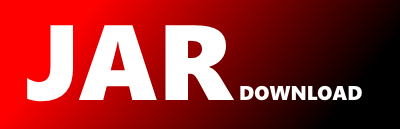
com.fizzed.bigmap.impl.AbstractBigMap Maven / Gradle / Ivy
/*
* Copyright 2019 Fizzed, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.fizzed.bigmap.impl;
import com.fizzed.bigmap.*;
import java.io.Closeable;
import java.io.IOException;
import java.io.UncheckedIOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.util.Comparator;
import java.util.Objects;
import java.util.UUID;
abstract public class AbstractBigMap implements BigMap {
protected final UUID id;
protected final Path directory;
protected final boolean persistent;
protected final ByteCodec keyCodec;
protected final Comparator keyComparator;
protected final ByteCodec valueCodec;
protected int size;
protected long keyByteSize;
protected long valueByteSize;
protected BigObjectListener listener;
protected BigObjectCloser closer;
public AbstractBigMap(
UUID id,
Path directory,
boolean persistent,
ByteCodec keyCodec,
Comparator keyComparator,
ByteCodec valueCodec) {
Objects.requireNonNull(id, "id was null");
Objects.requireNonNull(directory, "directory was null");
Objects.requireNonNull(keyCodec, "keyCodec was null");
Objects.requireNonNull(keyComparator, "keyComparator was null");
this.id = id;
this.directory = directory;
this.persistent = persistent;
this.keyCodec = keyCodec;
this.keyComparator = keyComparator;
this.valueCodec = valueCodec;
}
@Override
public BigObjectListener getListener() {
return this.listener;
}
@Override
public void setListener(BigObjectListener listener) {
this.listener = listener;
}
@Override
public UUID getId() { return this.id; }
@Override
public Path getDirectory() {
return this.directory;
}
@Override
public boolean isPersistent() {
return this.persistent;
}
@Override
final public boolean isClosed() {
return this.closer == null || this.closer.isClosed();
}
@Override
public ByteCodec getKeyCodec() {
return this.keyCodec;
}
@Override
public Comparator getKeyComparator() {
return this.keyComparator;
}
@Override
public ByteCodec getValueCodec() {
return this.valueCodec;
}
@Override
public long getKeyByteSize() {
return this.keyByteSize;
}
@Override
public long getValueByteSize() {
return this.valueByteSize;
}
@Override
public int size() {
return this.size;
}
@Override
public void open() {
try {
this.close();
} catch (IOException e) {
// do nothing
}
try {
if (this.directory != null) {
Files.createDirectories(this.directory.toAbsolutePath());
}
this._open();
this.size = 0;
this.keyByteSize = 0L;
this.valueByteSize = 0L;
} catch (Exception e) {
throw new BigMapDataException(e);
}
if (this.listener != null) {
this.listener.onOpened(this);
}
}
abstract protected void _open();
// protected void loadCounts() throws IOException {
// try (DBIterator it = this.db.iterator()) {
// it.seekToFirst();
// while (it.hasNext()) {
// Entry entry = it.next();
// this.size++;
// this.keyByteSize += entry.getKey().length;
// this.valueByteSize += entry.getValue().length;
// }
// }
// }
/*@Override
protected void finalize() throws Throwable {
super.finalize();
if (this.listener == null && !this.isClosed()) {
System.out.println("UH OH - BigMap @ " + this.directory + " was not closed and is being garbage collected!");
}
}*/
@Override
final public BigObjectCloser getCloser() {
return this.closer;
}
@Override
final public void close() throws IOException {
if (this.closer != null) {
this.closer.close();
this.closer = null;
}
if (this.listener != null) {
this.listener.onClosed(this);
}
}
@Override
public void clear() {
try {
this.close();
this.open();
} catch (IOException e) {
throw new UncheckedIOException(e);
}
}
public void _entryAdded(long keyByteSize, long valueByteSize) {
this.size++;
this.keyByteSize += keyByteSize;
this.valueByteSize += valueByteSize;
}
public void _entryRemoved(long keyByteSize, long valueByteSize) {
this.size--;
this.keyByteSize -= keyByteSize;
this.valueByteSize -= valueByteSize;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy