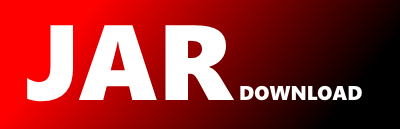
com.fleetpin.graphql.aws.lambda.LambdaCache Maven / Gradle / Ivy
package com.fleetpin.graphql.aws.lambda;
import java.lang.ref.WeakReference;
import java.time.Duration;
import java.time.Instant;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.ConcurrentLinkedQueue;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.function.Supplier;
public class LambdaCache {
private final static ConcurrentLinkedQueue>> entries = new ConcurrentLinkedQueue<>();
private final Duration writeTTL;
private final Map> map;
private final Function producuer;
public LambdaCache(Duration writeTTL, Function builder) {
this.writeTTL = writeTTL;
this.producuer = builder;
this.map = new ConcurrentHashMap<>();
entries.add(new WeakReference<>(this));
}
private CacheWrapper build(K key) {
V value = producuer.apply(key);
return new CacheWrapper(Instant.now(), value);
}
public V get(K key) {
return map.computeIfAbsent(key, this::build).value;
}
public V get(K key, Supplier consumer) {
return map.computeIfAbsent(key, __ -> new CacheWrapper(Instant.now(), consumer.get())).value;
}
public static void evict() {
var now = Instant.now();
var it = entries.iterator();
while(it.hasNext()) {
var v = it.next().get();
if(v == null) {
it.remove();
}else {
var vIt = v.map.values().iterator();
while(vIt.hasNext()) {
var value = vIt.next();
if(value.added.plus(v.writeTTL).isBefore(now)) {
vIt.remove();
}
}
}
}
entries.forEach(e -> {
});
}
private static class CacheWrapper {
private final Instant added;
private final V value;
public CacheWrapper(Instant added, V value) {
this.added = added;
this.value = value;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy