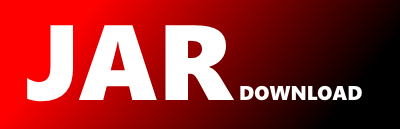
com.flipboard.goldengate.Processor Maven / Gradle / Ivy
package com.flipboard.goldengate;
import java.util.HashSet;
import java.util.Set;
import javax.annotation.processing.AbstractProcessor;
import javax.annotation.processing.Filer;
import javax.annotation.processing.Messager;
import javax.annotation.processing.ProcessingEnvironment;
import javax.annotation.processing.RoundEnvironment;
import javax.lang.model.SourceVersion;
import javax.lang.model.element.Element;
import javax.lang.model.element.ElementKind;
import javax.lang.model.element.TypeElement;
import javax.lang.model.util.Elements;
import javax.lang.model.util.Types;
import javax.tools.Diagnostic;
public class Processor extends AbstractProcessor {
public static Processor instance;
public Types typeUtils;
public Elements elementUtils;
public Filer filer;
public Messager messager;
@Override
public Set getSupportedAnnotationTypes() {
return new HashSet() {{
add(Bridge.class.getCanonicalName());
}};
}
@Override
public SourceVersion getSupportedSourceVersion() {
return SourceVersion.latestSupported();
}
@Override
public synchronized void init(ProcessingEnvironment processingEnv) {
super.init(processingEnv);
instance = this;
typeUtils = processingEnv.getTypeUtils();
elementUtils = processingEnv.getElementUtils();
filer = processingEnv.getFiler();
messager = processingEnv.getMessager();
}
@Override
public boolean process(Set extends TypeElement> annotations, RoundEnvironment roundEnv) {
for (Element annotatedElement : roundEnv.getElementsAnnotatedWith(Bridge.class)) {
// Make sure element is an interface declaration
if (annotatedElement.getKind() != ElementKind.INTERFACE) {
error(annotatedElement, "Only interfaces can be annotated with @%s", Bridge.class.getSimpleName());
return true;
}
try {
new BridgeInterface(annotatedElement).writeToFiler(filer);
} catch (Exception e) {
error(annotatedElement, "%s", e.getMessage());
}
}
return true;
}
private void error(Element e, String msg, Object... args) {
messager.printMessage(Diagnostic.Kind.ERROR, String.format(msg, args), e);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy