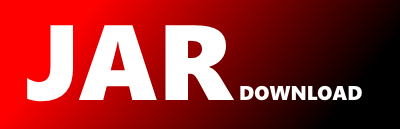
com.fizzed.rocker.model.TemplateModel Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2015 Fizzed Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.fizzed.rocker.model;
import com.fizzed.rocker.compiler.RockerOptions;
import com.fizzed.rocker.ContentType;
import com.fizzed.rocker.compiler.RockerUtil;
import java.util.ArrayList;
import java.util.LinkedHashMap;
import java.util.List;
/**
*
* @author joelauer
*/
public class TemplateModel {
// e.g. "views.system"
private final String packageName;
// e.g. "index.rocker.html"
private final String templateName;
private final ContentType contentType;
private final long modifiedAt;
// e.g. "index"
private final String name;
private final List imports;
private final List arguments;
private final List units;
private final RockerOptions options;
public TemplateModel(String packageName, String templateName, long modifiedAt, RockerOptions defaultOptions) {
this.packageName = packageName;
this.templateName = templateName;
this.name = RockerUtil.templateNameToName(templateName);
this.contentType = RockerUtil.templateNameToContentType(templateName);
this.modifiedAt = modifiedAt;
this.imports = new ArrayList<>();
this.arguments = new ArrayList<>();
this.units = new ArrayList<>();
this.options = defaultOptions;
}
public String getPackageName() {
return packageName;
}
public String getTemplateName() {
return templateName;
}
public ContentType getContentType() {
return contentType;
}
public String getName() {
return name;
}
public long getModifiedAt() {
return modifiedAt;
}
public List getImports() {
return imports;
}
public List getArguments() {
return arguments;
}
public boolean hasRockerBodyArgument() {
return getRockerBodyArgument() != null;
}
public Argument getRockerBodyArgument() {
if (!arguments.isEmpty()) {
Argument lastArgument = arguments.get(arguments.size() - 1);
if (lastArgument.getType().equals("RockerBody")) {
return lastArgument;
}
}
return null;
}
public List getArgumentsWithoutRockerBody() {
if (hasRockerBodyArgument()) {
return arguments.subList(0, arguments.size() - 1);
} else {
return arguments;
}
}
public RockerOptions getOptions() {
return options;
}
public List getUnits() {
return units;
}
public T getUnit(int index, Class type) {
return (T)units.get(index);
}
public T findUnitByOccurrence(Class type, int occurrence) {
if(occurrence < 1) {
throw new IllegalArgumentException("Occurrence needs to be >= 1");
}
int foundSoFar = 0;
for (TemplateUnit unit: units) {
if(type == unit.getClass()) {
if(++foundSoFar == occurrence) {
return (T) unit;
}
}
}
throw new RuntimeException("Failed to find occurrence: " + occurrence + " for TemplateUnit of type: " + type.getName());
}
public LinkedHashMap> createPlainTextMap(int chunkSize) {
// optimize static plain text constants
int index = 0;
LinkedHashMap> plainTextMap = new LinkedHashMap<>();
for (TemplateUnit unit : getUnits()) {
if (unit instanceof PlainText) {
PlainText plain = (PlainText)unit;
if (!plainTextMap.containsKey(plain.getText())) {
LinkedHashMap chunkMap = new LinkedHashMap<>();
plainTextMap.put(plain.getText(), chunkMap);
// split text into chunks
List chunks = RockerUtil.stringIntoChunks(plain.getText(), chunkSize);
for (int chunkIndex = 0; chunkIndex < chunks.size(); chunkIndex++) {
String varName = "PLAIN_TEXT_" + index + "_" + chunkIndex;
String chunkText = chunks.get(chunkIndex);
chunkMap.put(varName, chunkText);
}
index++;
}
}
}
return plainTextMap;
}
/**
* Build hash value representing all components from the "header" that would
* break an "interface" (used for reloading).
* @return
*/
public int createHeaderHash() {
StringBuilder s = new StringBuilder();
/**
// append each java import
for (JavaImport ji : imports) {
s.append(ji.getStatement());
s.append(";");
}
*/
s.append(this.contentType);
s.append(";");
for (Argument arg : arguments) {
s.append(arg.getType());
s.append(" ");
s.append(arg.getName());
s.append(";");
}
return s.toString().hashCode();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy