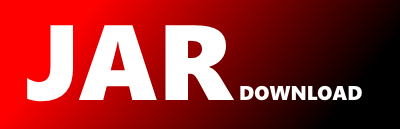
com.floreysoft.jmte.util.MiniParser Maven / Gradle / Ivy
Go to download
To build and locally install jar, javadoc and sources, please use:
mvn clean javadoc:jar source:jar install -Dmaven.test.skip=true
Tested on Maven 2.0.9, JDK 1.7
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy