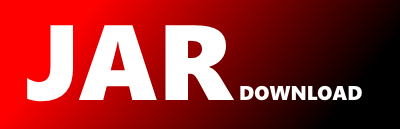
com.fizzgate.plugin.auth.ServiceConfig Maven / Gradle / Ivy
/*
* Copyright (C) 2020 the original author or authors.
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
package com.fizzgate.plugin.auth;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fizzgate.util.Consts;
import com.fizzgate.util.ThreadContext;
import com.fizzgate.util.UrlTransformUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.http.HttpMethod;
import java.util.*;
/**
* @author hongqiaowei
*/
public class ServiceConfig {
private static final Logger log = LoggerFactory.getLogger(ServiceConfig.class);
private String id;
public Map>
>
>
apiConfigMap = new HashMap<>();
public ServiceConfig(String id) {
this.id = id;
}
public void add(ApiConfig ac) {
for (String gatewayGroup : ac.gatewayGroups) {
Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy