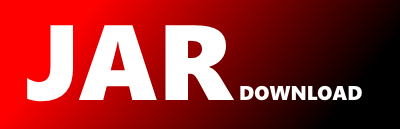
com.fizzed.rocker.runtime.Java8Iterator Maven / Gradle / Ivy
/*
* Copyright 2015 Fizzed Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.fizzed.rocker.runtime;
import com.fizzed.rocker.ForIterator;
import com.fizzed.rocker.RenderingException;
import com.fizzed.rocker.runtime.PrimitiveCollections.BooleanCollection;
import com.fizzed.rocker.runtime.PrimitiveCollections.ByteCollection;
import com.fizzed.rocker.runtime.PrimitiveCollections.CharacterCollection;
import com.fizzed.rocker.runtime.PrimitiveCollections.DoubleCollection;
import com.fizzed.rocker.runtime.PrimitiveCollections.FloatCollection;
import com.fizzed.rocker.runtime.PrimitiveCollections.IntegerCollection;
import com.fizzed.rocker.runtime.PrimitiveCollections.LongCollection;
import com.fizzed.rocker.runtime.PrimitiveCollections.ObjectCollection;
import com.fizzed.rocker.runtime.PrimitiveCollections.ShortCollection;
import java.io.IOException;
import java.util.Collection;
import java.util.Map;
public class Java8Iterator {
static public interface ConsumeCollection {
void accept(V v) throws RenderingException, IOException;
}
static public interface ConsumeCollectionWithIterator {
void accept(ForIterator i, V v) throws RenderingException, IOException;
}
static public interface ConsumeMap {
void accept(K k, V v) throws RenderingException, IOException;
}
static public interface ConsumeMapWithIterator {
void accept(ForIterator i, K k, V v) throws RenderingException, IOException;
}
static public void forEach(Collection items, ConsumeCollection consumer) throws RenderingException, IOException {
for (V item : items) {
consumer.accept(item);
}
}
static public void forEach(Collection items, ConsumeCollectionWithIterator consumer) throws RenderingException, IOException {
IndexOnlyForIterator it = new IndexOnlyForIterator(items.size());
for (V item : items) {
it.increment();
consumer.accept(it, item);
}
}
static public void forEach(Map items, ConsumeMap consumer) throws RenderingException, IOException {
for (Map.Entry item : items.entrySet()) {
consumer.accept(item.getKey(), item.getValue());
}
}
static public void forEach(Map items, ConsumeMapWithIterator consumer) throws RenderingException, IOException {
IndexOnlyForIterator it = new IndexOnlyForIterator(items.size());
for (Map.Entry item : items.entrySet()) {
it.increment();
consumer.accept(it, item.getKey(), item.getValue());
}
}
// support for primitive arrays w/o iterator
static public void forEach(boolean[] items, ConsumeCollection consumer) throws RenderingException, IOException {
forEach(new BooleanCollection(items), consumer);
}
static public void forEach(byte[] items, ConsumeCollection consumer) throws RenderingException, IOException {
forEach(new ByteCollection(items), consumer);
}
static public void forEach(char[] items, ConsumeCollection consumer) throws RenderingException, IOException {
forEach(new CharacterCollection(items), consumer);
}
static public void forEach(short[] items, ConsumeCollection consumer) throws RenderingException, IOException {
forEach(new ShortCollection(items), consumer);
}
static public void forEach(int[] items, ConsumeCollection consumer) throws RenderingException, IOException {
forEach(new IntegerCollection(items), consumer);
}
static public void forEach(long[] items, ConsumeCollection consumer) throws RenderingException, IOException {
forEach(new LongCollection(items), consumer);
}
static public void forEach(float[] items, ConsumeCollection consumer) throws RenderingException, IOException {
forEach(new FloatCollection(items), consumer);
}
static public void forEach(double[] items, ConsumeCollection consumer) throws RenderingException, IOException {
forEach(new DoubleCollection(items), consumer);
}
static public void forEach(Object[] items, ConsumeCollection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy