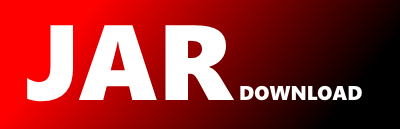
com.fitbur.assertj.util.diff.Delta Maven / Gradle / Ivy
/**
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
* Copyright 2012-2016 the original author or authors.
*/
package com.fitbur.assertj.util.diff;
import static com.fitbur.assertj.util.GroupFormatUtil.DEFAULT_END;
import static com.fitbur.assertj.util.GroupFormatUtil.DEFAULT_START;
import static com.fitbur.assertj.util.GroupFormatUtil.ELEMENT_SEPARATOR_WITH_NEWLINE;
import java.util.List;
import com.fitbur.assertj.presentation.StandardRepresentation;
import com.fitbur.assertj.util.IterableUtil;
/**
* Initially copied from https://code.google.com/p/java-diff-utils/.
*
* Describes the delta between original and revised texts.
*
* @author Dmitry Naumenko
* @param The type of the compared elements in the 'lines'.
*/
public abstract class Delta {
/** The original chunk. */
private Chunk original;
/** The revised chunk. */
private Chunk revised;
/**
* Specifies the type of the delta.
*
*/
public enum TYPE {
/** A change in the original. */
CHANGE,
/** A delete from the original. */
DELETE,
/** An insert into the original. */
INSERT
}
/**
* Construct the delta for original and revised chunks
*
* @param original Chunk describing the original text. Must not be {@code null}.
* @param revised Chunk describing the revised text. Must not be {@code null}.
*/
public Delta(Chunk original, Chunk revised) {
if (original == null) {
throw new IllegalArgumentException("original must not be null");
}
if (revised == null) {
throw new IllegalArgumentException("revised must not be null");
}
this.original = original;
this.revised = revised;
}
/**
* Verifies that this delta can be used to patch the given text.
*
* @param target the text to patch.
* @throws IllegalStateException if the patch cannot be applied.
*/
public abstract void verify(List target) throws IllegalStateException;
/**
* Applies this delta as the patch for a given target
*
* @param target the given target
* @throws IllegalStateException
*/
public abstract void applyTo(List target) throws IllegalStateException;
/**
* Returns the type of delta
* @return the type enum
*/
public abstract TYPE getType();
/**
* @return The Chunk describing the original text.
*/
public Chunk getOriginal() {
return original;
}
/**
* @param original The Chunk describing the original text to set.
*/
public void setOriginal(Chunk original) {
this.original = original;
}
/**
* @return The Chunk describing the revised text.
*/
public Chunk getRevised() {
return revised;
}
/**
* @param revised The Chunk describing the revised text to set.
*/
public void setRevised(Chunk revised) {
this.revised = revised;
}
@Override
public int hashCode() {
final int prime = 31;
int result = 1;
result = prime * result + ((original == null) ? 0 : original.hashCode());
result = prime * result + ((revised == null) ? 0 : revised.hashCode());
return result;
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (getClass() != obj.getClass())
return false;
@SuppressWarnings("rawtypes")
Delta other = (Delta) obj;
if (original == null) {
if (other.original != null)
return false;
} else if (!original.equals(other.original))
return false;
if (revised == null) {
if (other.revised != null)
return false;
} else if (!revised.equals(other.revised))
return false;
return true;
}
int lineNumber() {
return getOriginal().getPosition() + 1;
}
String formatLines(List lines) {
return IterableUtil.format(StandardRepresentation.STANDARD_REPRESENTATION, lines, DEFAULT_START, DEFAULT_END,
ELEMENT_SEPARATOR_WITH_NEWLINE, " ");
}
}