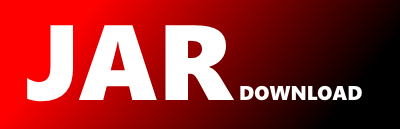
com.fizzed.rocker.antlr4.RockerParser Maven / Gradle / Ivy
// Generated from com/fizzed/rocker/antlr4/RockerParser.g4 by ANTLR 4.5.3
package com.fizzed.rocker.antlr4;
import org.antlr.v4.runtime.atn.*;
import org.antlr.v4.runtime.dfa.DFA;
import org.antlr.v4.runtime.*;
import org.antlr.v4.runtime.misc.*;
import org.antlr.v4.runtime.tree.*;
import java.util.List;
import java.util.Iterator;
import java.util.ArrayList;
@SuppressWarnings({"all", "warnings", "unchecked", "unused", "cast"})
public class RockerParser extends Parser {
static { RuntimeMetaData.checkVersion("4.5.3", RuntimeMetaData.VERSION); }
protected static final DFA[] _decisionToDFA;
protected static final PredictionContextCache _sharedContextCache =
new PredictionContextCache();
public static final int
ELSE_IF=1, ELSE=2, CASE=3, DEFAULT=4, LCURLY=5, RCURLY=6, COMMENT=7, PLAIN=8,
AT=9, MV_IMPORT=10, MV_OPTION=11, MV_ARGS=12, MV_IF=13, MV_SWITCH=14,
MV_FOR=15, MV_WITH=16, MV_CONTENT_CLOSURE=17, MV_VALUE_CLOSURE=18, MV_EVAL=19,
MV_NULL_TERNARY_LH=20, MV_VALUE=21, MV_NULL_TERNARY_RH=22;
public static final int
RULE_template = 0, RULE_plain = 1, RULE_plainBlock = 2, RULE_plainElseIfBlock = 3,
RULE_plainElseBlock = 4, RULE_comment = 5, RULE_importDeclaration = 6,
RULE_importStatement = 7, RULE_optionDeclaration = 8, RULE_optionStatement = 9,
RULE_argumentsDeclaration = 10, RULE_argumentsStatement = 11, RULE_templateContent = 12,
RULE_block = 13, RULE_ifBlock = 14, RULE_ifElseIfBlock = 15, RULE_ifElseBlock = 16,
RULE_forBlock = 17, RULE_withBlock = 18, RULE_withElseBlock = 19, RULE_contentClosure = 20,
RULE_contentClosureExpression = 21, RULE_valueClosure = 22, RULE_valueClosureExpression = 23,
RULE_value = 24, RULE_valueExpression = 25, RULE_nullTernary = 26, RULE_nullTernaryExpression = 27,
RULE_eval = 28, RULE_evalExpression = 29, RULE_switchBlock = 30, RULE_switchCase = 31,
RULE_switchDefault = 32;
public static final String[] ruleNames = {
"template", "plain", "plainBlock", "plainElseIfBlock", "plainElseBlock",
"comment", "importDeclaration", "importStatement", "optionDeclaration",
"optionStatement", "argumentsDeclaration", "argumentsStatement", "templateContent",
"block", "ifBlock", "ifElseIfBlock", "ifElseBlock", "forBlock", "withBlock",
"withElseBlock", "contentClosure", "contentClosureExpression", "valueClosure",
"valueClosureExpression", "value", "valueExpression", "nullTernary", "nullTernaryExpression",
"eval", "evalExpression", "switchBlock", "switchCase", "switchDefault"
};
private static final String[] _LITERAL_NAMES = {
null, null, null, null, null, "'{'", "'}'", null, null, "'@'"
};
private static final String[] _SYMBOLIC_NAMES = {
null, "ELSE_IF", "ELSE", "CASE", "DEFAULT", "LCURLY", "RCURLY", "COMMENT",
"PLAIN", "AT", "MV_IMPORT", "MV_OPTION", "MV_ARGS", "MV_IF", "MV_SWITCH",
"MV_FOR", "MV_WITH", "MV_CONTENT_CLOSURE", "MV_VALUE_CLOSURE", "MV_EVAL",
"MV_NULL_TERNARY_LH", "MV_VALUE", "MV_NULL_TERNARY_RH"
};
public static final Vocabulary VOCABULARY = new VocabularyImpl(_LITERAL_NAMES, _SYMBOLIC_NAMES);
/**
* @deprecated Use {@link #VOCABULARY} instead.
*/
@Deprecated
public static final String[] tokenNames;
static {
tokenNames = new String[_SYMBOLIC_NAMES.length];
for (int i = 0; i < tokenNames.length; i++) {
tokenNames[i] = VOCABULARY.getLiteralName(i);
if (tokenNames[i] == null) {
tokenNames[i] = VOCABULARY.getSymbolicName(i);
}
if (tokenNames[i] == null) {
tokenNames[i] = "";
}
}
}
@Override
@Deprecated
public String[] getTokenNames() {
return tokenNames;
}
@Override
public Vocabulary getVocabulary() {
return VOCABULARY;
}
@Override
public String getGrammarFileName() { return "RockerParser.g4"; }
@Override
public String[] getRuleNames() { return ruleNames; }
@Override
public String getSerializedATN() { return _serializedATN; }
@Override
public ATN getATN() { return _ATN; }
public RockerParser(TokenStream input) {
super(input);
_interp = new ParserATNSimulator(this,_ATN,_decisionToDFA,_sharedContextCache);
}
public static class TemplateContext extends ParserRuleContext {
public List plain() {
return getRuleContexts(PlainContext.class);
}
public PlainContext plain(int i) {
return getRuleContext(PlainContext.class,i);
}
public List comment() {
return getRuleContexts(CommentContext.class);
}
public CommentContext comment(int i) {
return getRuleContext(CommentContext.class,i);
}
public List importDeclaration() {
return getRuleContexts(ImportDeclarationContext.class);
}
public ImportDeclarationContext importDeclaration(int i) {
return getRuleContext(ImportDeclarationContext.class,i);
}
public List optionDeclaration() {
return getRuleContexts(OptionDeclarationContext.class);
}
public OptionDeclarationContext optionDeclaration(int i) {
return getRuleContext(OptionDeclarationContext.class,i);
}
public ArgumentsDeclarationContext argumentsDeclaration() {
return getRuleContext(ArgumentsDeclarationContext.class,0);
}
public List templateContent() {
return getRuleContexts(TemplateContentContext.class);
}
public TemplateContentContext templateContent(int i) {
return getRuleContext(TemplateContentContext.class,i);
}
public TemplateContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_template; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).enterTemplate(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).exitTemplate(this);
}
}
public final TemplateContext template() throws RecognitionException {
TemplateContext _localctx = new TemplateContext(_ctx, getState());
enterRule(_localctx, 0, RULE_template);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(72);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,1,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
setState(70);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,0,_ctx) ) {
case 1:
{
setState(66);
plain();
}
break;
case 2:
{
setState(67);
comment();
}
break;
case 3:
{
setState(68);
importDeclaration();
}
break;
case 4:
{
setState(69);
optionDeclaration();
}
break;
}
}
}
setState(74);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,1,_ctx);
}
setState(76);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,2,_ctx) ) {
case 1:
{
setState(75);
argumentsDeclaration();
}
break;
}
setState(81);
_errHandler.sync(this);
_la = _input.LA(1);
while ((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << LCURLY) | (1L << COMMENT) | (1L << PLAIN) | (1L << AT))) != 0)) {
{
{
setState(78);
templateContent();
}
}
setState(83);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class PlainContext extends ParserRuleContext {
public TerminalNode PLAIN() { return getToken(RockerParser.PLAIN, 0); }
public PlainBlockContext plainBlock() {
return getRuleContext(PlainBlockContext.class,0);
}
public PlainContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_plain; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).enterPlain(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).exitPlain(this);
}
}
public final PlainContext plain() throws RecognitionException {
PlainContext _localctx = new PlainContext(_ctx, getState());
enterRule(_localctx, 2, RULE_plain);
try {
enterOuterAlt(_localctx, 1);
{
setState(86);
switch (_input.LA(1)) {
case PLAIN:
{
setState(84);
match(PLAIN);
}
break;
case LCURLY:
{
setState(85);
plainBlock();
}
break;
default:
throw new NoViableAltException(this);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class PlainBlockContext extends ParserRuleContext {
public TerminalNode LCURLY() { return getToken(RockerParser.LCURLY, 0); }
public TerminalNode RCURLY() { return getToken(RockerParser.RCURLY, 0); }
public PlainElseBlockContext plainElseBlock() {
return getRuleContext(PlainElseBlockContext.class,0);
}
public List templateContent() {
return getRuleContexts(TemplateContentContext.class);
}
public TemplateContentContext templateContent(int i) {
return getRuleContext(TemplateContentContext.class,i);
}
public List plainElseIfBlock() {
return getRuleContexts(PlainElseIfBlockContext.class);
}
public PlainElseIfBlockContext plainElseIfBlock(int i) {
return getRuleContext(PlainElseIfBlockContext.class,i);
}
public PlainBlockContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_plainBlock; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).enterPlainBlock(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).exitPlainBlock(this);
}
}
public final PlainBlockContext plainBlock() throws RecognitionException {
PlainBlockContext _localctx = new PlainBlockContext(_ctx, getState());
enterRule(_localctx, 4, RULE_plainBlock);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(88);
match(LCURLY);
setState(92);
_errHandler.sync(this);
_la = _input.LA(1);
while ((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << LCURLY) | (1L << COMMENT) | (1L << PLAIN) | (1L << AT))) != 0)) {
{
{
setState(89);
templateContent();
}
}
setState(94);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(98);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==ELSE_IF) {
{
{
setState(95);
plainElseIfBlock();
}
}
setState(100);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(103);
switch (_input.LA(1)) {
case RCURLY:
{
setState(101);
match(RCURLY);
}
break;
case ELSE:
{
setState(102);
plainElseBlock();
}
break;
default:
throw new NoViableAltException(this);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class PlainElseIfBlockContext extends ParserRuleContext {
public TerminalNode ELSE_IF() { return getToken(RockerParser.ELSE_IF, 0); }
public List templateContent() {
return getRuleContexts(TemplateContentContext.class);
}
public TemplateContentContext templateContent(int i) {
return getRuleContext(TemplateContentContext.class,i);
}
public PlainElseIfBlockContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_plainElseIfBlock; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).enterPlainElseIfBlock(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).exitPlainElseIfBlock(this);
}
}
public final PlainElseIfBlockContext plainElseIfBlock() throws RecognitionException {
PlainElseIfBlockContext _localctx = new PlainElseIfBlockContext(_ctx, getState());
enterRule(_localctx, 6, RULE_plainElseIfBlock);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(105);
match(ELSE_IF);
setState(109);
_errHandler.sync(this);
_la = _input.LA(1);
while ((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << LCURLY) | (1L << COMMENT) | (1L << PLAIN) | (1L << AT))) != 0)) {
{
{
setState(106);
templateContent();
}
}
setState(111);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class PlainElseBlockContext extends ParserRuleContext {
public TerminalNode ELSE() { return getToken(RockerParser.ELSE, 0); }
public TerminalNode RCURLY() { return getToken(RockerParser.RCURLY, 0); }
public List templateContent() {
return getRuleContexts(TemplateContentContext.class);
}
public TemplateContentContext templateContent(int i) {
return getRuleContext(TemplateContentContext.class,i);
}
public PlainElseBlockContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_plainElseBlock; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).enterPlainElseBlock(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).exitPlainElseBlock(this);
}
}
public final PlainElseBlockContext plainElseBlock() throws RecognitionException {
PlainElseBlockContext _localctx = new PlainElseBlockContext(_ctx, getState());
enterRule(_localctx, 8, RULE_plainElseBlock);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(112);
match(ELSE);
setState(116);
_errHandler.sync(this);
_la = _input.LA(1);
while ((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << LCURLY) | (1L << COMMENT) | (1L << PLAIN) | (1L << AT))) != 0)) {
{
{
setState(113);
templateContent();
}
}
setState(118);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(119);
match(RCURLY);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class CommentContext extends ParserRuleContext {
public TerminalNode COMMENT() { return getToken(RockerParser.COMMENT, 0); }
public CommentContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_comment; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).enterComment(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).exitComment(this);
}
}
public final CommentContext comment() throws RecognitionException {
CommentContext _localctx = new CommentContext(_ctx, getState());
enterRule(_localctx, 10, RULE_comment);
try {
enterOuterAlt(_localctx, 1);
{
setState(121);
match(COMMENT);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ImportDeclarationContext extends ParserRuleContext {
public TerminalNode AT() { return getToken(RockerParser.AT, 0); }
public ImportStatementContext importStatement() {
return getRuleContext(ImportStatementContext.class,0);
}
public ImportDeclarationContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_importDeclaration; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).enterImportDeclaration(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).exitImportDeclaration(this);
}
}
public final ImportDeclarationContext importDeclaration() throws RecognitionException {
ImportDeclarationContext _localctx = new ImportDeclarationContext(_ctx, getState());
enterRule(_localctx, 12, RULE_importDeclaration);
try {
enterOuterAlt(_localctx, 1);
{
setState(123);
match(AT);
setState(124);
importStatement();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ImportStatementContext extends ParserRuleContext {
public TerminalNode MV_IMPORT() { return getToken(RockerParser.MV_IMPORT, 0); }
public ImportStatementContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_importStatement; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).enterImportStatement(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).exitImportStatement(this);
}
}
public final ImportStatementContext importStatement() throws RecognitionException {
ImportStatementContext _localctx = new ImportStatementContext(_ctx, getState());
enterRule(_localctx, 14, RULE_importStatement);
try {
enterOuterAlt(_localctx, 1);
{
setState(126);
match(MV_IMPORT);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class OptionDeclarationContext extends ParserRuleContext {
public TerminalNode AT() { return getToken(RockerParser.AT, 0); }
public OptionStatementContext optionStatement() {
return getRuleContext(OptionStatementContext.class,0);
}
public OptionDeclarationContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_optionDeclaration; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).enterOptionDeclaration(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).exitOptionDeclaration(this);
}
}
public final OptionDeclarationContext optionDeclaration() throws RecognitionException {
OptionDeclarationContext _localctx = new OptionDeclarationContext(_ctx, getState());
enterRule(_localctx, 16, RULE_optionDeclaration);
try {
enterOuterAlt(_localctx, 1);
{
setState(128);
match(AT);
setState(129);
optionStatement();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class OptionStatementContext extends ParserRuleContext {
public TerminalNode MV_OPTION() { return getToken(RockerParser.MV_OPTION, 0); }
public OptionStatementContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_optionStatement; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).enterOptionStatement(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).exitOptionStatement(this);
}
}
public final OptionStatementContext optionStatement() throws RecognitionException {
OptionStatementContext _localctx = new OptionStatementContext(_ctx, getState());
enterRule(_localctx, 18, RULE_optionStatement);
try {
enterOuterAlt(_localctx, 1);
{
setState(131);
match(MV_OPTION);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ArgumentsDeclarationContext extends ParserRuleContext {
public TerminalNode AT() { return getToken(RockerParser.AT, 0); }
public ArgumentsStatementContext argumentsStatement() {
return getRuleContext(ArgumentsStatementContext.class,0);
}
public ArgumentsDeclarationContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_argumentsDeclaration; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).enterArgumentsDeclaration(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).exitArgumentsDeclaration(this);
}
}
public final ArgumentsDeclarationContext argumentsDeclaration() throws RecognitionException {
ArgumentsDeclarationContext _localctx = new ArgumentsDeclarationContext(_ctx, getState());
enterRule(_localctx, 20, RULE_argumentsDeclaration);
try {
enterOuterAlt(_localctx, 1);
{
setState(133);
match(AT);
setState(134);
argumentsStatement();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ArgumentsStatementContext extends ParserRuleContext {
public TerminalNode MV_ARGS() { return getToken(RockerParser.MV_ARGS, 0); }
public ArgumentsStatementContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_argumentsStatement; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).enterArgumentsStatement(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).exitArgumentsStatement(this);
}
}
public final ArgumentsStatementContext argumentsStatement() throws RecognitionException {
ArgumentsStatementContext _localctx = new ArgumentsStatementContext(_ctx, getState());
enterRule(_localctx, 22, RULE_argumentsStatement);
try {
enterOuterAlt(_localctx, 1);
{
setState(136);
match(MV_ARGS);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class TemplateContentContext extends ParserRuleContext {
public CommentContext comment() {
return getRuleContext(CommentContext.class,0);
}
public BlockContext block() {
return getRuleContext(BlockContext.class,0);
}
public PlainContext plain() {
return getRuleContext(PlainContext.class,0);
}
public ContentClosureContext contentClosure() {
return getRuleContext(ContentClosureContext.class,0);
}
public ValueClosureContext valueClosure() {
return getRuleContext(ValueClosureContext.class,0);
}
public ValueContext value() {
return getRuleContext(ValueContext.class,0);
}
public NullTernaryContext nullTernary() {
return getRuleContext(NullTernaryContext.class,0);
}
public EvalContext eval() {
return getRuleContext(EvalContext.class,0);
}
public TemplateContentContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_templateContent; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).enterTemplateContent(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).exitTemplateContent(this);
}
}
public final TemplateContentContext templateContent() throws RecognitionException {
TemplateContentContext _localctx = new TemplateContentContext(_ctx, getState());
enterRule(_localctx, 24, RULE_templateContent);
try {
enterOuterAlt(_localctx, 1);
{
setState(146);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,10,_ctx) ) {
case 1:
{
setState(138);
comment();
}
break;
case 2:
{
setState(139);
block();
}
break;
case 3:
{
setState(140);
plain();
}
break;
case 4:
{
setState(141);
contentClosure();
}
break;
case 5:
{
setState(142);
valueClosure();
}
break;
case 6:
{
setState(143);
value();
}
break;
case 7:
{
setState(144);
nullTernary();
}
break;
case 8:
{
setState(145);
eval();
}
break;
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class BlockContext extends ParserRuleContext {
public IfBlockContext ifBlock() {
return getRuleContext(IfBlockContext.class,0);
}
public ForBlockContext forBlock() {
return getRuleContext(ForBlockContext.class,0);
}
public WithBlockContext withBlock() {
return getRuleContext(WithBlockContext.class,0);
}
public SwitchBlockContext switchBlock() {
return getRuleContext(SwitchBlockContext.class,0);
}
public BlockContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_block; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).enterBlock(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).exitBlock(this);
}
}
public final BlockContext block() throws RecognitionException {
BlockContext _localctx = new BlockContext(_ctx, getState());
enterRule(_localctx, 26, RULE_block);
try {
enterOuterAlt(_localctx, 1);
{
setState(152);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,11,_ctx) ) {
case 1:
{
setState(148);
ifBlock();
}
break;
case 2:
{
setState(149);
forBlock();
}
break;
case 3:
{
setState(150);
withBlock();
}
break;
case 4:
{
setState(151);
switchBlock();
}
break;
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class IfBlockContext extends ParserRuleContext {
public TerminalNode AT() { return getToken(RockerParser.AT, 0); }
public TerminalNode MV_IF() { return getToken(RockerParser.MV_IF, 0); }
public TerminalNode RCURLY() { return getToken(RockerParser.RCURLY, 0); }
public IfElseIfBlockContext ifElseIfBlock() {
return getRuleContext(IfElseIfBlockContext.class,0);
}
public IfElseBlockContext ifElseBlock() {
return getRuleContext(IfElseBlockContext.class,0);
}
public List templateContent() {
return getRuleContexts(TemplateContentContext.class);
}
public TemplateContentContext templateContent(int i) {
return getRuleContext(TemplateContentContext.class,i);
}
public IfBlockContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_ifBlock; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).enterIfBlock(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).exitIfBlock(this);
}
}
public final IfBlockContext ifBlock() throws RecognitionException {
IfBlockContext _localctx = new IfBlockContext(_ctx, getState());
enterRule(_localctx, 28, RULE_ifBlock);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(154);
match(AT);
setState(155);
match(MV_IF);
setState(159);
_errHandler.sync(this);
_la = _input.LA(1);
while ((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << LCURLY) | (1L << COMMENT) | (1L << PLAIN) | (1L << AT))) != 0)) {
{
{
setState(156);
templateContent();
}
}
setState(161);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(165);
switch (_input.LA(1)) {
case RCURLY:
{
setState(162);
match(RCURLY);
}
break;
case ELSE_IF:
{
setState(163);
ifElseIfBlock();
}
break;
case ELSE:
{
setState(164);
ifElseBlock();
}
break;
default:
throw new NoViableAltException(this);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class IfElseIfBlockContext extends ParserRuleContext {
public TerminalNode ELSE_IF() { return getToken(RockerParser.ELSE_IF, 0); }
public TerminalNode RCURLY() { return getToken(RockerParser.RCURLY, 0); }
public IfElseIfBlockContext ifElseIfBlock() {
return getRuleContext(IfElseIfBlockContext.class,0);
}
public IfElseBlockContext ifElseBlock() {
return getRuleContext(IfElseBlockContext.class,0);
}
public List templateContent() {
return getRuleContexts(TemplateContentContext.class);
}
public TemplateContentContext templateContent(int i) {
return getRuleContext(TemplateContentContext.class,i);
}
public IfElseIfBlockContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_ifElseIfBlock; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).enterIfElseIfBlock(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).exitIfElseIfBlock(this);
}
}
public final IfElseIfBlockContext ifElseIfBlock() throws RecognitionException {
IfElseIfBlockContext _localctx = new IfElseIfBlockContext(_ctx, getState());
enterRule(_localctx, 30, RULE_ifElseIfBlock);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(167);
match(ELSE_IF);
setState(171);
_errHandler.sync(this);
_la = _input.LA(1);
while ((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << LCURLY) | (1L << COMMENT) | (1L << PLAIN) | (1L << AT))) != 0)) {
{
{
setState(168);
templateContent();
}
}
setState(173);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(177);
switch (_input.LA(1)) {
case RCURLY:
{
setState(174);
match(RCURLY);
}
break;
case ELSE_IF:
{
setState(175);
ifElseIfBlock();
}
break;
case ELSE:
{
setState(176);
ifElseBlock();
}
break;
default:
throw new NoViableAltException(this);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class IfElseBlockContext extends ParserRuleContext {
public TerminalNode ELSE() { return getToken(RockerParser.ELSE, 0); }
public TerminalNode RCURLY() { return getToken(RockerParser.RCURLY, 0); }
public List templateContent() {
return getRuleContexts(TemplateContentContext.class);
}
public TemplateContentContext templateContent(int i) {
return getRuleContext(TemplateContentContext.class,i);
}
public IfElseBlockContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_ifElseBlock; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).enterIfElseBlock(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).exitIfElseBlock(this);
}
}
public final IfElseBlockContext ifElseBlock() throws RecognitionException {
IfElseBlockContext _localctx = new IfElseBlockContext(_ctx, getState());
enterRule(_localctx, 32, RULE_ifElseBlock);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(179);
match(ELSE);
setState(183);
_errHandler.sync(this);
_la = _input.LA(1);
while ((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << LCURLY) | (1L << COMMENT) | (1L << PLAIN) | (1L << AT))) != 0)) {
{
{
setState(180);
templateContent();
}
}
setState(185);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(186);
match(RCURLY);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ForBlockContext extends ParserRuleContext {
public TerminalNode AT() { return getToken(RockerParser.AT, 0); }
public TerminalNode MV_FOR() { return getToken(RockerParser.MV_FOR, 0); }
public TerminalNode RCURLY() { return getToken(RockerParser.RCURLY, 0); }
public List templateContent() {
return getRuleContexts(TemplateContentContext.class);
}
public TemplateContentContext templateContent(int i) {
return getRuleContext(TemplateContentContext.class,i);
}
public ForBlockContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_forBlock; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).enterForBlock(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).exitForBlock(this);
}
}
public final ForBlockContext forBlock() throws RecognitionException {
ForBlockContext _localctx = new ForBlockContext(_ctx, getState());
enterRule(_localctx, 34, RULE_forBlock);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(188);
match(AT);
setState(189);
match(MV_FOR);
setState(193);
_errHandler.sync(this);
_la = _input.LA(1);
while ((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << LCURLY) | (1L << COMMENT) | (1L << PLAIN) | (1L << AT))) != 0)) {
{
{
setState(190);
templateContent();
}
}
setState(195);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(196);
match(RCURLY);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class WithBlockContext extends ParserRuleContext {
public TerminalNode AT() { return getToken(RockerParser.AT, 0); }
public TerminalNode MV_WITH() { return getToken(RockerParser.MV_WITH, 0); }
public TerminalNode RCURLY() { return getToken(RockerParser.RCURLY, 0); }
public WithElseBlockContext withElseBlock() {
return getRuleContext(WithElseBlockContext.class,0);
}
public List templateContent() {
return getRuleContexts(TemplateContentContext.class);
}
public TemplateContentContext templateContent(int i) {
return getRuleContext(TemplateContentContext.class,i);
}
public WithBlockContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_withBlock; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).enterWithBlock(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).exitWithBlock(this);
}
}
public final WithBlockContext withBlock() throws RecognitionException {
WithBlockContext _localctx = new WithBlockContext(_ctx, getState());
enterRule(_localctx, 36, RULE_withBlock);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(198);
match(AT);
setState(199);
match(MV_WITH);
setState(203);
_errHandler.sync(this);
_la = _input.LA(1);
while ((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << LCURLY) | (1L << COMMENT) | (1L << PLAIN) | (1L << AT))) != 0)) {
{
{
setState(200);
templateContent();
}
}
setState(205);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(208);
switch (_input.LA(1)) {
case RCURLY:
{
setState(206);
match(RCURLY);
}
break;
case ELSE:
{
setState(207);
withElseBlock();
}
break;
default:
throw new NoViableAltException(this);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class WithElseBlockContext extends ParserRuleContext {
public TerminalNode ELSE() { return getToken(RockerParser.ELSE, 0); }
public TerminalNode RCURLY() { return getToken(RockerParser.RCURLY, 0); }
public List templateContent() {
return getRuleContexts(TemplateContentContext.class);
}
public TemplateContentContext templateContent(int i) {
return getRuleContext(TemplateContentContext.class,i);
}
public WithElseBlockContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_withElseBlock; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).enterWithElseBlock(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).exitWithElseBlock(this);
}
}
public final WithElseBlockContext withElseBlock() throws RecognitionException {
WithElseBlockContext _localctx = new WithElseBlockContext(_ctx, getState());
enterRule(_localctx, 38, RULE_withElseBlock);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(210);
match(ELSE);
setState(214);
_errHandler.sync(this);
_la = _input.LA(1);
while ((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << LCURLY) | (1L << COMMENT) | (1L << PLAIN) | (1L << AT))) != 0)) {
{
{
setState(211);
templateContent();
}
}
setState(216);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(217);
match(RCURLY);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ContentClosureContext extends ParserRuleContext {
public TerminalNode AT() { return getToken(RockerParser.AT, 0); }
public ContentClosureExpressionContext contentClosureExpression() {
return getRuleContext(ContentClosureExpressionContext.class,0);
}
public TerminalNode RCURLY() { return getToken(RockerParser.RCURLY, 0); }
public List templateContent() {
return getRuleContexts(TemplateContentContext.class);
}
public TemplateContentContext templateContent(int i) {
return getRuleContext(TemplateContentContext.class,i);
}
public ContentClosureContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_contentClosure; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).enterContentClosure(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).exitContentClosure(this);
}
}
public final ContentClosureContext contentClosure() throws RecognitionException {
ContentClosureContext _localctx = new ContentClosureContext(_ctx, getState());
enterRule(_localctx, 40, RULE_contentClosure);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(219);
match(AT);
setState(220);
contentClosureExpression();
setState(224);
_errHandler.sync(this);
_la = _input.LA(1);
while ((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << LCURLY) | (1L << COMMENT) | (1L << PLAIN) | (1L << AT))) != 0)) {
{
{
setState(221);
templateContent();
}
}
setState(226);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(227);
match(RCURLY);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ContentClosureExpressionContext extends ParserRuleContext {
public TerminalNode MV_CONTENT_CLOSURE() { return getToken(RockerParser.MV_CONTENT_CLOSURE, 0); }
public ContentClosureExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_contentClosureExpression; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).enterContentClosureExpression(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).exitContentClosureExpression(this);
}
}
public final ContentClosureExpressionContext contentClosureExpression() throws RecognitionException {
ContentClosureExpressionContext _localctx = new ContentClosureExpressionContext(_ctx, getState());
enterRule(_localctx, 42, RULE_contentClosureExpression);
try {
enterOuterAlt(_localctx, 1);
{
setState(229);
match(MV_CONTENT_CLOSURE);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ValueClosureContext extends ParserRuleContext {
public TerminalNode AT() { return getToken(RockerParser.AT, 0); }
public ValueClosureExpressionContext valueClosureExpression() {
return getRuleContext(ValueClosureExpressionContext.class,0);
}
public TerminalNode RCURLY() { return getToken(RockerParser.RCURLY, 0); }
public List templateContent() {
return getRuleContexts(TemplateContentContext.class);
}
public TemplateContentContext templateContent(int i) {
return getRuleContext(TemplateContentContext.class,i);
}
public ValueClosureContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_valueClosure; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).enterValueClosure(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).exitValueClosure(this);
}
}
public final ValueClosureContext valueClosure() throws RecognitionException {
ValueClosureContext _localctx = new ValueClosureContext(_ctx, getState());
enterRule(_localctx, 44, RULE_valueClosure);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(231);
match(AT);
setState(232);
valueClosureExpression();
setState(236);
_errHandler.sync(this);
_la = _input.LA(1);
while ((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << LCURLY) | (1L << COMMENT) | (1L << PLAIN) | (1L << AT))) != 0)) {
{
{
setState(233);
templateContent();
}
}
setState(238);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(239);
match(RCURLY);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ValueClosureExpressionContext extends ParserRuleContext {
public TerminalNode MV_VALUE_CLOSURE() { return getToken(RockerParser.MV_VALUE_CLOSURE, 0); }
public ValueClosureExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_valueClosureExpression; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).enterValueClosureExpression(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).exitValueClosureExpression(this);
}
}
public final ValueClosureExpressionContext valueClosureExpression() throws RecognitionException {
ValueClosureExpressionContext _localctx = new ValueClosureExpressionContext(_ctx, getState());
enterRule(_localctx, 46, RULE_valueClosureExpression);
try {
enterOuterAlt(_localctx, 1);
{
setState(241);
match(MV_VALUE_CLOSURE);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ValueContext extends ParserRuleContext {
public TerminalNode AT() { return getToken(RockerParser.AT, 0); }
public ValueExpressionContext valueExpression() {
return getRuleContext(ValueExpressionContext.class,0);
}
public ValueContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_value; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).enterValue(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).exitValue(this);
}
}
public final ValueContext value() throws RecognitionException {
ValueContext _localctx = new ValueContext(_ctx, getState());
enterRule(_localctx, 48, RULE_value);
try {
enterOuterAlt(_localctx, 1);
{
setState(243);
match(AT);
setState(244);
valueExpression();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ValueExpressionContext extends ParserRuleContext {
public TerminalNode MV_VALUE() { return getToken(RockerParser.MV_VALUE, 0); }
public ValueExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_valueExpression; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).enterValueExpression(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).exitValueExpression(this);
}
}
public final ValueExpressionContext valueExpression() throws RecognitionException {
ValueExpressionContext _localctx = new ValueExpressionContext(_ctx, getState());
enterRule(_localctx, 50, RULE_valueExpression);
try {
enterOuterAlt(_localctx, 1);
{
setState(246);
match(MV_VALUE);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class NullTernaryContext extends ParserRuleContext {
public TerminalNode AT() { return getToken(RockerParser.AT, 0); }
public NullTernaryExpressionContext nullTernaryExpression() {
return getRuleContext(NullTernaryExpressionContext.class,0);
}
public NullTernaryContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_nullTernary; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).enterNullTernary(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).exitNullTernary(this);
}
}
public final NullTernaryContext nullTernary() throws RecognitionException {
NullTernaryContext _localctx = new NullTernaryContext(_ctx, getState());
enterRule(_localctx, 52, RULE_nullTernary);
try {
enterOuterAlt(_localctx, 1);
{
setState(248);
match(AT);
setState(249);
nullTernaryExpression();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class NullTernaryExpressionContext extends ParserRuleContext {
public TerminalNode MV_NULL_TERNARY_LH() { return getToken(RockerParser.MV_NULL_TERNARY_LH, 0); }
public TerminalNode MV_NULL_TERNARY_RH() { return getToken(RockerParser.MV_NULL_TERNARY_RH, 0); }
public NullTernaryExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_nullTernaryExpression; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).enterNullTernaryExpression(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).exitNullTernaryExpression(this);
}
}
public final NullTernaryExpressionContext nullTernaryExpression() throws RecognitionException {
NullTernaryExpressionContext _localctx = new NullTernaryExpressionContext(_ctx, getState());
enterRule(_localctx, 54, RULE_nullTernaryExpression);
try {
enterOuterAlt(_localctx, 1);
{
setState(251);
match(MV_NULL_TERNARY_LH);
setState(252);
match(MV_NULL_TERNARY_RH);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class EvalContext extends ParserRuleContext {
public TerminalNode AT() { return getToken(RockerParser.AT, 0); }
public EvalExpressionContext evalExpression() {
return getRuleContext(EvalExpressionContext.class,0);
}
public EvalContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_eval; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).enterEval(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).exitEval(this);
}
}
public final EvalContext eval() throws RecognitionException {
EvalContext _localctx = new EvalContext(_ctx, getState());
enterRule(_localctx, 56, RULE_eval);
try {
enterOuterAlt(_localctx, 1);
{
setState(254);
match(AT);
setState(255);
evalExpression();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class EvalExpressionContext extends ParserRuleContext {
public TerminalNode MV_EVAL() { return getToken(RockerParser.MV_EVAL, 0); }
public EvalExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_evalExpression; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).enterEvalExpression(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).exitEvalExpression(this);
}
}
public final EvalExpressionContext evalExpression() throws RecognitionException {
EvalExpressionContext _localctx = new EvalExpressionContext(_ctx, getState());
enterRule(_localctx, 58, RULE_evalExpression);
try {
enterOuterAlt(_localctx, 1);
{
setState(257);
match(MV_EVAL);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class SwitchBlockContext extends ParserRuleContext {
public TerminalNode AT() { return getToken(RockerParser.AT, 0); }
public TerminalNode MV_SWITCH() { return getToken(RockerParser.MV_SWITCH, 0); }
public TerminalNode RCURLY() { return getToken(RockerParser.RCURLY, 0); }
public List switchCase() {
return getRuleContexts(SwitchCaseContext.class);
}
public SwitchCaseContext switchCase(int i) {
return getRuleContext(SwitchCaseContext.class,i);
}
public List switchDefault() {
return getRuleContexts(SwitchDefaultContext.class);
}
public SwitchDefaultContext switchDefault(int i) {
return getRuleContext(SwitchDefaultContext.class,i);
}
public List plain() {
return getRuleContexts(PlainContext.class);
}
public PlainContext plain(int i) {
return getRuleContext(PlainContext.class,i);
}
public List comment() {
return getRuleContexts(CommentContext.class);
}
public CommentContext comment(int i) {
return getRuleContext(CommentContext.class,i);
}
public SwitchBlockContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_switchBlock; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).enterSwitchBlock(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).exitSwitchBlock(this);
}
}
public final SwitchBlockContext switchBlock() throws RecognitionException {
SwitchBlockContext _localctx = new SwitchBlockContext(_ctx, getState());
enterRule(_localctx, 60, RULE_switchBlock);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(259);
match(AT);
setState(260);
match(MV_SWITCH);
setState(267);
_errHandler.sync(this);
_la = _input.LA(1);
while ((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << CASE) | (1L << DEFAULT) | (1L << LCURLY) | (1L << COMMENT) | (1L << PLAIN))) != 0)) {
{
setState(265);
switch (_input.LA(1)) {
case CASE:
{
setState(261);
switchCase();
}
break;
case DEFAULT:
{
setState(262);
switchDefault();
}
break;
case LCURLY:
case PLAIN:
{
setState(263);
plain();
}
break;
case COMMENT:
{
setState(264);
comment();
}
break;
default:
throw new NoViableAltException(this);
}
}
setState(269);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(270);
match(RCURLY);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class SwitchCaseContext extends ParserRuleContext {
public TerminalNode CASE() { return getToken(RockerParser.CASE, 0); }
public TerminalNode RCURLY() { return getToken(RockerParser.RCURLY, 0); }
public List templateContent() {
return getRuleContexts(TemplateContentContext.class);
}
public TemplateContentContext templateContent(int i) {
return getRuleContext(TemplateContentContext.class,i);
}
public SwitchCaseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_switchCase; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).enterSwitchCase(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).exitSwitchCase(this);
}
}
public final SwitchCaseContext switchCase() throws RecognitionException {
SwitchCaseContext _localctx = new SwitchCaseContext(_ctx, getState());
enterRule(_localctx, 62, RULE_switchCase);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(272);
match(CASE);
setState(276);
_errHandler.sync(this);
_la = _input.LA(1);
while ((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << LCURLY) | (1L << COMMENT) | (1L << PLAIN) | (1L << AT))) != 0)) {
{
{
setState(273);
templateContent();
}
}
setState(278);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(279);
match(RCURLY);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class SwitchDefaultContext extends ParserRuleContext {
public TerminalNode DEFAULT() { return getToken(RockerParser.DEFAULT, 0); }
public TerminalNode RCURLY() { return getToken(RockerParser.RCURLY, 0); }
public List templateContent() {
return getRuleContexts(TemplateContentContext.class);
}
public TemplateContentContext templateContent(int i) {
return getRuleContext(TemplateContentContext.class,i);
}
public SwitchDefaultContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_switchDefault; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).enterSwitchDefault(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof RockerParserListener ) ((RockerParserListener)listener).exitSwitchDefault(this);
}
}
public final SwitchDefaultContext switchDefault() throws RecognitionException {
SwitchDefaultContext _localctx = new SwitchDefaultContext(_ctx, getState());
enterRule(_localctx, 64, RULE_switchDefault);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(281);
match(DEFAULT);
setState(285);
_errHandler.sync(this);
_la = _input.LA(1);
while ((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << LCURLY) | (1L << COMMENT) | (1L << PLAIN) | (1L << AT))) != 0)) {
{
{
setState(282);
templateContent();
}
}
setState(287);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(288);
match(RCURLY);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static final String _serializedATN =
"\3\u0430\ud6d1\u8206\uad2d\u4417\uaef1\u8d80\uaadd\3\30\u0125\4\2\t\2"+
"\4\3\t\3\4\4\t\4\4\5\t\5\4\6\t\6\4\7\t\7\4\b\t\b\4\t\t\t\4\n\t\n\4\13"+
"\t\13\4\f\t\f\4\r\t\r\4\16\t\16\4\17\t\17\4\20\t\20\4\21\t\21\4\22\t\22"+
"\4\23\t\23\4\24\t\24\4\25\t\25\4\26\t\26\4\27\t\27\4\30\t\30\4\31\t\31"+
"\4\32\t\32\4\33\t\33\4\34\t\34\4\35\t\35\4\36\t\36\4\37\t\37\4 \t \4!"+
"\t!\4\"\t\"\3\2\3\2\3\2\3\2\7\2I\n\2\f\2\16\2L\13\2\3\2\5\2O\n\2\3\2\7"+
"\2R\n\2\f\2\16\2U\13\2\3\3\3\3\5\3Y\n\3\3\4\3\4\7\4]\n\4\f\4\16\4`\13"+
"\4\3\4\7\4c\n\4\f\4\16\4f\13\4\3\4\3\4\5\4j\n\4\3\5\3\5\7\5n\n\5\f\5\16"+
"\5q\13\5\3\6\3\6\7\6u\n\6\f\6\16\6x\13\6\3\6\3\6\3\7\3\7\3\b\3\b\3\b\3"+
"\t\3\t\3\n\3\n\3\n\3\13\3\13\3\f\3\f\3\f\3\r\3\r\3\16\3\16\3\16\3\16\3"+
"\16\3\16\3\16\3\16\5\16\u0095\n\16\3\17\3\17\3\17\3\17\5\17\u009b\n\17"+
"\3\20\3\20\3\20\7\20\u00a0\n\20\f\20\16\20\u00a3\13\20\3\20\3\20\3\20"+
"\5\20\u00a8\n\20\3\21\3\21\7\21\u00ac\n\21\f\21\16\21\u00af\13\21\3\21"+
"\3\21\3\21\5\21\u00b4\n\21\3\22\3\22\7\22\u00b8\n\22\f\22\16\22\u00bb"+
"\13\22\3\22\3\22\3\23\3\23\3\23\7\23\u00c2\n\23\f\23\16\23\u00c5\13\23"+
"\3\23\3\23\3\24\3\24\3\24\7\24\u00cc\n\24\f\24\16\24\u00cf\13\24\3\24"+
"\3\24\5\24\u00d3\n\24\3\25\3\25\7\25\u00d7\n\25\f\25\16\25\u00da\13\25"+
"\3\25\3\25\3\26\3\26\3\26\7\26\u00e1\n\26\f\26\16\26\u00e4\13\26\3\26"+
"\3\26\3\27\3\27\3\30\3\30\3\30\7\30\u00ed\n\30\f\30\16\30\u00f0\13\30"+
"\3\30\3\30\3\31\3\31\3\32\3\32\3\32\3\33\3\33\3\34\3\34\3\34\3\35\3\35"+
"\3\35\3\36\3\36\3\36\3\37\3\37\3 \3 \3 \3 \3 \3 \7 \u010c\n \f \16 \u010f"+
"\13 \3 \3 \3!\3!\7!\u0115\n!\f!\16!\u0118\13!\3!\3!\3\"\3\"\7\"\u011e"+
"\n\"\f\"\16\"\u0121\13\"\3\"\3\"\3\"\2\2#\2\4\6\b\n\f\16\20\22\24\26\30"+
"\32\34\36 \"$&(*,.\60\62\64\668:<>@B\2\2\u012c\2J\3\2\2\2\4X\3\2\2\2\6"+
"Z\3\2\2\2\bk\3\2\2\2\nr\3\2\2\2\f{\3\2\2\2\16}\3\2\2\2\20\u0080\3\2\2"+
"\2\22\u0082\3\2\2\2\24\u0085\3\2\2\2\26\u0087\3\2\2\2\30\u008a\3\2\2\2"+
"\32\u0094\3\2\2\2\34\u009a\3\2\2\2\36\u009c\3\2\2\2 \u00a9\3\2\2\2\"\u00b5"+
"\3\2\2\2$\u00be\3\2\2\2&\u00c8\3\2\2\2(\u00d4\3\2\2\2*\u00dd\3\2\2\2,"+
"\u00e7\3\2\2\2.\u00e9\3\2\2\2\60\u00f3\3\2\2\2\62\u00f5\3\2\2\2\64\u00f8"+
"\3\2\2\2\66\u00fa\3\2\2\28\u00fd\3\2\2\2:\u0100\3\2\2\2<\u0103\3\2\2\2"+
">\u0105\3\2\2\2@\u0112\3\2\2\2B\u011b\3\2\2\2DI\5\4\3\2EI\5\f\7\2FI\5"+
"\16\b\2GI\5\22\n\2HD\3\2\2\2HE\3\2\2\2HF\3\2\2\2HG\3\2\2\2IL\3\2\2\2J"+
"H\3\2\2\2JK\3\2\2\2KN\3\2\2\2LJ\3\2\2\2MO\5\26\f\2NM\3\2\2\2NO\3\2\2\2"+
"OS\3\2\2\2PR\5\32\16\2QP\3\2\2\2RU\3\2\2\2SQ\3\2\2\2ST\3\2\2\2T\3\3\2"+
"\2\2US\3\2\2\2VY\7\n\2\2WY\5\6\4\2XV\3\2\2\2XW\3\2\2\2Y\5\3\2\2\2Z^\7"+
"\7\2\2[]\5\32\16\2\\[\3\2\2\2]`\3\2\2\2^\\\3\2\2\2^_\3\2\2\2_d\3\2\2\2"+
"`^\3\2\2\2ac\5\b\5\2ba\3\2\2\2cf\3\2\2\2db\3\2\2\2de\3\2\2\2ei\3\2\2\2"+
"fd\3\2\2\2gj\7\b\2\2hj\5\n\6\2ig\3\2\2\2ih\3\2\2\2j\7\3\2\2\2ko\7\3\2"+
"\2ln\5\32\16\2ml\3\2\2\2nq\3\2\2\2om\3\2\2\2op\3\2\2\2p\t\3\2\2\2qo\3"+
"\2\2\2rv\7\4\2\2su\5\32\16\2ts\3\2\2\2ux\3\2\2\2vt\3\2\2\2vw\3\2\2\2w"+
"y\3\2\2\2xv\3\2\2\2yz\7\b\2\2z\13\3\2\2\2{|\7\t\2\2|\r\3\2\2\2}~\7\13"+
"\2\2~\177\5\20\t\2\177\17\3\2\2\2\u0080\u0081\7\f\2\2\u0081\21\3\2\2\2"+
"\u0082\u0083\7\13\2\2\u0083\u0084\5\24\13\2\u0084\23\3\2\2\2\u0085\u0086"+
"\7\r\2\2\u0086\25\3\2\2\2\u0087\u0088\7\13\2\2\u0088\u0089\5\30\r\2\u0089"+
"\27\3\2\2\2\u008a\u008b\7\16\2\2\u008b\31\3\2\2\2\u008c\u0095\5\f\7\2"+
"\u008d\u0095\5\34\17\2\u008e\u0095\5\4\3\2\u008f\u0095\5*\26\2\u0090\u0095"+
"\5.\30\2\u0091\u0095\5\62\32\2\u0092\u0095\5\66\34\2\u0093\u0095\5:\36"+
"\2\u0094\u008c\3\2\2\2\u0094\u008d\3\2\2\2\u0094\u008e\3\2\2\2\u0094\u008f"+
"\3\2\2\2\u0094\u0090\3\2\2\2\u0094\u0091\3\2\2\2\u0094\u0092\3\2\2\2\u0094"+
"\u0093\3\2\2\2\u0095\33\3\2\2\2\u0096\u009b\5\36\20\2\u0097\u009b\5$\23"+
"\2\u0098\u009b\5&\24\2\u0099\u009b\5> \2\u009a\u0096\3\2\2\2\u009a\u0097"+
"\3\2\2\2\u009a\u0098\3\2\2\2\u009a\u0099\3\2\2\2\u009b\35\3\2\2\2\u009c"+
"\u009d\7\13\2\2\u009d\u00a1\7\17\2\2\u009e\u00a0\5\32\16\2\u009f\u009e"+
"\3\2\2\2\u00a0\u00a3\3\2\2\2\u00a1\u009f\3\2\2\2\u00a1\u00a2\3\2\2\2\u00a2"+
"\u00a7\3\2\2\2\u00a3\u00a1\3\2\2\2\u00a4\u00a8\7\b\2\2\u00a5\u00a8\5 "+
"\21\2\u00a6\u00a8\5\"\22\2\u00a7\u00a4\3\2\2\2\u00a7\u00a5\3\2\2\2\u00a7"+
"\u00a6\3\2\2\2\u00a8\37\3\2\2\2\u00a9\u00ad\7\3\2\2\u00aa\u00ac\5\32\16"+
"\2\u00ab\u00aa\3\2\2\2\u00ac\u00af\3\2\2\2\u00ad\u00ab\3\2\2\2\u00ad\u00ae"+
"\3\2\2\2\u00ae\u00b3\3\2\2\2\u00af\u00ad\3\2\2\2\u00b0\u00b4\7\b\2\2\u00b1"+
"\u00b4\5 \21\2\u00b2\u00b4\5\"\22\2\u00b3\u00b0\3\2\2\2\u00b3\u00b1\3"+
"\2\2\2\u00b3\u00b2\3\2\2\2\u00b4!\3\2\2\2\u00b5\u00b9\7\4\2\2\u00b6\u00b8"+
"\5\32\16\2\u00b7\u00b6\3\2\2\2\u00b8\u00bb\3\2\2\2\u00b9\u00b7\3\2\2\2"+
"\u00b9\u00ba\3\2\2\2\u00ba\u00bc\3\2\2\2\u00bb\u00b9\3\2\2\2\u00bc\u00bd"+
"\7\b\2\2\u00bd#\3\2\2\2\u00be\u00bf\7\13\2\2\u00bf\u00c3\7\21\2\2\u00c0"+
"\u00c2\5\32\16\2\u00c1\u00c0\3\2\2\2\u00c2\u00c5\3\2\2\2\u00c3\u00c1\3"+
"\2\2\2\u00c3\u00c4\3\2\2\2\u00c4\u00c6\3\2\2\2\u00c5\u00c3\3\2\2\2\u00c6"+
"\u00c7\7\b\2\2\u00c7%\3\2\2\2\u00c8\u00c9\7\13\2\2\u00c9\u00cd\7\22\2"+
"\2\u00ca\u00cc\5\32\16\2\u00cb\u00ca\3\2\2\2\u00cc\u00cf\3\2\2\2\u00cd"+
"\u00cb\3\2\2\2\u00cd\u00ce\3\2\2\2\u00ce\u00d2\3\2\2\2\u00cf\u00cd\3\2"+
"\2\2\u00d0\u00d3\7\b\2\2\u00d1\u00d3\5(\25\2\u00d2\u00d0\3\2\2\2\u00d2"+
"\u00d1\3\2\2\2\u00d3\'\3\2\2\2\u00d4\u00d8\7\4\2\2\u00d5\u00d7\5\32\16"+
"\2\u00d6\u00d5\3\2\2\2\u00d7\u00da\3\2\2\2\u00d8\u00d6\3\2\2\2\u00d8\u00d9"+
"\3\2\2\2\u00d9\u00db\3\2\2\2\u00da\u00d8\3\2\2\2\u00db\u00dc\7\b\2\2\u00dc"+
")\3\2\2\2\u00dd\u00de\7\13\2\2\u00de\u00e2\5,\27\2\u00df\u00e1\5\32\16"+
"\2\u00e0\u00df\3\2\2\2\u00e1\u00e4\3\2\2\2\u00e2\u00e0\3\2\2\2\u00e2\u00e3"+
"\3\2\2\2\u00e3\u00e5\3\2\2\2\u00e4\u00e2\3\2\2\2\u00e5\u00e6\7\b\2\2\u00e6"+
"+\3\2\2\2\u00e7\u00e8\7\23\2\2\u00e8-\3\2\2\2\u00e9\u00ea\7\13\2\2\u00ea"+
"\u00ee\5\60\31\2\u00eb\u00ed\5\32\16\2\u00ec\u00eb\3\2\2\2\u00ed\u00f0"+
"\3\2\2\2\u00ee\u00ec\3\2\2\2\u00ee\u00ef\3\2\2\2\u00ef\u00f1\3\2\2\2\u00f0"+
"\u00ee\3\2\2\2\u00f1\u00f2\7\b\2\2\u00f2/\3\2\2\2\u00f3\u00f4\7\24\2\2"+
"\u00f4\61\3\2\2\2\u00f5\u00f6\7\13\2\2\u00f6\u00f7\5\64\33\2\u00f7\63"+
"\3\2\2\2\u00f8\u00f9\7\27\2\2\u00f9\65\3\2\2\2\u00fa\u00fb\7\13\2\2\u00fb"+
"\u00fc\58\35\2\u00fc\67\3\2\2\2\u00fd\u00fe\7\26\2\2\u00fe\u00ff\7\30"+
"\2\2\u00ff9\3\2\2\2\u0100\u0101\7\13\2\2\u0101\u0102\5<\37\2\u0102;\3"+
"\2\2\2\u0103\u0104\7\25\2\2\u0104=\3\2\2\2\u0105\u0106\7\13\2\2\u0106"+
"\u010d\7\20\2\2\u0107\u010c\5@!\2\u0108\u010c\5B\"\2\u0109\u010c\5\4\3"+
"\2\u010a\u010c\5\f\7\2\u010b\u0107\3\2\2\2\u010b\u0108\3\2\2\2\u010b\u0109"+
"\3\2\2\2\u010b\u010a\3\2\2\2\u010c\u010f\3\2\2\2\u010d\u010b\3\2\2\2\u010d"+
"\u010e\3\2\2\2\u010e\u0110\3\2\2\2\u010f\u010d\3\2\2\2\u0110\u0111\7\b"+
"\2\2\u0111?\3\2\2\2\u0112\u0116\7\5\2\2\u0113\u0115\5\32\16\2\u0114\u0113"+
"\3\2\2\2\u0115\u0118\3\2\2\2\u0116\u0114\3\2\2\2\u0116\u0117\3\2\2\2\u0117"+
"\u0119\3\2\2\2\u0118\u0116\3\2\2\2\u0119\u011a\7\b\2\2\u011aA\3\2\2\2"+
"\u011b\u011f\7\6\2\2\u011c\u011e\5\32\16\2\u011d\u011c\3\2\2\2\u011e\u0121"+
"\3\2\2\2\u011f\u011d\3\2\2\2\u011f\u0120\3\2\2\2\u0120\u0122\3\2\2\2\u0121"+
"\u011f\3\2\2\2\u0122\u0123\7\b\2\2\u0123C\3\2\2\2\35HJNSX^diov\u0094\u009a"+
"\u00a1\u00a7\u00ad\u00b3\u00b9\u00c3\u00cd\u00d2\u00d8\u00e2\u00ee\u010b"+
"\u010d\u0116\u011f";
public static final ATN _ATN =
new ATNDeserializer().deserialize(_serializedATN.toCharArray());
static {
_decisionToDFA = new DFA[_ATN.getNumberOfDecisions()];
for (int i = 0; i < _ATN.getNumberOfDecisions(); i++) {
_decisionToDFA[i] = new DFA(_ATN.getDecisionState(i), i);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy