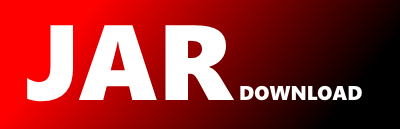
com.fitbur.mockito.internal.stubbing.answers.AnswerFunctionalInterfaces Maven / Gradle / Ivy
package com.fitbur.mockito.internal.stubbing.answers;
import com.fitbur.mockito.invocation.InvocationOnMock;
import com.fitbur.mockito.stubbing.Answer;
/**
* Functional interfaces to make it easy to implement answers in Java 8
*/
public class AnswerFunctionalInterfaces {
/**
* Hide constructor to avoid instantiation of class with only static methods
*/
private AnswerFunctionalInterfaces() {
}
/**
* Construct an answer from a two parameter answer interface
* @param answer answer interface
* @param return type
* @param input parameter 1 type
* @return a new answer object
*/
public static Answer toAnswer(final Answer1 answer) {
return new Answer() {
@SuppressWarnings("unchecked")
public T answer(InvocationOnMock invocation) throws Throwable {
return answer.answer((A)invocation.getArgument(0));
}
};
}
/**
* Construct an answer from a two parameter answer interface
* @param answer answer interface
* @param input parameter 1 type
* @return a new answer object
*/
public static Answer toAnswer(final VoidAnswer1 answer) {
return new Answer() {
@SuppressWarnings("unchecked")
public Void answer(InvocationOnMock invocation) throws Throwable {
answer.answer((A)invocation.getArgument(0));
return null;
}
};
}
/**
* Construct an answer from a two parameter answer interface
* @param answer answer interface
* @param return type
* @param input parameter 1 type
* @param input parameter 2 type
* @return a new answer object
*/
public static Answer toAnswer(final Answer2 answer) {
return new Answer() {
@SuppressWarnings("unchecked")
public T answer(InvocationOnMock invocation) throws Throwable {
return answer.answer(
(A)invocation.getArgument(0),
(B)invocation.getArgument(1));
}
};
}
/**
* Construct an answer from a two parameter answer interface
* @param answer answer interface
* @param input parameter 1 type
* @param input parameter 2 type
* @return a new answer object
*/
public static Answer toAnswer(final VoidAnswer2 answer) {
return new Answer() {
@SuppressWarnings("unchecked")
public Void answer(InvocationOnMock invocation) throws Throwable {
answer.answer(
(A)invocation.getArgument(0),
(B)invocation.getArgument(1));
return null;
}
};
}
/**
* Construct an answer from a three parameter answer interface
* @param answer answer interface
* @param return type
* @param input parameter 1 type
* @param input parameter 2 type
* @param input parameter 3 type
* @return a new answer object
*/
public static Answer toAnswer(final Answer3 answer) {
return new Answer() {
@SuppressWarnings("unchecked")
public T answer(InvocationOnMock invocation) throws Throwable {
return answer.answer(
(A)invocation.getArgument(0),
(B)invocation.getArgument(1),
(C)invocation.getArgument(2));
}
};
}
/**
* Construct an answer from a three parameter answer interface
* @param answer answer interface
* @param input parameter 1 type
* @param input parameter 2 type
* @param input parameter 3 type
* @return a new answer object
*/
public static Answer toAnswer(final VoidAnswer3 answer) {
return new Answer() {
@SuppressWarnings("unchecked")
public Void answer(InvocationOnMock invocation) throws Throwable {
answer.answer(
(A)invocation.getArgument(0),
(B)invocation.getArgument(1),
(C)invocation.getArgument(2));
return null;
}
};
}
/**
* Construct an answer from a four parameter answer interface
* @param answer answer interface
* @param return type
* @param input parameter 1 type
* @param input parameter 2 type
* @param input parameter 3 type
* @param input parameter 4 type
* @return a new answer object
*/
public static Answer toAnswer(final Answer4 answer) {
return new Answer() {
@SuppressWarnings("unchecked")
public T answer(InvocationOnMock invocation) throws Throwable {
return answer.answer(
(A)invocation.getArgument(0),
(B)invocation.getArgument(1),
(C)invocation.getArgument(2),
(D)invocation.getArgument(3));
}
};
}
/**
* Construct an answer from a four parameter answer interface
* @param answer answer interface
* @param input parameter 1 type
* @param input parameter 2 type
* @param input parameter 3 type
* @param input parameter 4 type
* @return a new answer object
*/
public static Answer toAnswer(final VoidAnswer4 answer) {
return new Answer() {
@SuppressWarnings("unchecked")
public Void answer(InvocationOnMock invocation) throws Throwable {
answer.answer(
(A)invocation.getArgument(0),
(B)invocation.getArgument(1),
(C)invocation.getArgument(2),
(D)invocation.getArgument(3));
return null;
}
};
}
/**
* Construct an answer from a five parameter answer interface
* @param answer answer interface
* @param return type
* @param input parameter 1 type
* @param input parameter 2 type
* @param input parameter 3 type
* @param input parameter 4 type
* @param input parameter 5 type
* @return a new answer object
*/
public static Answer toAnswer(final Answer5 answer) {
return new Answer() {
@SuppressWarnings("unchecked")
public T answer(InvocationOnMock invocation) throws Throwable {
return answer.answer(
(A)invocation.getArgument(0),
(B)invocation.getArgument(1),
(C)invocation.getArgument(2),
(D)invocation.getArgument(3),
(E)invocation.getArgument(4));
}
};
}
/**
* Construct an answer from a five parameter answer interface
* @param answer answer interface
* @param input parameter 1 type
* @param input parameter 2 type
* @param input parameter 3 type
* @param input parameter 4 type
* @param input parameter 5 type
* @return a new answer object
*/
public static Answer toAnswer(final VoidAnswer5 answer) {
return new Answer() {
@SuppressWarnings("unchecked")
public Void answer(InvocationOnMock invocation) throws Throwable {
answer.answer(
(A)invocation.getArgument(0),
(B)invocation.getArgument(1),
(C)invocation.getArgument(2),
(D)invocation.getArgument(3),
(E)invocation.getArgument(4));
return null;
}
};
}
/**
* One parameter function which returns something
* @param return type
* @param input parameter 1 type
*/
public interface Answer1 {
T answer(A a);
}
/**
* One parameter void function
* @param input parameter 1 type
*/
public interface VoidAnswer1 {
void answer(A a);
}
/**
* Two parameter function which returns something
* @param return type
* @param input parameter 1 type
* @param input parameter 2 type
*/
public interface Answer2 {
T answer(A a, B b);
}
/**
* Two parameter void function
* @param input parameter 1 type
* @param input parameter 2 type
*/
public interface VoidAnswer2< A, B> {
void answer(A a, B b);
}
/**
* Three parameter function which returns something
* @param return type
* @param input parameter 1 type
* @param input parameter 2 type
* @param input parameter 3 type
*/
public interface Answer3 {
T answer(A a, B b, C c);
}
/**
* Two parameter void function
* @param input parameter 1 type
* @param input parameter 2 type
* @param input parameter 3 type
*/
public interface VoidAnswer3< A, B, C> {
void answer(A a, B b, C c);
}
/**
* Three parameter function which returns something
* @param return type
* @param input parameter 1 type
* @param input parameter 2 type
* @param input parameter 3 type
* @param input parameter 4 type
*/
public interface Answer4 {
T answer(A a, B b, C c, D d);
}
/**
* Two parameter void function
* @param input parameter 1 type
* @param input parameter 2 type
* @param input parameter 3 type
* @param input parameter 4 type
*/
public interface VoidAnswer4 {
void answer(A a, B b, C c, D d);
}
/**
* Three parameter function which returns something
* @param return type
* @param input parameter 1 type
* @param input parameter 2 type
* @param input parameter 3 type
* @param input parameter 4 type
* @param input parameter 5 type
*/
public interface Answer5 {
T answer(A a, B b, C c, D d, E e);
}
/**
* Two parameter void function
* @param input parameter 1 type
* @param input parameter 2 type
* @param input parameter 3 type
* @param input parameter 4 type
* @param input parameter 5 type
*/
public interface VoidAnswer5< A, B, C, D, E> {
void answer(A a, B b, C c, D d, E e);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy