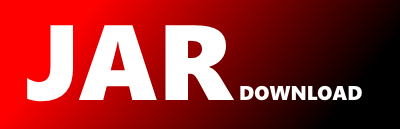
com.fluidbpm.program.api.vo.field.Field Maven / Gradle / Ivy
Show all versions of fluid-api Show documentation
/*
* Koekiebox CONFIDENTIAL
*
* [2012] - [2017] Koekiebox (Pty) Ltd
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property
* of Koekiebox and its suppliers, if any. The intellectual and
* technical concepts contained herein are proprietary to Koekiebox
* and its suppliers and may be covered by South African and Foreign Patents,
* patents in process, and are protected by trade secret or copyright law.
* Dissemination of this information or reproduction of this material is strictly
* forbidden unless prior written permission is obtained from Koekiebox.
*/
package com.fluidbpm.program.api.vo.field;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
import javax.xml.bind.annotation.XmlTransient;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import com.fluidbpm.program.api.util.UtilGlobal;
import com.fluidbpm.program.api.util.elasticsearch.exception.FluidElasticSearchException;
import com.fluidbpm.program.api.vo.ABaseFluidElasticSearchJSONObject;
import com.fluidbpm.program.api.vo.ABaseFluidJSONObject;
import com.fluidbpm.program.api.vo.form.Form;
import com.fluidbpm.program.api.vo.item.FluidItem;
/**
* Represents an Fluid Field for Form, User, Route and Global.
*
* {@code Field} can be part of Electronic Form or Form Definition in Fluid.
*
* @author jasonbruwer
* @since v1.0
*
* @see Form
* @see FluidItem
* @see ABaseFluidElasticSearchJSONObject
*/
public class Field extends ABaseFluidElasticSearchJSONObject {
public static final long serialVersionUID = 1L;
private String fieldName;
private Object fieldValue;
private String fieldDescription;
private String fieldType;
private String typeMetaData;
public static final String LATITUDE_AND_LONGITUDE = "Latitude and Longitude";
public static final String PLAIN_KEYWORD = "Plain Keyword";
/**
* The JSON mapping for the {@code Field} object.
*/
public static class JSONMapping {
public static final String FIELD_NAME= "fieldName";
public static final String FIELD_DESCRIPTION = "fieldDescription";
public static final String FIELD_VALUE = "fieldValue";
public static final String FIELD_TYPE = "fieldType";
public static final String TYPE_META_DATA = "typeMetaData";
/**
* The JSON mapping for Elastic Search data types.
* See {@code https://www.elastic.co/guide/en/elasticsearch/reference/current/mapping-types.html}.
*/
public static final class Elastic
{
public static final String FIELD_TYPE = "fieldType";
//Used only for mapping types in ES...
public static final String MAPPING_ONLY_TYPE = "type";
}
}
/**
*
* The types of Fields and Value fieldType mapping is;
*
*
* Field Fluid vs Java mapping
*
* Field Type in Fluid
* Field Type value in Java
*
*
* {@code Text}
* {@code String}
*
*
* {@code TrueFalse}
* {@code Boolean}
*
*
* {@code DateTime}
* {@code Date}
*
*
* {@code Decimal}
* {@code java.lang.Double}
*
*
* {@code MultipleChoice}
* {@code MultiChoice}
*
*
* {@code ParagraphText}
* {@code String}
*
*
* {@code Table}
* {@code com.fluidbpm.program.api.vo.field.TableField}
*
*
*/
public static enum Type {
Text,
TextEncrypted,
TrueFalse,
DateTime,
Decimal,
MultipleChoice,
ParagraphText,
//Table field only supported by Database (Memcached statement)...
Table,
//Only for visual purposes...
Label
}
/**
* The field fieldType for Elastic Search.
* See {@code https://www.elastic.co/guide/en/elasticsearch/reference/current/mapping-types.html}
*/
public static class ElasticSearchType {
//String...
public static final String TEXT = "text";
public static final String KEYWORD = "keyword";
public static final String IP = "ip";
//Numeric...
public static final String DOUBLE = "double";
public static final String LONG = "long";
public static final String INTEGER = "integer";
//Date...
public static final String DATE = "date";
//Boolean...
public static final String BOOLEAN = "boolean";
//Binary...
public static final String BINARY = "binary";
//Geo...
public static final String GEO_POINT = "geo_point";//41.12,-71.34 (Lat, Lon)
//Nested...
public static final String NESTED = "nested";
//Object...
public static final String OBJECT = "object";
}
/**
* Constructor to set the Id, Field Name, Value and Type.
*
* @param fieldIdParam Field Id.
* @param fieldNameParam Sets Field Name.
* @param fieldValueParam Sets Field Value.
* @param fieldTypeParam Sets Field Type.
*/
public Field(
Long fieldIdParam,
String fieldNameParam,
Object fieldValueParam,
Type fieldTypeParam
) {
this(fieldIdParam);
this.setFieldName(fieldNameParam);
this.setTypeAsEnum(fieldTypeParam);
this.setFieldValue(fieldValueParam);
}
/**
* Sets the Id associated with a Field.
*
* @param fieldIdParam Field Id.
*/
public Field(Long fieldIdParam) {
super();
this.setId(fieldIdParam);
}
/**
* Sets the Name associated with a Field.
*
* @param fieldNameParam Field Name.
*/
public Field(String fieldNameParam) {
super();
this.setFieldName(fieldNameParam);
}
/**
* Constructor to set the Field Name, Value and Type.
*
* @param fieldNameParam Sets Field Name.
* @param fieldValueParam Sets Field Value.
* @param fieldTypeParam Sets Field Type.
*/
public Field(String fieldNameParam, Object fieldValueParam, Type fieldTypeParam) {
this.setFieldName(fieldNameParam);
this.setTypeAsEnum(fieldTypeParam);
this.setFieldValue(fieldValueParam);
}
/**
* Constructor to set the Field Name, Value.
*
* @param fieldNameParam Sets Field Name.
* @param fieldValueParam Sets Field Value.
*/
public Field(String fieldNameParam, Object fieldValueParam) {
this.setFieldName(fieldNameParam);
this.fieldType = null;
this.typeMetaData = null;
this.setFieldValue(fieldValueParam);
}
/**
* Constructor to set the Field Name and {@code MultiChoice} Value.
*
* @param fieldNameParam Sets Field Name.
* @param multiChoiceParam Sets Field Value as {@code MultiChoice}.
*/
public Field(String fieldNameParam, MultiChoice multiChoiceParam) {
this.setFieldName(fieldNameParam);
this.setTypeAsEnum(Field.Type.MultipleChoice);
this.setFieldValue(multiChoiceParam);
}
/**
* Populates local variables with {@code jsonObjectParam}.
*
* @param jsonObjectParam The JSON Object.
*/
public Field(JSONObject jsonObjectParam) {
super(jsonObjectParam);
//Field Name...
if (!this.jsonObject.isNull(JSONMapping.FIELD_NAME)) {
this.setFieldName(this.jsonObject.getString(JSONMapping.FIELD_NAME));
}
//Field Description...
if (!this.jsonObject.isNull(JSONMapping.FIELD_DESCRIPTION)) {
this.setFieldDescription(this.jsonObject.getString(JSONMapping.FIELD_DESCRIPTION));
}
//Field Type...
if (!this.jsonObject.isNull(JSONMapping.FIELD_TYPE)) {
this.setFieldType(this.jsonObject.getString(JSONMapping.FIELD_TYPE));
}
//Meta Data...
if (!this.jsonObject.isNull(JSONMapping.TYPE_META_DATA)) {
this.setTypeMetaData(this.jsonObject.getString(JSONMapping.TYPE_META_DATA));
}
//Field Value...
if (!this.jsonObject.isNull(JSONMapping.FIELD_VALUE)) {
Object objFromKey = this.jsonObject.get(JSONMapping.FIELD_VALUE);
if (objFromKey instanceof JSONObject) {
JSONObject jsonObject = this.jsonObject.getJSONObject(JSONMapping.FIELD_VALUE);
//Multi Choices Selected Multi Choices...
if((jsonObject.has(MultiChoice.JSONMapping.SELECTED_MULTI_CHOICES) ||
jsonObject.has(MultiChoice.JSONMapping.SELECTED_CHOICES)) ||
jsonObject.has(MultiChoice.JSONMapping.SELECTED_CHOICES_COMBINED)) {
this.setFieldValue(new MultiChoice(jsonObject));
} else if (Type.MultipleChoice.toString().equals(this.getFieldType()) &&
(jsonObject.has(MultiChoice.JSONMapping.TYPE) && jsonObject.has(MultiChoice.JSONMapping.VALUE))) {
//[Payara] mapping for rest...
String typeVal = jsonObject.getString(MultiChoice.JSONMapping.TYPE);
if (MultiChoice.JSONMapping.TYPE_STRING.equals(typeVal)) {
this.setFieldValue(
new MultiChoice(
new JSONObject(jsonObject.getString(
MultiChoice.JSONMapping.VALUE))));
}
} else if (jsonObject.has(TableField.JSONMapping.TABLE_RECORDS)) {
//Table Field...
this.setFieldValue(new TableField(jsonObject));
}
} else if (objFromKey instanceof Long) {
Long castedLong = this.jsonObject.getLong(JSONMapping.FIELD_VALUE);
if (this.getTypeAsEnum() != null && this.getTypeAsEnum() == Type.DateTime) {
this.setFieldValue(new Date(castedLong));
} else {
this.setFieldValue(castedLong);
}
} else if (objFromKey instanceof Number) {
this.setFieldValue(this.jsonObject.getLong(JSONMapping.FIELD_VALUE));
} else if (objFromKey instanceof Boolean) {
this.setFieldValue(this.jsonObject.getBoolean(JSONMapping.FIELD_VALUE));
} else {
this.setFieldValue(this.jsonObject.getString(JSONMapping.FIELD_VALUE));
}
}
}
/**
* Default constructor.
*/
public Field() {
super();
}
/**
* Gets the name of {@code this} {@code Field}.
*
* @return The Field Name.
*/
public String getFieldName() {
return this.fieldName;
}
/**
* Sets the name of {@code this} {@code Field}.
*
* @param fieldNameParam The New Field Name.
*/
public void setFieldName(String fieldNameParam) {
this.fieldName = fieldNameParam;
}
/**
* Converts the {@code getFieldName} to upper_camel_case.
*
* @return {@code getFieldName()} as upper_camel_case.
*/
@XmlTransient
public String getFieldNameAsUpperCamel() {
return new UtilGlobal().toCamelUpperCase(this.getFieldName());
}
/**
* Gets the description of {@code this} {@code Field}.
*
* @return The Field Description.
*/
public String getFieldDescription() {
return this.fieldDescription;
}
/**
* Sets the description of {@code this} {@code Field}.
*
* @param fieldDescriptionParam The Field Description.
*/
public void setFieldDescription(String fieldDescriptionParam) {
this.fieldDescription = fieldDescriptionParam;
}
/**
* Gets the value of {@code this} {@code Field}.
*
* @return The Field Value.
*
* @see Type
*/
public Object getFieldValue() {
return this.fieldValue;
}
/**
* Gets the value of {@code this} {@code Field} as a {@code String}.
*
* @return The Field Value.
*
* @see Type
*/
@XmlTransient
public String getFieldValueAsString() {
Object returnObj = this.getFieldValue();
return (returnObj == null) ? null : returnObj.toString();
}
/**
* Gets the value of {@code this} {@code Field} as a {@code Double}.
*
* @return The Field Value.
*
* @see Type
*/
@XmlTransient
public Double getFieldValueAsDouble() {
Object obj = this.getFieldValue();
if (obj == null) {
return null;
}
if (obj instanceof Double) {
return (Double)obj;
}
if (obj instanceof Number) {
return ((Number)obj).doubleValue();
}
return null;
}
/**
* Gets the value of {@code this} {@code Field} as a {@code Long}.
*
* @return The Field Value.
*
* @see Type
*/
@XmlTransient
public Long getFieldValueAsLong() {
Object obj = this.getFieldValue();
if (obj == null) {
return null;
}
if (obj instanceof Long) {
return (Long)obj;
}
if (obj instanceof Number) {
return ((Number)obj).longValue();
}
return null;
}
/**
* Gets the value of {@code this} {@code Field} as a {@code Integer}.
*
* @return The Field Value.
*
* @see Type
*/
@XmlTransient
public Integer getFieldValueAsInteger() {
Object obj = this.getFieldValue();
if (obj == null) {
return null;
}
if (obj instanceof Integer) {
return (Integer)obj;
}
if (obj instanceof Number) {
return ((Number)obj).intValue();
}
return null;
}
/**
* Gets the value of {@code this} {@code Field} as a {@code Number}.
*
* @return The Field Value.
*
* @see Type
*/
@XmlTransient
public Number getFieldValueAsNumber() {
Object obj = this.getFieldValue();
if (obj == null) {
return null;
}
if (obj instanceof Number) {
return (Number)obj;
}
return null;
}
/**
* Gets the value of {@code this} {@code Field} as a {@code Boolean}.
*
* @return The Field Value.
*
* @see Type
*/
@XmlTransient
public Boolean getFieldValueAsBoolean() {
Object obj = this.getFieldValue();
if (obj == null) {
return null;
}
if (obj instanceof Boolean) {
return (Boolean)obj;
}
return null;
}
/**
* Gets the value of {@code this} {@code Field} as a {@code Date}.
*
* @return The Field Value.
*
* @see Type
*/
@XmlTransient
public Date getFieldValueAsDate() {
Object obj = this.getFieldValue();
if (obj == null) {
return null;
}
//Real Date...
if (obj instanceof Date) {
return (Date)obj;
} else if (obj instanceof Long) {
//Long...
Long longValue = (Long)obj;
if (longValue.longValue() > 0) {
return new Date(longValue.longValue());
}
}
return null;
}
/**
* Gets the value of {@code this} {@code Field} as a {@code MultiChoice}.
*
* @return The Field Value.
*
* @see Type
* @see MultiChoice
*/
@XmlTransient
public MultiChoice getFieldValueAsMultiChoice() {
Object obj = this.getFieldValue();
if (obj == null) {
return null;
}
if (obj instanceof MultiChoice) {
return (MultiChoice)obj;
}
return null;
}
/**
* Gets the value of {@code this} {@code Field} as a {@code TableField}.
*
* @return The Field Value.
*
* @see Type
* @see TableField
*/
@XmlTransient
public TableField getFieldValueAsTableField() {
Object obj = this.getFieldValue();
if (obj == null) {
return null;
}
if (obj instanceof TableField) {
return (TableField)obj;
}
return null;
}
/**
* Sets the value of {@code this} {@code Field}.
*
* @param fieldValueParam The New Field Value.
*
* @see Type
*/
public void setFieldValue(Object fieldValueParam) {
this.fieldValue = fieldValueParam;
if (this.getFieldType() == null && fieldValueParam != null) {
//Date...
if (fieldValueParam instanceof Date) {
this.setTypeAsEnum(Type.DateTime);
} else if (fieldValueParam instanceof Number) {
//Number...
this.setTypeAsEnum(Type.Decimal);
} else if (fieldValueParam instanceof MultiChoice) {
//MultiChoice...
this.setTypeAsEnum(Type.MultipleChoice);
} else if (fieldValueParam instanceof TableField) {
//Table Field...
this.setTypeAsEnum(Type.Table);
} else if (fieldValueParam instanceof String) {
//Text...
this.setTypeAsEnum(Type.Text);
} else if (fieldValueParam instanceof Boolean) {
//Boolean...
this.setTypeAsEnum(Type.TrueFalse);
}
}
}
/**
* Gets the Type of {@code this} {@code Field}.
*
* @return The Field Type.
*
* @see Type
*/
public String getFieldType() {
return this.fieldType;
}
/**
* Sets the Type of {@code this} {@code Field} as {@code String}.
*
* @param fieldTypeParam The Field fieldType.
*
* @see Type
*/
public void setFieldType(String fieldTypeParam) {
this.fieldType = fieldTypeParam;
}
/**
* Sets the Type of {@code this} {@code Field} as {@code enum}.
*
* @param typeParam The Field fieldType.
*
* @see Type
*/
@XmlTransient
public void setTypeAsEnum(Type typeParam) {
if (typeParam == null) {
this.fieldType = null;
return;
}
this.fieldType = typeParam.name();
}
/**
* Gets the Type of {@code this} {@code Field} as {@code enum}.
*
* @return The Field fieldType.
*
* @see Type
*/
@XmlTransient
public Type getTypeAsEnum() {
if (this.getFieldType() == null || this.getFieldType().trim().isEmpty()) {
return null;
}
return Type.valueOf(this.getFieldType());
}
/**
*
* Gets the Meta-Data of {@code this} {@code Field}.
*
*
* Also referred to as Field Type sub-data.
* This defines the {@code Field} even further.
*
*
* Example: Text field may be {@code Plain}, {@code Masked}, {@code Latitude and Longitude}.
*
* @return The Field Meta-Data.
*
* @see Type
*/
public String getTypeMetaData() {
return this.typeMetaData;
}
/**
*
* Gets the Meta-Data of {@code this} {@code Field}.
*
*
* Also referred to as Field Type sub-data.
* This defines the {@code Field} even further.
*
*
* Example: Text field may be {@code Plain}, {@code Masked}, {@code Latitude and Longitude}.
*
* @param typeMetaDataParam The Field Meta-Data.
*
* @see Type
*/
public void setTypeMetaData(String typeMetaDataParam) {
this.typeMetaData = typeMetaDataParam;
}
/**
* Conversion to {@code JSONObject} from Java Object.
*
* @return {@code JSONObject} representation of {@code Field}
* @throws JSONException If there is a problem with the JSON Body.
*
* @see ABaseFluidJSONObject#toJsonObject()
*/
@Override
@XmlTransient
public JSONObject toJsonObject() throws JSONException {
JSONObject returnVal = super.toJsonObject();
//Field Name...
if (this.getFieldName() != null) {
returnVal.put(JSONMapping.FIELD_NAME,this.getFieldName());
}
//Field Description...
if (this.getFieldDescription() != null) {
returnVal.put(JSONMapping.FIELD_DESCRIPTION,this.getFieldDescription());
}
//Field Value...
if (this.getFieldValue() != null) {
//Text...
if (this.getFieldValue() instanceof String) {
returnVal.put(JSONMapping.FIELD_VALUE, this.getFieldValue());
} else if (this.getFieldValue() instanceof Number) {
//Decimal...
returnVal.put(JSONMapping.FIELD_VALUE,
((Number)this.getFieldValue()).doubleValue());
} else if (this.getFieldValue() instanceof Boolean) {
//True False...
returnVal.put(JSONMapping.FIELD_VALUE,
(Boolean)this.getFieldValue());
} else if (this.getFieldValue() instanceof Date) {
//Date Time...
returnVal.put(JSONMapping.FIELD_VALUE,
this.getDateAsLongFromJson((Date)this.getFieldValue()));
} else if (this.getFieldValue() instanceof MultiChoice) {
//Multi Choice...
returnVal.put(JSONMapping.FIELD_VALUE,
((MultiChoice)this.getFieldValue()).toJsonObject());
} else if (this.getFieldValue() instanceof TableField) {
//Table Field...
returnVal.put(JSONMapping.FIELD_VALUE,
((TableField)this.getFieldValue()).toJsonObject());
} else {
returnVal.put(JSONMapping.FIELD_VALUE, this.getFieldValue());
}
}
//Type...
if (this.getFieldType() != null) {
returnVal.put(JSONMapping.FIELD_TYPE, this.getFieldType());
}
//Type Meta Data...
if (this.getTypeMetaData() != null) {
returnVal.put(JSONMapping.TYPE_META_DATA,this.getTypeMetaData());
}
return returnVal;
}
/**
* Creates the mapping object required by Elastic Search when making
* use of enhanced data-types.
*
* See {@code https://www.elastic.co/guide/en/elasticsearch/reference/current/mapping-types.html}.
*
* @return {@code JSONObject} representation of {@code Field} for
* ElasticSearch mapping.
* @throws JSONException If there is a problem with the JSON Body.
*/
@Override
@XmlTransient
public JSONObject toJsonMappingForElasticSearch() throws JSONException {
String fieldNameUpperCamel = this.getFieldNameAsUpperCamel();
if (fieldNameUpperCamel == null) {
return null;
}
String elasticType = this.getElasticSearchFieldType();
if (elasticType == null) {
return null;
}
JSONObject returnVal = new JSONObject();
returnVal.put(JSONMapping.Elastic.MAPPING_ONLY_TYPE, elasticType);
return returnVal;
}
/**
* Conversion to {@code JSONObject} for storage in ElasticSearch.
*
* @return {@code JSONObject} representation of {@code Field}
* @throws JSONException If there is a problem with the JSON Body.
*
* @see ABaseFluidJSONObject#toJsonObject()
*/
@Override
@XmlTransient
public JSONObject toJsonForElasticSearch() throws JSONException {
if (!this.doesFieldQualifyForElasticSearchInsert()) {
return null;
}
JSONObject returnVal = new JSONObject();
String fieldIdAsString = this.getFieldNameAsUpperCamel();
Object fieldValue = this.getFieldValue();
//Table Field...
if (fieldValue instanceof TableField) {
TableField tableField = (TableField)this.getFieldValue();
if (tableField.getTableRecords() != null && !tableField.getTableRecords().isEmpty()) {
JSONArray array = new JSONArray();
for (Form record : tableField.getTableRecords()) {
if (record.getId() == null) {
continue;
}
array.put(record.getId());
}
returnVal.put(fieldIdAsString, array);
}
} else if (fieldValue instanceof MultiChoice) {
//Multiple Choice...
MultiChoice multiChoice = (MultiChoice)this.getFieldValue();
if (multiChoice.getSelectedMultiChoices() != null &&
!multiChoice.getSelectedMultiChoices().isEmpty()) {
JSONArray array = new JSONArray();
for (String selectedChoice : multiChoice.getSelectedMultiChoices()) {
Long selectedChoiceAsLong = null;
try {
if (!selectedChoice.isEmpty() &&
Character.isDigit(selectedChoice.charAt(0))) {
selectedChoiceAsLong = Long.parseLong(selectedChoice);
}
} catch (NumberFormatException nfe) {
selectedChoiceAsLong = null;
}
//When not long, store as is...
if (selectedChoiceAsLong == null) {
array.put(selectedChoice);
} else {
array.put(selectedChoiceAsLong.longValue());
}
}
returnVal.put(fieldIdAsString, array);
}
} else if((fieldValue instanceof Number || fieldValue instanceof Boolean) ||
fieldValue instanceof String) {
//Other valid types...
if ((fieldValue instanceof String) &&
LATITUDE_AND_LONGITUDE.equals(this.getTypeMetaData())) {
String formFieldValueStr = fieldValue.toString();
UtilGlobal utilGlobal = new UtilGlobal();
String latitude = utilGlobal.getLatitudeFromFluidText(formFieldValueStr);
String longitude = utilGlobal.getLongitudeFromFluidText(formFieldValueStr);
fieldValue = (latitude.concat(UtilGlobal.COMMA).concat(longitude));
}
returnVal.put(fieldIdAsString, fieldValue);
} else if (fieldValue instanceof Date) {
//Date...
returnVal.put(fieldIdAsString, ((Date)fieldValue).getTime());
} else {
//Problem...
throw new FluidElasticSearchException(
"Field Value of field-type '"+fieldValue.getClass().getSimpleName()
+"' and Value '"+ fieldValue+"' is not supported.");
}
return returnVal;
}
/**
* Populate the object based on the ElasticSearch JSON structure.
*
* @param jsonObjectParam The JSON object to populate from.
* @return {@link Field} - The field to be added, if invalid a {@code null}
* will be returned.
*
* @throws JSONException If there is a problem with the JSON Body.
*
* @see ABaseFluidJSONObject#toJsonObject()
*/
@XmlTransient
public Field populateFromElasticSearchJson(
JSONObject jsonObjectParam
) throws JSONException {
if (this.getFieldNameAsUpperCamel() == null) {
return null;
}
String fieldIdAsString = this.getFieldNameAsUpperCamel();
if (jsonObjectParam.isNull(fieldIdAsString)) {
return null;
}
Field.Type type;
if((type = this.getTypeAsEnum()) == null) {
return null;
}
Object formFieldValue = jsonObjectParam.get(fieldIdAsString);
Field fieldToAdd = null;
switch (type) {
case DateTime:
if (formFieldValue instanceof Long) {
fieldToAdd = new Field(
this.getId(),
this.getFieldName(),
new Date(((Long)formFieldValue).longValue()),
type);
}
break;
case Decimal:
if (formFieldValue instanceof Number) {
fieldToAdd = new Field(
this.getId(),
this.getFieldName(),
((Number)formFieldValue).doubleValue(),
type);
}
break;
case MultipleChoice:
if (formFieldValue instanceof JSONArray) {
JSONArray casted = (JSONArray)formFieldValue;
List selectedChoices = new ArrayList();
for (int index = 0;index < casted.length();index++) {
selectedChoices.add(casted.get(index).toString());
}
if (selectedChoices.isEmpty()) {
return null;
}
MultiChoice multiChoiceToSet = new MultiChoice(selectedChoices);
fieldToAdd = new Field(
this.getId(),
this.getFieldName(),
multiChoiceToSet,
type);
}
break;
case Table:
List