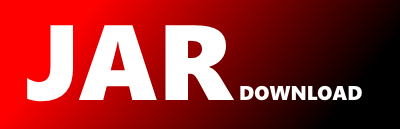
com.fizzed.crux.util.Agg Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2020 Fizzed, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.fizzed.crux.util;
import java.util.Comparator;
import java.util.Objects;
/**
* Aggregates (e.g. min/max), with null-safety!
*
* @author jjlauer
*/
public class Agg {
static public class OrderedValue {
protected final Comparator super T> comparator;
protected T value;
protected OrderedValue(Comparator super T> comparator, T initialValue) {
this.comparator = comparator;
this.value = initialValue;
}
public boolean isAbsent() {
return this.value == null;
}
public boolean isPresent() {
return this.value != null;
}
public Maybe maybe() {
return Maybe.of(this.value);
}
/**
* Clears the current value by setting it to null.
*/
public void clear() {
this.value = null;
}
/**
* Directly sets the current value.
* @param value
*/
public void set(T value) {
this.value = value;
}
/**
* Gets the current value.
*
* @return
*/
public T get() {
return value;
}
/**
* Null-safe apply a new value, calculate the agg (e.g. min or max), and
* returns the new value. Nulls are skipped for evaluation!
*
* @param newValue
* @return True if modified, otherwise false.
*/
public boolean apply(T newValue) {
return this.apply(newValue, false);
}
/**
* Apply a new value, calculate the agg, and returns the new value. If
* you do includeNull, you MUST provide a null-safe comparator otherwise
* you will get a runtime NullPointerException.
*
* @param newValue
* @param includeNulls
* @return True if modified, otherwise false.
*/
public boolean apply(T newValue, boolean includeNulls) {
if (includeNulls || newValue != null) {
if (!includeNulls && this.value == null) {
if (newValue != null) {
this.value = newValue;
return true;
} else {
return false;
}
} else {
int c = this.comparator.compare(this.value, newValue);
if (c > 0) {
this.value = newValue;
return true;
}
}
}
return false;
}
}
static public class Min extends OrderedValue {
protected Min(Comparator super T> comparator, T initialValue) {
super(comparator, initialValue);
}
}
static public class Max extends OrderedValue {
protected Max(Comparator super T> comparator, T initialValue) {
super(comparator, initialValue);
}
}
/**
* Helps aggregate the min value according to natural ordering of the type.
*
* @param
* @return
*/
static public Min min() {
final Comparator comparator = Comparator.naturalOrder();
return min(comparator, null);
}
/**
* Helps aggregate the min value according to natural ordering of the type.
*
* @param
* @param type
* @return
*/
static public Min min(Class type) {
final Comparator comparator = Comparator.naturalOrder();
return min(comparator, null);
}
/**
* Helps aggregate the min value according to the natural ordering of the type
* of initial value.
*
* @param
* @param initialValue
* @return
*/
static public Min min(V initialValue) {
final Comparator comparator = Comparator.naturalOrder();
return min(comparator, initialValue);
}
/**
* Helps aggregate the min value. Comparator is the natural ordering
* of values such that 1 < 2.
*
* @param
* @param comparator
* @return
*/
static public Min min(Comparator comparator) {
return min(comparator, null);
}
/**
* Helps aggregate the min value. Comparator is the natural ordering
* of values such that 1 < 2.
*
* @param
* @param comparator
* @param initialValue
* @return
*/
static public Min min(Comparator comparator, V initialValue) {
return new Min(comparator, initialValue);
}
/**
* Helps aggregate the max value according to natural ordering of the type.
*
* @param
* @return
*/
static public Max max() {
final Comparator comparator = Comparator.naturalOrder();
return max(comparator, null);
}
/**
* Helps aggregate the max value according to natural ordering of the type.
*
* @param
* @param type
* @return
*/
static public Max max(Class type) {
final Comparator comparator = Comparator.naturalOrder();
return max(comparator, null);
}
/**
* Helps aggregate the max value according to the natural ordering of the type
* of initial value.
*
* @param
* @param initialValue
* @return
*/
static public Max max(V initialValue) {
final Comparator comparator = Comparator.naturalOrder();
return max(comparator, initialValue);
}
/**
* Helps aggregate the max value. Comparator is the natural ordering
* of values such that 1 < 2.
*
* @param
* @param comparator
* @return
*/
static public Max max(Comparator comparator) {
return max(comparator, null);
}
/**
* Helps aggregate the max value. Comparator is the natural ordering
* of values such that 1 < 2.
*
* @param
* @param comparator
* @param initialValue
* @return
*/
static public Max max(Comparator comparator, V initialValue) {
Objects.requireNonNull(comparator, "comparator was null");
return new Max(comparator.reversed(), initialValue);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy