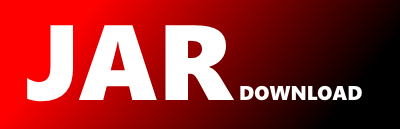
com.fitbur.mockito.internal.util.MockUtil Maven / Gradle / Ivy
/*
* Copyright (c) 2007 Mockito contributors
* This program is made available under the terms of the MIT License.
*/
package com.fitbur.mockito.internal.util;
import static com.fitbur.mockito.internal.handler.MockHandlerFactory.createMockHandler;
import com.fitbur.mockito.Mockito;
import com.fitbur.mockito.exceptions.misusing.NotAMockException;
import com.fitbur.mockito.internal.InternalMockHandler;
import com.fitbur.mockito.internal.configuration.plugins.Plugins;
import com.fitbur.mockito.internal.creation.settings.CreationSettings;
import com.fitbur.mockito.internal.util.reflection.LenientCopyTool;
import com.fitbur.mockito.invocation.MockHandler;
import com.fitbur.mockito.mock.MockCreationSettings;
import com.fitbur.mockito.mock.MockName;
import com.fitbur.mockito.plugins.MockMaker;
import com.fitbur.mockito.plugins.MockMaker.TypeMockability;
@SuppressWarnings("unchecked")
public class MockUtil {
private static final MockMaker mockMaker = Plugins.getMockMaker();
public TypeMockability typeMockabilityOf(Class> type) {
return mockMaker.isTypeMockable(type);
}
public T createMock(MockCreationSettings settings) {
MockHandler mockHandler = createMockHandler(settings);
T mock = mockMaker.createMock(settings, mockHandler);
Object spiedInstance = settings.getSpiedInstance();
if (spiedInstance != null) {
new LenientCopyTool().copyToMock(spiedInstance, mock);
}
return mock;
}
public void resetMock(T mock) {
InternalMockHandler oldHandler = (InternalMockHandler) getMockHandler(mock);
MockCreationSettings settings = oldHandler.getMockSettings();
MockHandler newHandler = createMockHandler(settings);
mockMaker.resetMock(mock, newHandler, settings);
}
public InternalMockHandler getMockHandler(T mock) {
if (mock == null) {
throw new NotAMockException("Argument should be a mock, but is null!");
}
if (isMockitoMock(mock)) {
MockHandler handler = mockMaker.getHandler(mock);
return (InternalMockHandler) handler;
} else {
throw new NotAMockException("Argument should be a mock, but is: " + mock.getClass());
}
}
public boolean isMock(Object mock) {
// double check to avoid classes that have the same interfaces, could be great to have a custom mockito field in the proxy instead of relying on instance fields
return isMockitoMock(mock);
}
public boolean isSpy(Object mock) {
return isMockitoMock(mock) && getMockSettings(mock).getDefaultAnswer() == Mockito.CALLS_REAL_METHODS;
}
private boolean isMockitoMock(T mock) {
return mock != null && mockMaker.getHandler(mock) != null;
}
public MockName getMockName(Object mock) {
return getMockHandler(mock).getMockSettings().getMockName();
}
public void maybeRedefineMockName(Object mock, String newName) {
MockName mockName = getMockName(mock);
//TODO SF hacky...
MockCreationSettings mockSettings = getMockHandler(mock).getMockSettings();
if (mockName.isDefault() && mockSettings instanceof CreationSettings) {
((CreationSettings) mockSettings).setMockName(new MockNameImpl(newName));
}
}
public MockCreationSettings getMockSettings(Object mock) {
return getMockHandler(mock).getMockSettings();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy