy;
}
@VisibleForTesting
public Comparator> getComparator() {
if (comparisonStrategy instanceof ComparatorBasedComparisonStrategy) { return ((ComparatorBasedComparisonStrategy) comparisonStrategy)
.getComparator(); }
return null;
}
/**
* Verifies that the given {@code List} contains the given object at the given index.
* @param info contains information about the assertion.
* @param actual the given {@code List}.
* @param value the object to look for.
* @param index the index where the object should be stored in the given {@code List}.
* @throws AssertionError if the given {@code List} is {@code null} or empty.
* @throws NullPointerException if the given {@code Index} is {@code null}.
* @throws IndexOutOfBoundsException if the value of the given {@code Index} is equal to or greater than the size of the given
* {@code List}.
* @throws AssertionError if the given {@code List} does not contain the given object at the given index.
*/
public void assertContains(AssertionInfo info, List> actual, Object value, Index index) {
assertNotNull(info, actual);
Iterables.instance().assertNotEmpty(info, actual);
checkIndexValueIsValid(index, actual.size() - 1);
Object actualElement = actual.get(index.value);
if (areEqual(actualElement, value)) return;
throw failures.failure(info, shouldContainAtIndex(actual, value, index, actual.get(index.value), comparisonStrategy));
}
/**
* Verifies that the given {@code List} does not contain the given object at the given index.
* @param info contains information about the assertion.
* @param actual the given {@code List}.
* @param value the object to look for.
* @param index the index where the object should be stored in the given {@code List}.
* @throws AssertionError if the given {@code List} is {@code null}.
* @throws NullPointerException if the given {@code Index} is {@code null}.
* @throws AssertionError if the given {@code List} contains the given object at the given index.
*/
public void assertDoesNotContain(AssertionInfo info, List> actual, Object value, Index index) {
assertNotNull(info, actual);
checkIndexValueIsValid(index, Integer.MAX_VALUE);
int indexValue = index.value;
if (indexValue >= actual.size()) return;
Object actualElement = actual.get(index.value);
if (!areEqual(actualElement, value)) return;
throw failures.failure(info, shouldNotContainAtIndex(actual, value, index, comparisonStrategy));
}
/**
* Verifies that the actual list is sorted into ascending order according to the natural ordering of its elements.
*
* All list elements must implement the {@link Comparable} interface and must be mutually comparable (that is, e1.compareTo(e2)
* must not throw a ClassCastException for any elements e1 and e2 in the list), examples :
*
* - a list composed of {"a1", "a2", "a3"} is ok because the element type (String) is Comparable
* - a list composed of Rectangle {r1, r2, r3} is NOT ok because Rectangle is not Comparable
* - a list composed of {True, "abc", False} is NOT ok because elements are not mutually comparable
*
* Empty lists are considered sorted. Unique element lists are considered sorted unless the element type is not Comparable.
*
* @param info contains information about the assertion.
* @param actual the given {@code List}.
*
* @throws AssertionError if the actual list is not sorted into ascending order according to the natural ordering of its
* elements.
* @throws AssertionError if the actual list is null
.
* @throws AssertionError if the actual list element type does not implement {@link Comparable}.
* @throws AssertionError if the actual list elements are not mutually {@link Comparable}.
*/
public void assertIsSorted(AssertionInfo info, List> actual) {
assertNotNull(info, actual);
if (comparisonStrategy instanceof ComparatorBasedComparisonStrategy) {
// instead of comparing elements with their natural comparator, use the one set by client.
Comparator> comparator = ((ComparatorBasedComparisonStrategy) comparisonStrategy).getComparator();
assertIsSortedAccordingToComparator(info, actual, comparator);
return;
}
try {
// sorted assertion is only relevant if elements are Comparable, we assume they are
List> comparableList = listOfComparableElements(actual);
// array with 0 or 1 element are considered sorted.
if (comparableList.size() <= 1) return;
for (int i = 0; i < comparableList.size() - 1; i++) {
// array is sorted in ascending order iif element i is less or equal than element i+1
if (comparableList.get(i).compareTo(comparableList.get(i + 1)) > 0)
throw failures.failure(info, shouldBeSorted(i, actual));
}
} catch (ClassCastException e) {
// elements are either not Comparable or not mutually Comparable (e.g. List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy