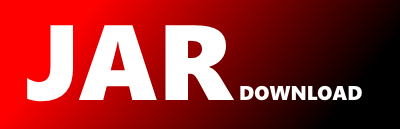
com.fitbur.assertj.util.Arrays Maven / Gradle / Ivy
/**
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
* Copyright 2012-2016 the original author or authors.
*/
package com.fitbur.assertj.util;
import static java.lang.reflect.Array.getLength;
import static java.util.Collections.emptyList;
import static com.fitbur.assertj.util.Preconditions.checkNotNull;
import java.lang.reflect.Array;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import com.fitbur.assertj.presentation.Representation;
/**
* Utility methods related to arrays.
*
* @author Alex Ruiz
* @author Joel Costigliola
*/
public class Arrays extends GroupFormatUtil {
private static final String NULL = "null";
/**
* Indicates whether the given object is not {@code null} and is an array.
*
* @param o the given object.
* @return {@code true} if the given object is not {@code null} and is an array, otherwise {@code false}.
*/
public static boolean isArray(Object o) {
return o != null && o.getClass().isArray();
}
/**
* Indicates whether the given array is {@code null} or empty.
*
* @param the type of elements of the array.
* @param array the array to check.
* @return {@code true} if the given array is {@code null} or empty, otherwise {@code false}.
*/
public static boolean isNullOrEmpty(T[] array) {
return array == null || isEmpty(array);
}
/**
* Returns an array containing the given arguments.
*
* @param the type of the array to return.
* @param values the values to store in the array.
* @return an array containing the given arguments.
*/
@SafeVarargs
public static T[] array(T... values) {
return values;
}
/**
* Returns all the non-{@code null} elements in the given array.
*
* @param the type of elements of the array.
* @param array the given array.
* @return all the non-{@code null} elements in the given array. An empty list is returned if the given array is
* {@code null}.
*/
public static List nonNullElementsIn(T[] array) {
if (array == null) return emptyList();
List nonNullElements = new ArrayList<>();
for (T o : array) {
if (o != null) nonNullElements.add(o);
}
return nonNullElements;
}
/**
* Returns {@code true} if the given array has only {@code null} elements, {@code false} otherwise. If given array is
* empty, this method returns {@code true}.
*
* @param the type of elements of the array.
* @param array the given array. It must not be null.
* @return {@code true} if the given array has only {@code null} elements or is empty, {@code false} otherwise.
* @throws NullPointerException if the given array is {@code null}.
*/
public static boolean hasOnlyNullElements(T[] array) {
checkNotNull(array);
if (isEmpty(array)) return false;
for (T o : array) {
if (o != null) return false;
}
return true;
}
/**
* Returns the {@code String} representation of the given array, or {@code null} if the given object is either
* {@code null} or not an array. This method supports arrays having other arrays as elements.
*
* @param representation
* @param array the object that is expected to be an array.
* @return the {@code String} representation of the given array.
*/
public static String format(Representation representation, Object o) {
if (!isArray(o)) return null;
return isObjectArray(o) ? smartFormat(representation, (Object[]) o) : formatPrimitiveArray(representation, o);
}
private static boolean isEmpty(T[] array) {
return array.length == 0;
}
private static boolean isObjectArray(Object o) {
return isArray(o) && !isArrayTypePrimitive(o);
}
private static boolean isArrayTypePrimitive(Object o) {
return o != null && o.getClass().getComponentType().isPrimitive();
}
static IllegalArgumentException notAnArrayOfPrimitives(Object o) {
return new IllegalArgumentException(String.format("<%s> is not an array of primitives", o));
}
private static String smartFormat(Representation representation, Object[] iterable) {
Set
© 2015 - 2024 Weber Informatics LLC | Privacy Policy