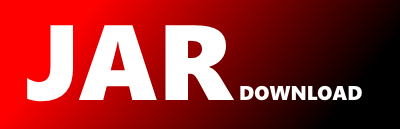
com.fitbur.assertj.util.Throwables Maven / Gradle / Ivy
/**
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
* Copyright 2012-2016 the original author or authors.
*/
package com.fitbur.assertj.util;
import static com.fitbur.assertj.util.Lists.newArrayList;
import java.io.PrintWriter;
import java.io.StringWriter;
import java.util.*;
/**
* Utility methods related to {@link Throwable}
s.
*
* @author Alex Ruiz
*/
public final class Throwables {
private static final String ORG_ASSERTJ_CORE_ERROR_CONSTRUCTOR_INVOKER = "com.fitbur.assertj.error.ConstructorInvoker";
private static final String JAVA_LANG_REFLECT_CONSTRUCTOR = "java.lang.reflect.Constructor";
private static final String ORG_ASSERTJ = "org.assert";
/**
* Appends the stack trace of the current thread to the one in the given {@link Throwable}
.
*
* @param t the given {@code Throwable}.
* @param methodToStartFrom the name of the method used as the starting point of the current thread's stack trace.
*/
public static void appendStackTraceInCurentThreadToThrowable(Throwable t, String methodToStartFrom) {
List stackTrace = newArrayList(t.getStackTrace());
stackTrace.addAll(stackTraceInCurrentThread(methodToStartFrom));
t.setStackTrace(stackTrace.toArray(new StackTraceElement[stackTrace.size()]));
}
private static List stackTraceInCurrentThread(String methodToStartFrom) {
List filtered = stackTraceInCurrentThread();
List toRemove = new ArrayList<>();
for (StackTraceElement e : filtered) {
if (methodToStartFrom.equals(e.getMethodName())) {
break;
}
toRemove.add(e);
}
filtered.removeAll(toRemove);
return filtered;
}
private static List stackTraceInCurrentThread() {
return newArrayList(Thread.currentThread().getStackTrace());
}
/**
* Removes the AssertJ-related elements from the {@link Throwable}
stack trace that have little value for
* end user. Therefore, instead of seeing this:
* org.junit.ComparisonFailure: expected:<'[Ronaldo]'> but was:<'[Messi]'>
* at sun.reflect.NativeConstructorAccessorImpl.newInstance0(Native Method)
* at sun.reflect.NativeConstructorAccessorImpl.newInstance(NativeConstructorAccessorImpl.java:39)
* at sun.reflect.DelegatingConstructorAccessorImpl.newInstance(DelegatingConstructorAccessorImpl.java:27)
* at java.lang.reflect.Constructor.newInstance(Constructor.java:501)
* at com.fitbur.assertj.error.ConstructorInvoker.newInstance(ConstructorInvoker.java:34)
* at com.fitbur.assertj.error.ShouldBeEqual.newComparisonFailure(ShouldBeEqual.java:111)
* at com.fitbur.assertj.error.ShouldBeEqual.comparisonFailure(ShouldBeEqual.java:103)
* at com.fitbur.assertj.error.ShouldBeEqual.newAssertionError(ShouldBeEqual.java:81)
* at com.fitbur.assertj.internal.Failures.failure(Failures.java:76)
* at com.fitbur.assertj.internal.Objects.assertEqual(Objects.java:116)
* at com.fitbur.assertj.api.AbstractAssert.isEqualTo(AbstractAssert.java:74)
* at examples.StackTraceFilterExample.main(StackTraceFilterExample.java:13)
*
* We get this:
* org.junit.ComparisonFailure: expected:<'[Ronaldo]'> but was:<'[Messi]'>
* at sun.reflect.NativeConstructorAccessorImpl.newInstance0(Native Method)
* at sun.reflect.NativeConstructorAccessorImpl.newInstance(NativeConstructorAccessorImpl.java:39)
* at sun.reflect.DelegatingConstructorAccessorImpl.newInstance(DelegatingConstructorAccessorImpl.java:27)
* at examples.StackTraceFilterExample.main(StackTraceFilterExample.java:20)
* @param throwable the {@code Throwable} to filter stack trace.
*/
public static void removeAssertJRelatedElementsFromStackTrace(Throwable throwable) {
List filtered = newArrayList(throwable.getStackTrace());
StackTraceElement previous = null;
for (StackTraceElement element : throwable.getStackTrace()) {
if (element.getClassName().contains(ORG_ASSERTJ)) {
filtered.remove(element);
// Handle the case when AssertJ builds a ComparisonFailure by reflection (see ShouldBeEqual.newAssertionError
// method), the stack trace looks like:
//
// java.lang.reflect.Constructor.newInstance(Constructor.java:501),
// com.fitbur.assertj.error.ConstructorInvoker.newInstance(ConstructorInvoker.java:34),
//
// We want to remove java.lang.reflect.Constructor.newInstance element because it is related to AssertJ.
if (previous != null && JAVA_LANG_REFLECT_CONSTRUCTOR.equals(previous.getClassName())
&& element.getClassName().contains(ORG_ASSERTJ_CORE_ERROR_CONSTRUCTOR_INVOKER)) {
filtered.remove(previous);
}
}
previous = element;
}
StackTraceElement[] newStackTrace = filtered.toArray(new StackTraceElement[filtered.size()]);
throwable.setStackTrace(newStackTrace);
}
/**
* Get the root cause (ie the last non null cause) from a {@link Throwable}.
*
* @param throwable the {@code Throwable} to get root cause from.
* @return the root cause if any, else {@code null}.
*/
public static Throwable getRootCause(Throwable throwable) {
if (throwable.getCause() == null) return null;
Throwable cause;
while ((cause = throwable.getCause()) != null) throwable = cause;
return throwable;
}
/**
* Get the stack trace from a {@link Throwable} as a {@link String}.
*
*
* The result of this method vary by JDK version as this method uses
* {@link Throwable#printStackTrace(java.io.PrintWriter)}. On JDK1.3 and earlier, the cause exception will not be
* shown unless the specified throwable alters printStackTrace.
*
*
* @param throwable the {@code Throwable} to get stack trace from.
* @return the stack trace as a {@link String}.
*
* @author Daniel Zlotin
*/
public static String getStackTrace(Throwable throwable) {
StringWriter sw = null;
PrintWriter pw = null;
try {
sw = new StringWriter();
pw = new PrintWriter(sw, true);
throwable.printStackTrace(pw);
return sw.getBuffer().toString();
} finally {
Closeables.closeQuietly(sw, pw);
}
}
private Throwables() {}
}