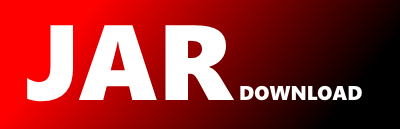
com.flash3388.flashlib.io.devices.SpeedControllerGroup Maven / Gradle / Ivy
package com.flash3388.flashlib.io.devices;
import com.castle.util.closeables.Closer;
import com.flash3388.flashlib.control.Direction;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
/**
* A container for multiple speed controllers. Grouping controllers in such manner allows simultaneous
* control of several motors. When motors should be activated together, this container is extremely useful.
*
* @since FlashLib 1.0.0
*/
public class SpeedControllerGroup implements SpeedController, DeviceGroup {
private final List mControllers;
private boolean mIsInverted;
/**
* Creates a new container for a list of speed controller.
*
* @param controllers list of controllers to be contained
*/
public SpeedControllerGroup(Collection controllers){
mControllers = Collections.unmodifiableList(new ArrayList<>(controllers));
}
public SpeedControllerGroup(SpeedController... controllers) {
this(Arrays.asList(controllers));
}
/**
* {@inheritDoc}
*
* Sets the speed value to all the speed controllers contained in this object.
*
*/
@Override
public void set(double speed) {
Direction direction = mIsInverted ? Direction.BACKWARD : Direction.FORWARD;
for (SpeedController controller : mControllers) {
controller.set(speed, direction);
}
}
/**
* {@inheritDoc}
*
* Stops all the speed controllers contained in this object.
*
*/
@Override
public void stop() {
for (SpeedController controller : mControllers) {
controller.stop();
}
}
/**
* {@inheritDoc}
*
* Gets the average speed value of all the speed controllers contained in this object.
*
*/
@Override
public double get() {
double totalSpeed = 0.0;
for (SpeedController controller : mControllers) {
totalSpeed += controller.get();
}
return totalSpeed / mControllers.size();
}
/**
* {@inheritDoc}
*/
@Override
public boolean isInverted() {
return mIsInverted;
}
/**
* {@inheritDoc}
*
* Sets all the speed controllers contained in this object .
*
*/
@Override
public void setInverted(boolean inverted) {
mIsInverted = inverted;
}
@Override
public void close() throws IOException {
Closer closer = Closer.empty();
closer.addAll(mControllers);
try {
closer.close();
} catch (Exception e) {
throw new IOException(e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy